Array of Pointers in C Language with Example Programs
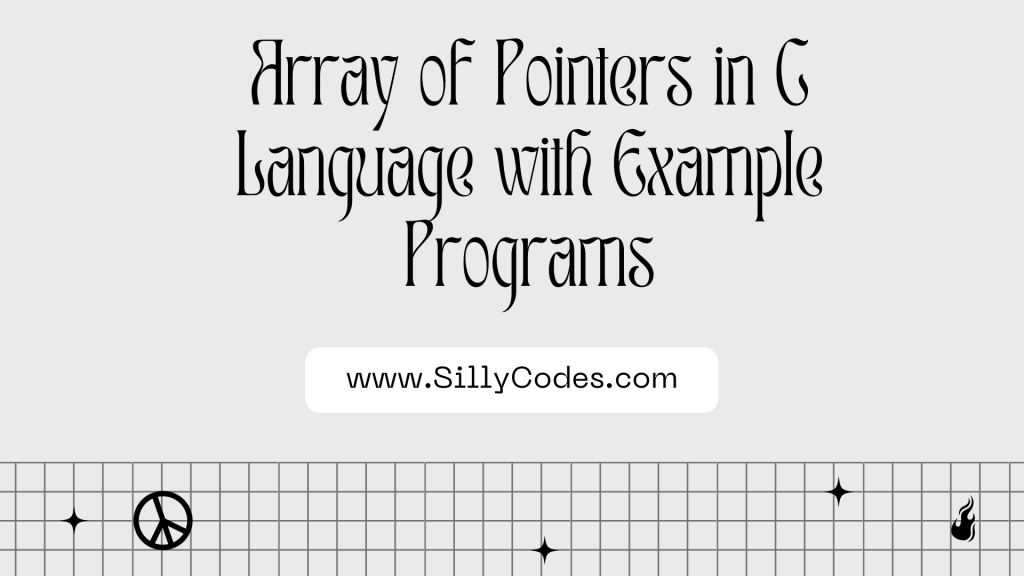
Program Description:
We have looked at the Pointer to an Array in earlier articles. In today’s article, We will look at the Array of Pointers in C programming language with example programs.
📢Recommended Reading:
An Array of Pointers in C Programming:
As the name suggests, An array of pointers is an Array where every element of the array is a pointer variable.
Unlike the normal array, All elements of the array of pointers are pointer variables, which are capable to store the address of another variable.
Here is the syntax to create the array of pointers in C.
Declaring An Array of Pointers in C:
We can declare an array of pointers by specifying the datatype name followed by the asterisk symbol( *) like below.
data_type *arrOfPointers[SIZE];
Here
- data_type is the datatype of the pointer variables.
- * denotes we are creating pointer variables.
- arrOfPointers is the name of the array of pointers variables.
- SIZE is the number of elements in the array(i.e Size of an array).
Let’s look at an example.
Example of An Array of Pointer in C:
int *arrPtr[100];
We created an array called arrPtr with size of 100 elements, Where each element is an integer pointer.
Let’s look at an example program to understand the Array of Pointers in C programming language
Program to Understand An Array Of Pointers in C Language:
In the following program, We have created an array of pointers called arrOfPtr with the size 5. So all five elements of the arrOfPtr is an integer pointer.
We also created five variables i, j, k, l, and m and initiated with values 44, 63, 1, 77, and 11 respectively.
1 |
int i = 44, j = 63, k = 1, l = 77, m = 11; |
Then we stored the address of the above five variables in the arrOfPtr array’s five elements. ( As all elements of the array are pointers).
Finally, We iterated over the array of pointers using a for loop and accessed the values of the above variables through the array of pointer variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
/*     Program to understand Array of Pointers in C Language     sillycodes.com */  #include <stdio.h>  int main() {      // Initialize 5 integers     int i = 44, j = 63, k = 1, l = 77, m = 11;      // create an array of pointers     int *arrOfPtr[5];      // store the address of variables     arrOfPtr[0] = &i;     arrOfPtr[1] = &j;     arrOfPtr[2] = &k;     arrOfPtr[3] = &l;     arrOfPtr[4] = &m;      printf("Get details of Variables using Array of Pointers: \n");      // Iterate over the Array of Pointers     for(int i=0; i<5; i++)     {         // Value of pointer         printf("Value of Pointer arrOfPtr[%d]:%p \n", i, arrOfPtr[i]);          // Value of variable - Dereference pointer         printf("Value of Variable through pointer (*arrOfPtr[%d]):%d\n", i, *arrOfPtr[i]);     }      return 0; } |
We used the *arrOfPtr[i](Dereference Operator) to get the value of the variable stored at the ith index of the arrOfPtr array.
