Passing arrays to functions in C Language
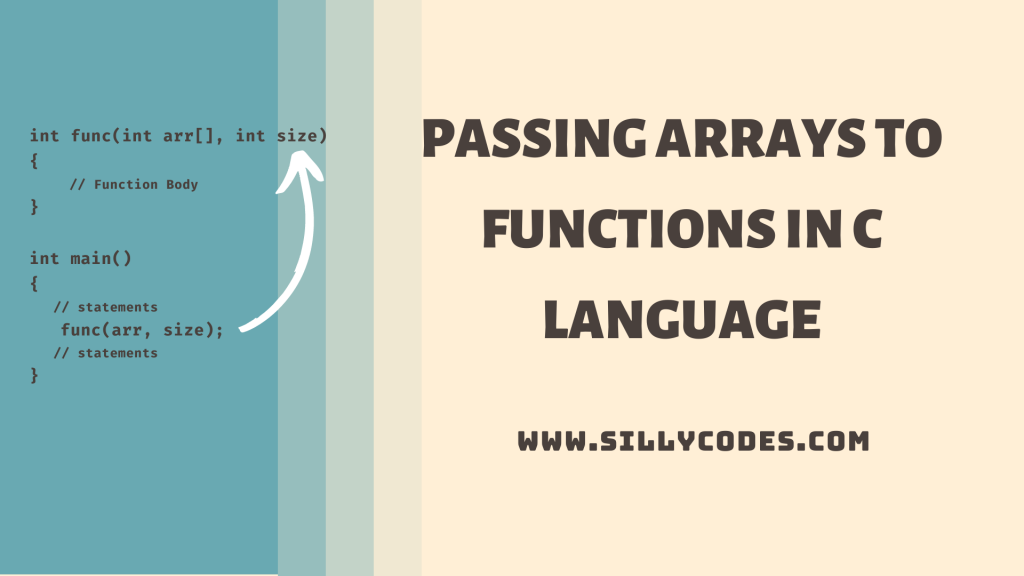
- Introduction:
- Passing Arrays to Functions in C:
- Passing a One-dimensional Array to Function in C:
- Passing arrays to functions in C – Array as the Formal Argument:
- Passing arrays to functions in C – Pointer as the Formal Argument:
- Passing Two-dimensional Arrays (2D arrays) to Function in C:
- Different ways to Pass 2d arrays to functions in C:
- Conclusion:
Introduction:
We looked at the one-dimensional arrays and two-dimensional arrays in our earlier posts, In today’s article, we are going to look at passing arrays to functions in C programming language with example programs.
A function is a basic concept in the C programming language that is a block of code that performs a specific task. One of the primary benefits of using functions is that they allow users to write modular and reusable code, which can make your programs more efficient and manageable.
Prerequisites:
This tutorial is part of the Arrays tutorials series.
- C Arrays – How to Create and Use 1D-Arrays in C language
- Multi-Dimensional Arrays – 2-D arrays in C language with Example Programs
Passing Arrays to Functions in C:
The arrays are passed by address( &) in the C language. So any changes made inside the function will reflect on the caller.
The arrays are similar to the other datatype variables( int, float, etc), so We can also pass them to functions. We can pass the whole array to function or individual array elements. We can pass multi-dimension arrays to functions as well.
📢 Arrays are passed by address, So any changes made in the called function will reflect on the caller function.
Passing a One-dimensional Array to Function in C:
Let’s look at how we can pass a one-dimensional array to function in the C programming language.
Function Call (Actual Arguments):
The C Array name holds the address of the first array element( arr[0]).
- So to pass an array to a function, We can simply specify the array name in the actual arguments like below
- func(array_name, size);
- We can also explicitly use the address of operator(&) in the function call like below
- func(&array_name, array_size);
The above two cases are valid.
When we pass an array to a function, the function has no idea how big the array is, so it can’t tell how many elements are in it. To allow the function to access the entire array, we need to let the function know the size of the array. This can be accomplished in various ways, but one common method is passing the array’s size as a separate argument to the function. That is the reason we are specifying the array_size in the above function calls.
Function Definition ( Formal Arguments ):
There are several syntaxes for formal arguments to receive the array. Let us examine them.
