Recursion in C Language with Example Programs
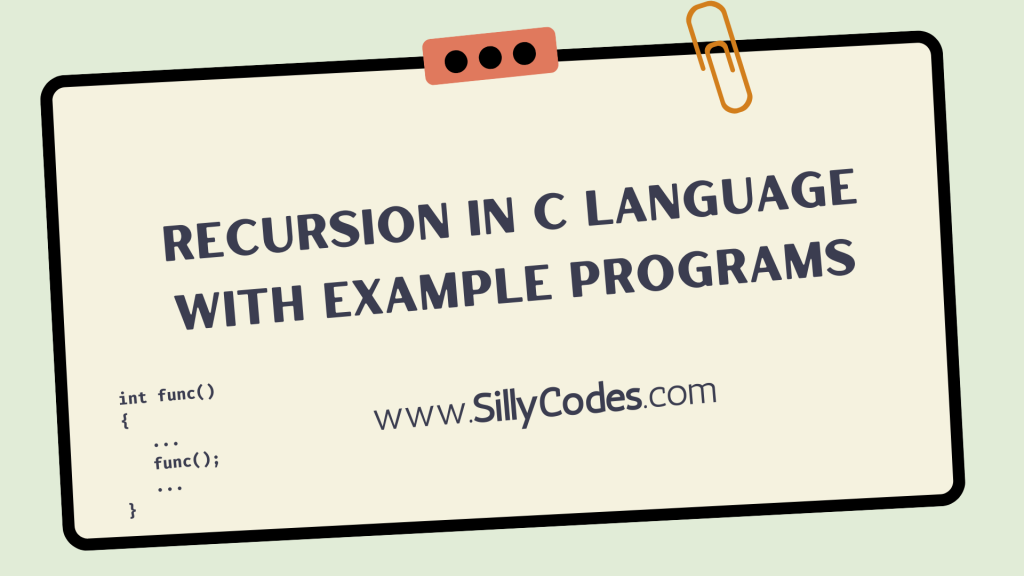
- Introduction:
- Recursion in C language:
- How does the recursion work in C Language? ( Recursive function call flow):
- Example 1: Factorial of a number using Recursion in C Language:
- Factorial of Number(fact) Recursive functions call flow:
- Example 2: Sum of Digits of a Number using Recursion in C Language:
- Sum of Digits of a number recursive function Explanation:
- Advantages and disadvantages of Recursion:
- Conclusion:
- Related Articles:
- Related Programs:
Introduction:
We have looked at the call by value and call by reference in our earlier posts, In today’s article, We are going to look at the recursion in c language with Example Programs.
Recursion in C language:
Function calling itself is called recursion. Any function which calls itself is called a recursive function.
If we have a complex problem, Then break down the complex problem into smaller and simpler problems. and try to solve those smaller problems(using a function and calling it repetitively) and finally add all these smaller problems to create the complete solution. It will make sense once we look at an example program.
The recursive functions are used extensively in data structures and algorithms, For example, To traverse a tree (inorder, preorder, and post-order traversal) we use the recursion.
📢Function calling itself is called recursion. Any function which calls itself is called a recursive function.
