Program to Swap Two Numbers | Swapping Program in C language
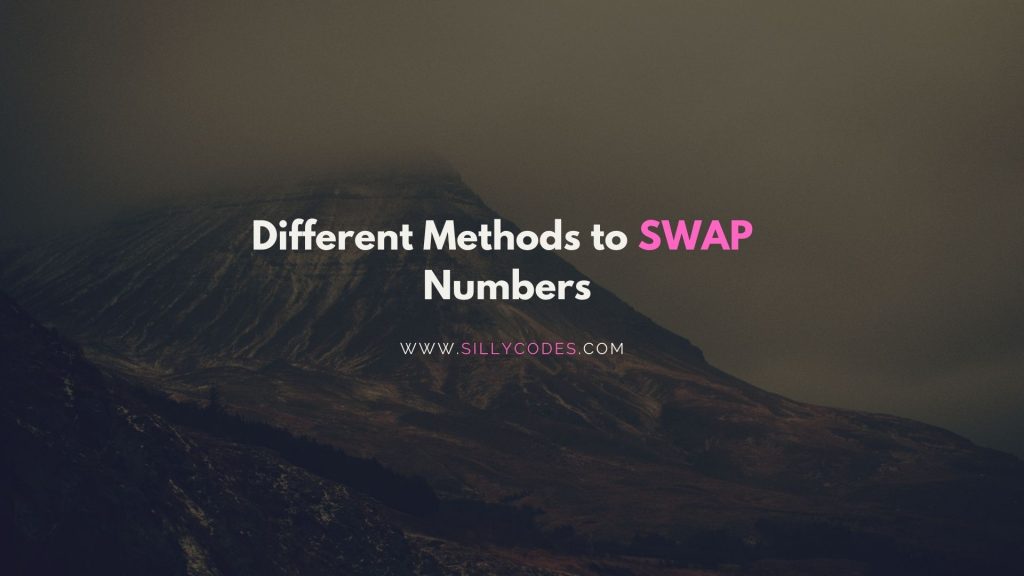
Program Introduction:
Write a Program to swap two numbers in C Language. We will discuss two methods. The First method is swapping two numbers using the temporary variable ( or third variable). The second method is swapping two integers without using any temporary variable.
Example 1:
Input:
Enter two numbers.
1 |
10 20 |
Output:
Output after swapping the numbers
1 |
20 10 |
Method 1: Swap two Numbers using Temporary Variable :
The program will take two Integers as the input and then swap those two integers. We are going to use the temporary variable to swap the integers.
We will take two integers from user, Lets say. variable 'a' and variable 'b'.
First of all, We are going to store the value of 'a' to a temporary variable named 'temp'.
Then we will copy variable 'b' value to variable 'a'. Now the variable 'b' have the variable 'a' value.
Now use the stored temporary variable 'temp'( temp have the value of 'a' )and copy the 'temp'variable value to the variable 'b’. The variable 'b' got the value of variable 'a'. Finally, variables and 'a'and 'b' values are swapped.
The temporary variable helped us to take variable 'a' value as backup. So that we can copy it to variable 'b' in later step.
But we can also swap the two variables without using any temporary variable. We will discuss about it later in this tutorial.
Program : Swap two numbers using Third variable :
Here is the program to swap two integers with the help of third variable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
#include<stdio.h> int main() {     // Take three variable, Two variable 'a' and 'b' for input     // third variable is our temporary variable named 'temp'     int a, b, temp;     printf("Enter Two Numbers : ");     scanf("%d%d", &a, &b);      // Let's print the values of 'a' and 'b' before swapping     printf("Before Swapping :: Values of a : %d \t  b : %d \n", a, b);      // Take backup of variable 'a' value. and copy it to variable 'temp'     temp = a;      // Copy value of variable 'b' into the variable 'a'     a = b;      // Now copy the value of 'temp' into the variable 'b'.     // Presently temp have the value of 'a', So b will have 'a'     b = temp;      // Now the numbers are swapped.     // Let's print the values of 'a' and 'b' After swapping     printf("After Swapping  :: Values of a : %d \t  b : %d \n", a, b);     return 0; } |
Program Output :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Compilling the program using gcc compiler $ gcc swap-temp.c  // Our executable file is a.out, So run it using below command. $ ./a.out Enter Two Numbers : 10 20 Before Swapping :: Values of a : 10      b : 20 After Swapping :: Values of a : 20        b : 10  // Example 2 $ gcc swap-temp.c $ ./a.out Enter Two Numbers : 500 1000 Before Swapping :: Values of a : 500      b : 1000 After Swapping  :: Values of a : 1000    b : 500  // Example 3 $ ./a.out Enter Two Numbers : 99 11 Before Swapping :: Values of a : 99      b : 11 After Swapping  :: Values of a : 11      b : 99 $ |
From the above output, We can see the give two numbers are swapped.
