Pointers in C Language – How to Create and use pointers
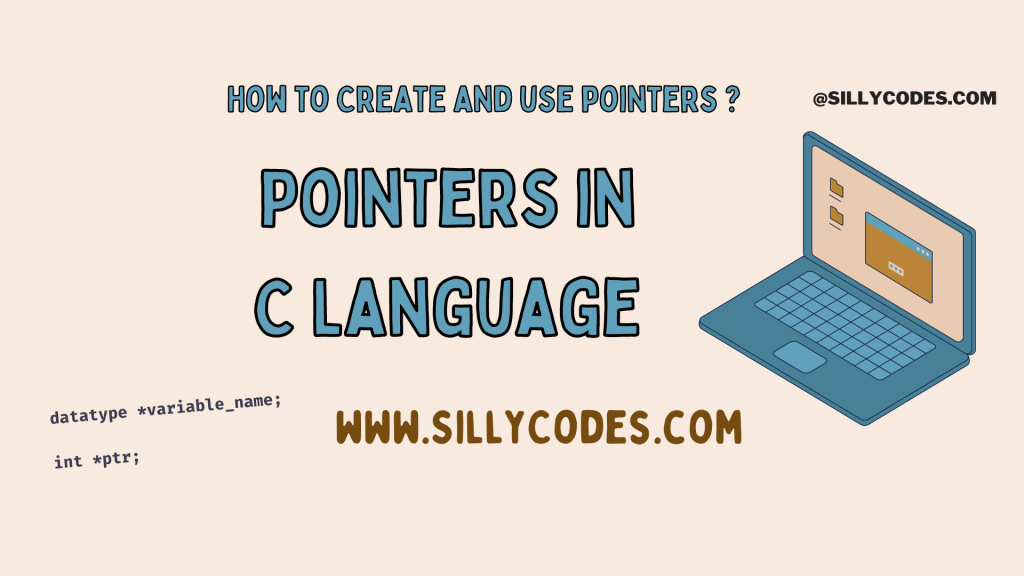
- What is a Pointer variable in C?
- How are Variables stored in Memory?
- Address of Operator(&) in C – How to get the memory address of variables :
- Pointers in C Programming Language:
- Indirection operator or Dereference Operator (*) in C Language:
- Changing the variable value through the pointer variable in C:
- Things to remember while using the Pointers in C:
- What are the uses of Pointers in C Programming Language:
Pointers in C – Introduction:
We have looked at the Strings in C language in earlier articles, In today’s article, We will learn about the Pointers in C programming. We will look at how to declare, initialize, and use pointers in C language with example programs. This article is going to be a long one with a detailed explanation. So let’s get started.
📢 This Program is part of the C Programming Language tutorials series.
What is a Pointer variable in C?
A Pointer is a special variable that holds the memory address of another variable.
📢 The pointer variable points to a memory address of another variable.
Before going dive deep into the pointers in C language it is important to know the basics of memory allocation.
We need to know
- How the variables are stored in memory and What is meant by the memory address.
- How to get the memory address of variables
How are Variables stored in Memory?
The main memory of a computer is called Random Access Memory (RAM), and it is used to store variables and other program-related information.
The computer RAM is made up of bytes that are ordered sequentially. Each byte has an index number, which is referred to as its address ( memory address ).
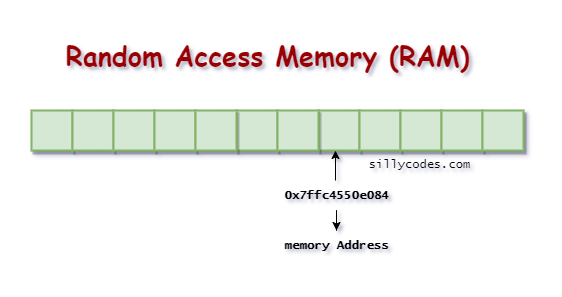
Let’s look at how a variable is stored in the memory.
If we create any variable, The C compiler needs to reverse memory for the variable in the primary memory(RAM).
In the C programming language, we normally specify the variable name together with the variable’s data type during the variable creation. So that the compiler knows how much memory(how many bytes) it should allocate for the said variable.
Let’s look at an example to make our understanding concrete.
int score = 300;
- We have created a variable called score with the integer( int) data type.
- As we already know the default Integer size in C is 4 Bytes. So the C compiler will go ahead and reserves the 4 consecutive Bytes in the memory
- As specified earlier, This memory location will have an address. Let’s say the compiler allocated the address 0x7ffc4550e084 for the score variable.