- Array as the Formal Argument
- Pointer as the Formal Argument
The above two methods are valid and they both accept the array address. So any changes we made inside the called function will reflect on the caller. So we need to be careful while modifying the array elements.
Passing arrays to functions in C – Array as the Formal Argument:
Here is the syntax of the array as the formal argument.
1 2 3 4 |
return_type func_name(data_type array_name[], int array_size) { Â Â Â Â // statements } |
In the above code, We used array_name[] array. Let’s look at the program to understand Passing arrays to functions in C language.
Example to understand Passing an arrays to function:
C Program to create a function to print the elements of the passed array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program: Create a Function to print the elements of the array     Author: sillycodes.com */  #include <stdio.h> #define ARRAY_SIZE 10      // Macro with array size  /** * @brief - Function to display the array elements * * @param arr[] - Input array * @param size  - Size of the input array. */ void display(int arr[], int size) {     int i;      // Iterate over the array upto 'size' and print elements     for(i=0; i<size; i++)     {         // print the array element         printf("arr[%d] = %d \n", i, arr[i]);     }  }  int main() {     // Create a array     int arr[ARRAY_SIZE] = {0, 11, 22, 33, 44, 55, 66, 77, 88, 99};      // Pass the array to function     // Function call - Call the display function with array name and size     display(arr, ARRAY_SIZE);      return 0; } |
Program Algorithm:
- The program defines a function display(). The display function takes in an integer array arr[] and its size size as arguments. The display() function doesn’t return any value – void display(int arr[], int size);
- The display function then iterates through all the elements in the array and prints each element along with its index to the standard output. The display function uses two for loops to print the elements.
- We created an array arr of size ARRAY_SIZE and initialized with {0, 11, 22, 33, 44, 55, 66, 77, 88, 99} values in the main function.
- Then the display() function is then called with arr and ARRAY_SIZE as arguments – display(arr, ARRAY_SIZE);
- The display() function will print the elements of arr to the standard output.
- We also used a Macro in the program. The #define ARRAY_SIZE 10 line is a preprocessor directive that defines a macro named ARRAY_SIZE with the value 10. The macro is then used as the size of the arr array in the main() function.
Program Output:
Compile and Run the program using your compiler.
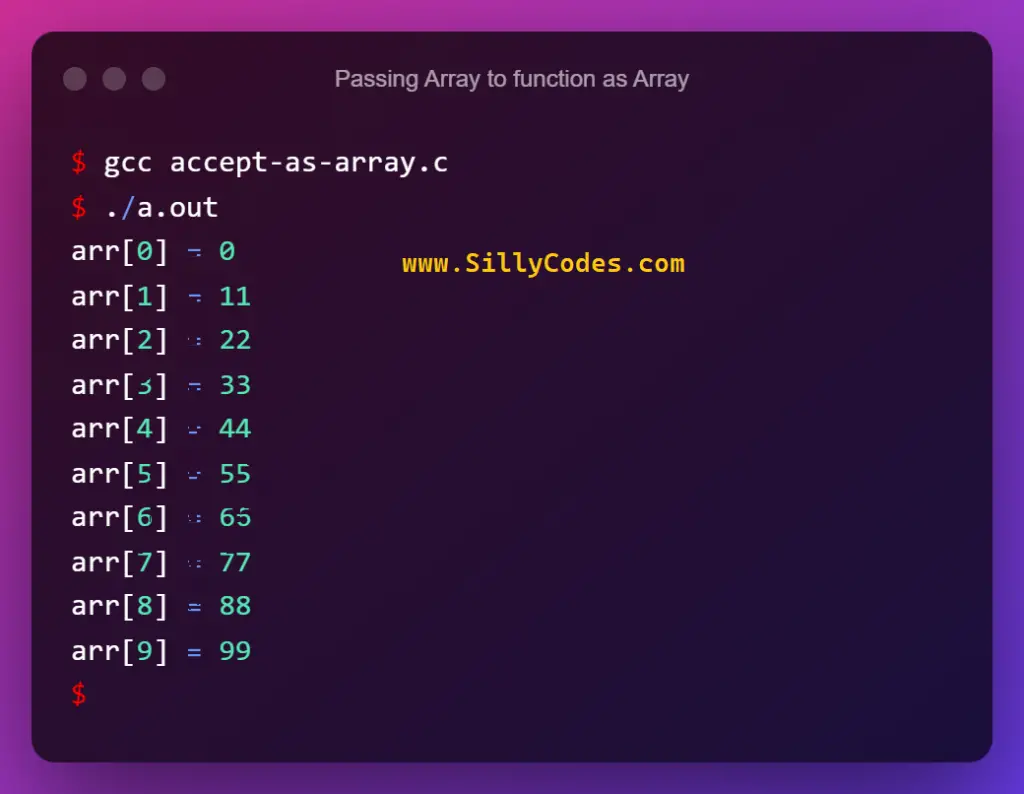
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc accept-as-array.c $ ./a.out arr[0] = 0 arr[1] = 11 arr[2] = 22 arr[3] = 33 arr[4] = 44 arr[5] = 55 arr[6] = 66 arr[7] = 77 arr[8] = 88 arr[9] = 99 $ |
As you can see from the above output, The program displayed all elements of the 2-D array with row and column indices.
