2D arrays in C (Multi-dimensional arrays) language
- 2D arrays in C Introduction:
- Multi-Dimensional Array in C:
- Two-Dimensional Arrays or 2-D Arrays in C Language:
- Declaring 2-D Arrays in C:
- Accessing the 2-D arrays:
- Initialization of 2D arrays in C:
- Program to understand the two-dimensional or 2-D arrays in C Programming language:
- Program to Input and Display the 2-D array or Matrix (Read and Print 2-D Array):
- Example Program 2: Sum of all elements of 2D Arrays in C Language:
- Multi-dimensional Arrays Practice Programs (Matrix Programs):
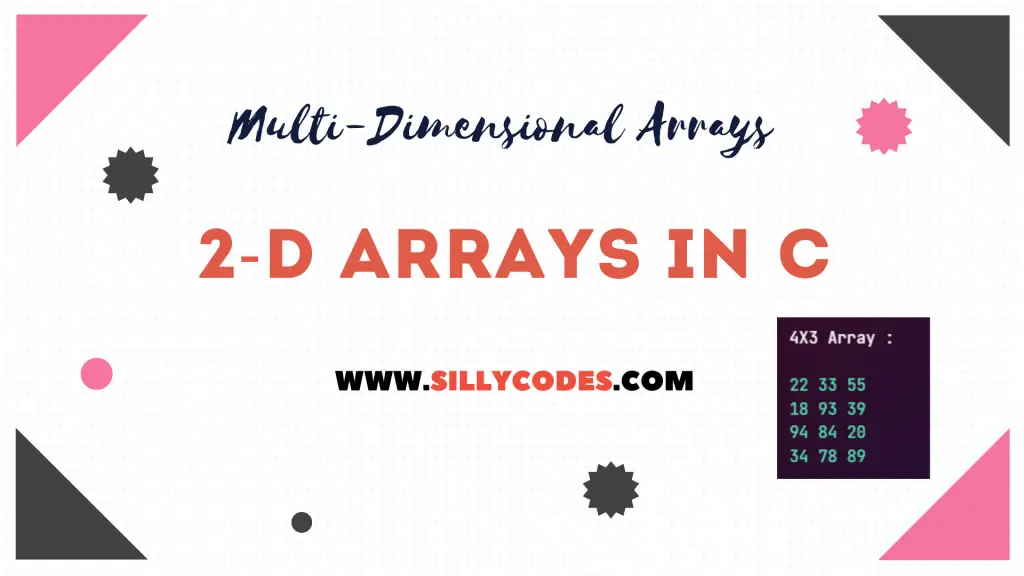
2D arrays in C Introduction:
We have looked at the one-dimensional arrays in our earlier article, In today’s article, We are going to look at Multi-Dimensional Arrays, In particular 2D arrays in C language – How to create, access, and modify the 2-D arrays in C programming language.
📢 It is recommended to know the array basics to understand the 2D array. Please go through the following article to learn more about one-dimensional arrays.
Multi-Dimensional Array in C:
The multi-dimensional arrays are the array of arrays. Which means each element in the multi-dimensional array is an array.
The multi-dimensional arrays are also called Matrix.
C language supports different dimensional arrays like,
- One dimensional array or 1-D Array – The One dimensional array is an array of elements/values(Int, float, etc)
- Two-Dimensional Array or 2-D Array – The Two-dimensional array is an array of 1-D arrays. So each element in the 2D array is a 1-D array.
- Three-Dimensional Array or 3-D Array – The three-dimensional array is an array of 2-D Arrays. So each element is a 2D array.
- …
- …
- N-Dimensional Array or N-D Array – The N-Dimensional array is an array of N-1 arrays
Syntax of multi-dimensional Array
1 2 |
// Example of N-D Array data_type array_name[SIZE1][Size2][Size3]....[SizeN]; |
We will look at two-dimensional arrays or 2-D arrays in this article.
📢 Related Programs: Arrays Practice Programs in C
Two-Dimensional Arrays or 2-D Arrays in C Language:
The Two Dimensional Arrays or 2-D arrays are collections of one-dimensional arrays ( Array of 1-D Arrays). We can create two-dimensional arrays by specifying the two subscripts [ ].