Syntax of Recursive function:
Here is the syntax of the recursive function ( Recursion in C )
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
void recursive_fun() {     // statments     ....     recursive_fun();     ....     // statements }   int main() {     // statements     ....     recursive_fun();     ....     // statements  } |
How does the recursion work in C Language? ( Recursive function call flow):
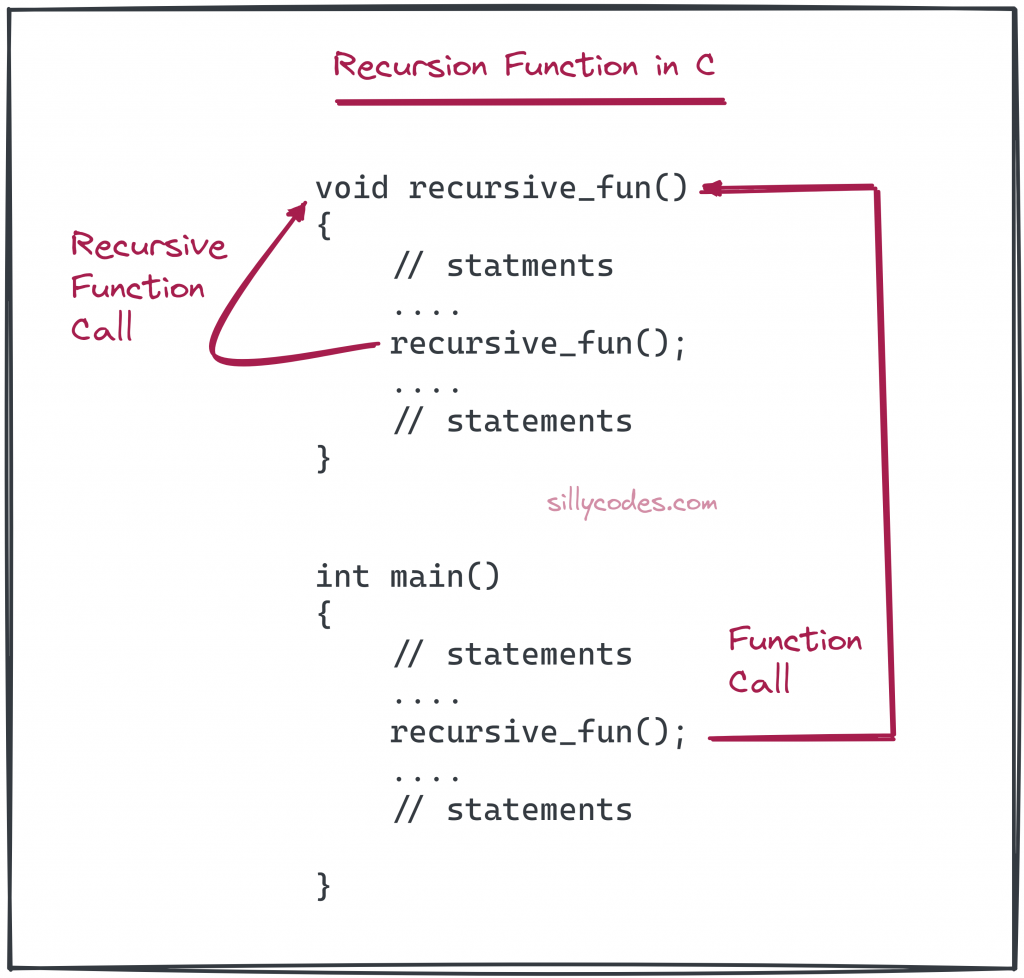
If we look at the above syntax of the recursive function, We can see that we called the recursive_fun() from the main() function. Which takes the program control to recursive_func().
Inside the body of the recursive_func(), We called the recursive_func() again. ( Function called itself). So we will execute the recursive_fun() again, which calls recursive_func() again. This process continues and creates an Infinite recursion.
To solve the Infinite recursion, We need to have the Base Condition or Exit condition for the recursive function. Typically we use if else statement to stop the recursion when a certain condition is met. ( so that function stops calling itself) and execution unravels back to the main().
For each recursive function call, One stack frame is pushed to the stack, Which contains the local variables, formal arguments, and return address of function. So recursive calls take memory in the RAM ( stack ).
To write a recursive function,
- We should be able to divide the problem into similar and smaller subtasks ( So that we can call the same function again and again)
- We should also decide the proper base condition for the recursion So that we can stop the recursion. Otherwise, we might get into the infinite recursion.
Let’s look at a couple of example programs to understand the recursion in the C programming language.
Example 1: Factorial of a number using Recursion in C Language:
Write a C Program to calculate the factorial of a number using the recursion.
We have already looked at the factorial of the number program earlier using the iterative method, In this program, we will calculate the factorial using the recursion.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/*     Program: Calculate Factorial using recursion in C */  #include<stdio.h>  // function declaraion int fact(int);  // main function int main() {     int num;      // Take user input     printf("Enter a Number to calculate factorial : ");     scanf("%d", &num);      // call the fact() function     printf("Factorial of %d is : %d \n", num, fact(num));      return 0; }  /*     arguments_list: int     return_type: int     Description: The fact function calculates factorial recursively. */ int fact(int n) {     // base condition     if(n == 0)         return 1;     else     {         // recursive call with 'n-1'         return ( n * fact(n-1));     } } |
Program Output:
Let’s compile and run the program.
