For loop in C language with Example programs
- Introduction:
- For loop in C Language:
- Syntax of the for loop in C language:
- Flow Diagram of For Loop in C Programming:
- How for Loop Work in C?
- Program to understand the For loop in C programming:
- Program Explanation: For Loop in C Step by Step walk-through:
- Example 2: For loop to generate multiplication table for given number:
- Exercise For loop Program:
- For loop without Initialization:
- Infinite Loops using the for loop in C language:
- Conclusion:
- Related Articles:
- Practice programs on Loops:
- Tutorials Index:
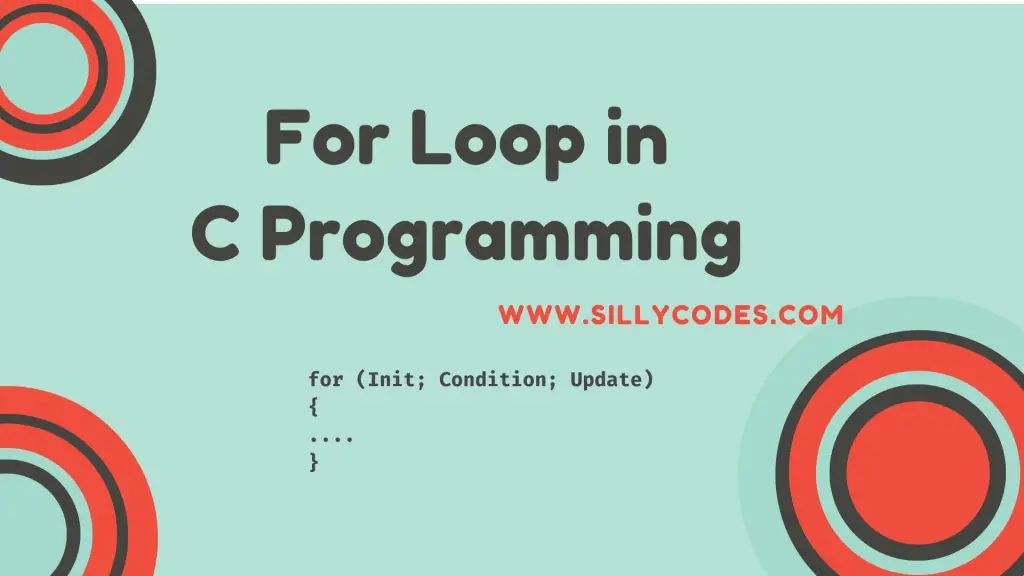
Introduction:
We have discussed the while loop in our earlier article, In today’s article, We will look at the for loop in C language with example programs.
For loop in C Language:
We use the for loop to iterate over a block of code multiple times. The loop will continue until the loop condition becomes false. The for loop is very similar to the while loop.
Here is the syntax of the for loop
Syntax of the for loop in C language:
1 2 3 4 5 |
for( initialization; condition; update) { // Inside the for loop .... .... } |
Flow Diagram of For Loop in C Programming:
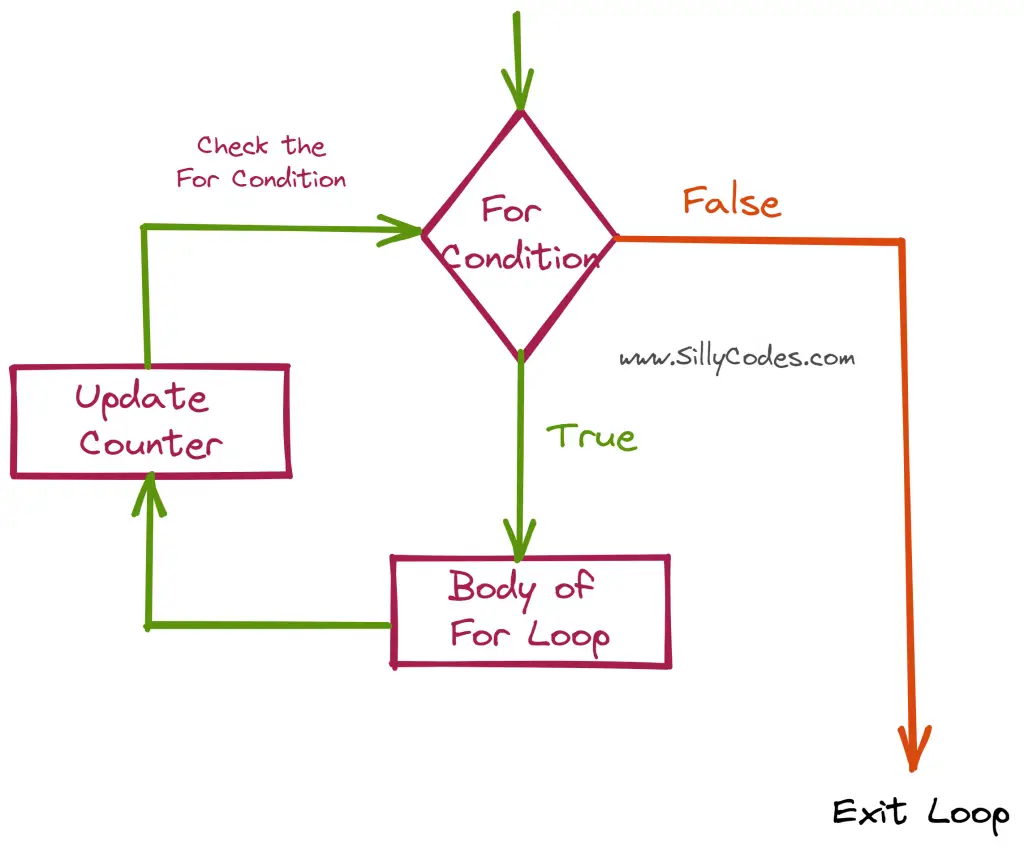
How for Loop Work in C?