The two-dimensional array elements are stored in the contiguous memory. We can represent a 2-D array as the Matrix.
The 2-D arrays are used to store and manipulate data in tabular format, with each row representing a different record and each column representing a different field or attribute of that record.
Declaring 2-D Arrays in C:
Syntax of the 2-D array declaration.
1 |
data_type array_name[row_size][column_size]; |
Where
- data_type – The data type of the array elements
- array_name – Name of the 2-D array
- row_size – The number of rows in the 2-D array
- column_size – The number of columns in the 2-D array
📢 The total number of elements in the two-dimensional array is equal to the product of row_size and column_size.
Total Elements in 2-D Array = row_size * column_size
Example of 2-D Array:
1 2 |
// 3X4 Array. int data[3][4]; |
Here we created a 2-D array called data which has 3 rows and 4 columns (using two subscripts). The data array stores integer data type values. The data array contains the 12 elements ( 3 * 4)
Accessing the 2-D arrays:
To access the individual elements of the array, We can use the row and column indices.
The element in the 2-D array can be accessed by using the array_name[row_index][column_index], Here the row_index and column_index are the indices of the rows and columns which start from zero(0).
So the First element of the 2-D array will be stored at array_name[0][0]
Here is the representation of the above int data[3][4] array.
Column 0 | Column 1 | Column 2 | Column 3 | |
---|---|---|---|---|
Row 0 | data[0][0] | data[0][1] | data[0][2] | data[0][3] |
Row 1 | data[1][0] | data[1][1] | data[1][2] | data[1][3] |
Row 2 | data[2][0] | data[2][1] | data[2][2] | data[2][3] |
As specified earlier, We can access 2-D array elements using the row index and column index.
- To access the element of the First row and third column, We can use the data[0][2]
- To access the element of the Third row and First column, We can use the data[2][0]
📢The rows and column index of the two-dimensional / 2-D array starts with the index Zero(0).
Initialization of 2D arrays in C:
The two-dimensional arrays can be initialized by specifying the values for the array elements like this.
1 2 3 4 5 6 7 |
// Array with 3 Rows and 5 Columns  int arr[3][5] = {         {10, 20, 30, 40, 50},          // First Row         {60, 70, 80, 90, 100},        // Second Row         {110, 120, 130, 140, 150}      // Third Row }; |
Here we have created an array called arr, which has 3 Rows and 5 columns. Here we used curly braces to divide and represent each row.
We can create the 2-D arrays by specifying the all elements in single curly braces like below.
1 2 3 |
// Method 2: Â int arr[3][5] = { 10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120, 130, 140, 150 }; |
The second method is not readable and error-prone. So it is a good idea to stick to the first method.
If you are Initializing the 2-D array, Then the first dimension( row_size) is optional. But you have to specify the second dimension size.
1 2 3 4 5 |
int arr[][5] = { Â Â Â Â Â Â Â Â {10, 20, 30, 40, 50}, Â Â Â Â Â Â Â Â {60, 70, 80, 90, 100}, Â Â Â Â Â Â Â Â {110, 120, 130, 140, 150} }; |
If you don’t specify the second dimension size,
1 2 3 4 5 |
int arr[3][] = { Â Â Â Â Â Â Â Â {10, 20, 30, 40, 50}, Â Â Â Â Â Â Â Â {60, 70, 80, 90, 100}, Â Â Â Â Â Â Â Â {110, 120, 130, 140, 150} Â Â Â Â }; |
Then you probably will get the compilation error like note: declaration of ‘arr’ as multidimensional array must have bounds for all dimensions except the first
Program to understand the two-dimensional or 2-D arrays in C Programming language:
C Program to Initialize and display the 2-D array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/*     Program to understand 2-D Array     Sillycodes.com */ #include<stdio.h> int main() {     int i, j;     // Initialize the 2-D array     int arr[3][5] = {         {10, 20, 30, 40, 50},         {60, 70, 80, 90, 100},         {110, 120, 130, 140, 150}     };      // int arr[3][5] = { 10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120, 130,  };      // Print the 2-D array      // iterate over each row     for(i=0; i<3; i++)     {         // iterate over each column         for(j=0; j<5; j++)         {             printf("arr[%d][%d] = %d \n", i, j, arr[i][j]);         }     }     return 0; } |
2-D Array Example Program Explanation:
In this program, we created a two-dimensional array arr. which holds integer elements. The arr[3][5] array contains 3 rows and 5 columns.
