Pointer Arithmetic in C Language
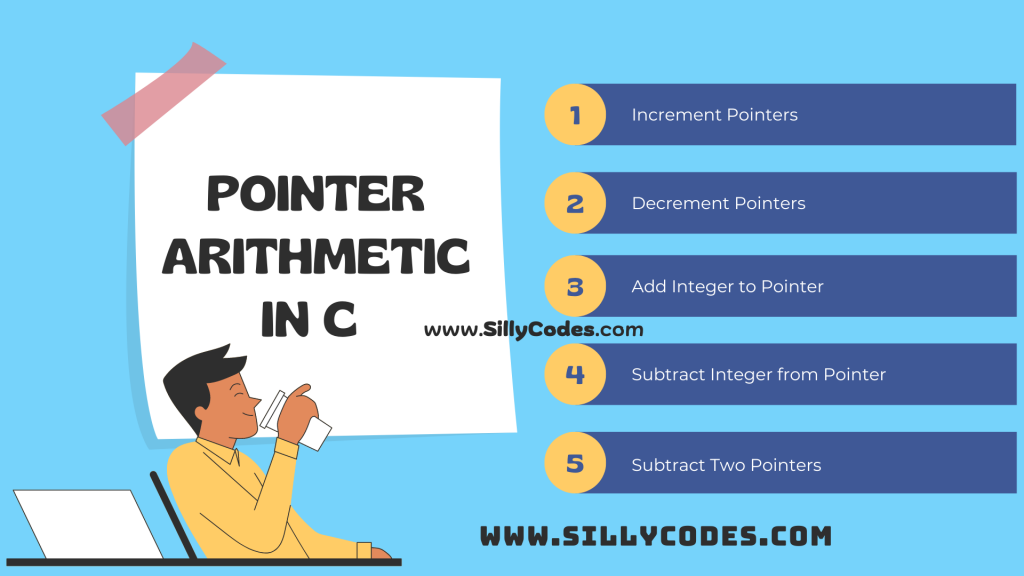
Introduction to Pointer Arithmetic in C:
We have looked at the Introductions to pointers in our previous article, In today’s article we will look at pointer arithmetic in c programming language. and what arithmetic operations we can perform on pointers with example programs.
Pointer Arithmetic in C Programming:
As pointers store the memory address of another variable. So We can’t perform all arithmetic operations on the pointer similar to the other normal variables.
The valid operations on pointers are
- Incrementing the Pointer variable
- Decrementing the pointer variable
- Adding an Integer to Pointer
- Subtracting an Integer from Pointers
- Subtraction of Pointer variable from another pointer variable
- Finally, We can also perform a Comparison of two pointer variables. ( Like == , !=, <, >, ≤ , and >= )
We can’t perform the below operations on the pointers. Invalid Operations on Pointer variables are
- Addition of Two Pointers
- Multiplication of Two Pointers
- Division of Two Pointers
Let’s look at the above pointer arithmetic in C language in detail.
Incrementing Pointer in C Language:
Let’s say we have a pointer variable called intPtr, which is an integer pointer and it is pointing to the memory address of the num integer variable.
1 2 3 |
int num = 100; Â int *intPtr = # |
Let’s say the memory address of the num is equal to 0x1000 also the size of the integer variable is 4 Bytes.
1 2 |
// increment 'intPtr' intPtr++; |
In General, The increment operation increases the value of the variable by one. Similarly, As the pointer variable contains the memory address, incrementing it increases the memory address value by N bytes. Where N is the size of the pointer variable data type.
In the above case, Incrementing the intPtrcause the pointer variable to point to the memory address 0x1004. This is because the pointer datatype is of integer type, and the size of an integer is 4 bytes.
📢Incrementing the integer pointer increases the pointer value by
4 Bytes
Before the increment operation pointer value is
0x1000
intPtr++;
After the increment operation pointer value is 0x1004
Let’s look at another example to understand the pointer increment operation. Incrementing the character pointer variable.
1 2 3 |
char ch = 'v'; Â char *chPtr = &ch; |
Here we have created a character ch and character pointer chPtr and the chPtr is holding the address of the ch variable.
Let’s say the address of the ch variable is 0x9990, Then chPtr is currently holding the 0x9990.
