Infinite loop in C Programming
- Introduction:
- Pre-Requisite:
- Infinite Loop in C programming:
- How do Infinite Loops occur in C language?
- Un-Intentional Infinite loops in C Programming:
- Un-Intentional Infinite loops – Forgot to Decrement the Control Variable:
- Avoiding Infinite loops:
- Intentional Infinite Loops in C language:
- Infinite loop in C using the while loop:
- Program to Demonstrate Infinite while loop:
- Infinite loops due to Mis-placed Semicolons:
- Infinite loop in C using the for loop:
- Infinite for loop program:
- Infinite for loop due to always True condition:
- Infinite loop in C using the do while loop:
- Program – Infinite do while loop in C:
- Infinite loop in C language using the goto statements:
- Infinite loop in C Programming using the Macro:
- Conclusion:
- Related C lang Loops Tutorials:
- C Programming Tutorials Index:
- Loops Practise Programs:
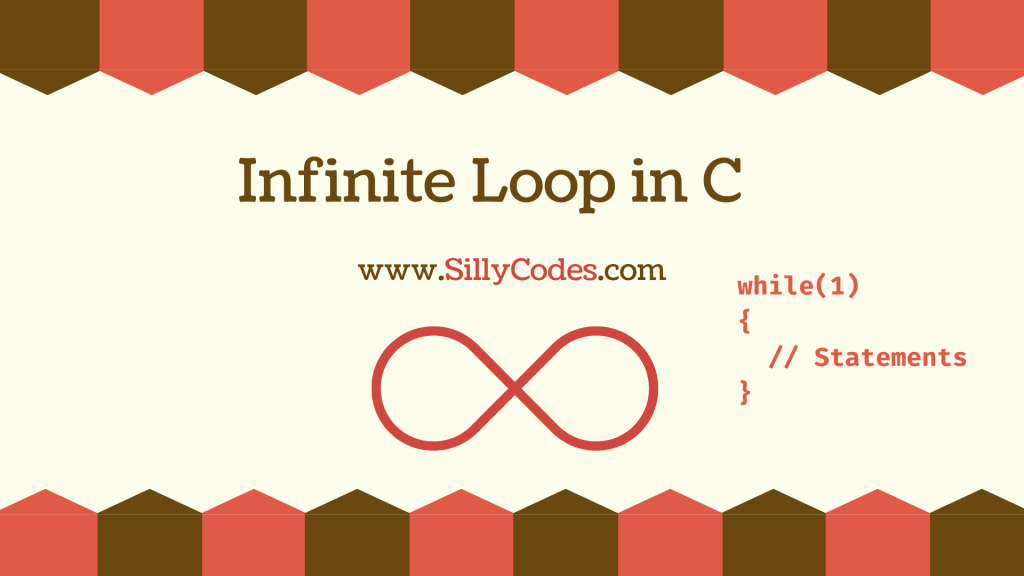
Introduction:
We have discussed about the while loop, for loop, and do while loop in C language in our earlier articles, In today’s article we are going to learn about the Infinite loop in C language and different ways to create the Infinite loops.
Pre-Requisite:
We have briefly discussed the infinite loops in our Loops tutorials. It is recommended to go through the below loops tutorials
Infinite Loop in C programming:
Any loop can become an Infinite loop if the loop condition never becomes False. So the loop condition is always evaluated as True and the loop will run forever. This scenario is called Infinite Loop.
If you look at any of the loop syntaxes, We have a Loop Condition and loop control variable,
Here is an example program to print numbers from 1 to 5.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
/* Program : Print Numbers */ #include<stdio.h> int main(){ int loopControlVariable= 1; while(loopControlVariable <= 5) // This is 'loop Condition' { printf("Counter value : %d \n", loopControlVariable ); // We need to increment the `loopControlVariable ` value. loopControlVariable++; } return 0; } |
Program Output:
1 2 3 4 5 6 7 |
$ ./a.out Counter value : 1 Counter value : 2 Counter value : 3 Counter value : 4 Counter value : 5 $ |
Here the loopControlVariable <= 5 is the Loop Condition and loopControlVariable is Loop Control variable
So each loop will have a Control variable ( loopControlVariable ), We need to make sure to increment or decrement the control variable loopControlVariable to meet our loop condition. In the above case, we incremented the loopControlVariable .