- The for loop execution starts with the Initialization statement.
- The Initialization statement is used to initialize any variables and It is going to execute only once.
- Once the Initialization is completed, the Condition is evaluated.
- If the Condition returns True, Then the body of the For loop will be executed.
- Once the body of the for loop is executed, For loop will execute the update statement, Where we usually increment or decrement our control variable.
- Once the Update step is completed, For loop will check the Condition again, If it is evaluated to True, the body of the for will be executed, followed by the update statement.
- The above step will continue until the Condition returns False, Once the Condition becomes False, Then for loop will terminate and program control comes out of the for loop.
📢 The Initialization statement is executed only once, And it is an Optional statement. You can skip it but make sure to include the semicolon (;), We will look at one example about it in the following sections.
📢 The Update step is very important, So make sure to update your loop control variable appropriately to avoid infinite loops.
Program to understand the For loop in C programming:
Let’s look at an example program to understand the for loop in C Language
We will write a program to print the odd numbers from 1 to 10 using the for loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include<stdio.h> int main() { // We will use `i` as the counter int i; /* 1. Initialization: 'i = 1', We set our counter - i = 1 2. Condition: 'i<=10', This is our loop condition - loop will continue until this becomes false 3. Update : 'i++', Our loop control variable update statement */ for( i = 1; i<=10; i++) { // check if the `i` is Odd number using modulus if (i%2 == 1) { printf("%d ", i); } } // New line printf("\n"); return 0; } |
Save the program as the for-loop.c and build and run it.
For loop Example Program Output:
1 2 3 4 |
$ gcc for-loop.c $ ./a.out 1 3 5 7 9 $ |
Program Explanation: For Loop in C Step by Step walk-through:
The for loop has three main steps
- Initialization: We have initialized our loop control variable i = 1
- Condition: This is our loop condition, We need to find the odd numbers up to 10, So we set our condition as i ≤ 10, The for loop will continue until loop condition becomes False
- Update: This is where we update our loop control variable i. We are updating our control variable i by 1 at each iteration using the post-increment operation – i++.
Let’s look at the step-by-step walk-through:
First Iteration:
At the start,
- Initialization:
- The loop control variable i is initialized with value of 1,
- Check Condition:
- The value of i is 1 so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 1, So it is an Odd number. So the number will be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 2
Output so far: 1
Second Iteration:
📢 The initialization step won’t be executed. It will be executed only once (at the start of the for loop).
- Check Condition:
- The value of i is 2 so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True, the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 2, So it is not an Odd number. So the number won’t be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 3.
Output so far: 1
Third Iteration:
- Check Condition:
- The value of i is 3 so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True. The body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 3, So it is an Odd number. So the number will be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 4
Output so far: 1 3
Fourth Iteration:
- Check Condition:
- The value of i is 4. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True, the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 4, So it is not an Odd number. So the number won’t be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 5.
Output so far: 1 3
Fifth Iteration:
- Check Condition:
- The value of i is 5. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True. The body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 5, So it is an Odd number. So the number will be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 6.
Output so far: 1 3 5
Sixth Iteration:
- Check Condition:
- The value of i is 6. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True, the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 6, So it is not an Odd number. So the number won’t be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 7.
Output so far: 1 3 5
Seventh Iteration:
- Check Condition:
- The value of i is 7. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True. The body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 7, So it is an Odd number. So the number will be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 8.
Output so far: 1 3 5 7
Eighth Iteration:
- Check Condition:
- The value of i is 8. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True, the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 8, So it is not an Odd number. So the number won’t be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 9.