To display the two-dimensional array we need to use two for loops ( Similarly, we need 3 loops to print the 3-d array,..)
- The outer for loop is used to iterate over the rows. So the outer for loop started from the index and went till the 3. – for(i=0; i<3; i++)
- The Inner For loop is used to iterate over the columns. As the arr array contains 5 columns, The inner for loop will start from goes till 5 – for(j=0; j<5; j++)
- At each iteration, Print the array element using row and column indices – arr[i][j]
Program Output:
Compile and Run the program.
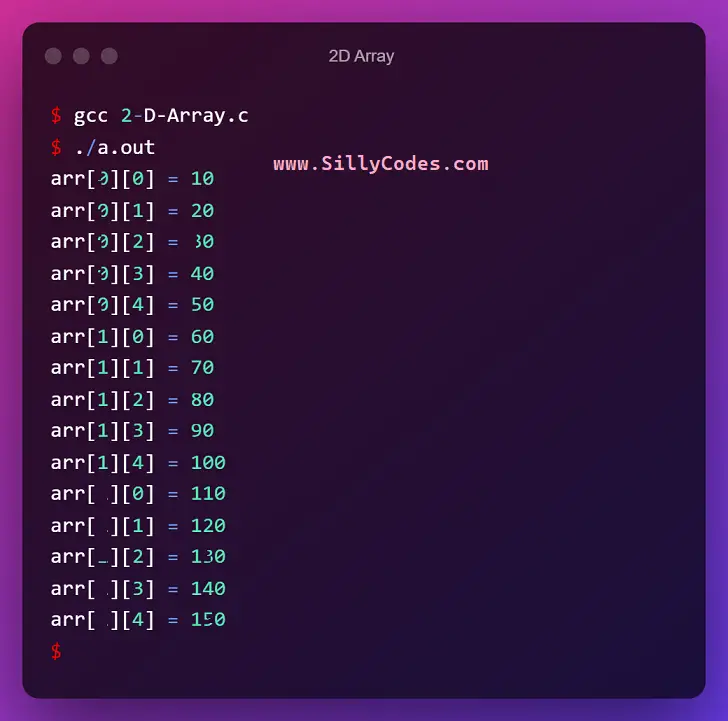
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc 2-D-Array.c $ ./a.out arr[0][0] = 10 arr[0][1] = 20 arr[0][2] = 30 arr[0][3] = 40 arr[0][4] = 50 arr[1][0] = 60 arr[1][1] = 70 arr[1][2] = 80 arr[1][3] = 90 arr[1][4] = 100 arr[2][0] = 110 arr[2][1] = 120 arr[2][2] = 130 arr[2][3] = 140 arr[2][4] = 150 $ |
As you can see from the above output, The program is displaying all elements of the two-dimensional array (2-D array) with element indices.
Program to Input and Display the 2-D array or Matrix (Read and Print 2-D Array):
C Program to read the 2-D array input from the user and display all elements. We use for loops to iterate over the 2-D array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     Program to Read and Print the 2-D array     Sillycodes.com */ #include<stdio.h> int main() {     int i, j;     // Initialize the 2-D array     // Array with 4-Rows and 3-Columns     int data[4][3];      // Take the user input and update the array.     printf("Please provide input for 4X3 Array\n");     // iterate over each row     for(i=0; i<4; i++)     {         // iterate over each column         for(j=0; j<3; j++)         {             printf("data[%d][%d] : ", i, j);             scanf("%d", &data[i][j]);         }     }      // Print the 2-D array     printf("Provided 4X3 Array : \n");     // iterate over each row     for(i=0; i<4; i++)     {         // iterate over each column         for(j=0; j<3; j++)         {             printf("%d ", data[i][j]);         }         // add new line after each row         printf("\n");     }      return 0; } |
Program Explanation:
- We created a 2-D array data[4][3]. The data array holds 4 rows and 3 columns.