1 2 |
// increment the 'chPtr' chPtr++; |
If we increment the chPtrpointer variable, Then the chPtrpointer value will be raised by the number of bytes equal to the character size. As the character size is 1 Byte, So the pointer value will be equal to 0x9991 after the increment operation.
📢Incrementing the character pointer increases the pointer value by 1 Byte
Before the increment operation pointer value is 0x9990
chPtr++;
After the increment operation pointer value is 0x9991
📢The increment operation on the pointer variable increases the pointer value by the number of bytes of pointers datatype.
Program to understand the Increment pointer in c:
The following program demonstrates the behavior of pointer increment operation in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program to understand pointer increment operation     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int num = 100;     // create a pointer variable 'intPtr'     int *intPtr = #      char ch = 'v';     char *chPtr = &ch;       // value of integer pointer variable.     printf("Before Increment: Integer pointer(intPtr): %p\n", intPtr);      // increment the integer pointer     intPtr++;      printf("After  Increment: Integer pointer(intPtr): %p\n", intPtr);      // value of character pointer     printf("Before Increment: Character pointer(chPtr): %p\n", chPtr);      // increment character pointer     chPtr++;      printf("After  Increment: Character pointer(chPtr): %p\n", chPtr);      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 |
$ gcc pointer-increment.c $ ./a.out Before Increment: Integer pointer(intPtr): 0x7fff143c9e04 After  Increment: Integer pointer(intPtr): 0x7fff143c9e08 Before Increment: Character pointer(chPtr): 0x7fff143c9e03 After  Increment: Character pointer(chPtr): 0x7fff143c9e04 $ |
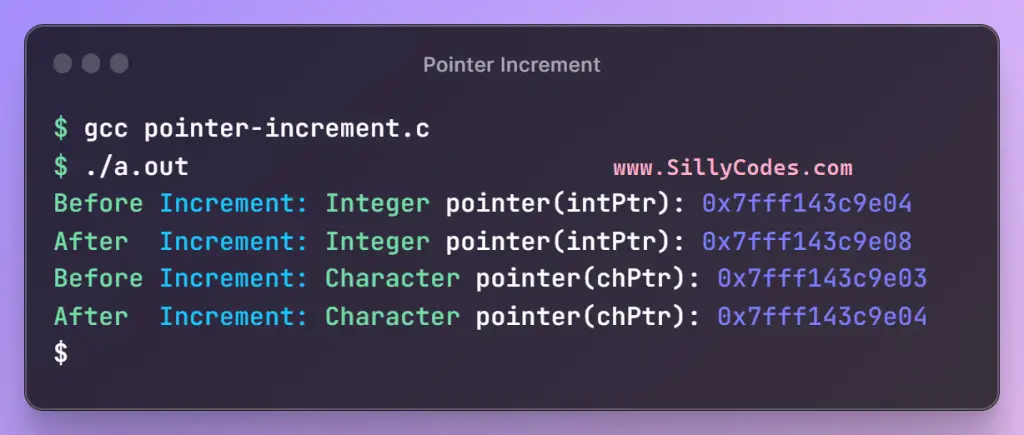
Pointer Increment Program Explanation:
In the above program, We have created an integer variable num and integer pointer intPtr. We also created the character variable ch and character pointer chPtr.
- The integer pointer
intPtr is holding the address of
num
- The initial value of the intPtr is 0x7fff143c9e04
- Then we performed the increment operation – intPtr++;
- The value of the integer pointer is increased by 4 bytes and The intPtr value after the increment operation is 0x7fff143c9e08. If you observe the memory addresses the value is increased by 4 Bytes ( which is size of the integer in my present machine)
- Similarly, The character pointer
chPtr is storing the address of
ch.
- Initial value of the chPtris 0x7fff143c9e03
- Then we incremented the chPtr by 1 – chPtr++;
- The resultant value of the chPtr is 0x7fff143c9e04, As the character size is equal to 1 Byte, The character pointer value is increased by 1 Byte due to the increment operation
Decrementing a pointer variable in C – Pointer arithmetic in c:
The decrementing the pointer variable will decrease the value of the pointer by N Bytes ( where N is the size of the pointer datatype)
This is very similar to the above pointer increment operation. Let’s look at an example.
1 2 3 4 |
int price = 50; Â // create a pointer variable int pricePtr = &price; |
Let’s say the value of the pricePtris equal to 0x4450, which means the address of the price variable is 0x4450
Decrement the pricePtr pointer variable.
