goto Statement in C Language with Examples
- Introduction:
- The goto statement in C language:
- Syntax of goto statement in C:
- Flow Diagram of goto statement in C Language:
- Program to understand the goto statement in C Programming:
- Example Program: Print Multiplication table using the goto statement:
- The goto Statement with multiple functions in C:
- Why avoid the goto statement?
- Infinite loops due to multiple goto statements:
- When to use the goto statement in C – To Break out of Nested Loops:
- Conclusion:
- Related Tutorials:
- C Tutorials Index:
- C Loops Practice programs:
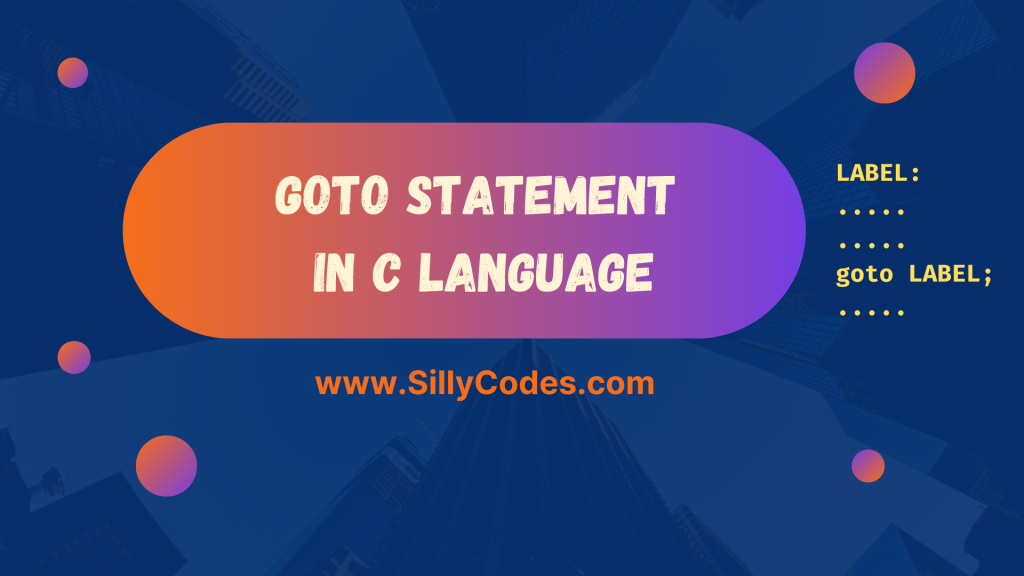
Introduction:
We have looked at the while loop, for loop, and do while loop in our earlier articles. In today’s article, We will look at the goto statement in C language with example programs. We also look at when to use and when to avoid the goto statement with examples and detailed explanations.
The goto statement in C language:
The goto statement is an un-conditional control statement in C.
The goto statement is used to jump from one statement to another statement (which is prefixed with the label) inside any function. goto is used to iterate over a code block.
📢 goto Statement is also called the jump statement.
Goto Statement can be used to
- Repeat a code block
- Break loops ( We can also break out of Nested Loops)
The goto statement must be used inside of a single function. The goto statement cannot be distributed among several functions.
📢 The goto statement must be used within the single function.
The goto is avoided since it makes the program more complex and difficult to read. Since we can accomplish the majority of these scenarios with C-Language Loops, it is not advisable to use the goto statement, Except in the cases where we absolutely need the goto statement. Like if you want to break out of nested loops.
Syntax of goto statement in C:
We have two different syntaxes for the goto statements.
C goto syntax 1:
1 2 3 4 5 6 7 |
// statements; .... goto LABLE; .... .... LABEL: ..... |
C goto Syntax 2:
1 2 3 4 5 6 7 |
// statements .... LABEL: .... .... goto LABEL; .... |
Here the LABEL is an Identifier, you can use any valid Identifier here. Make sure you follow the Identifiers naming rules.
Whenever the compiler comes across the goto statement, It will look for the LABEL and transfer the program control to LABEL: statement. This way we can jump from one statement in the function to another statement.
To understand the goto statement better, let’s have a look at an example program.