Passing arrays to functions in C – Pointer as the Formal Argument:
Here is a program to read input from the user and print it using functions.
Example to understand Passing an array to function (Pointer):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
/*     Program: Function to take input from the user and update array     Author: sillycodes.com */  #include <stdio.h> #define ARRAY_SIZE 5      // Macro with array size  // Function diclaration void display(int *arr, int size);  /** * @brief - Function to read input from user and update the array * * @param arr[] - Input array * @param size  - Size of the input array. */ void readInput(int *arr, int size) {     int i;      printf("Please provide input values for array\n");     // Iterate over the array upto 'size' and print elements     for(i=0; i<size; i++)     {         // Ask for the user input and store it in array.         printf("arr[%d] : ", i);         scanf("%d", &arr[i]);     }  }  int main() {     // Create a array     int arr[ARRAY_SIZE];      // Pass the array to function     // Function call - Call the readInput function with array name and size     readInput(arr, ARRAY_SIZE);       //display the results     // call the display function with (arr, size)     display(arr, ARRAY_SIZE);      return 0; }  /** * @brief - Function to display the array element on standard output * * @param arr[] - Input array * @param size  - Size of the input array. */ void display(int *arr, int size) {     int i;      printf("Array Elements are: \n");     // Iterate over the array upto 'size' and print elements     for(i=0; i<size; i++)     {         // print the array element         printf("arr[%d] = %d \n", i, arr[i]);     }  } |
Program Algorithm:
- Create a macro named ARRAY_SIZE with the size of the array.
- In the main() function, declare an integer array arr with ARRAY_SIZE elements.
- Call the
readInput() function from the
main() function and pass the
arr and its size as arguments. The
readInput() function will prompt the user for input values for each element of the array and update the array.
- The readInput() function takes an integer pointer arr and its size as formal arguments – void readInput(int *arr, int size)
- It then prompts the user for input values for each element of the array( arr).
- The values are read using scanf() function and stored in the corresponding array( arr) element.
- Call the
display() function and pass the
arr and its size as arguments. The
display() function will print the elements of the array to the console.
- The display function also takes an integer pointer arr and size as formal arguments – void display(int *arr, int size)
- Then it uses a for loop to iterate over each element of the arr , it prints the element’s index and its corresponding value.