- Finally, It will update the score variable memory address (i.e 0x7ffc4550e084) with the value 300.
- Now, We have created a variable named score , which is stored in the memory address 0x7ffc4550e084 and has a value 300.
Here is the graphical representation of how variables are stored in the C programming language.
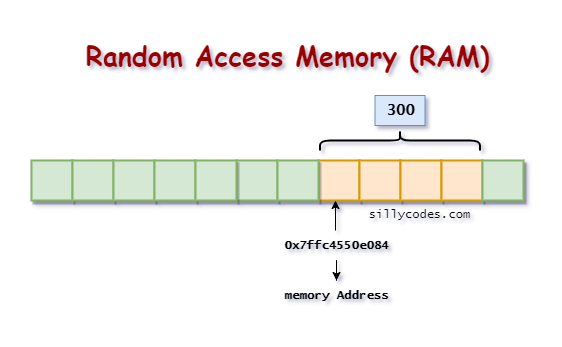
ℹ️ The Integer size varies depending on your system architecture.
Address of Operator(&) in C – How to get the memory address of variables :
We can get the memory address of any variables using the address of operator or reference operator.
The address of operator is denoted by the & ampersand symbol. This is a unary operator and can be used with a single operand.
Syntax of Address of Operator (&):
1 2 3 4 5 |
//syntax &variable_name; // example &score; |
In order to get the memory address of the variable, Place the address of operator(&) before the variable name.
We can get the memory address of the score variable by using the &score ( address of operator)
📢We have used the address of times many times so far, If you recall, We always passed the variables with the memory address to the scanf functions.
scanf(“%d”, &num);
Let’s look at a program to get the address of a variable in c language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* Program to demonstrate the address of operator in C sillycodes.com */ #include<stdio.h> int main() { // declare a variable int score = 300; // variable value printf("Value of score: %d\n", score); // get the address of 'score' variable printf("Address of score: %p\n", &score); return 0; } |
We used the %p format specifier to print the memory address. You can check all available format specifiers in the following article.
Program Output:
Let’s compile and Run the program using GCC compiler or your favorite compiler.
1 2 3 4 5 |
$ gcc memory-address.c $ ./a.out Value of score: 300 Address of score: 0x7fff0034a9f4 $ |
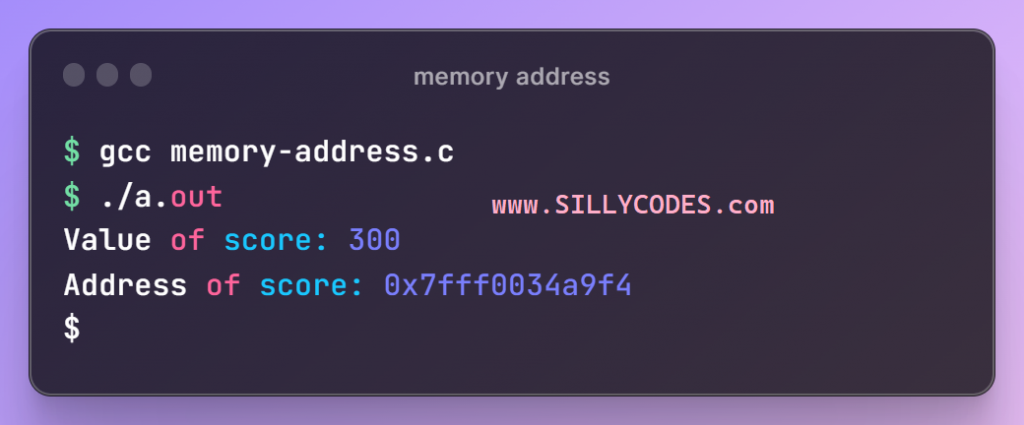
The scorevariable is stored at the memory address 0x7fff0034a9f4in RAM.
📢If you run the above program, You might get a different value for the memory address.
Pointers in C Programming Language:
A pointer variable is a variable that stores the address of another variable.
Similar to other variables, The pointer variable also has the datatype, and variable name, and also occupies space in the memory.
Here is the pictorial representation of the pointer storing another variable memory address.