Flow Diagram of goto statement in C Language:
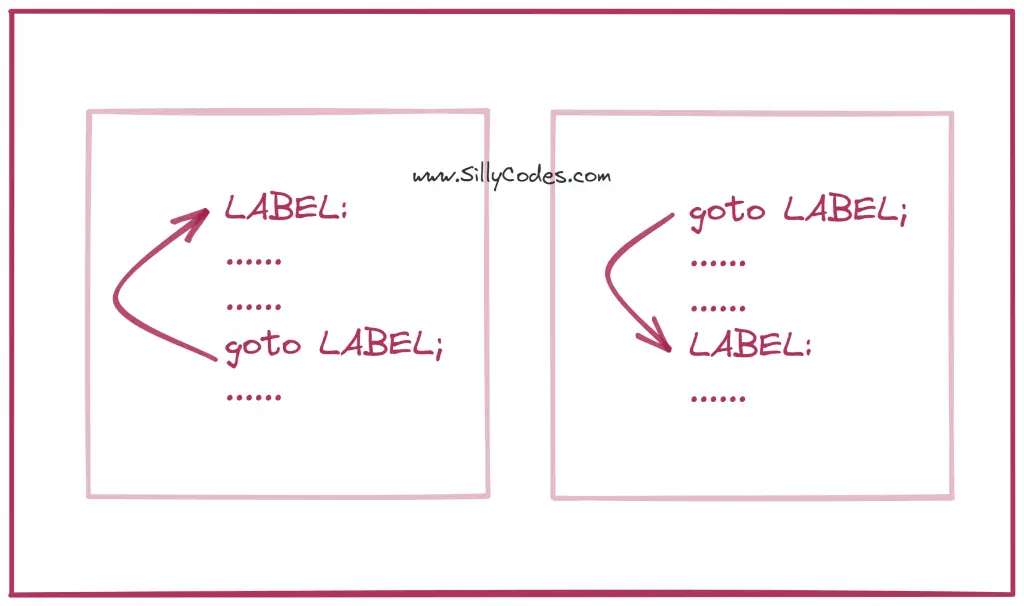
Program to understand the goto statement in C Programming:
Let’s write a program to print the multiplication table for the user-provided number using the goto statement.
Here we are going to use the goto to repeat a code block. We can achieve the same thing using the C Loops as well. but let’s try it with the break statement.
Example Program: Print Multiplication table using the goto statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/* Program: Mutliplication table using the goto statement. Author: sillycodes.com */ #include<stdio.h> int main() { // Ask the user for the input int num; printf("Enter the number you want mutliplication table: "); scanf("%d", &num); // our counter. int counter = 1; // goto label PRODUCT: // print the table entry. printf("%d * %d = %d \n", num, counter, num*counter); // Increment the counter counter++; // we will print multiplication table upto 10 // so check the `counter` // if the 'counter' is below 10, then we will jump back to `PRODUCT` Label // if the 'counter' is above 10, We will exit. if (counter <= 10) { goto PRODUCT; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
$ gcc goto-statement.c $ ./a.out Enter the number you want mutliplication table: 4 4 * 1 = 4 4 * 2 = 8 4 * 3 = 12 4 * 4 = 16 4 * 5 = 20 4 * 6 = 24 4 * 7 = 28 4 * 8 = 32 4 * 9 = 36 4 * 10 = 40 $ ./a.out Enter the number you want mutliplication table: 7 7 * 1 = 7 7 * 2 = 14 7 * 3 = 21 7 * 4 = 28 7 * 5 = 35 7 * 6 = 42 7 * 7 = 49 7 * 8 = 56 7 * 9 = 63 7 * 10 = 70 $ |
Multiplication Table Program Explanation:
As you can see from the above output, we have used the goto PRODUCT; and PRODUCT: to iterate over the code block. It is like a C Loop.
We used a counter variable to track the number of iterations. At each iteration, we printed the multiplication table entry.
The goto Statement with multiple functions in C:
As we have mentioned earlier, The goto statement can’t spread across multiple functions. which results in the compilation error.
Let’s look at an example program, where we will have the goto LABEL; in one function and LABEL: Identifier in another function.
Multi-function goto statement example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
#include<stdio.h> void task1() { // here i am marking my LABEL - 'MUTLIFUNCTION' MUTLIFUNCTION: printf("Doing Task1 \n"); } int main() { printf("Inside Main function \n"); // Here I am using the 'goto' statement goto MUTLIFUNCTION; return 0; } |
Program Output:
1 2 3 4 5 6 |
$ gcc goto-multi-func.c goto-multi-func.c: In function ‘main’: goto-multi-func.c:14:5: error: label ‘MUTLIFUNCTION’ used but not defined 14 | goto MUTLIFUNCTION; | ^~~~ $ |
As you can see from the above output, We got the error error: label ‘MUTLIFUNCTION’ used but not defined , As the goto statement works within the single function, The compiler is not able to find the MULTIFUNCTION label inside the main function. So compiler generated the error message.