Output so far: 1 3 5 7
Ninth Iteration:
- Check Condition:
- The value of i is 9. so The for loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True. The body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 9, So it is an Odd number. So the number will be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 10.
Output so far: 1 3 5 7 9
Tenth Iteration:
- Check Condition:
- The value of i is 10. so The for-loop condition i ≤ 10 is evaluated as True
- Execute Body:
- As the condition is evaluated as True, the body of for loop is executed.
- Which checks if i is an odd number using the modulus operator and prints i variable if it Odd number, Our i present value is 10, So it is not an Odd number. So the number won’t be printed on the console.
- Update:
- After that, We need to update the control variable, We are increasing the i by 1, So our i variable becomes 11.
Output so far: 1 3 5 7 9
Eleventh Iteration:
- Check Condition:
- The value of i is 11. so The for loop condition i ≤ 10 is evaluated as False
As the for loop condition is evaluated as False, The for loop terminates, and program control comes out of the loop.
Final Output : 1 3 5 7 9
Example 2: For loop to generate multiplication table for given number:
Let’s write another program to make our understanding concrete.
We will write a program to generate the multiplication table. The program will ask for a number and generates the multiplication table for the given number up to 10.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* Program : Generate Multiplication Table using for loop */ #include<stdio.h> int main() { // We will use `i` as the counter int i; int num; // Take the input from the user and store in 'num' printf("Enter number to generate the table: "); scanf("%d", &num); printf("Multiplication Table of %d: \n", num); /* 1. Initialization: 'i = 1', We set our counter 'i' to 1 2. Condition: 'i<=10', This is our loop condition - loop will continue until this becomes false 3. Update : 'i++', Our loop control variable update statement */ for (i = 1; i<= 10; i++) { // Create the table printf("%d * %d = %d\n", num, i, num * i); } return 0; } |
For loop Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
$ gcc for-table.c $ $ ./a.out Enter number to generate the table: 5 Multiplication Table of 5: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 5 * 6 = 30 5 * 7 = 35 5 * 8 = 40 5 * 9 = 45 5 * 10 = 50 $ ./a.out Enter number to generate the table: 10 Multiplication Table of 10: 10 * 1 = 10 10 * 2 = 20 10 * 3 = 30 10 * 4 = 40 10 * 5 = 50 10 * 6 = 60 10 * 7 = 70 10 * 8 = 80 10 * 9 = 90 10 * 10 = 100 $ ./a.out Enter number to generate the table: 7 Multiplication Table of 7: 7 * 1 = 7 7 * 2 = 14 7 * 3 = 21 7 * 4 = 28 7 * 5 = 35 7 * 6 = 42 7 * 7 = 49 7 * 8 = 56 7 * 9 = 63 7 * 10 = 70 $ |
Multiplication Table Program Explanation:
The program started with asking the user for an input number and we are storing that number in num variable.
We also created a counter variable i
As we need to print the table up to 10 times, We decided to use the for loop
- We initialized the counter variable i to 1.
- Our condition is i ≤ 10 , Which means for loop will continue until i value reaches greater than 10
- We are updating the control variable i by 1 at each iteration.
So the above for loop iterates 10 times and at each iteration prints one line (by multiplying i and num) on to the console. Thus generating the multiplication table up to 10.
Exercise For loop Program:
The above program, by default, generates the table up to 10 numbers. Re-Write the above multiplication table program to generate the table up to the user-given number.
You need to take two numbers from the user.
Expected Output:
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc for-table.c $ ./a.out Enter number to generate the table: 5 Enter the limit: 5 Multiplication Table of 5: 5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25 $ |
For loop without Initialization:
We usually see many programs without the for loop initialization statement. Sometimes you already have the desired value ready, so you don’t need to initialize again.
Here is a program to print numbers from 1 to 10.
1 2 3 4 5 6 7 8 |
#include<stdio.h> int main() { int counter = 1; for(; counter <=10; counter++) { printf("%d ", counter); } printf("\n"); } |
Program Output:
1 2 3 4 |
$ gcc for-loop-3.c $ ./a.out 1 2 3 4 5 6 7 8 9 10 $ |
As you can see we not initialized the counter at the start of for loop.
📢 All three statements of the for loop are optional.
Infinite Loops using the for loop in C language:
As we have discussed in our previous article, If you forgot to update the loop control variable properly, Then there will be a good chance of creating infinite loops.
Here is an example of an unintentional infinite loop.
Let’s say, We want to print the numbers from 1 to 5 on the console. but we forgot to increment the counter, Then it can create the infinite loop.