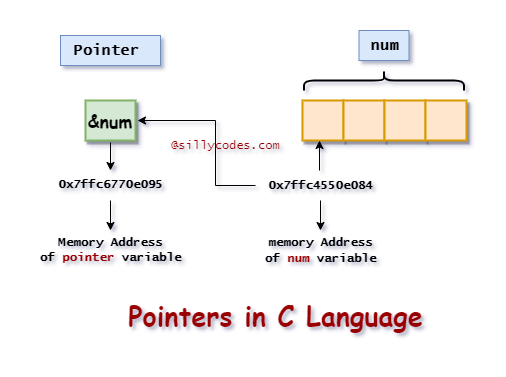
Declaration of Pointer variables in C Language:
We can declare a pointer variable by using the * (Asterisk symbol). Here is the syntax of the pointer declaration.
Syntax of Pointer declaration:
1 |
datatype *pointerName; |
Here
- pointerName is the pointer name. This can be any valid C identifier.
- * (Asterisk) specifies the pointerName is a pointer variable.
- dataype is the datatype of the pointer. Do note that, This pointer points to datatype variable.
Let’s look at a few examples of the pointer variables.
1 2 3 4 5 6 7 8 9 10 |
// Integer pointer int * intPtr; // character pointer char * chPtr; // we can declare multiple pointers like below int * p1, *p2, *p3; |
We created a pointer variable called intPtr which is an integer pointer. The intPtr points to the integer variable.
Similarly, the chPtr is a character pointer and points to the character data.
So we need to create the pointer with the datatype the same as the data it is going to point.
Size of the Pointer variables in C:
The Pointer variables are also variables. So the pointers will also take up some space in the memory.
But unlike other normal variables, All Pointers(either it is integer pointers, character pointers, float pointers, etc.) store the memory address of another variable.
As the pointer variable stores only the memory address, The size of the pointer variable is 4 Bytes ( The pointer size will change based on your compiler and OS architecture).
📢All pointers store only the memory address, So the size of all pointers is the same. ( Generally, The size of the pointer variable is 4 bytes)
Initializing the Pointer variables:
By default, Pointer variables will have garbage values. So it is always recommended to initialize the pointer variables.
Here is the syntax to initialize the pointer variables
1 |
datatype *pointerName = &variableName; |
Here
- pointerName is the name of the pointer
- datatype is the data type of the pointer and variableName.
- * (Asterisk) denotes we are creating the pointer variable
- variableName is the variable, The address pointer( pointerName) is going to store. This variable should be the type of datatype.
- & (Address of operator) used to get the address of the variableName.
Let’s look at an example.
1 2 3 4 5 |
// Initialize a integer variable int score = 300; // Initialize a pointer variable 'intPtr' int *intPtr = &score; |
We have created an integer pointer variable named intPtr and initialized it with the address of the score integer variable.
Now, The intPtr is storing the address of score variable. ( Pointer storing the address of another variable).
Here is the graphical representation of how pointers work in the c programming language.
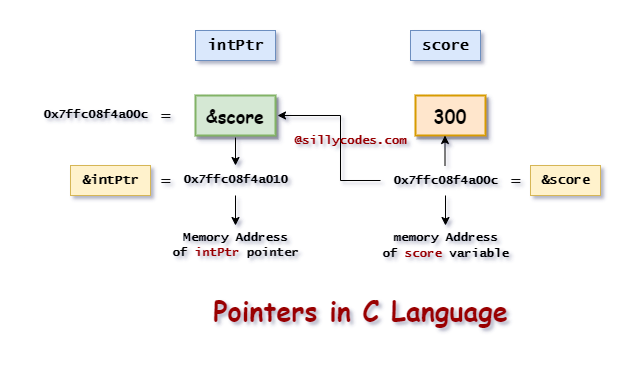
Let’s look at a program to demonstrate how pointers work in the C.
