Pre Increment and Post Increment in c language
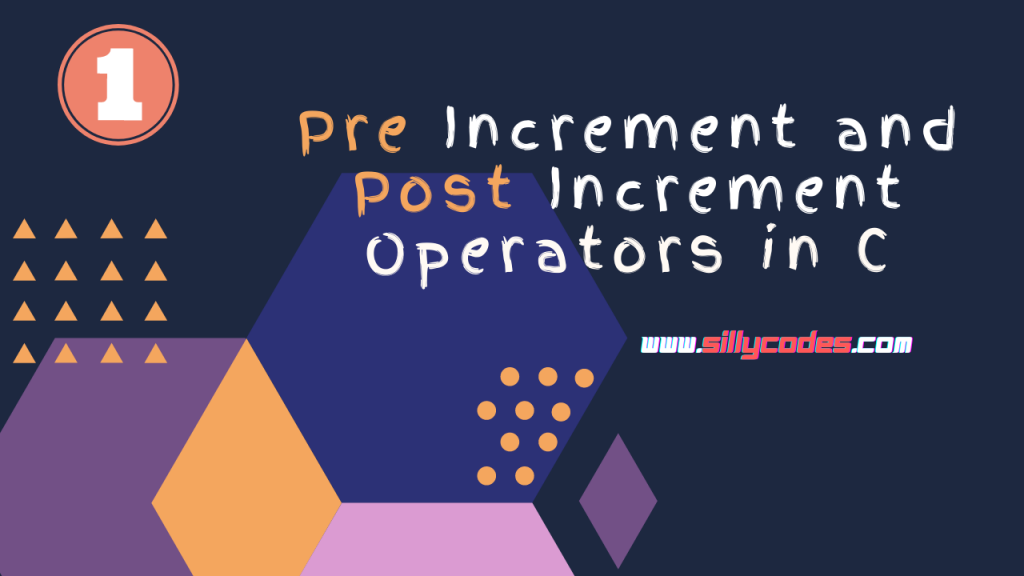
Intro to Pre Increment and Post Increment operators in c:
Pre increment and Post increments operators are used to increase the value of the variable by one(1).
These Increment operators are Unary operators. This means they work on a single operand. unlike the Binary operators which operate on two operands.
Pre Increment and Post Increment operators have a higher priority compared to all the Binary operators.
When these operators are mixed with Addition or subtraction or any other binary operators, The pre/post increment operators get the priority and these operations are evaluated first.
We have two types of Increment Operators
- Pre-Increment Operator
- Post-Increment Operator.
The main difference between the pre increment and post increment is the place of the operator with respect to operand. and which defines how we increment the variable value.
We can apply the increment operators on the variables only. We can’t apply them to the constant values.
Example: ++10;
Above example code will gives us the compilation error. As 10 is the constant number, We can’t apply increment operator on it.
Pre Increment and Post Increment Operators:
Let’s discuss pre/post increment operators in detail with examples.
Pre Increment Operators:
In the Pre Increment operation the Increment operator(++) will be written before the operand.
Example : ++a;
Here ++ is a unary Increment Operator. As this unary increment operator and came before the operand 'a'. We call this operation a Pre Increment operation.
In the Pre Increment operation, The operator Increments the value of the variable (‘a’) first, then incremented value is used to evaluate the Expression.
Lets take an examples to understand it better.
Example:
1 2 |
num = 10; y = ++num; |
In the above example, num the variable value is 10. Then we applied pre-increment operation on variable num and then assigned the result to variable y.
As we discussed earlier. The pre-increment operator first increments the value of the num by 1. So the num becomes 11. Then the Incremented value (i.e 11) is assigned to the variable y. So after the above operation, the value of the variable y is 11.
📢 Pre Increment Operator, First Increments the value of the variable. Then the Incremented value is used to evaluate the expression
Program to Understand the Pre Increment Operator in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
#include <stdio.h> int main() {     int a = 10;     int b = 20;         // Increment 'a' by 1     // Increment 'b' by 1     // add both 'a' and 'b'     // Assign result to variable 'c'     int c = ++a + ++b;      // Values of 'a', 'b' and 'c' after above increment and assign operation     printf(" a:%d, b:%d and c:%d \n", a, b, c);     return 0; } |
In the above program, We took three variables 'a', 'b'and 'c'. We initialised ‘a’ with 10 and ‘b’ with 20.
Then we used the pre-increment operator on both variables 'a' and 'b' then assigned the sum of 'a'and 'b' to the variable 'c'.
int c = ++a + ++b;
Here the ++aoperation will increment the value of the variable 'a'by 1. So variable 'a' becomes 11. Similarly, the ++boperation will increment the value of variable 'b' by 1. So variable 'b' becomes 21.
Then we will add both variable 'a' (11) and variable 'b' (21). The final result will be 32(c).
Program Output:
1 2 3 4 |
$ gcc pre-increment.c $ ./a.out a:11, b:21 and c:32 $ |
Post Increment Operator:
If Increment operator will be written after the operand. Then we call it as the post-increment operation.
Syntax : b++;
Here ++ is a unary Increment Operator. As this unary increment operator came after the operand 'b'. We call this operation a Post Increment operation.
In the Post Increment operation, The expression is evaluated first then The operator Increments the value of the variable (‘b’).
Example:
1 2 |
b = 31 res = b++; |
In the above example, The original value of the variable 'b' will be assigned to 'res'variable then the variable 'b' value will be incremented by 1. So after completing the above lines of code, The variable 'b' value becomes 32. And the variable 'res' contains the value ’31’.
📢. Post-Increment Operator, First Evaluates the expression then increments the value of the variable by 1.
Program to understand Post-Increment Operator:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
#include <stdio.h> int main() {     int a = 10;     int b = 20;          // As this is post-increment     // First take the value of orignal value of 'a'     // and variable 'b'     // Add those two values. Assign result to variable 'c'     // Increment 'a' by 1     // Increment 'b' by 1     int c = a++ + b++;      // Values of 'a', 'b' and 'c' after above increment and assign operation     printf(" a:%d, b:%d and c:%d \n", a, b, c);     return 0; } |
In the above code, We will first evaluate the expression. That means we are going to take the original values of variable 'a' and variable 'b'. i.e 10 and 20 respectively.
We will add them and assign the result to variable 'c'. So 'c' becomes 30.
Then as we done with the expression, We are going to increment the variable 'a' by 1. Similarly we increment value of the variable 'b' by 1. So at the end variable 'a' becomes 11, variable 'b' becomes 21, and variable 'c' is 30.
Output:
1 2 3 4 |
$ gcc pre-increment.c $ ./a.out a:11, b:21 and c:30 $ |
1 Response
[…] Pre Increment and Post Increment in c language […]