📢 If you forgot to increment or decrement the control variable, Then the loop condition won’t become False, Which means the loop condition always returns True. This causes the body of the loop to execute forever. This phenomenon is called Infinite Loop in programming.
How do Infinite Loops occur in C language?
We can have infinite loops in two ways
- Un-Intentional Infinite loops
- Intentional Infinite loops
let’s discuss about them in detail.
Un-Intentional Infinite loops in C Programming:
The Un-Intentional Infinite loops occur due to the bugs in our source code.
- When we forgot to increment or decrement the loop control variable, etc.
Un-Intentional Infinite loops – Forgot to Decrement the Control Variable:
Here is an example program, where we want to print the numbers from 10 to 1 (Descending order)
C Program print numbers in Descending order:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
/* Program : Infinite loops in C Language */ #include<stdio.h> int main(){ int counter = 10; while(counter > 0) { printf("Counter value : %d \n", counter); // We forgot to decrement the `counter` value. } return 0; } |
Infinite loop Program Output:
Build and Run the program using any compiler.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc infinite-loop.c $ ./a.out Counter value : 10 Counter value : 10 Counter value : 10 Counter value : 10 Counter value : 10 .... .... Counter value : 10 ^C ( Press Control+C ) $ |
Here we forgot to Decrement the counter (loop control variable) the value inside the while loop, So the value of the counter remained at the 10 forever.
We have the loop condition as while ( counter > 0 ), As the Counter value is not decreasing and staying at 10, So the loop condition will always return True and the loop runs forever. Which creates the Infinite loop.
If you run the above program, You will see the continuous printing of Counter value : 10 string on the console. Use the CTRL + C to kill the program (In Linux). Close the windows for PC users.
Avoiding Infinite loops:
To avoid the infinite loop, Make sure to change your loop control variables properly. You need to increment or decrement based on the loop condition.
For the above program, We can avoid the Infinite loop by Decrementing the counter value inside the while loop
Here is the working example, without an Infinite loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
/* Program : Infinite loops in C Language */ #include<stdio.h> int main(){ int counter = 10; while(counter > 0) { printf("Counter value : %d \n", counter); // Decrement the `counter` value. counter--; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc infinite.c $ ./a.out Counter value : 10 Counter value : 9 Counter value : 8 Counter value : 7 Counter value : 6 Counter value : 5 Counter value : 4 Counter value : 3 Counter value : 2 Counter value : 1 $ |
As you can see from the above output, Our program printed the numbers from 10 to 1 in descending order using the while loop. If you observe the program, We just added the counter--; to decrement the counter value.
So Initially the counter = 10 and at each iteration it will reduce by 1, So by the tenth iteration it reaches 1 and at the eleventh Iteration counter becomes 0 , The (counter > 0) loop condition will be evaluated as False and the while loop will terminate.
Intentional Infinite Loops in C language:
There will be many cases where you want to run your program forever.
For example, A Web Server can run forever to serve the requests from clients. In such cases, The web server needs to be online and running forever. In such cases, we use Infinite loops.
We can create the Infinite loop using all the loops and even using the goto statement.
- while loop
- for loop
- do while loop
- goto statement
- Macro
let’s look at each one of them in detail.
Infinite loop in C using the while loop:
If you want to create the infinite loop intentionally, Then you can simply pass any True value to while condition like below, Which creates the infinite loop.
Syntax:
1 2 3 |
while(1) { // statements } |
The above condition while(1) is evaluated as true because the value 1 is not a False value.
