Different ways to Read and Print Strings in C Language
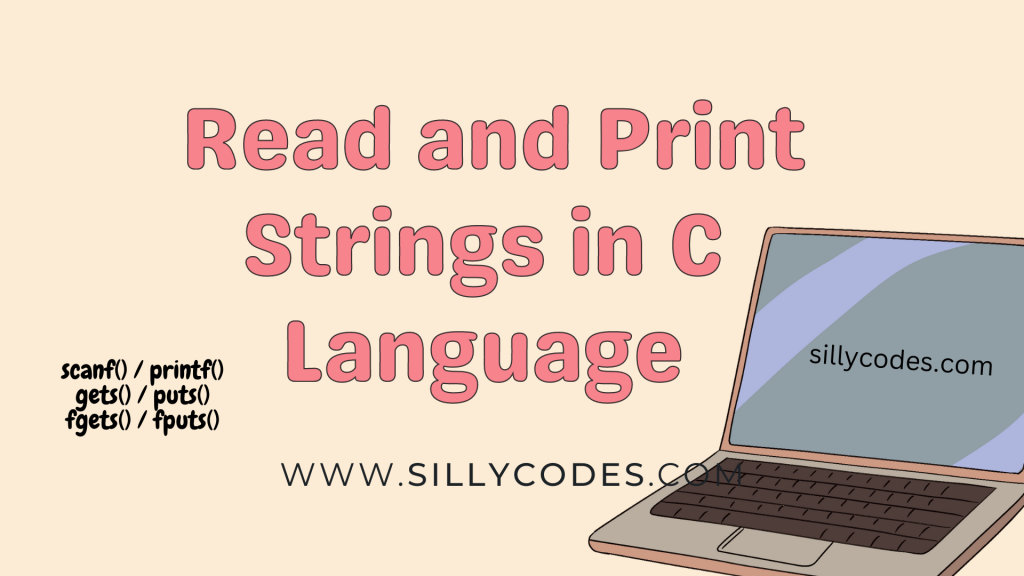
Read and Print Strings in C Language Introduction:
We are going to look at a program to understand how to Read and Print Strings in C programming language. Strings in C language are one-dimensional character arrays. All strings in the C language will terminate with the NULL ( \0) character.
Reading Strings using scanf() function:
We can use the scanf function to read the input from the user. Use the scanf function with the %s format specifier to read the string from the user.
But the scanf() function can only read the input string till it encounters any white space character(such as space, tab, etc). So If you are string contains the white spaces, Then the string up to the first whitespace will be read using the scanf function.
Please have a look at the following example, Where we used scanf() function with %s format specifier to read the input string and store it in variable secret
1 |
scanf("%s", secret); |
Note, That we used the %s format specifier, You find the list of Format specifiers in the following article – Format Specifier in C Language.
Printing strings using the printf() function:
Similar to scanf we can use the printf function with %s format specifier to print the strings on the standard Output
Here is an example to print a string on the console using the printf function.
1 |
printf("%s", secret); |
One thing to note, The printf() function can print the strings with whitespace.
Let’s look at an example to read and print strings from the user.
Read and Print Strings in C using scanf() and printf():
The following program will prompt the user to enter a string, then read the string using the scanf() function and print the string on the console using the printf() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/*     Program to Read and Print String in C 'scanf' and 'printf'     SillyCodes.com */  #include <stdio.h> int main() {     // declare a string     char name[100];      // Read the string from the user     printf("Please enter your name : ");     scanf("%s", name);         // Print the name on the console using printf     printf("Your Name is : %s \n", name);      return 0; } |
Compile and Run the Program.