1 2 3 4 5 6 7 8 |
#include<stdio.h> int main() { int counter = 1; for(; counter <=5; ) { printf("%d ", counter); } printf("\n"); } |
Infinite for loop output:
1 2 3 4 5 |
$ ./a.out 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 ..... CTRL + C $ |
Note: Press the CTRL + C to stop the Infinite loop( In Linux and Mac). Close the output window if you are on Windows PC.
We missed updating the loop control variable counter in the above example. To Avoid Infinite loops, We need to check loop Condition Properly, and Update the Loop Control variable (i.e Counter)
Infinite loop with for loop:
All Three options of the for loop are optional, So you can create an Infinite loop by not specifying any options like below.
The above code will generate the Infinite Loop.
📢 We still need to specify the semicolons (;) , Otherwise the compiler will throw an error.
Conclusion:
We have discussed about the For loop in C programming language with example programs. We also looked at the step-by-step walk-through of the for loop. Finally, we briefly covered Infinite loops using the for loop. In the following article, We will learn about the do while loop in C Language.
Related Articles:
- While loop in C with Example Programs
- Do while Loop in C language with Example Programs
- Infinite Loops in C Language – How to Create and Avoid Infinite Loops
- Break Statement in C Language
- Continue Statement in C
- Goto Statement in C Langauge
- if statement and if..else statement in C
- Nested if..else and If..else Ladder in C
- Switch Statement in C
50 Responses
[…] For Loop in C Language with Example Programs […]
[…] have discussed about the while loop and for loop in c language in our earlier articles, In today’s article, We are going to look at the do […]
[…] have looked at the while loop, for loop, and do while loop in our earlier articles, In today’s article we will learn about the break […]
[…] for loop […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C Language with Example Programs […]
[…] a program to Count the number of digits in C language using loops (while and for, etc). The program should accept a positive number from the user and count the number of individual […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C Language with Example Programs […]
[…] a Program to print first n prime numbers in C programming language. We are going to use two for loops (Nested Loops) to calculate the first n prime numbers. The program is going to accept a positive […]
[…] For Loop in C with Examples […]
[…] For Loop in C with Examples […]
[…] For Loop […]
[…] For Loop in C Language with Example Programs […]
[…] For Loop in C […]
[…] is recommended to have the basic knowledge of the C Loops like while loop and for loop and goto statement. As we are going to use them in this […]
[…] For Loop in C Language with Example Programs […]
[…] Loops in C Language – While Loop, For loop […]
[…] For Loop in C […]
[…] of the first n natural numbers. We need to use the recursion and should not use the C Loops ( like for, while, and do-while) to calculate the […]
[…] we need to find any element in the array, Then we need to traverse the array using a C loop (for loop, while loop, and do while […]
[…] another for loop to iterate over the array. The loop starts from Zero and goes till […]
[…] a For loop to Iterate through the array and update the array elements using the scanf […]
[…] input from the user for the array’s elements, and update the numbers array. Use a For Loop to iterate over the elements and update […]
[…] To Merge the two arrays, We will go through the all elements of the first array ( arr1) and copy them the mergedArr element by element using a For Loop. Once the first array is copied to mergedArr. Then we will go through the second input array( arr2), and Appends the arr2 elements to the mergedArr using another For Loop. […]
[…] Iterate over the original array from i=0 to i<size using a For Loop […]
[…] Iterate over the original array from i=0 to i<size using a For Loop […]
[…] a For loop to iterate over the array element and update numbers[i] and […]
[…] Read user input, We need to create two For Loops, One For Loop will iterate over the ROWS, and the Second For Loop will iterate over the […]
[…] Prompt the user to provide the array elements. Use a For Loop to iterate over the array elements and update […]
[…] function uses two For loops to iterate over the given matrix A and updates its elements by using the scanf function – […]
[…] the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] a For loop to Iterate through the array and update the array elements using the scanf […]
[…] the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the […]
[…] over the Second String( str2) and Append elements to str1: Start a For loop to iterate over the str2 and append characters of the str2 to first string str1. As we are appending to str1, The […]
[…] a For Loop start from i=0 go till the terminating NULL character. ( for(i = 0; inputStr[i]; i++) […]
[…] Iterate over the string and compare the characters pointed by the start and end indices. […]
[…] a For loop to Iterate over the string. Start the loop from i=0 and go till the terminating NULL character. i.e […]
[…] a For loop by initializing variable i with 0. It will continue looping as long as the current character ( […]
[…] function Iterates over the Original String using a For Loop and Copy elements from source string to destination […]
[…] Now, The random array is stored in the randPtr. Iterate over it and display the contents on the console using a for loop. […]
[…] Print the array elements by iterating over the ptr using a for loop. […]
[…] it will continue and prompt the user to provide the values for each integer variable using a for loop and store the values in the allocated memory. We then printed the integer variable on the console […]