break statement in C Language with Example programs
- Introduction:
- Break statement in C language:
- Syntax of break statement:
- Break Statement Flow Diagram:
- Program to demonstrate break statement in C language:
- Program Explanation: Find a number in an array program Algorithm:
- Break statement with the while loop in C:
- Break Statement with for loop:
- Do while loop with break statement in C:
- Break Statement with Switch Statement:
- Break Statement with the Nested Loops in C language:
- Break Statement with the Nested switch cases:
- Conclusion:
- Loops and break statement practice programs:
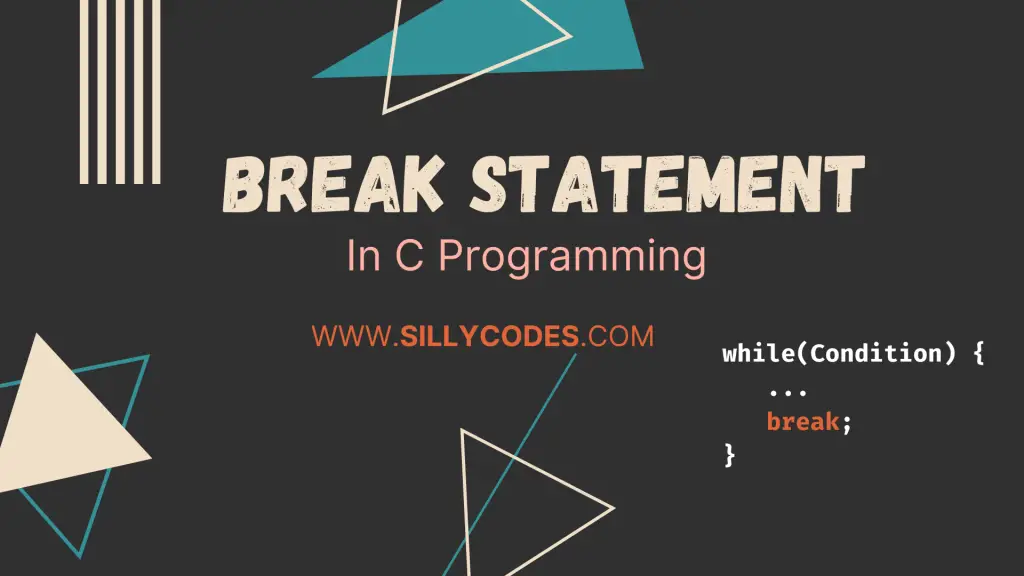
Introduction:
We have looked at the while loop, for loop, and do while loop in our earlier articles, In today’s article we will learn about the break statement in c language and how and where we can use the break statement. So let’s get started.
Break statement in C language:
The break statement is used to terminate a loop or switch statement. You can terminate a loop even before the loop condition become False.
As we discussed earlier in our loops tutorials, The loops are used to iterate over a block of code until the loop condition becomes False. However, there are situations when we wish to end the loop even before the loop condition is false. The break statement can be used in these situations to end the loop.
📢 We can use the break statement to terminate the Loops ( any loop – while, for, and do-while) and switch statements in the C programming language
Syntax of break statement:
1 |
break; |
Break Statement Example Usage:
1 2 3 4 5 |
while(loopCondition) { // Statements break; // Statements } |
Break Statement Flow Diagram:
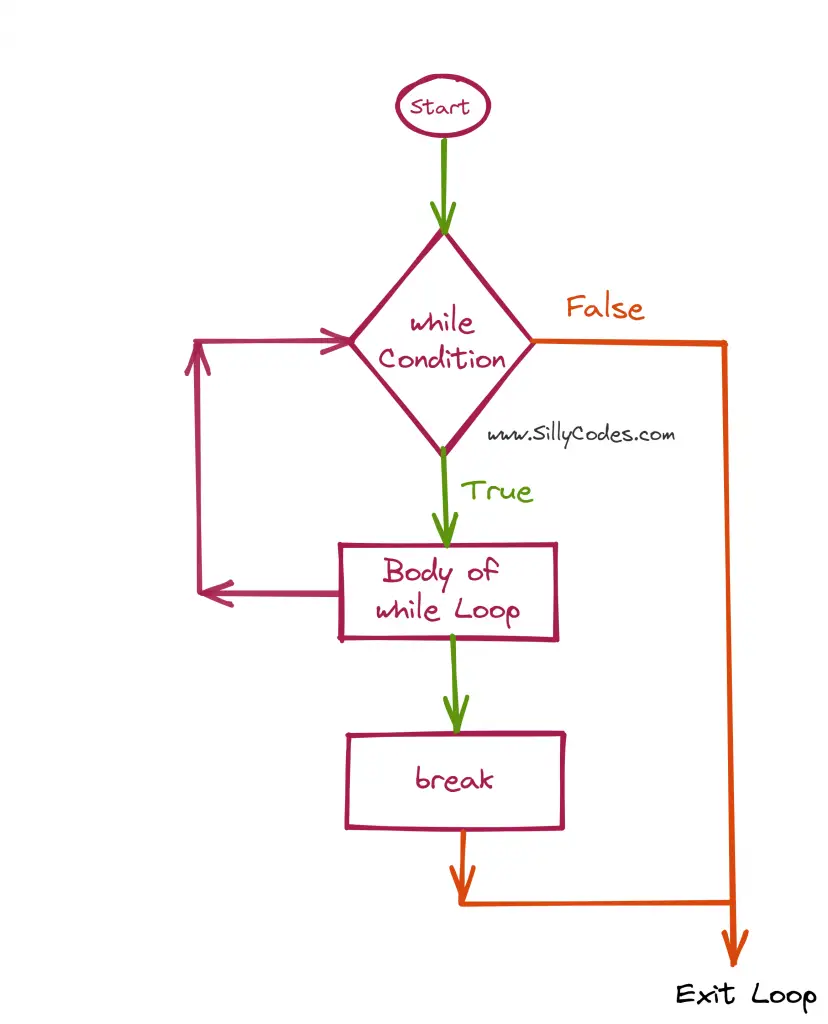
Use the break keyword inside the body of the loop or the body of the switch statement.
The break statement will terminate the loop or switch and control will come out of the loop or switch.
📢 We can also use the break statement inside the Nested Loops and the Nested switch statements. If we use break statement with Nested loops,etc then only the inner-most loop will terminate.
Program to demonstrate break statement in C language:
Let’s write a C program to search for a number in a given array of numbers. Once we found the number, we are going to break out of the loop. As we no longer need to check the remaining elements in the array.
We are going to use the Linear search for simplicity.