1 2 3 4 5 |
$ gcc factorial-recursive.c $ ./a.out Enter a Number to calculate factorial : 5 Factorial of 5 is : 120 $ |
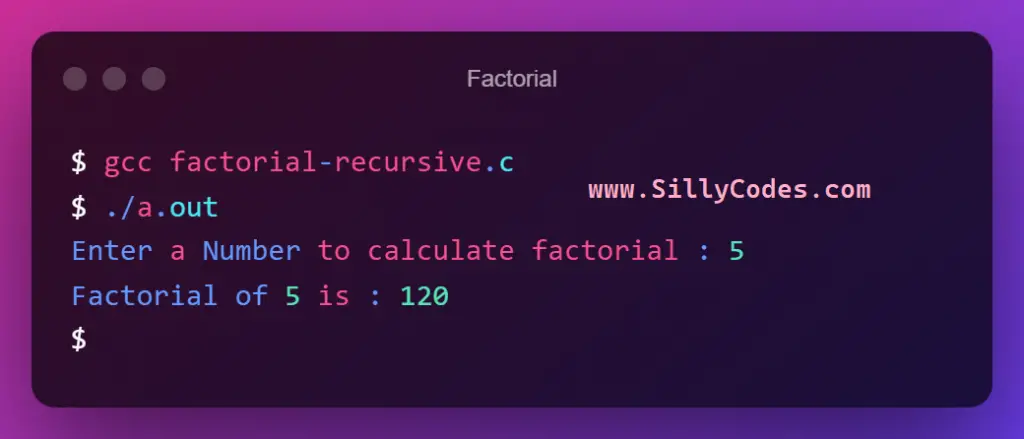
Factorial of Number(fact) Recursive functions call flow:
- The main() function started by asking for the user input and we stored it in the variable num. Let’s say the user provided the num as the 5.
- Then we called the fact function with the user input i.e fact(num) which fact(5)
- The program counter jumped to fact() function and started executing the fact() function.
- As we have learned earlier, To create the recursive function, We need to divide the problem into smaller subtasks and should have a base condition. Here the base condition is if(n == 0), As we know the Factorial of the number 0 is 1. So we know that we reached the end, So we can stop our recursion.
- Call From main() function : The Initial value of the number
n is not 0, which is
5(
n=5), So the
base condition won’t be executed and we go to the
else statement. Where we used the
return ( n * fact(n-1));, Here we are calling the
fact function again, but this time, Instead of calling with
n, We are calling it with
n-1. And we are also multiplying the result of
fact(n-1) with
n.
- return ( n * fact(n-1)); which is return ( 5 * fact(5-1));
- First Recursive Call : The above
fact(n-1) call creates a recursive call to
fact function, As the value of
n for the second call is
4, The base condition won’t be executed, We will go to the else statement again. Where we will call the
fact function again with
n=n-1 which is
3.
- return ( 4 * fact(4-1) ); which is return ( 4 * fact(3) );
- Second Recursive Call: In the next recursive call, The value of
n will be
3, The base condition won’t be executed, We will go to the else statement again. Where we will call the
fact function again with
n=n-1 which is
2.
- return ( 3 * fact(3-1) );which is return ( 3 * fact(2) );
- Third Recursive Call: In the next recursive call, The value of
n will be
2, The base condition won’t be executed, We will go to the else statement again. Where we will call the
fact function again with
n=n-1 which is
1.
- return ( 2 * fact(2-1) );which is return ( 2 * fact(1) );
- Fourth Recursive Call: In the next recursive call, The value of
n will be
1, The base condition
if(n == 0) won’t be executed, We will go to
else statement again. Where we will call the
fact function again with
n=n-1 which is
.
- return ( 1 * fact(1-1) );which is return ( 1 * fact(0) );
- Fifth Recursive Call: Now in this recursive call to
fact function, The
n value became
. So our base condition
if(n==0) will be
True, As we reached the
number = 0, We stop the recursion and return
1 ( As the factorial of
is
1). Which takes the program control back to above step – 9.
- This function returns 1
- Now one by one each recursive function returns in the backward direction ( stack unwinding ) and returns
n * fact(n-1)
- Fifth call return 1
- Fourth recursive call returns 1 * fact(0) which is 1 * 1 and fact(1) = 1, return 1
- Third recursive call returns 2 * fact(1) which is 2 * 1 and return 2
- Second recursive call returns 3 * fact(2) which is 3 * 2 and return 6
- First recursive call returns 4 * fact(3) which is 4 * 6 and return 24
- Call from main() function returns return ( 5 * fact(4)); which is equal to 5 * 24, i.e 120. So the function fact returns value 120 to the main() function.
- The main() function gets 120 and prints on the console using the printf function.