1 2 3 4 5 |
$ gcc read-print-string.c $ ./a.out Please enter your name : Max Your Name is : Max $ |
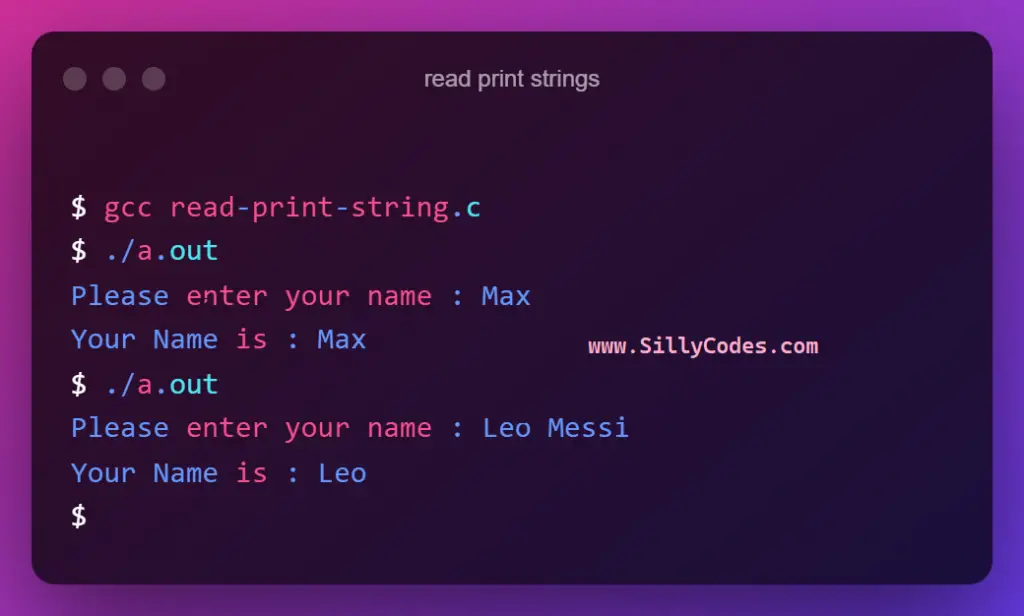
Let’s try to read string with white space (space )
1 2 3 4 |
$ ./a.out Please enter your name : Leo Messi Your Name is : Leo $ |
As excepted, The scanf() function read the input only up to the first white space, So the name variable contains only the Leo
To read the strings with white spaces we need to use other functions like gets and the fgets
Reading strings with whitespaces using gets() function:
The gets() function is used to read a line from the Standard Input (i.e STDIN). The gets function reads the characters until it encounters a New Line ( \n) or EOF (End of File)
Here is the prototype of the gets() function:
char *gets(char * s );
Let’s look at an example to read a string with spaces from the standard input using the gets function.
In the following program, We are going to use a gets function to read a string( name) from the user and printf() function print the name on the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/*     Program to Read and Print String in C 'gets' and 'printf'     SillyCodes.com */  #include <stdio.h> int main() {     // declare a string     char name[100];      // Read the string from the user     printf("Please enter your name(May contain spaces) : ");     gets(name);      // Print the name on the console using printf     printf("Your Name is : %s \n", name);      return 0; } |
Compile and run the program.
1 2 3 4 5 6 7 8 |
$ gcc read-print-string.c $ ./a.out Please enter your name(May contain spaces) : Roger Federer Your Name is : Roger Federer $ ./a.out Please enter your name(May contain spaces) : Lewis Hamilton Your Name is : Lewis Hamilton $ |
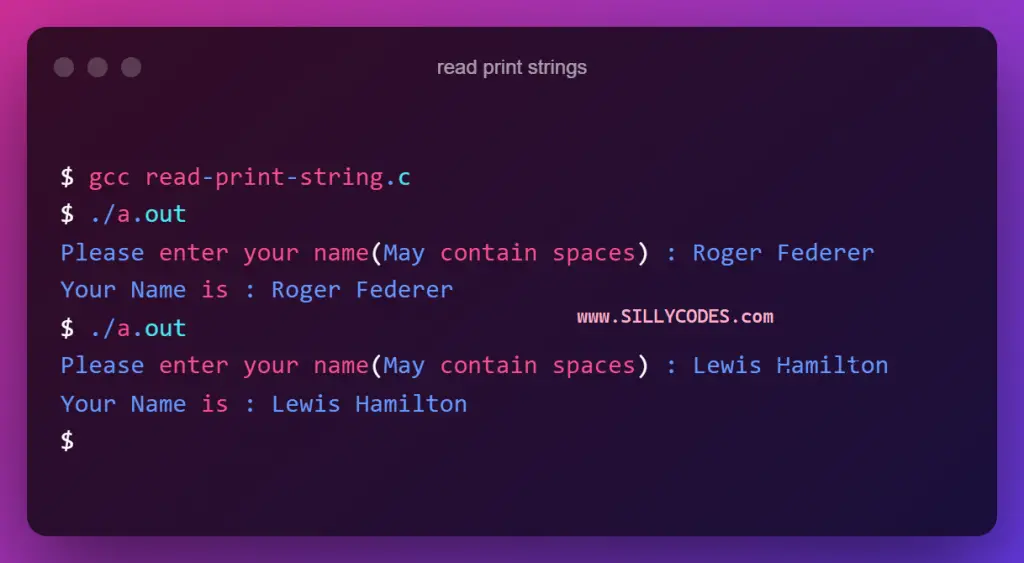
As you can see from the above output, We are able to read the string with spaces using the gets() function.
📢The getsfunction won’t do any Boundary-checks
Printing strings using puts() function:
The puts() function is used to print the string on the standard output. We can use it as an alternative to the printf() function.
Here is the syntax of the puts function in c programming.
int puts(const char *s);
As you can see from the syntax, It takes a constant character pointer(string – s) and writes the string (i.e s) on the console. The puts function also adds the new line character at the end of the string.
Let’s look at an example to read and print strings using the gets and puts functions.