Program to demonstrate how pointers work in C programming:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* Program to demonstrate how pointers works in c language sillycodes.com */ #include<stdio.h> int main() { // declare a variable int score = 300; // Initialize a pointer variable 'intPtr' and store address of 'score' int *intPtr = &score; // variable value printf("Value of score: %d\n", score); // get the address of 'score' variable printf("Address of score: %p\n", &score); // value of 'intPtr' pointer variable printf("Value of intPtr(pointer) :%p\n", intPtr); // Address of 'intPtr' pointer variable printf("Address of intPtr(pointer): %p\n", &intPtr); return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 |
$ gcc memory-address.c $ ./a.out Value of score: 300 Address of score: 0x7ffc08f4a00c Value of intPtr(pointer) :0x7ffc08f4a00c Address of intPtr(pointer): 0x7ffc08f4a010 $ |
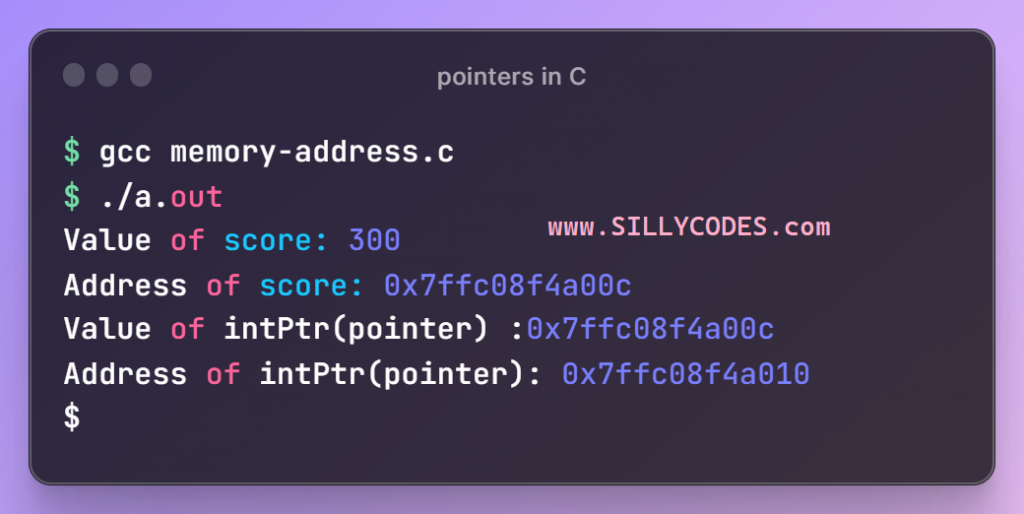
Program Explanation – How pointers works in C:
- We started the program by creating an integer variable called score and initialized with the value 300.
- Then we created an integer pointer named
intPtr and initialized it with the address of the
score integer variable.
- A couple of things to note here, We used the Asterisk ( *) to create the pointer variable. ( i.e int * intPtr)
- We also used the address of operator (&) to get the address of the score integer variable.( i.e &score)
- Print the Value of the score variable using the printf("Value of score: %d\n", score);. This prints the 300
- Next, we displayed the address of the
score variable using the address of & operator – This prints the address of the
score variable which in this case is equal to
0x7ffc08f4a00c
- printf(“Address of score: %p\n”, &score);
- Then we printed the value of the pointer variable
intPtr using another
printf function. Here we used the
%p format specifier as the
intPtr is holding the address of the
score. If you notice, The value of the
intPtr and the address of the
score variable are the same. i.e
:0x7ffc08f4a00c
- printf("Value of intPtr(pointer) :%p\n", intPtr);
- Finally, The pointer variable
intPtr will also have a memory address, To print the address of the
intPtr pointer variable, We used the &intPtr . which is equal to
0x7ffc08f4a010
- printf(“Address of intPtr(pointer): %p\n”, &intPtr);
📢Note that, If you run the above program, the memory address values might be different for you.
Indirection operator or Dereference Operator ( *) in C Language:
So far we looked at how to store the address of a variable using the pointer variable. We can access the value of the variable pointed by the pointer variable using the dereference operator ( *).
The dereference operator also called as the indirection operator in c programming language.
Program to understand the dereference operator in c:
Let’s look at an example to understand the dereference operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* Program to demonstrate the dereference operator in C sillycodes.com */ #include<stdio.h> int main() { // create a variable int num = 100; // create a pointer variable 'ptr' int *ptr = # // value of 'num' printf("Value of num: %d\n", num); // address of 'num' printf("Address of num: %p\n", &num); // value of 'ptr' printf("Value of ptr:%p\n", ptr); // value variable pointed by 'ptr' pointer // i.e Get 'num' value using 'ptr' pointer printf("Value of variable pointed by ptr(*ptr): %d\n", *ptr); return 0; } |
Program Output:
Compile the program.
$ gcc dereference.c
Run the program.