1 2 |
// decrement the pointer pricePtr--; |
By decrementing the pointer variable pricePtr, The value of the pointer will be reduced by the 4 Bytes,( As the size of the int is 4 Bytes).
So the pricePtr will contain the value 0x4446 after the decrement operation.
Example to demonstrate the pointer decrement operation:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program to understand pointer Decrement operation     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int price = 100;     // create a pointer variable 'pricePtr'     int *pricePtr = &price;      char c = 'B';     char *cPtr = &c;       // value of integer pointer variable.     printf("Before Decrement: Integer pointer(pricePtr): %p\n", pricePtr);      // Decrement the integer pointer     pricePtr--;      printf("After  Decrement: Integer pointer(pricePtr): %p\n", pricePtr);      // value of character pointer     printf("Before Decrement: Character pointer(cPtr): %p\n", cPtr);      // Decrement character pointer     cPtr--;      printf("After  Decrement: Character pointer(cPtr): %p\n", cPtr);      return 0; } |
Program Output:
Let’s compile and run the above program.
1 2 3 4 5 6 7 |
$ gcc decrement-pointer.c $ ./a.out Before Decrement: Integer pointer(pricePtr): 0x7ffd08d7c7c4 After  Decrement: Integer pointer(pricePtr): 0x7ffd08d7c7c0 Before Decrement: Character pointer(cPtr): 0x7ffd08d7c7c3 After  Decrement: Character pointer(cPtr): 0x7ffd08d7c7c2 $ |
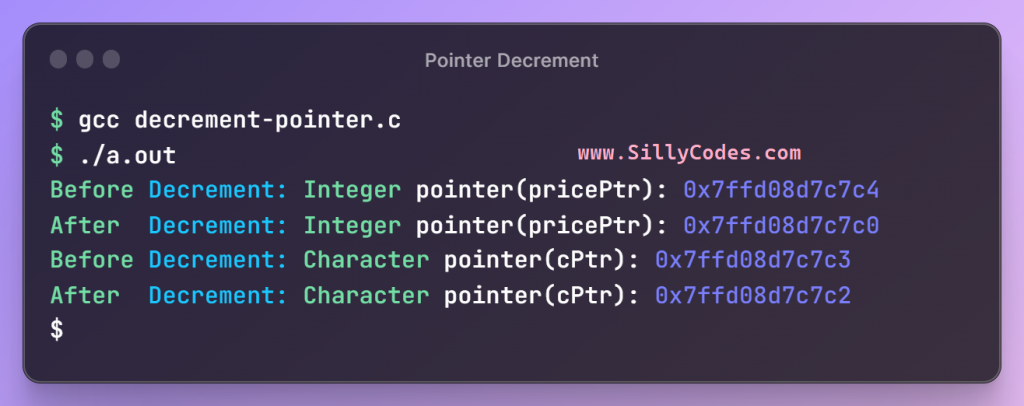
As we can see from the above output, The pointer decrement operation decreased the integer pointer( pricePtr) value by 4 Bytes ( 0x7ffd08d7c7c4 to 0x7ffd08d7c7c0)
Similarly, Decrementing the character pointer reduced the character pointer( cPtr) value by 1 Byte ( 0x7ffd08d7c7c3 to 0x7ffd08d7c7c2)
📢We use the pointer increment and decrement operators heavily to traverse the Arrays and strings in C.
Adding an integer to a pointer in c language:
We can add an integer or number to a pointer variable. Incrementing the pointer variable once increased the pointer value by N bytes ( where N is the size of the pointer datatype)
So if we add an integer or number M to the pointer, It will increase the pointer value by M * N Bytes. ( where N is the size of the pointer datatype).
Let’s look at an example.
1 2 3 4 5 6 |
int age = 25; Â int agePtr = &age; Â // Add integer 2 to pointer agePtr + 2 |
In the above example, We created an integer variable age and a pointer variable agePtr. The agePtr is storing the address of the age variable. Then we added an integer 2 to the agePtr pointer variable.
Let’s say the address of the age integer variable is 0x5000 , So the agePtr is holding the 0x5000 as the value.