Here is the pictorial representation of the Factorial Recursive function call flow or Stack flow:
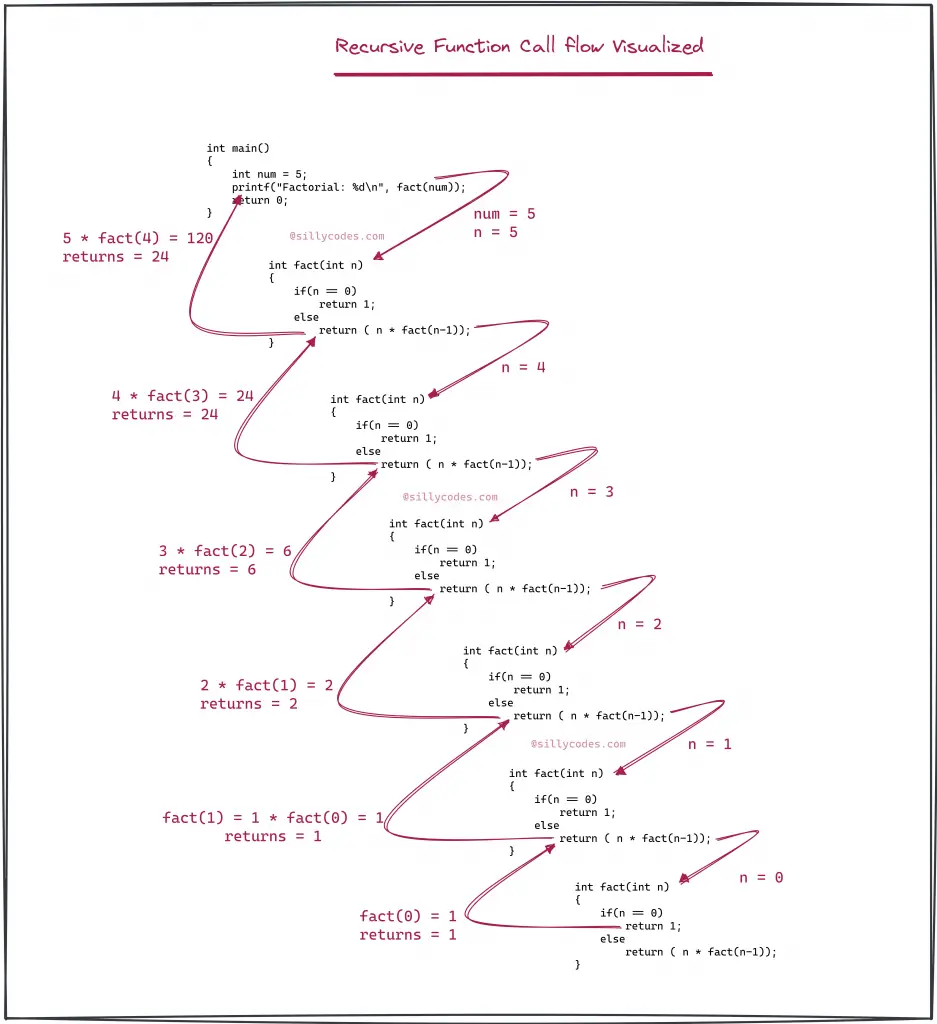
Example 2: Sum of Digits of a Number using Recursion in C Language:
Let’s look at another program to make our understanding concrete. Write a C Program to calculate the sum of digits of a number using the recursive function.