1 2 3 4 5 6 |
$ ./a.out Value of num: 100 Address of num: 0x7ffc01a267dc Value of ptr:0x7ffc01a267dc Value of variable pointed by ptr(*ptr): 100 $ |
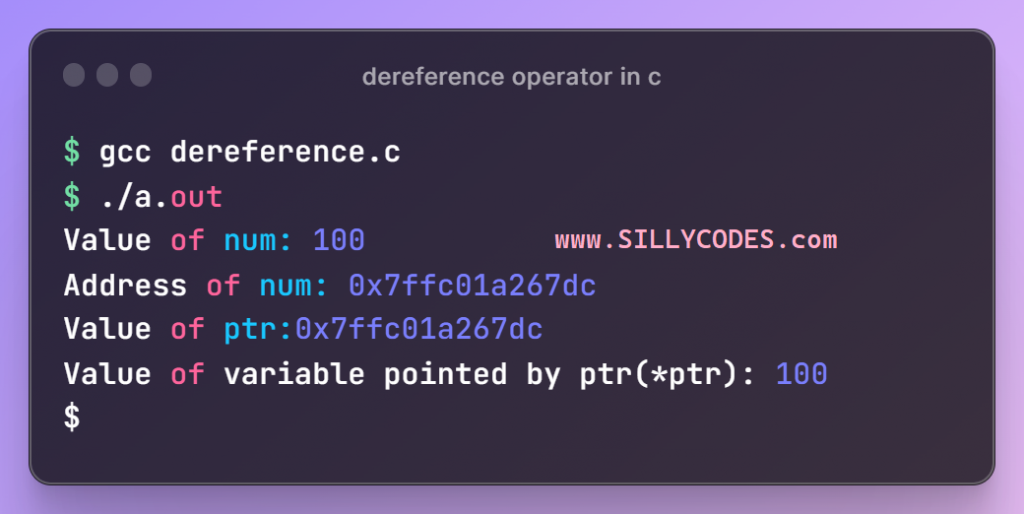
Pointer dereferencing Program Explanation:
As we can see from the above output, We have defined an integer variable num with the value 100 and a pointer variable with ptr and initialized it with the address of the num
Now, The pointer variable is storing the address of the num variable and we can also access the value of the num variable by using the dereference operator( *).
So we used the first three printf functions to print the value of num and address of num and the value of ptr (i.e address of num)variable respectively.
Finally, We used the dereference operator( *) with the pointer( ptr) variable to get the value of the num variable.
printf("Value of variable pointed by ptr(*ptr): %d\n", *ptr);
which prints the value of the num variable i.e 100
Changing the variable value through the pointer variable in C:
Let’s change the above program to change the variable value through the pointer. We can update the variable value by using the dereference operator like below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* Program to demonstrate the dereference operator in C sillycodes.com */ #include<stdio.h> int main() { // create a variable int num = 100; // create a pointer variable 'ptr' int *ptr = # // value of 'num' printf("Value of num: %d\n", num); // value variable pointed by 'ptr' pointer // i.e Get 'num' value using 'ptr' pointer printf("Value of variable pointed by ptr(*ptr): %d\n", *ptr); // update the 'num' using pointer *ptr = 50000; // After update printf("Value of 'num' variable(After change):%d\n", num); return 0; } |
Program Output:
Compile and Run the C Program.
1 2 3 4 5 6 |
$ dereference-change-value.c $ ./a.out Value of num: 100 Value of variable pointed by ptr(*ptr): 100 Value of 'num' variable(After change):50000 $ |
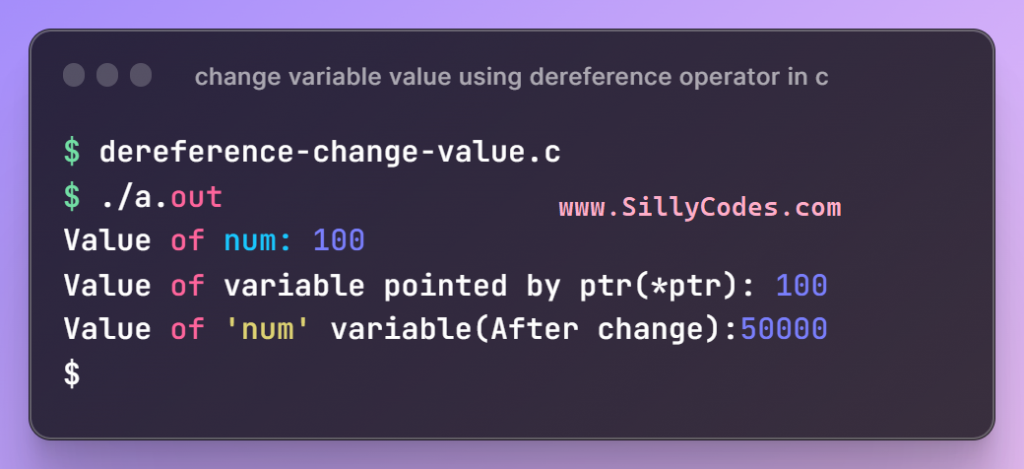
As you can see from the above output, The value of the variable num is changed by using the pointer variable ptr.