If we add the integer 2 to the agePtr, It will increase the agePtr value by 8 Bytes. ( 2 * Size of Integer which is 2 * 4 )
Similarly, If we add 5 to agePtr, It will increase the agePtr value by 20 Bytes.
Adding an Integer to Pointer in C Program:
Let’s look at a program to understand how Adding an Integer to a pointer works in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/*     Program to adding integer to pointer in c     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int age = 25;     // create a pointer variable 'agePtr'     int *agePtr = &age;      // value of integer pointer variable.     printf("Before Addition: Integer pointer(agePtr): %p\n", agePtr);      // Addition the integer pointer     printf("After  Addition: Integer pointer(agePtr): %p\n", agePtr + 2);      return 0; } |
Save the above program as pointer-addition.c
Program Output:
Compile and Run the above pointer-addition.c program using GCC compiler (Any compiler)
1 2 3 4 5 |
$ gcc pointer-addition.c $ ./a.out Before Addition: Integer pointer(agePtr): 0x7ffe2b30d60c After  Addition: Integer pointer(agePtr): 0x7ffe2b30d614 $ |
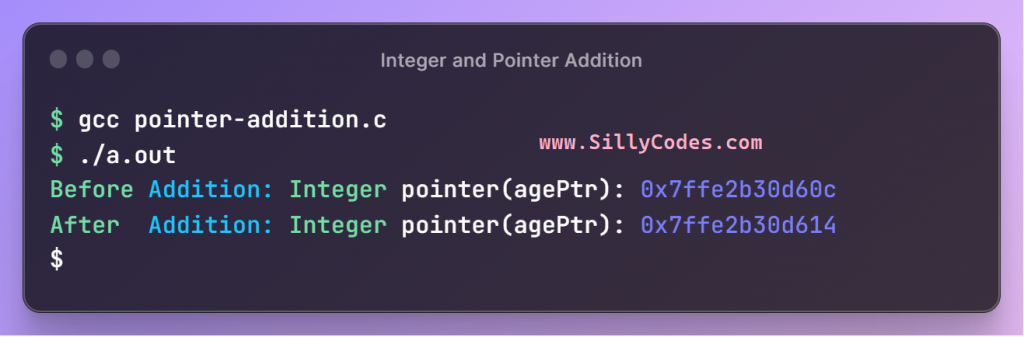
If you notice, The agePtr value is increased by the 8 Bytes. As the above addresses are in hexadecimal values, We need to convert them to decimal values to see the difference.
0C is equal to 12.
14 is equal to 20.
As we can see, The agePtr value is increased by 8 Bytes.
Subtracting an Integer from a Pointer variable in C:
Similar to the above integer and pointer addition operation, Subtracting an integer M from the pointer will reduce the pointer value by M * N Bytes. ( where N is the size of the pointer datatype).
So if you remove 3 from the integer pointer, It will reduce the pointer value by 12 Bytes
📢Adding or subtracting an integer from a pointer results in a pointer variable.
Here is the program to understand the pointer and the integer subtraction operation
Program to demonstrate Pointer and Integer Subtraction Operation in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/*     Program to understand pointer Subtraction operation     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int sub = 25;     // create a pointer variable 'subPtr'     int *subPtr = ⊂      // value of integer pointer variable.     printf("Before Subtraction: Integer pointer(subPtr): %p\n", subPtr);      // Subtraction the integer pointer     printf("After  Subtraction: Integer pointer(subPtr): %p\n", subPtr - 3);      return 0; } |
Program Output:
Compile the program
$ gcc pointer-subtraction.c
Run the program.
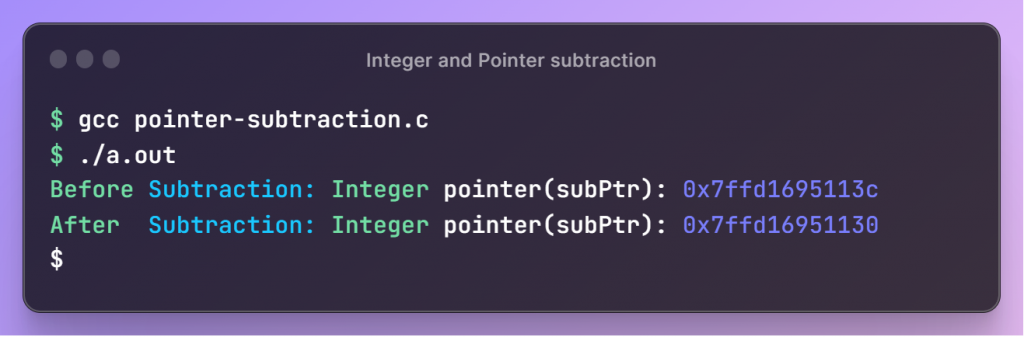
In the above program, We subtracted the number 3 from the integer pointer subPtr. So the value of the integer pointer is reduced by 12 Bytes. ( 3 * 4 = 12 ) .