Read and Print Strings in C using gets() and puts() functions Program:
In the following C program, we read a string from the user using the gets function and print the given string on the console using the puts function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/*     Program to Read and Print String in C 'gets' and 'puts'     SillyCodes.com */  #include <stdio.h> int main() {     // declare a string     char name[100];      // Read the string from the user     printf("Please enter your name(May contain spaces) : ");     gets(name);         // Print the name on the console using puts     puts(name);      return 0; } |
Let’s compile and run the following program using GCC compiler (Any compiler)
1 2 3 4 5 |
$ gcc read-print-string.c $ ./a.out Please enter your name(May contain spaces) : Dennis Ritchie Dennis Ritchie $ |
As you can see from the above output, The program is able to read and print the string with space using the gets and puts functions respectively.
📢The getsfunction won’t do any Boundary-checks for the provided buffer. So it is advised to use it with caution or you can use functions like fgetsand getlinefunctions to read the string from the user.
If you notice your compiler might be throwing the following error when you use the gets function – warning: the gets function is dangerous and should not be used.
Reading strings from stdin using fgets() function:
We can also use the fgets() function to read the strings from a stream. In our case, The stream is STDIN (Standard Input).
Syntax of fgets() function:
1 |
char * fgets ( char * s, int size, FILE * stream ); |
The fgets() function takes the input string( s) and size of the buffer (s izeof(s)) and the stream ( STDIN).
So to read the string from the standard input using the fgets() function, Use
1 |
fgets(name, sizeof(name), stdin); |
📢 We use the fgets() function to read the data from the any stream ( FILE, etc)
Priting the strings on stdout using the fputs() function:
The fputs() function is used to write the data to the specified stream. We can use it to print the strings on to the standard output or standard error.
Here is the syntax of the fputs function
int fputs ( const char * s, FILE * stream );
The fputs() function writes string pointed by the s to the file stream stream. So to print the string on the console, We can specify the stream as the stdout.
fputs(name, stdout);
Let’s look at an example program to read and print strings using the fgetsand fputsfunction respectively.
Read and Print Strings using fgets() and fputs() functions in C Language:
The following program will read the strings from the standard input using the fgets function and writes the given string back on the stdout using the fputs function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/*     Program to Read and Print String in C 'fgets' and 'fputs'     SillyCodes.com */  #include <stdio.h> int main() {     // declare a string     char name[100];      // Read the string from the user using fgets     printf("Please enter your name(May contain spaces) : ");     fgets(name, sizeof(name), stdin);      // Print the name on the console using fputs     fputs(name, stdout);      return 0; } |
Let’s compile and run the program.
1 2 3 4 5 |
$ gcc read-print-string-fgets-fputs.c $ ./a.out Please enter your name(May contain spaces) : Rafa Nadal Rafa Nadal $ |
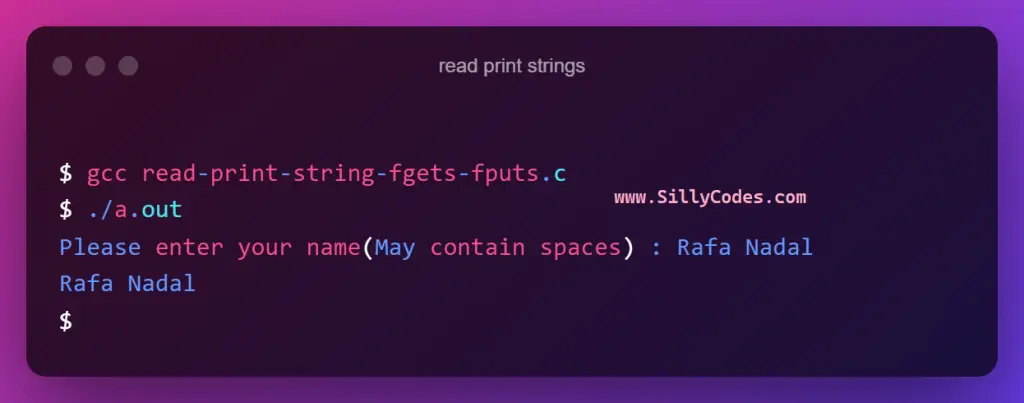
As we can see from the above output, We read the user input using the fgets() function and printed the given string using the fputs() function.
📢 We use the fgets() and fputs() functions to read and write the data from any file stream ( FILE, etc)
Conclusion:
We have looked at the different methods to read and print the strings in the c programming language. We started with the normal printf() function and scanf() function and then looked at the gets() function and puts() function and finally we looked at the fgets() and fputs() function to read and print the strings respectively.
Related Articles:
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- Passing Arrays to Functions
9 Responses
[…] earlier articles, We looked at the strlen function and strcpy functions. In today’s article, We will look at the strcat in C Programming language with example […]
[…] Different ways to Read and Print strings in C […]
[…] Different ways to Read and Print strings in C […]
[…] Read and Print Strings in C Language […]
[…] Different ways to Read and Print strings in C […]
[…] Different ways to Read and Print strings in C […]
[…] Different ways to Read and Print strings in C […]
[…] Read the two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings. […]
[…] Different ways to Read and Print Strings in C […]