To change the variable, We used the *ptr(i.e *ptr = 50000) to update the integer variable num via the pointer variable ptr. We can see it from the above output and the value of the num variable before and after the change.
Things to remember while using the Pointers in C:
1) Make sure to assign the memory address of a variable to a pointer variable.
1 2 3 |
// Correct int n1 = 44; int *p1 = &n1; |
The above example is the correct way to assign the memory address of a variable to a pointer variable.
1 2 3 |
// Incorrect int n1 = 44; int p2 = &n2; |
If you note, we missed the asterisk symbol(*) before the p2. So the p2 variable is not a pointer variable. It is just another integer variable, so the variable p2 can’t store the address of another variable.
2) If you declare and assign the pointer variables. make sure to use the pointer while assigning the address.
1 2 3 4 |
// Correct way int n3 = 63; int *ptr3; ptr3 = &n3; |
We declared the ptr3 pointer and then assigned the address of the variable n3 to ptr3. Now the ptr3 stores the memory address of n3.
1 2 3 4 |
// Incorrect way int n4 = 63; int *ptr4; *ptr4 = &n4; // Not correct |
We used the *ptr4 to update the address of &n4. This is not correct. We don’t use dereference operator( *) to update addresses.
We should use dereference operator( *) only to update the value of the variable pointed by the pointer.
In the above case, The variable n4 will now have a value that is equal to its own memory address. ( something like 130295644). This is not intended.
What are the uses of Pointers in C Programming Language:
There are many uses for pointers. few of them are,
- Pointers are used for Dynamic memory allocation ( malloc, free)
- We can pass the variables as references( addresses) to functions. – Learn more about the Call by value and call by reference
- Accessing, Modifying, and Traversing the Array, String, and Structure elements.
- Returning more than one value from the functions.
- Pointers can also be used to implement the data structures like linked lists, trees, etc.
Conclusion:
We have learned about how variables are stored in the computer memory, What are pointers in C, and how to declare, Initialize and update pointers with example programs. We also looked at the address of operator(&) and the dereference operator(*).
Related C Language Articles:
- 300+ C Practice Programs (Index)
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- Passing Arrays to Functions
- Procedural Programming Languages Vs Structural Programming Languages
- Different methods to Swap Two Numbers
- Command line arguments in C programming with examples
16 Responses
[…] have looked at the introduction to pointers in an earlier post. In today’s article, We will look at the address of operator in c programming language with […]
[…] is recommended to go through the Introduction to Pointers tutorial to better understand this […]
[…] have discussed about the pointers in c, address of operator, and dereference operator in earlier posts, In today’s post we are going to […]
[…] is recommended to go through the introduction to the pointers in C and how to use the address of operator and dereference operator in c to better understand the […]
[…] Pointers in C Programming Language – Declare, Initialize, and use pointers […]
[…] Pointers in C Programming Language – Declare, Initialize, and use pointers […]
[…] have looked at the introduction to pointers, and pointer arithmetics in earlier articles. In today’s article, We will look at the pointer to […]
[…] have looked at the Introduction to pointers and pointer to pointer variable in earlier posts. In today’s article, We will look at the […]
[…] a function returning pointer in c programming language. As we have discussed earlier in the introduction to pointer and pointers and array articles, We can return a pointer variable from a function in C language. We […]
[…] previous articles, we looked at pointers in C and Double pointers in C with example programs. In this article, we will look at function pointers […]
[…] Pointers in C Language – How to Create and use pointers […]
[…] have covered the C Pointers, Pointer Arithmetic, and Pointer to pointers in earlier articles. In today’s article, We will […]
[…] Pointers in C Language – How to Create and use pointers […]
[…] our previous article, We have looked at the pointers and pointer programs. In today’s article, We will look at what is Static Memory allocation and […]
[…] Pointers in C Language – How to Create and use pointers […]
[…] call to the malloc() function is successful, Then the malloc() function will allocate 40 Bytes of memory in the HEAP section and The starting address of the allocated memory will be stored in the iPtr […]