Assignment Operator in C Language | L- value Error in C programming
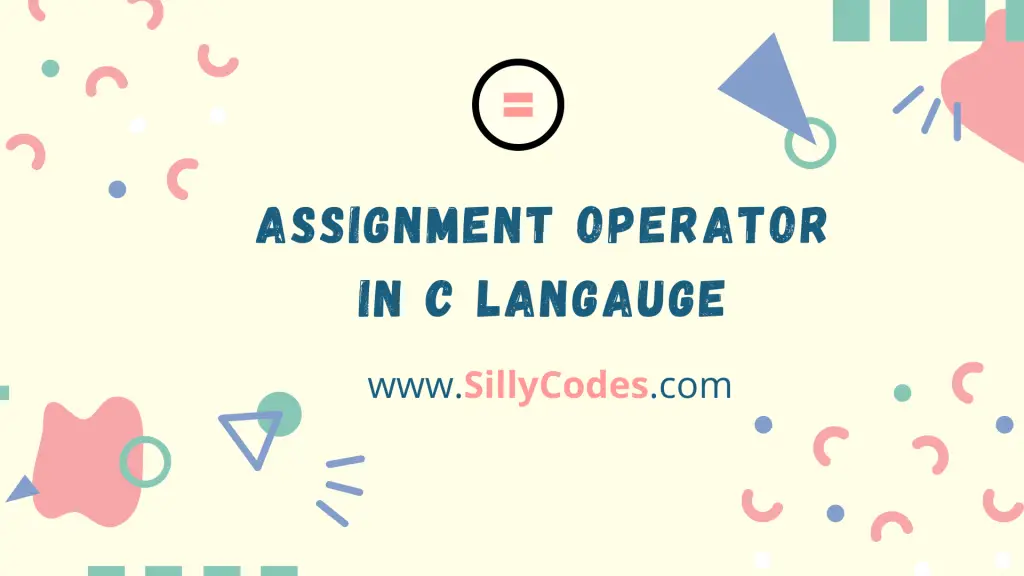
Introduction:
In our previous articles, we discussed the Arithmetic Operators in C Language. In today’s article, We will learn about the Assignment Operator in C Programming. We also discuss the L-Value error in programming.
Assignment Operator in C Langauge ( = ) :
The C programming language has assignment operators to create and assign values to the variables.
We represent the Assignment operator using the equal to( = ) symbol in C programming.
Syntax Of Assignment Operator:
1 |
L-Value = R-Value |
The assignment operator is for used assigning values. In general assignment operator assigns the value of the right-hand ( R-Value) side operand to the Left-hand (L-Value) side Operand.
The assignment operator is a binary operator so it must need two operands.
The assignment operator has two values. The Left-side value is called as L-Value and The Right-side value is called as R-Value.
The Assignment Operation will copy the R-Value to L-Value.
The left-side value ( L-Value ) of the Assignment Operator must be a Variable.
The assignment operator has the Lowest Precedence or Priority compared to all other operators except Comma Operator.
For full Priority of Operators please visit – see full priority table/precedence table
Example Program to understand Assignment Operator:
1 2 3 4 5 6 7 8 9 10 11 12 |
#include<stdio.h> int main() { int x; // Here we are assigning the value '10' to variable 'x' x = 10; // Print the value of 'x' using 'printf' printf("value of x is : %d \n", x); return 0; } |
In above Example x = 10 is assignment operation.
Here x is the left-side value or L-Value and The value 10 is the right-side value or R-Value, By using the assignment operator we assigned the value 10 to the variable x.
Program Output:
1 |
value of x is : 10 |
As you can see the value of x is 10.
Example 2 : L – Value Error in C programming :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include<stdio.h> int main() { // Create a Variable 'x' and assign value '10' int x = 10; // Here we are trying to assign the value of 'x' to '20' // As 20 is constant, This operation is not allowed. // Which results into the compilation error. 20 = x; // Try to print the value of 'x' printf("value of x is : %d n", x); return 0; } |
The Left-side value of the Assignment Operator must be Variable. So we basically trying to assign R-Value to L-Value. So L-Value must-have capacity to store the R-Value. But constants and numbers can’t store a value.
📢 The Left-side or L-Value of the Assignment Operator must be a Variable.
Program Output
1 2 |
error: lvalue required as left operand of assignment 20 = x ; |
Complex Assignment Operations in C Programming:
In the C programming language, We have few compound assignment operators to perform the assignments along with some other arithmetic operations.
For example,
x = 5; // simple assignment
x += 10; // compound operation
Simple Assginment Operation:
x = 10;
This is a simple assignment, Here we have created a variable x and assigned value 10 to x.
Compound Addition ( Add then Assigne += ):
x += 20;
This is a compound operation. Which is equal to
x = x + 20;
Here first x+20 is calculated then x is updated with the resulting value of x+20 operation.
Similarly, we can have other compound arithmetic operations, Please look at the following table for all compound Operations.
Operator | Syntax | Description | Example |
---|---|---|---|
Assignment ( = ) | a = b | Creates variable
a and assigns value b to it. | >
a = 10; > printf("%d", a); 10 |
Compound Addition (
+= ) or Add and Assign | a += b | First, Evaluate the addition of
a+b then assign results to a Step 1: a+b Step 2 : a = a+b | >
printf("%d", a); 10 > a += 10 > printf("%d", a); 20 |
Compound Subtract (
-= ) or Subtract and Assign | a -= b | First, Evaluate the
a-b then assign the result to a | >
printf("%d", a); 10 > a -= 10 > printf("%d", a); 0 (zero) |
Compound Product (
*= ) or Multiply and Assign | a *= b | First, Evaluate the
a*b then assign the result to a | >
printf("%d", a); 10 > a *= 10 > printf("%d", a); 100 |
Compound Division (
/= ) or Division and Assign | a /= b | First, Evaluate the
a/b then assign the result to a | >
printf("%d", a); 10 > a /= 10 > printf("%d", a); 1 |
Compound Modulus (
%=) or Modulus and Assign | a %= b | First, Evaluate the
a%b then assign the result to a | >
printf("%d", a); 10 > a %= 10 > printf("%d", a); 0 ( zero ) |
The Compound Assignment statements are elegant and try to use them in the programs.
Conclusion:
In this article, We discussed the Assignment Operators and Compound Assignment operators in the C language. We also looked at a few example code snippets and L-Value Error.
Related Tutorials:
- Start Here – Step by step tutorials to Learn C programming Online with Example Programs – SillyCodes
- Modulo operator in C explained with Examples.
- Arithmetic operators in C language.
- Compilation Stages in C language.
- Identifiers in C language and Rules for naming Identifiers.
- Structure of C Language.
2 Responses
[…] Assignment Operators. […]
[…] Assignment Operators. […]