Program to Demonstrate Infinite while loop:
1 2 3 4 5 6 7 8 9 10 11 12 |
/* Program : Infinite while loop in c */ #include<stdio.h> int main(){ // while condition always returns `true` while(1) { printf("Server is running... \n"); } } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc infinite-while.c $ ./a.out Server is running... Server is running... Server is running... Server is running... Server is running... Server is running... Server is running... .... .... Server is running... ^C $ |
📢 Note: You can pass any True value to while condition, You are not restricted to using only 1
Here is another example, Where we are using the 100 as the loop condition, which is non-zero value so always returns True
Infinite while loop example 2:
1 2 3 4 5 6 7 8 |
#include<stdio.h> int main(){ // while condition always returns `true` while(100) { printf("Server is running... \n"); } } |
Above code also creates the Infinite loop.
Infinite loops due to Mis-placed Semicolons:
If you add the semicolon at the end of the while condition then it will create an Infinite loop.
Syntax:
1 2 3 4 5 |
while(LoopCondition); // We have semicolon here. { // statements // counter++ // incrementing the control variable } |
If you look at the above syntax, We have mistakenly added a Semicolon ( ; ) after the while condition , before the body of the while.
What does it do?
It actually creates a Dummy condition, The compiler will think, and the while loop is ended with the semicolon(;). and the body of the loop becomes another code block but not under the while loop.
So the body of the loop won’t be executed. We have loop control variable - i.e Counter, Which is incremented inside the body. But as the body is not executing, The counter value won’t increase and the LoopCondition won’t become false. This creates the Infinite loop.
Misplaced Semicolon (;) Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
/* Program : Infinite loops due to misplaced semicolon */ #include<stdio.h> int main(){ int counter = 0; while(counter <= 5); // Mistakenly added 'semicolon' here { printf("Counter is : %d \n", counter); counter++; // Incrementing the counter } return 0; } |
Program Output:
1 2 3 4 |
$ gcc infinite.c $ ./a.out ^C $ |
As you can see from the above output, Nothing will be printed.
Due to the semicolon at the end of the while loop ( while(counter <= 5);), The loop body won’t be executables. So the printf line won’t be executed and even the counter++ won’t be executed. So our loop condition never becomes False, Thus creating the Infinite loop.
📢 Don’t add the Semicolonafter the loop condition which creates a dummy loop, and the loop body won’t execute as part of the loop.
Avoiding Infinte loop:
To solve the infinite loop, Just remove the semicolon after the while condition like below.
1 2 3 4 5 6 7 8 9 10 |
#include<stdio.h> int main(){ int counter = 0; while(counter <= 5) // 'semicolon' is removed now. { printf("Counter is : %d \n", counter); counter++; // Increment the counter } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 |
$ gcc infinite.c $ ./a.out Counter is : 0 Counter is : 1 Counter is : 2 Counter is : 3 Counter is : 4 Counter is : 5 $ |
Infinite loop in C using the for loop:
We can create the Infinite loops using the for loop using the following syntax
1 2 3 4 5 6 |
for ( ; ; ) { .... // statements .... } |
As you can see, We have not specified any condition for the loop, so the loop will never become False.
📢 All three statements (Init, condition, and update) of the for loop are optional.
Infinite for loop program:
Here is an example program
1 2 3 4 5 6 7 8 |
#include<stdio.h> int main() { for( ; ; ) { printf("Listening for requests: \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc infinite-for.c $ ./a.out Listening for requests: Listening for requests: Listening for requests: Listening for requests: .... .... Listening for requests: ^C $ ^C $ |
As you can see from the above output, The string Listening for requests will print forever. As we don’t have any condition and for loop never terminates.
Infinite for loop due to always True condition:
We can also create the Infinite for loop by specifying the Truevalue in the for loop condition field like this.
1 2 3 4 5 6 7 8 |
#include<stdio.h> int main() { for( ; 1 ; ) { printf("Listening for requests: \n"); } return 0; } |
This program also runs forever and never returns. The output of this program is the same as the above program.
Misplaced semicolons in for loop:
The misplaced semicolons won’t create infinite loops in the case of the for loop. As for loop has the update statement as part of the loop options. So the counter will update.