- Two for loops are used to accept user input. The outer for loop iterates over the rows, while the inner for loop iterates over the columns. At each iteration, obtain the integer data from the user and store it in data[i][j] using the scanf function.
- To display all array elements of a 2-D Array. We should follow similar steps as input. So, use two for loops to iterate over rows and columns, printing the data[i][j] value at each iteration using printf function.
Program Output:
Compile and run the program using your favorite compiler.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
$ gcc 2-D-input-output.c $ ./a.out Please provide input for 4X3 Array data[0][0] : 1 data[0][1] : 2 data[0][2] : 3 data[1][0] : 4 data[1][1] : 5 data[1][2] : 6 data[2][0] : 7 data[2][1] : 8 data[2][2] : 9 data[3][0] : 10 data[3][1] : 11 data[3][2] : 12 Provided 4X3 Array : 1 2 3 4 5 6 7 8 9 10 11 12 $ $ ./a.out Please provide input for 4X3 Array data[0][0] : 22 data[0][1] : 33 data[0][2] : 55 data[1][0] : 18 data[1][1] : 93 data[1][2] : 39 data[2][0] : 94 data[2][1] : 84 data[2][2] : 20 data[3][0] : 34 data[3][1] : 78 data[3][2] : 89 Provided 4X3 Array : 22 33 55 18 93 39 94 84 20 34 78 89 $ |
Example Program 2: Sum of all elements of 2D Arrays in C Language:
Write a C Program to calculate the sum of all elements in a 2D Array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/*     Program to calculate sum of all elements of 2-D array     Sillycodes.com */ #include<stdio.h> int main() {     int i, j, sum=0;     // Initialize the 2-D array     // Array with 4-Rows and 3-Columns     int values[3][3];      // Take the user input and update the array.     printf("Please provide input for 4X3 Array\n");     // iterate over each row     for(i=0; i<3; i++)     {         // iterate over each column         for(j=0; j<3; j++)         {             printf("values[%d][%d] : ", i, j);             scanf("%d", &values[i][j]);         }     }      // iterate over each row     for(i=0; i<3; i++)     {         // iterate over each column         for(j=0; j<3; j++)         {             sum = sum + values[i][j];         }     }      // Print the results     printf("Sum of all elements of values array is : %d \n", sum);      return 0; } |
Sum of 2D array elements Program explanation:
The sum of all elements of the 2D array program adds all elements in the 2D array and returns the result.
- The program creates a values A 2-d array of size [3][3], which means the values array contains three rows and three columns.
- The next step is to take the user input and update the array. We used two for loops to read the input from the user and updated the array elements.
- Create a variable called sum and initialize with zero(0). The sum variable holds the sum of array elements.
- Then we need to go through the all elements of the array and add each element to the sum variable.
- Display the sum variable on the standard output (console)
Program Output:
Let’s compile and run the program and observe the output.