Subtract Two Pointers in C language:
C Programming language allows us to subtract two pointers of the same data type. The subtraction of pointers will give us how far two pointers are located in the memory. Often pointer subtraction is used to calculate the number of elements(of the same type) between the two memory addresses.
For example, If an integer pointer( ptr1) is pointing to the memory address 0x1008 and another integer pointer( ptr2) is pointing to 0x1000, Then the subtraction of pointers ptr1 - ptr2 will return value 2. which is equal to the 2 integer places ( assuming integer size as 4 Bytes).
📢Subtraction of two pointers results in an integer value ( long int)
Program to Subtract Two Pointers in C Programming:
Let’s look at the example program to subtract two pointers in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/*     Program to subtract two pointers in c     sillycodes.com */  #include<stdio.h>  int main() {     // create two variables     int n1 = 10;     int n2 = 20;      // create two pointer     int *ptr1 = &n1;     int *ptr2 = &n2;      // display the values of two pointers     printf("ptr1: %p\n", ptr1);     printf("ptr2: %p\n", ptr2);      // subtract two pointers     printf("Subtraction of ptr2 and ptr1(ptr2-ptr1)  is: %ld\n", ptr2-ptr1);         return 0; } |
Program Output:
Let’s compile and Run the program and observe the output.
1 2 3 4 5 6 |
$ gcc pointer-subtraction.c $ ./a.out ptr1: 0x7fff1821f3c0 ptr2: 0x7fff1821f3c4 Subtraction of ptr2 and ptr1(ptr2-ptr1)Â Â is: 1 $ |
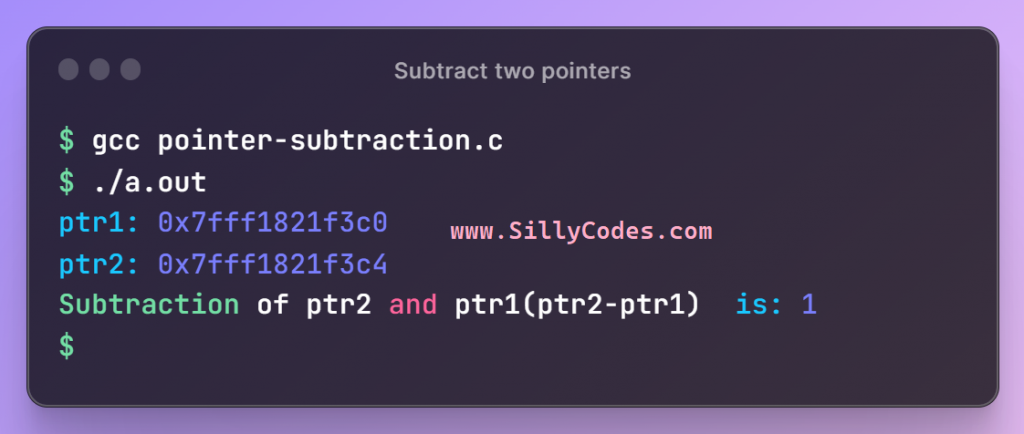
As we can see from the above output, The ptr2 value is 0x7fff1821f3c4 and ptr1 value is 0x7fff1821f3c0. So the subtraction of two pointers ptr2-ptr1 is 1. ( As the ptr2 and ptr1 are integer pointers)
Comparison of Two Pointers in C language:
As we specified earlier, The c programming language supports the comparison of two pointer variables.
The allowed comparison operations are
- Equality Operator==
- Not Equal(Inequality) Operator !=
- Less than Operator <
- Greater than Operator >
- Less than and Equal operator <=
- Greater than an equal operator >=
Let’s look at a program to check if two pointers are equal.