📢 Misplaced semicolons won’t create infinite loops in the case of the for loop.
Have a look at the following program
Misplaced Semicolon Program:
1 2 3 4 5 6 7 8 9 |
#include<stdio.h> int main() { int i; for( i = 1; i <= 5 ; i++ ); // We have the 'semicolon' here { printf("%d: \n", i); } return 0; } |
Program Output:
1 2 3 4 |
$ gcc infinite-for.c $ ./a.out 6: $ |
Infinite loop in C using the do while loop:
We can create the Infinite loop using do while loop by simply passing the true value to while loop condition.
Here is the syntax
1 2 3 4 |
do { /* code */ } while (1); |
Here is an example program, which creates an Infinite loop.
Program – Infinite do while loop in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
/* Program: Infinite loop using do while loop */ #include<stdio.h> int main() { int i=1; do { printf("Value of %d \n", i); i++; } while (1); // Always True. return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc infinite-do-while.c $ ./a.out ... ... Value of 24627 Value of 24628 Value of 24629 Value of 24630 Value of 24631 Value of 24632 Value of 24633 ^C $ |
If you see the above code, We have specified the while(1) as our condition. So the loop will continue forever and we see continuous printing of numbers.
We have already looked at a Menu driven calculator program using the Infinite do while loop. Here is the link to it.
Infinite loop in C language using the goto statements:
We can create the Infinite loop using the go statements. Here is the syntax
1 2 3 4 5 |
label // statements goto label |
Infinite loop in C Programming using the Macro:
We can create infinite loops using Marcos as well. Please look at the following example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
/* Program: Infinite loop using Macro */ #include<stdio.h> #define INFINITE_LOOP while(1) int main() { int i=1; INFINITE_LOOP { printf("Running Forever \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc infinite-marco.c $ ./a.out ... ... Running Forever Running Forever Running Forever Running Forever Running Forever Running Forever Running Forever Running Forever Running Forever ^C [CRTL + C ] $ ^C |
As you can see from the above program, We have created an Infinite loop using the Macro. As it is Macro, the Compiler will simply substitute the INFINITE_LOOP with while(1) during the Pre-Processing step.
After the pre-processing step above code will look like the below.
Pre-Processing is one of the steps in the compilation process of the C Program. To generate the source code after the preprocessing step, Use the following command.
1 |
$ gcc -E infinite-macro.c -o pre-processor.i |
If you open the pre-processor.i file, You will see the following code.
1 2 3 4 5 6 7 8 9 10 |
int main() { int i=1; while(1) { printf("Running Forever \n"); } return 0; } |
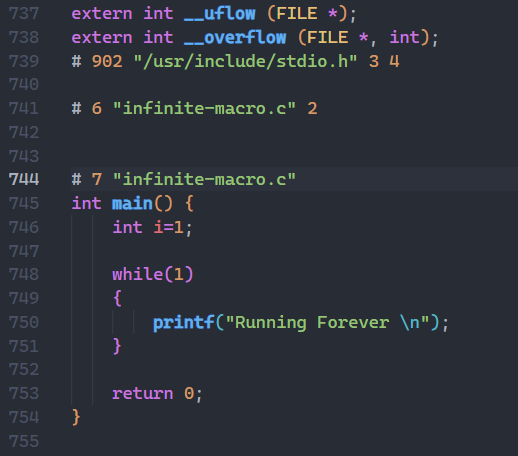
Here the INFINTE_LOOP macro has been replaced by the while(1) expression. So it is essentially became the infinite loop using a while loop.
Conclusion:
We have discussed the Infinite loop in C language and Learnt about the Un-Intentional and Intentional Infinite loops. Finally, we looked at how we can create infinite loops using the different types of loops in C Programming Language.
Related C lang Loops Tutorials:
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
2 Responses
[…] Infinite loop in C Programming language with Examples […]
[…] the Infinite Loop and Check if the max is evenly divisible by num1 and num2 using ((max % num1 == 0) && (max […]