📢 The goto statement only works within a single function.
Why avoid the goto statement?
Because if you have nested goto statements or multiple goto statements, Then we might end up with Infinite loops.
We have discussed about the infinite loops in c in the following article
Please have a look at the following program.
Infinite loops due to multiple goto statements:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/* Program: Nested/Mutliple goto statements */ #include<stdio.h> int main() { // Nested and mutliple goto statements B: printf("At LABEL B \n"); goto A; A: printf("At LABEL A \n"); goto B; printf("Outside of goto \n"); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc goto-nested.c $ ./a.out .... .... At LABEL A At LABEL B At LABEL A At LABEL B At LABEL A At LABEL B At LABEL A At LABEL B At LABEL A At LABEL B At LABEL A At LABEL B At LABEL A ^C $ ^C $ |
Multiple goto statements program Explanation:
We have used two goto statements in the above program. and we used them in a such way that they are going to execute forever. which caused the infinite loop.
So we need to be careful while using multiple or nested goto statements to avoid unnecessary bugs.
📢 It is recommended to avoid nested or multiple goto statements, which reduce the code readability and may cause unexpected bugs.
When to use the goto statement in C – To Break out of Nested Loops:
There will be a situation where you want to break out the nested loops, Then you can use the goto statement to jump out of all loops.
If you the break statement in a nested loop, Only the InnerMost loop will be terminated. So we can use the goto statement to break out of all loops.
Here is an example program.
Break out of all Nested loops using goto statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* program: Break Nested loops with Goto Author: sillycodes.com */ #include<stdio.h> int main() { int i, j; // Create a Nested loop using the for loops for(i=0; i<=10; i++) { for(j=0; j<=10; j++) { // break out all loops once the // value 'j' reaches 5 if(j == 5) { printf("j is 5, breaking out of all loops using goto statement \n"); goto OUT; } printf("i: %d, j:%d \n", i, j); } } OUT: printf("Outside of Nested loops \n"); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 |
$ gcc goto-nested-loops.c $ ./a.out i: 0, j:0 i: 0, j:1 i: 0, j:2 i: 0, j:3 i: 0, j:4 j is 5, breaking out of all loops using goto statement Outside of Nested loops $ |
As you can see from the above output, Two nested loops are terminated once j reached 5. Here we used the goto statement to terminate all loops. Unlike the break statement, goto terminates the loops based on the label, Here we specified the label ( OUT ) outside of the nested loops.
As soon as the goto OUT; was executed, The program control came out of both loops and reached OUT: statement.
The goto statement will be appropriate in a lot of other places. Please only use it when necessary.
Conclusion:
In this article, We have learned about the goto statement in the C Programming language. We looked at when to use the goto statement and when not to use the goto statement. Finally, we looked at the nested loops with goto statement.
Related Tutorials:
- Control Statements
- Loops
15 Responses
[…] Goto Statement in C Language […]
[…] are using the goto statement (Jump statement) to take the control back to the start of the […]
[…] Goto Statement in C Language […]
[…] Goto Statement in C Language […]
[…] Goto Statement in C […]
[…] Prompt the user for Input again, We are going to use the Goto Statement in C language. The Goto statement is a Jump Statement used to jump from one statement in a function from another […]
[…] Goto Statement in C […]
[…] Goto Statement […]
[…] This Program accepts one integer from the user and prints first n prime numbers. If the user enters a Negative number, We display an error message and Ask for the user’s input again using the Goto Statement. […]
[…] Goto Statement in C Language […]
[…] Goto Statement in C Language […]
[…] do that, We are going to use the Goto Statement, Which is a Jump statement used to jump from one statement to another statement in the C […]
[…] is recommended to have the basic knowledge of the C Loops like while loop and for loop and goto statement. As we are going to use them in this […]
[…] Check if the n is a negative number, If so display an error message ( ERROR! Enter a Valid Number). Ask for the user input again. Take the program control back to the start of the program using the Goto Statement in C […]
[…] Check for a negative number and display the error message if the n is a negative number. and allow the user to provide the input again using the Goto Statement […]