Let’s look at the another way to swap the numbers without using any extra or third variable.
Method 2 : Swap two numbers without using third or temporary variable:
In this method, We are going to use small math trick to swap the numbers. Here is the algorithm.
1 |
b = (a*b)/(a=b); |
Algorithm to swap two numbers without extra variable:
- Let’s take two variables 'a'and 'b' and We are going to swap these two variables.
- First of all, We are going to multiply both variables 'a'and variable 'b'. And The result would be 'ab'. We will use this product as the Numerator for our division.
- Then we are going use a=bOperation. This means we are copying the value of 'b'into the variable 'a'.
- Now the variable 'a' have the desired value of 'b'.
- Then in the denominator, We got final value as 'b'.
- Now we will divide our numerator 'ab’ with the denominator 'b', The result would be value of 'a'
- Then we are assigning this result value i.e 'a' to variable 'b'. Now the variable 'b'have the desired value of variable 'a'
- Finally, Two numbers are swapped without using any extra variable.
So by using above algorithm, We can simply swap two integers without using any third variable in a single line of code.
Let’s transform above logic into the code.
Swapping Two Numbers without Extra Variable Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include<stdio.h> int main() {     // Take three variable, Two variable 'a' and 'b' for input     // third variable is our temporary variable named 'temp'     int a, b, temp;     printf("Enter Two Numbers : ");     scanf("%d%d", &a, &b);      // Let's print the values of 'a' and 'b' before swapping     printf("Before Swapping :: Values of a : %d \t  b : %d \n", a, b);      // Here in the Numerator we are multiplying variable 'a' and variable 'b', So it will become ab     // then we are dividing it by (a = b ) means b will be copied to a, then Numerator ab will be divided by b,     // After dividing ab with b, we get a.     // Then we are assigning a to b.     // So at the end a have the value of b  and b have the value of a     b = (a*b)/(a=b);      // Now the numbers are swapped.     // Let's print the values of 'a' and 'b' After swapping     printf("After Swapping  :: Values of a : %d \t  b : %d \n", a, b);     return 0; } |
Please go through the comments to understand the program.
Program Output:
We are going to compile the program using the gcc compiler. You can learn more about the compiling and running c program in following article. Hello World Program in C language – SillyCodes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
// Compile the program using gcc compiler venkey$ gcc swap.c  // Run the generated executable file venkey$./a.out Enter Two Numbers : 10 20 Before Swapping :: Values of a : 10      b : 20 After Swapping  :: Values of a : 20      b : 10 venkey$  // Example 2 : Swap two numbers venkey$./a.out Enter Two Numbers : 99 33 Before Swapping :: Values of a : 99      b : 33 After Swapping  :: Values of a : 33      b : 99  // Third Example, Swap two numbers venkey$./a.out Enter Two Numbers : 44 31 Before Swapping :: Values of a : 44      b : 31 After Swapping  :: Values of a : 31      b : 44 venkey$ |
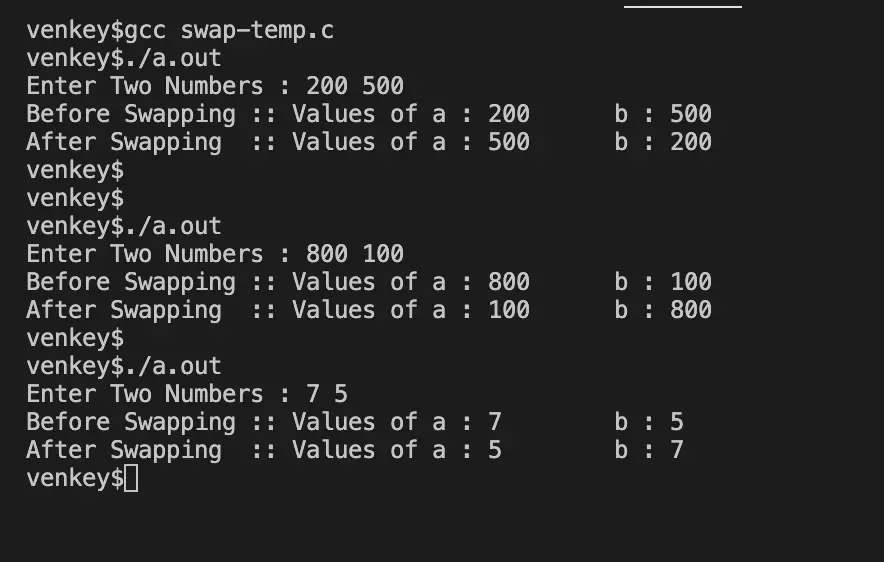
These are the not only ways to swap the two integers, We have many other techniques as well.