📢 Related Program: Sum of digits program using Iterative method (Loops)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/*     Program: Sum of Digits of a number using Recursion */  #include<stdio.h>  // function declaraion int sumOfDigits(int);  // main function int main() {     int n;      // Take Input     printf("Enter a Number : ");     scanf("%d", &n);      // Print Results     printf("Sum of digits of number %d is : %d \n", n, sumOfDigits(n));      return 0; }   /*     Function_name: sumOfDigits     arguments_list: int     return_type: int     Description: Calculate the sum of digits of number 'n' */ int sumOfDigits(int n) {     // Our Base Condition     if(n == 0)         return 0;         /*       Get the last number using modulus operator       Then call the sumOfDigits by removing last number by using division operator.     */     return ( (n % 10) + sumOfDigits(n / 10) ); } |
Program Output:
1 2 3 4 |
$ gcc sum-of-digits-recursive.c $ ./a.out Enter a Number : 921 Sum of digits of number 921 is : 12 $ |
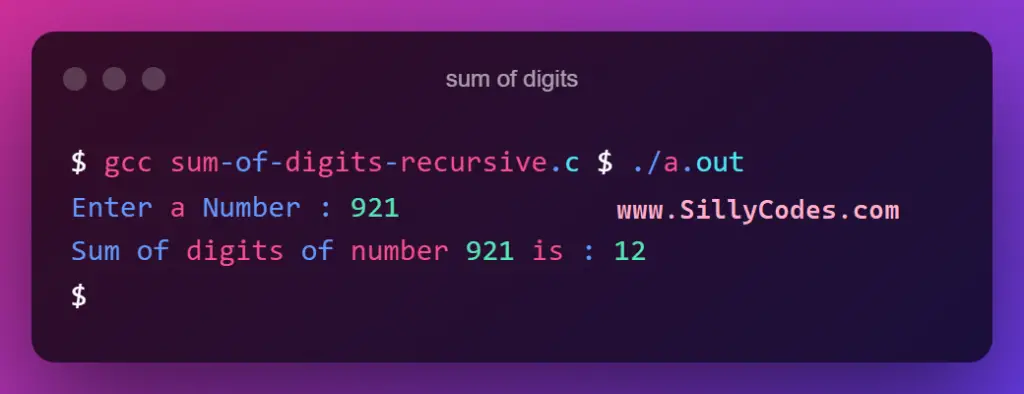
Sum of Digits of a number recursive function Explanation:
- The program starts by asking input number from the user and stores it in variable n. Let’s say the user provided the number 921.
- Then main() function calls, The sumOfDigits function with n(which is 921) to get the sum of digits in the number n.
- Call from Main() Function: sumOfDigits() function execution will start, and we used the if(n == 0) as our base condition. The base condition will be False, So we continue to the next statement.
- Here
return ( (n % 10) + sumOfDigits(n / 10) );, we are getting the last element from the given number
n using the Modulus operator using
n%10, Then we are calling the
sumOfDigits function (Recursive call) and passing the
n/10 ( which actually removes the last digit as we already processed it)
- Let’s apply the example values – i.e n = 921
- return ( (921 % 10) + sumOfDigits(921 / 10) );
- return ( (1) + sumOfDigits(92) );
- First Recursive Call: The
sumOfDigits(92) creates a recursive call to
sumOfDigits function. Now the
n value is
92, So the base condition will be
False, We go to the next statement and make another recursive call.
- return ( (92 % 10) + sumOfDigits(92 / 10) );
- return ( (2) + sumOfDigits(9) );
- Second Recursive Call: The value of
n is
9, So the base condition will be
False, We will go to the next statement and makes another recursive call.
- return ( (9 % 10) + sumOfDigits(9 / 10) );
- return ( (9) + sumOfDigits(0) );
- Third Recursive Call: The value of n is . So the base condition n == 0 will be executed and the sumOfDigits function returns with value .
- Now all The functions start to return one by one in the backward direction, Here are the return values of each function
- Second Recursive Call returns ( 9 + 0 ) which is 9
- First Recursive Call returns ( 2 + 9 ) which is 11
- The sumOfDigits function returns ( 1 + 11 ) which is 12. and sumOfDigits function returns value to main function.
- Finally, the Main() function prints the result onto the console.
Advantages and disadvantages of Recursion:
- Recursion makes code compact and elegant.
- We can easily solve many data structures and algorithm problems using Recursion.
- Memory Usage: As we know, For each recursive function call, There will be a stack frame pushed to memory(Stack region), So there will be a memory overhead for recursion. If you use a high number of recursive calls, Then we might end up using a lot of extra RAM.
- The recursive function should have the appropriate base condition, Otherwise, We might create the Infinite recursive function.
- Recursion is slower compared to the iterative method (Loops) due to the cost of stack push and pops.
- Debugging the recursive functions is hard.
Conclusion:
We have looked at the Recursion in C Programming Language with Example Programs. We also looked at the recursive function call flow and how functions are pushed to the stack and how recursive functions return to create the final output. Finally, We looked at the advantages and disadvantages of the recursive functions (recursion in general).
Related Articles:
Related Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- Arithmetic Operations using Functions
- Add Two Numbers using Function
- 100+ C Practice Programs
- C Tutorials Index
3 Responses
[…] Recursion in C […]
[…] have discussed the Recursion and Call by value and Call by Reference in our Earlier articles, In today’s article, we are […]
[…] Recursion in C Language with Example Programs and Call Flow […]