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
$ gcc accept-as-pointer.c $ ./a.out Please provide input values for array arr[0] : 82 arr[1] : 84 arr[2] : 29 arr[3] : 84 arr[4] : 23 Array Elements are: arr[0] = 82 arr[1] = 84 arr[2] = 29 arr[3] = 84 arr[4] = 23 $ ./a.out Please provide input values for array arr[0] : 8 arr[1] : 3 arr[2] : 4 arr[3] : 1 arr[4] : 2 Array Elements are: arr[0] = 8 arr[1] = 3 arr[2] = 4 arr[3] = 1 arr[4] = 2 $ |
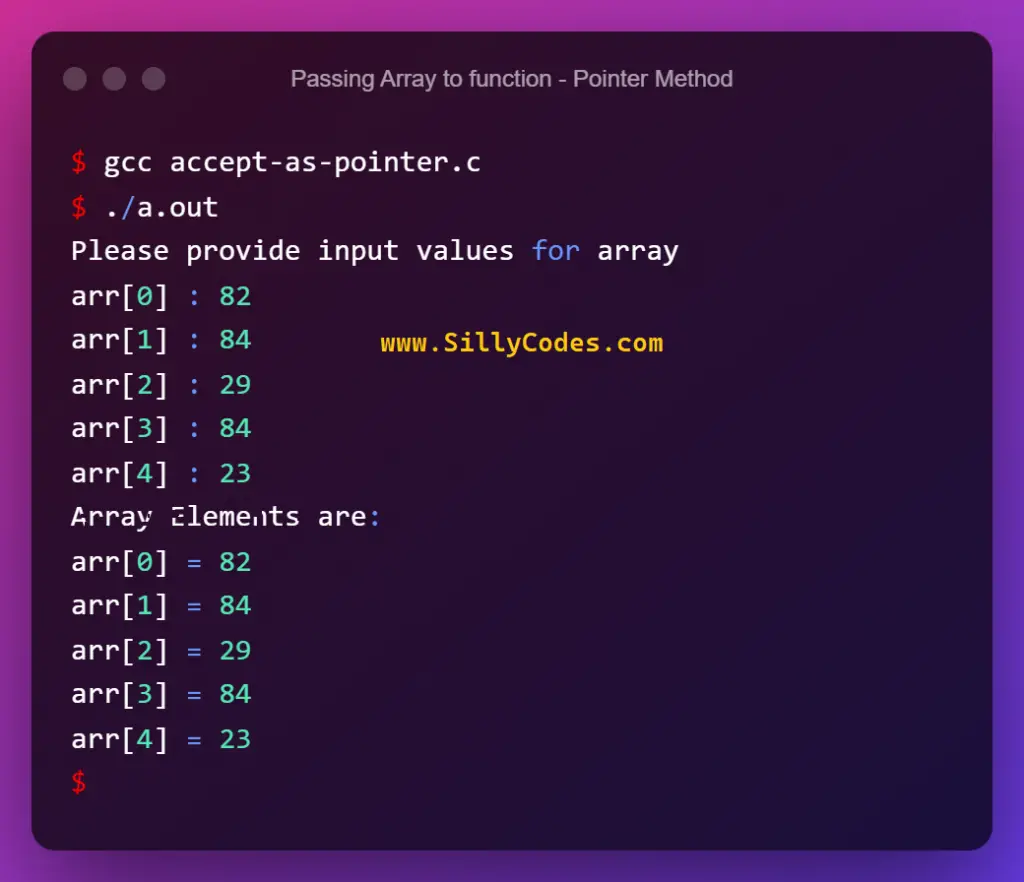
Passing Two-dimensional Arrays (2D arrays) to Function in C:
We use the array or pointer (formal arguments) to pass the two-dimensional array to function.
If we are using the array as a formal argument, Then we must specify the second dimension, have look at below syntax
1 2 3 4 |
int func(int arr[][columns], int rows) { // statements } |
The columns is mandatory as the compiler needs to know how many elements the array contains.
let’s look at an example program to pass a 2-D array to function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program to pass 2-D array to function */ #include<stdio.h> #define COLUMNS 2  void print(int arr[][COLUMNS], int rows) {     int i, j;     // iterate over each row     for(i=0; i<rows; i++)     {         // iterate over each column         for(j=0; j<COLUMNS; j++)         {             printf("arr[%d][%d] = %d \n", i, j, arr[i][j]);         }     } }   int main() {     int i, j;     // Initialize the 2-D array     int arr[4][2] = {         {54, 44},         {65, 17},         {11, 92},         {34, 84}     };      printf("Array is: \n");     // Call the print function     print(arr, 4);      return 0; } |
Here we have created a print function and passed the arr 2D array to print function, The print function accepts the arr 2-D array and prints its elements.