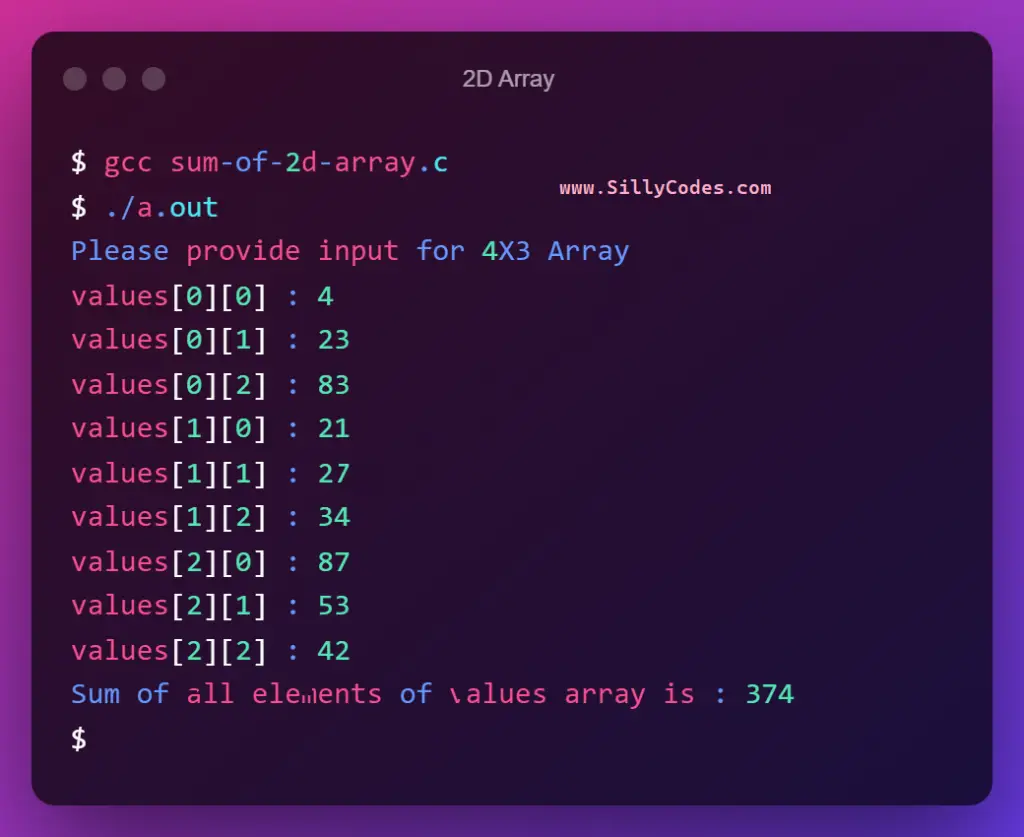
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
$ gcc sum-of-2d-array.c $ ./a.out Please provide input for 4X3 Array values[0][0] : 4 values[0][1] : 23Â Â values[0][2] : 83 values[1][0] : 21 values[1][1] : 27 values[1][2] : 34 values[2][0] : 87 values[2][1] : 53 values[2][2] : 42 Sum of all elements of values array is : 374 $ ./a.out Please provide input for 4X3 Array values[0][0] : 8 values[0][1] : 7 values[0][2] : 9 values[1][0] : 2 values[1][1] : 3 values[1][2] : 4 values[2][0] : 1 values[2][1] : 9 values[2][2] : 7 Sum of all elements of values array is : 50 $ |
Conclusion:
We have learned about multidimensional arrays in c programming, In particular, we concentrated on 2d arrays in C language. We looked at how to declare, initialize, and process the 2d arrays. Finally, we looked at a couple of examples to make our understanding concrete.
In the next article, We are going to look at How to Pass the Arrays to functions.
Multi-dimensional Arrays Practice Programs (Matrix Programs):
- C Program to Read and Print Matrix or 2D Array
- C Program to demonstrate How to Pass 2D Array to Functions
- C Program to Multiplication of two Matrices
- C Program to Add Two Matrices
- C Program to Subtract Two Matrices
- C Program to Calculate the Transpose of Matrix
- C Program to Check Two Matrices are Equal
- C Program to Check Multiplicability of Two Matrices
- C Program to Check a Sparse Matrix
- Matrix and Scaler Multiplication Program in C
13 Responses
[…] 2D arrays in C with Example programs […]
[…] looked at the one-dimensional arrays and two-dimensional arrays in our earlier posts, In today’s article, we are going to look at passing arrays to functions in […]
[…] 2D arrays in C with Example programs […]
[…] 2D-Arrays in C – How to Declare, Access, and Modify 2D arrays […]
[…] 2D Arrays in C Language – How to Create and Use 2D Arrays or Matrices in C […]
[…] 2D arrays in C with Example programs […]
[…] Multi-Dimensional Arrays in C […]
[…] Multi-Dimensional Arrays in C […]
[…] Multi-dimensional Arrays in C […]
[…] Multi-Dimensional Arrays in C […]
[…] Multi-dimensional Arrays in C […]
[…] Multi-Dimensional Arrays in C […]
[…] have looked at the Arrays and multi-dimensional arrays in earlier articles. In today’s article, We will look at the Strings in C programming language. […]