📢 The C-Array’s are used to store multiple values of a similar datatype. We are going to discuss the array’s in upcoming tutorials.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/* Program: Find a Number in given Array */ #include<stdio.h> int main() { int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int i = 0; int num, arrSize; // Take the input from the user printf("Enter a Number to search: "); scanf("%d", &num); // Calculate the size of the array - 'arr' arrSize = sizeof(arr)/sizeof(arr[0]); // Traverse over the array and search for the element 'num' in 'arr'. for(i=0; i<arrSize; i++) { // Check for the 'num' and if any element match the 'num' // Print the number as found and // 'break' out of the loop as we no longer need to iterate. if(num == arr[i]) { printf("Number %d is found in the array \n", num); break; } } // Check if the 'i' value is equal to 'arrSize', // Which means we reached end and not found the 'num' in 'arr' // Display the 'Not found' message. if(i == arrSize) { printf("Number %d is not found \n", num); } return 0; } |
Save the above program as findNumber.c and compile and run the program using your IDE.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc findNumber.c $ ./a.out Enter a Number to search: 5 Number 5 is found in the array $ ./a.out Enter a Number to search: 10 Number 10 is found in the array $ ./a.out Enter a Number to search: 11 Number 11 is not found $ ./a.out Enter a Number to search: 3 Number 3 is found in the array $ ./a.out Enter a Number to search: 31 Number 31 is not found $ |
Program Explanation: Find a number in an array program Algorithm:
- We have used an array ( arr) to store the 10 values from 1 to 10.
- And asked the user for a number ( num) to search.
- Then we calculated the size of the array using the sizeof(arr)/sizeof(arr[0]); and stored the result in arrSize variable.
- Once we got the above details, We Iterated over the array using the for loop.
- At Each Iteration, we check if the array element at the index
i and the
num are equal.
- If it is equal, Then we found the number. So we are displaying the Number found message and immediately we are using the break statement to terminate the loop. As we found the num, We no need to look at the other elements in the array.
- If it is not Equal, Then we go to the next Iteration.
- Until we find a match or get to the end of the array, the above step will be repeated.
- Once we come out of the loop, We are checking if the i is equal to arrSize, If we don’t find a match, Then we will iterate over all elements of the array, So the i value will reach the length of the array, which is arrSize. So if we don’t find the match, Display the Not Found message.
One thing to note here is, If we find the number( num) then we are not iterating over the remaining elements in the array. The break statement is being used to end the loop.
In the worst case, if the provided number happens to be the last number in the array, Then we will need to traverse over every element of the array.
Now Let’s look at how we can use the break statement with different loops and switch case.