Program Output:
Let’s compile and run the program.
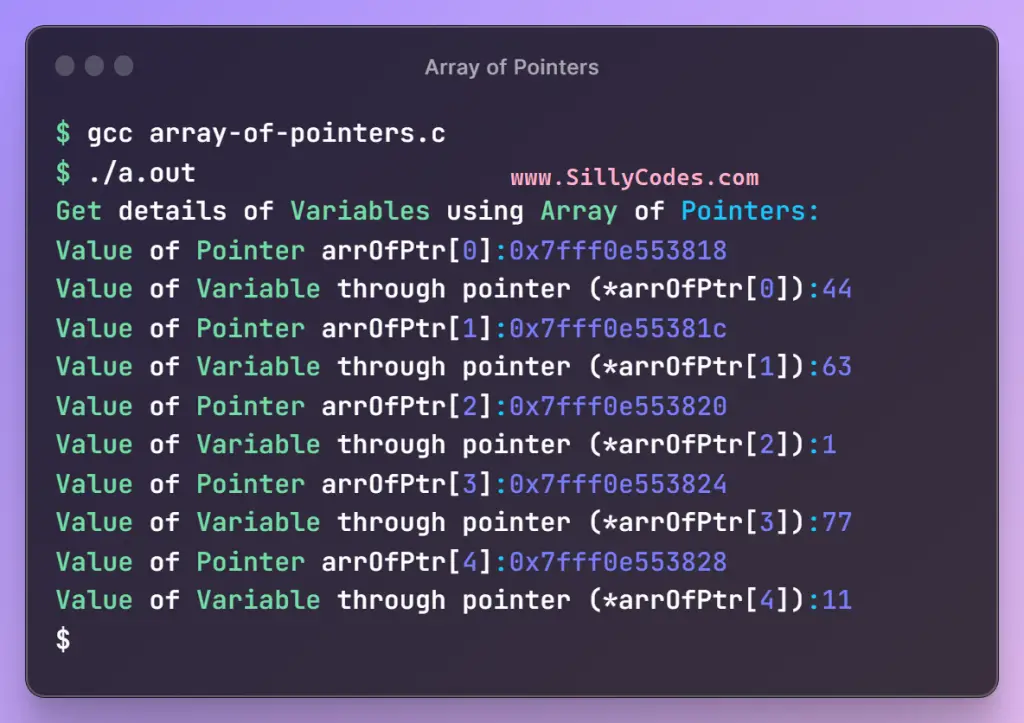
As we can see from the above output, We were able to store the address of the integer variables, and when we dereferenced the elements of the arrOfPtr array we got the integer variables values.
So the array of pointers arrOfPtr is able to store addresses of other variables.
An Array of Pointers with 2D Array in C:
We can use an Array of pointers to access and traverse the 2-dimensional array easily in c programming language.
Each element of the Array of Pointer variable is a pointer variable and the Two-dimensional array is a collection of one-dimensional arrays.
The two-dimensional array elements are stored in the contiguous memory. We can represent a 2-D array as the Matrix.
📢 Learn More about the 2-D Array at – 2D-Array in C – How to Create and Modify Two-dimensional array in C.
How to Store the 2D Array using Array of Pointer Variables:
So we can use an Array of Pointer variables to store the address of a 2D Array. Where each element of the array of pointers will store the address of each row of the 2D-Array.
For example, If you have a 3X3 two-dimensional array( inputArr) i.e Matrix and an Array of three-pointer variables ( arrOfPtr).
int inputArr[3][3];
int *arrOfPtr[3];
Then,
The first element of the array of pointer variables will store the 0th element of the starting row (0th Row – Index starts from zero) of the 2D-Array.
arrOfPtr[0] will store the inputArr[0]
Similarly, 1st index pointer in the array of pointers stores the 1st index row of the matrix.
arrOfPtr[1] will store the inputArr[1]
and
arrOfPtr[2] will store the inputArr[2]
This way we can store the 2D-Array or Matrix with an Array of Pointers variable.
How to Get 2D-Array Elements using the Array of Pointer variable:
We can access the element so the 2D-Array through the array of pointer variables by dereferencing the array of pointer variables arrOfPtr Twice.