Let’s discuss one more bonus method.
Bonus Method : Swapping two numbers using Bitwise XOR Operator:
We can also swap variables by using the bitwise XOR operator without using third variable.
Swap numbers using XOR Operator Algorithm:
1 |
a ^= b ^= a ^= b; |
Above statement is a complex statement, Which is the combination of following statements.
1 2 3 |
    a ^= b;     b ^= a;     a ^= b; |
Okay, Above statements also complex statements. Lets re-write them.
1 2 3 |
    a = a ^ b;     b = b ^ a;     a = a ^ b; |
XOR Swapping Algorithm:
- Step 1, We apply bitwise XOR on variable 'a' and variable 'b'. And assign the result to variable 'a'.
- Step 2: We will apply the bitwise XOR on variable 'b'and variable 'a'. And then assign the result to variable 'b'.
- Step 3: We will perform the bitwise XOR on variable 'a' and variable 'b'. And then assign the result to variable 'a'.
- After performing above three steps, The values of variable ‘a’ and variable ‘b’ will be swapped.
Program: Swap numbers using Bitwise XOR operator:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
#include<stdio.h> int main() {     // Take three variable, Two variable 'a' and 'b' for input     // third variable is our temporary variable named 'temp'     int a, b, temp;     printf("Enter Two Numbers : ");     scanf("%d%d", &a, &b);      // Let's print the values of 'a' and 'b' before swapping     printf("Before Swapping :: Values of a : %d \t  b : %d \n", a, b);      // In this method, We are going to use XOR operator to swap the numbers.     a ^= b ^= a ^= b;      // Another represntation of same logic     // a ^= b;     // b ^= a;     // a ^= b;      // More readable representation of above logic. All three are same.     // a = a ^ b;     // b = b ^ a;     // a = a ^ b;      // Now the numbers are swapped.     // Let's print the values of 'a' and 'b' After swapping     printf("After Swapping  :: Values of a : %d \t  b : %d \n", a, b);     return 0; } |
Program Output:
We are using GNU GCC compiler to compile and run our programs.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
venkey$ gcc swap3.c  venkey$./a.out Enter Two Numbers : 10 20 Before Swapping :: Values of a : 10      b : 20 After Swapping  :: Values of a : 20      b : 10 venkey$  venkey$./a.out Enter Two Numbers : 877 344 Before Swapping :: Values of a : 877      b : 344 After Swapping  :: Values of a : 344      b : 877 venkey$  venkey$./a.out Enter Two Numbers : 1000 5555 Before Swapping :: Values of a : 1000    b : 5555 After Swapping  :: Values of a : 5555    b : 1000 venkey$ venkey$ |
Conclusion:
In this article, We discussed about different ways to swap the numbers. We discussed about the swapping using the temporary variable. We also looked at other methods like using Bitwise XOR to swap the numbers.
Related Programs:
- C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes
- Armstrong Number Program in C Language – SillyCodes
8 Responses
[…] There are other ways to swap the numbers, We have covered all those methods in the following article. Program to Swap Two Numbers | Swapping Program in C language – SillyCodes […]
[…] Program to Swap Two Numbers | Swapping Program in C language – SillyCodes […]
[…] Program to Swap Two Numbers | Swapping Program in C language – SillyCodes […]
[…] our previous articles, We have discussed about different ways to swap the numbers. In this article, We are going to discuss about the swap two number without using third variable […]
[…] Different methods to Swap Two Numbers […]
[…] C Program to Swap Two numbers [With and without temporary variable ] […]
[…] C Program to Swap Two numbers [With and without temporary variable ] […]
[…] Related Program – Different Methods to Swap two Numbers in C Language […]