- Break statement with a while loop
- Break statement with for loop
- Break statement with do while loop
- Break statement with a switch statement
Break statement with the while loop in C:
We can specify the break statement to exit out of the while loop. Here is an example program.
Program: while loop with break statement example:
The while loop is going to iterate from 1 to 10. and we are going to terminate the loop once we reached number 3 using the break statement.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/* Program: while loop with break statement example */ #include<stdio.h> int main() { int i = 0; while(i<=10) { if (i == 3) { printf("The value of i is reached %d, Stopping the loop \n", i); break; } // Increment the loop control variable 'i' i++; } printf("Out of while loop \n"); return 0; } |
Program Output:
1 2 3 4 5 |
$ gcc while-break.c $ ./a.out The value of i is reached 3, Stopping the loop Out of while loop $ |
Break Statement with for loop:
The above number search program used the for loop to iterate over the array elements and once we found the number, terminated the loop using break.
Let’s look at another example, We are going to have an Infinite for loop and stop the loop when the count value reaches 10.
Learn more about the Infinite loops in the following article
Program to understand for loop with break statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
/* Program: for loop with break statement example */ #include<stdio.h> int main() { int counter = 0; // Create a Infinite for loop for(; ; counter++) { // check if the variable 'counter' reached '10'. // if it is equal to '10'. Stop the loop. if (counter == 10) { printf("Counter value reached %d, Stopping the loop using break statement \n", counter); break; } } printf("Out of for loop \n"); return 0; } |
Program Output:
1 2 3 4 5 |
$ gcc for-break.c $ ./a.out Counter value reached 10, Stopping the loop using break statement Out of for loop $ |
As you can see we have an Infinite for loop, which has a counter variable, The counter variable starts from 0 and for each iteration increments the counter by 1. We want to stop the loop once the counter reached the value of 10.
So we are checking the value of the counter with 10 at each iteration and at the tenth Iteration, the value of the counter became equal to the 10. So we stopped the loop using the break statement.
Do while loop with break statement in C:
Similarly, we can terminate the do-while loop using the break statement.
Here is an example.
Program to understand do while loop with break:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/* Program: do while loop with break statement example */ #include<stdio.h> int main() { int cnt = 0; // Create a Infinite do while loop do { // Terminate the loop once the value of the `cnt` becomes `7` if (cnt == 7) { printf("The value of cnt reached %d, Terminate the loop \n", cnt); break; } cnt++; } while(1); printf("Out of do while loop \n"); return 0; } |
Program Output:
1 2 3 4 5 |
$ gcc while-break.c $ ./a.out The value of cnt reached 7, Terminate the loop Out of do while loop $ |
Break Statement with Switch Statement:
We have looked at the Switch Statement in our previous article. The switch is a mutli-way decision-making statement. The switch statement has the cases. If the given switch condition matches any of the cases, the respective code block will be executed. To come out of the case, We use the break statement.
📢 The break statement is one of the essential parts of the switch statement, As it helps to come out of the switch case after executing the desired case. Forgetting to include the break statement after the case can lead to the execution of subsequent cases. So make sure to add the break statement whenever it is needed.
Match Numbers using the switch statement and break statement in C language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/* Program: Switch Statement or Switch case with break statement in C Language */ #include<stdio.h> int main() { int num; printf("Enter a Number (1-5): "); scanf("%d", &num); // Check the given 'num' againes the cases. switch(num) { // If the case matches the given number 'num', // Then we print the message and 'break' out of the switch statement. case 1: printf("You Entered 1 \n"); // using 'break' to terminate the switch statement. // if you forgot to specify the 'break', Then subsequent cases will execute. break; case 2: printf("You Entered 2 \n"); break; case 3: printf("You Entered 3 \n"); break; case 4: printf("You Entered 4 \n"); break; case 5: printf("You Entered 5 \n"); break; default: printf("Invalid Number \n"); break; } printf("Outside of the switch statement \n"); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc while-break.c $ ./a.out Enter a Number (1-5): 1 You Entered 1 Outside of the switch statement $ ./a.out Enter a Number (1-5): 5 You Entered 5 Outside of the switch statement $ ./a.out Enter a Number (1-5): 10 Invalid Number Outside of the switch statement $ ./a.out Enter a Number (1-5): 8 Invalid Number Outside of the switch statement $ ./a.out Enter a Number (1-5): 3 You Entered 3 Outside of the switch statement $ |
Break Statement with the Nested Loops in C language:
We can use the break statement in the Nested loops. When we use the break in the Nested loop, Only the inner-most loop will Terminate. and control comes out to the next loop.