Program Output:
Compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc pass-2d-array.c $ ./a.out Array is: arr[0][0] = 54 arr[0][1] = 44 arr[1][0] = 65 arr[1][1] = 17 arr[2][0] = 11 arr[2][1] = 92 arr[3][0] = 34 arr[3][1] = 84 $ |
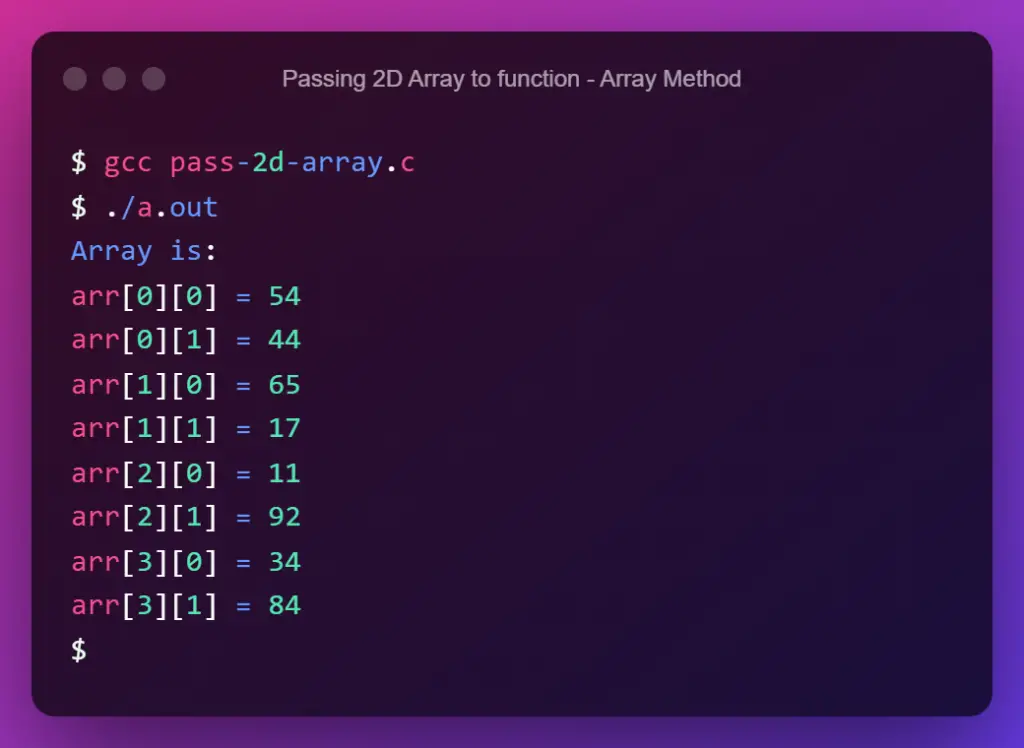
Different ways to Pass 2d arrays to functions in C:
Here are a few other syntaxes we can use to pass the 2-D array to function (Accepting as an 2D array).
1 2 3 4 5 6 7 |
#define ROWSÂ Â 4 #define COLUMNS 3 Â int func(int arr[ROWS][COLUMNS]) { // statements } |
Pointer as formal arguments
1 2 3 4 5 6 7 8 9 10 |
int func(int *array, int rows, int columns) { // statements }  int main() {   // statememts   func(arr, rows, columns); } |
Conclusion:
We looked at how to pass an array to a function, What are the different ways we can pass the array to a function with example programs. We also covered how to pass the multidimensional array or 2-d array to function.
Related Articles:
- Functions in C Language with Example Programs
- Types of Functions in C
- Call by Value and Call by Address / Call by Reference in C Langauge
- Recursion in C Language with Example Programs and Call Flow
- Variable Argument functions in C Language
13 Responses
[…] How to pass arrays to functions in C […]
[…] How to pass arrays to functions in C […]
[…] 📢Related Article: Passing Arrays to Functions in C […]
[…] better understand the following program, Please go through the C Arrays, Functions in C, and How to Pass Arrays to Functions […]
[…] How to pass arrays to functions in C […]
[…] How to pass arrays to functions in C […]
[…] Passing Arrays to the functions in C programming […]
[…] How to pass arrays to functions in C […]
[…] How to pass arrays to functions in C […]
[…] Passing Arrays to Functions in C […]
[…] How to pass Arrays to the Functions […]
[…] Passing 2D Arrays to Functions […]
[…] Passing Arrays to Functions […]