C Program to Check Two Pointers are Equal:
The following program compares pointer variables and displays the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
/*     Program to check two pointers are equal     sillycodes.com */  #include<stdio.h>  int main() {     // create two variables     int n1 = 10;     int n2 = 20;      // create three pointers     int *ptr1 = &n1;     int *ptr2 = &n2;      int *ptr3 = &n1;      // display the values of two pointers     printf("ptr1: %p\n", ptr1);     printf("ptr2: %p\n", ptr2);     printf("ptr3: %p\n", ptr3);          // check if two pointers 'ptr1' and 'ptr2' are equal     if(ptr1 == ptr2)     {         // Equal         printf("Pointers 'ptr1' and 'ptr2' are Equal\n");     }     else     {         // Not Equal         printf("Pointers 'ptr1' and 'ptr2' are Not Equal\n");     }       // check if two pointers 'ptr1' and 'ptr3' are equal     if(ptr1 == ptr3)     {         printf("Pointers 'ptr1' and 'ptr3' are Equal\n");     }     else     {         printf("Pointers 'ptr1' and 'ptr3' are Not Equal\n");     }      return 0; } |
We created three-pointers in the above program and stored the addresses of the two numbers. The ptr1 and ptr3 storing the address of the n1 variable and the ptr2 is storing the address of the n2 variable. Finally, We compared the pointer variables i.e Compared the pointers ptr2 and ptr3 with the pointer ptr1.
Program Output:
Compile and Run the above C Program.
1 2 3 4 5 6 7 8 |
$ gcc pointers-equal.c $ ./a.out ptr1: 0x7ffe83c59518 ptr2: 0x7ffe83c5951c ptr3: 0x7ffe83c59518 Pointers 'ptr1' and 'ptr2' are Not Equal Pointers 'ptr1' and 'ptr3' are Equal $ |
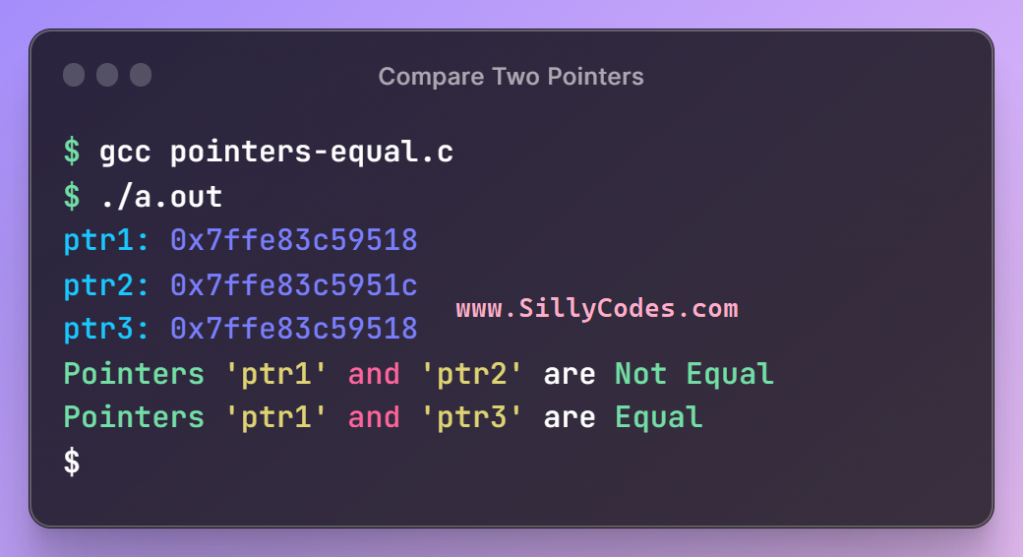
As we can see from the above output, The pointers ptr1 and ptr2 are pointing to different variables so their memory address is different. So the ptr1 and ptr2 are not equal.
Similarly, The ptr1 and ptr2 are pointing to the same variable n1, So they are equal.
Pointer Arithmetic in C – Conclusion:
In this article, We have looked at the pointer arithmetic in detail. We looked at the incrementing and decrementing pointers in C, We then discussed how to compare pointers, as well as how to add and subtract integer values from pointers.
2 Responses
[…] have looked at the introduction to pointers, and pointer arithmetics in earlier articles. In today’s article, We will look at the pointer to pointer in c language or […]
[…] Pointer Arithmetic in C Language […]