Note that, Using the break statement in the Nested loop won’t terminate all Loops. It will only terminate the inner-most loop where the break is used.
Let’s look at an example program.
Example Program to understand break with Nested loops:
We are going to create a Nested loop. The Outer loop will iterate from 1 to 5 and at each Iteration The Inner loop will iterate from 1 to 3 ( Actually loop will go up to 5, but we are using break statement to terminate the loop once the value of b reaches 3)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/* Nested loops with break statement */ #include<stdio.h> int main() { // Initialize the two variables with 'one's int a, b; // Create a Nested loop. for(a=1; a<=5; a++) { // Outer loop start printf("OuterLoop - a : %d \n", a); // Create Inner loop for(b=1; b<=5; b++) { // Inner loop start printf("InnerLoop - b : %d \n", b); // Break when 'b' reaches 3 // This 'break' only terminates the Inner loop. // Outer loop will continue. if(b == 3) { printf("Terminating Innerloop\n"); break; } } // Inner loop close } // Outer loop close return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
$ gcc nested-break.c $ ./a.out OuterLoop - a : 1 InnerLoop - b : 1 InnerLoop - b : 2 InnerLoop - b : 3 Terminating Innerloop OuterLoop - a : 2 InnerLoop - b : 1 InnerLoop - b : 2 InnerLoop - b : 3 Terminating Innerloop OuterLoop - a : 3 InnerLoop - b : 1 InnerLoop - b : 2 InnerLoop - b : 3 Terminating Innerloop OuterLoop - a : 4 InnerLoop - b : 1 InnerLoop - b : 2 InnerLoop - b : 3 Terminating Innerloop OuterLoop - a : 5 InnerLoop - b : 1 InnerLoop - b : 2 InnerLoop - b : 3 Terminating Innerloop $ |
As you can see from the above program for each iteration of the outer loop, The inner loop is iterating from 1 to 3. And Once the b reaches 3, We are terminating the Inner loop.
Break Statement with the Nested switch cases:
Similar to the nested loops, If we use the break statement in Nested switch statements, The innermost switch statement will be terminated. And control comes out to the next switch statement.
Program to understand the break statement with switch case:
Here is a Restaurant order program. Here we used two switch statements (Nested switch case).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
/* Switch Statement Example program */ #include <stdio.h> int main(void) { // Take two values from the user int number; int type; printf("Press 1 for Non-Veg, 2 for Veg: "); scanf("%d",&number); switch(number) { case 1: // User selected Non-Veg // Ask for the customizations printf("Press 1 for Chicken, 2 for Mutton, and 3 for Fish : "); scanf("%d", &type); switch(type){ case 1: printf("Your order Non-Veg - Chicken received \n"); break; case 2: printf("Your order Non-Veg - Mutton received \n"); break; case 3: printf("Your order Non-Veg - Fish received \n"); break; default: printf("Invalid Option \n"); break; } break; case 2: // User selected Veg // Ask for customizations printf("Press 1 Mashroom, 2 for Panner, and 3 for Salad : "); scanf("%d", &type); switch(type){ case 1: printf("Your order Veg - Mashroom received \n"); break; case 2: printf("Your order Veg - Panner received \n"); break; case 3: printf("Your order Veg - Salad received \n"); break; default: printf("Invalid Option \n"); break; } break; default: printf("Invalid Option - Please try again \n"); break; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc nested-switch-statement.c -o nested-switch $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 2 Your order Non-Veg - Mutton received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 2 Press 1 Mashroom, 2 for Panner, and 3 for Salad : 1 Your order Veg - Mashroom received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 2 Your order Non-Veg - Mutton received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 3 Your order Non-Veg - Fish received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 2 Press 1 Mashroom, 2 for Panner, and 3 for Salad : 3 Your order Veg - Salad received $ |
As we have discussed earlier, The break statement won’t terminate all switch statements. It will only stop the switch statement where break is specified.
Conclusion:
We have looked at the break statement in C language, and we also learned where we can use break statement and how it will affect the flow of the program. Then we saw how we can use break statement with different loops and the switch statement with example programs. Finally, We looked at break with Nested loops and nested switch statements.
If you reached it so far, Then you would also enjoy our other tutorials, Here is the Index of the tutorials.
5 Responses
[…] Break Statement in C with Example Programs […]
[…] have looked at the loops and break Statement in earlier posts. In today’s article, We are going to discuss about the Continue Statement in C […]
[…] Break Statement in C with Example Programs […]
[…] the max is evenly divisible without any remainder, Then max is our LCM. Print it and break the […]
[…] Check if the numbers[i] == numbers[j]. If this condition is true, Then we found a Duplicate, So break the inner […]