For Example to get the value at the row index i and column index j of the 2D Array, We can dereference twice the array of pointer variables like below.
arrOfPtr[i][j]
Let’s plug everything together and create a program that demonstrates how to store the 2D array using an array of pointers and how to get the element values of the 2D Array using the array of pointer variables.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/*     Program to Access 2D Array elements using an Array of Pointers in C Language     sillycodes.com */  #include <stdio.h>  // Size of Array #define ROWS 3 #define COLUMNS 3  int main() {      // declare two variables     int i, j;      // create a 2D Array     int inputArr[ROWS][COLUMNS] = {                                     {10, 20, 30},                                     {40, 50, 60},                                     {70, 80, 90}                                   };      // create an array of pointers     int *arrOfPtr[ROWS];      // store the address of variables     for(i=0; i<ROWS; i++)     {         // store address of each 1D array to pointer         arrOfPtr[i] = inputArr[i];     }      printf("Access elements of Array(inputArr) using Array of Pointers(arrOfPtr): \n");      // Iterate over the 2D Array and print values     for(i=0; i<ROWS; i++)     {         // Value of pointer         // printf("Value of Pointer arrOfPtr[%d]:%p \n", i, arrOfPtr[i]);                 for(j=0; j<COLUMNS; j++)         {             // Display value of 2D-Array using array of pointers             printf("inputArr[%d][%d] : %d\n", i, j, arrOfPtr[i][j]);                     }     }      return 0; } |
Program Output:
Compile the program.
$ gcc array-of-pointers-2d-array.c
The above command generates the executable file named a.out. Run the executable file.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Access elements of Array(inputArr) using Array of Pointers(arrOfPtr): inputArr[0][0] : 10 inputArr[0][1] : 20 inputArr[0][2] : 30 inputArr[1][0] : 40 inputArr[1][1] : 50 inputArr[1][2] : 60 inputArr[2][0] : 70 inputArr[2][1] : 80 inputArr[2][2] : 90 $ |
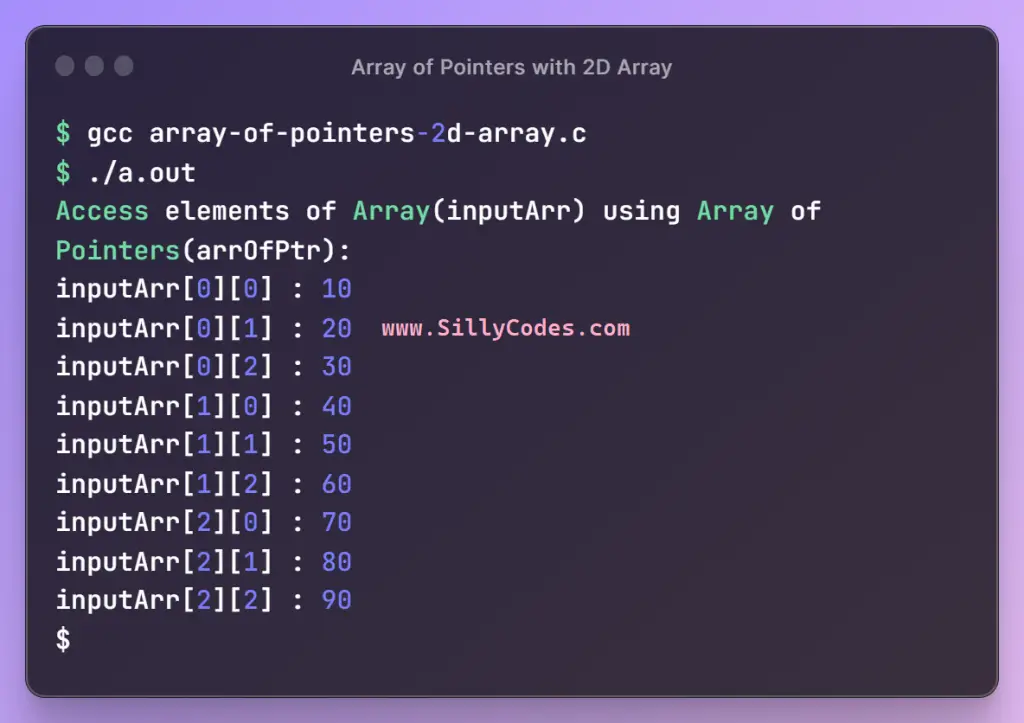
As we can see from the above output, We are able to store the 2D-array using an array of pointer variables and We are also able to dereference twice and get the values of the 2D-Array using the Array of pointer variables.
Related Pointers Tutorials and Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Size of Pointer in C Language
- Add Two Numbers using Pointers in C Language
- Program to perform Arithmetic Operations using Pointers in C
- Swap Two Numbers using Pointers in C Language
- Pointer Arithmetic in C Language
- Pointer to Pointer in C – Double Pointer in C
- Pointers and Arrays in C Language with Example Programs
- Accessing Array Elements using Pointers in C
- Print String Elements using Pointers in C Language
- Function Returning Pointer in C Programming Language
- Function Pointers in C with Example Programs
1 Response
[…] Array of Pointers in C Language with Example Programs […]