Operators Precedence and Associativity in C Language
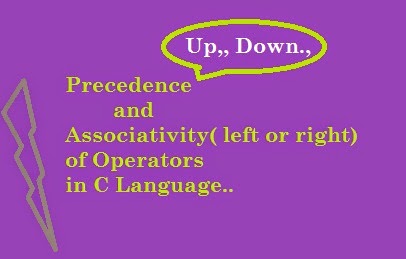
Introduction:
We have discussed about the Operators in C Programming language so far. But what if we have more than one operator in an expression. Then how to evaluate such kind of expressions. So to overcome this problem we have Operators Precedence and Associativity in C Language.
Operators Precedence and Associativity in C :
According to the language specification, Each and every Operator is given a precedence level. So Higher precedence operators are evaluated first after that the next priority or precedence level operators evaluated, so on until we reach finish the expression.
We have different precedence levels for different operators. and one precedence level can have multiple operators.
For example, Multiplication ( *), Division ( /) and Modulo division ( %) have the same precedence level.
So by using the Precedence of the Operators, We can decide which operator should be evaluated first, But what if we get the expression with more than one operator from the same precedence level?
If we get multiple operators from the Same precedence level, Then we use the Operators Associativity to determine which operation to do first.
If the Operators precedence is same, Then depending upon the operators associativity we will evaluate the expression. So Associativity is kind of tie-breaker (🎾) if Precedence is equal.
📢 If you get more than one operator from the same precedence level, Then use the Associativity to evaluate the expression
Operators Precedence and Associativity in C Table :
Level | Symbol | Description | Associativity |
---|---|---|---|
1 | ++ – – ( ) [ ] -> . | Prefix increment Prefix decrement Function call and subexpression Array subscript Structure pointer Structure member | left to right |
2 | ! ~ – + (type) * & sizeof | Logical negation ( Logical NOT ) 1’s complement Unary negation Unary plus Type cast Pointer dereference Address of Operator Sizeof Operator | right to left |
3 | * / % | Multiplication Division Modulus (integer remainder) | left to right |
4 | + – | Binary Addition Binary Subtraction | left to right |
5 | << >> | Bitwise left shift Bitwise right shift | left to right |
6 | < <= > >= | Less than Less than or equal to Greater than Greater than or equal to | left to right |
7 | == != | Equality Not equal | left to right |
8 | & | Bitwise AND | left to right |
9 | ^ | Bitwise exclusive OR (XOR) | left to right |
10 | | | Bitwise inclusive OR | left to right |
11 | && | Logical AND | left to right |
12 | || | Logical inclusive OR | left to right |
13 | ?: | Conditional Operator | right to left |
14 | = += -= *= /= %= <<= >>= &= ^= | | Assignment Compound Addition Compound subtraction Compound multiplication Compound division Compound modulus Compound bitwise left shift Compound bitwise right shift Compound bitwise AND Compound bitwise exclusive OR Compound bitwise OR | right to left |
15 | , ++ – – | Sequence point (list separator) Postfix increment Postfix decrement | left to right |
Example to deministrate Operators Precedence and Associativity:
For example, If you have a expression like below.
10 * 40 / 20 % 6
Here we have three Operators, Multiplication * , Division / and Modulo Division %.
So according to above Precedence and Associativity table, We can see these three operators have the same precedence level. So we need to depend on the Associativity.
The associativity of multiplication, Division, and Modulo division operators is from left to right. So we need to evaluate the above expression from left to right.
The first operation will be,
10 * 40 result is 400 and expression became 400 / 20 % 6
Now we need to evaluate 400 / 20which is equal to 20.
The expression became 20 / 6 , The result of this expression is 2.
The final result is 2.
For the 10 * 40 / 20 % 6 Expression, We got the result as 2. So Let’s write a C Program to verify the result.
Example Program : Precedence and Associativity in C Language:
1 2 3 4 5 6 7 |
#include<stdio.h> int main() { int j = 10 * 40 / 20 % 6; printf("j value is : %d \n", j); return 0; } |
Output:
1 |
j value is : 2 |
As you can see from the above output, The value of 10 * 40 / 20 % 6 Expression is 2.
So by using above Precedence and Associativity of Operators, We can evaluate any kind of complex expressions. If you are confused with difficult expressions, Please look at the Precedence and associativity table to evaluate expression.
4 Responses
[…] Precedence of Operators […]
[…] Precedence of Operators […]
[…] 📢 As we are presently looking at arithmetic operators, Below table contains only arithmetic operators priority and associativity. To know the full list of Operators Precedence and Associativity – Operators Precedence and Associativity in C Language – SillyCodes […]
[…] Increment Operator have Highest priority than all Binary Operators. Because Unary operators have higher priority than Binary operators. You can see Full priority table here C operators Priority table. […]