Strings in C Language – Create, Access, and Modify Strings
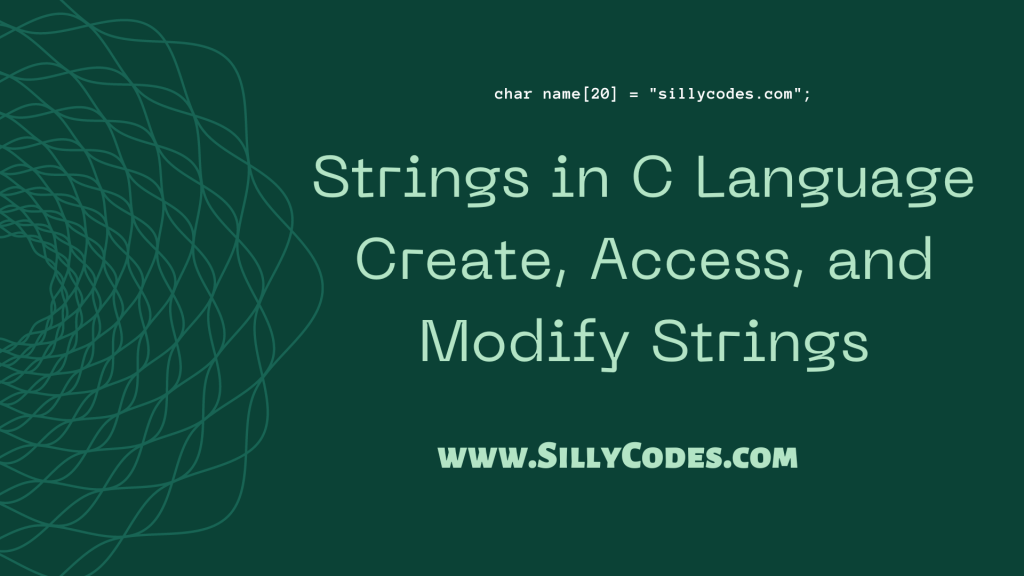
- Strings in C Language:
- Declaring Strings in C Language:
- Initialization of Strings in C:
- Accessing the String Elements in C:
- Program to Read a string from user and print string on console:
- Reading a Line (string with spaces) using the gets() function:
- Passing String to Functions:
- Example Program: How to Pass Strings to Functions in C Language:
- String Functions:
Introduction:
We have looked at the Arrays and multi-dimensional arrays in earlier articles. In today’s article, We will look at the Strings in C programming language. How to Create, access, and modify the strings in C.
Strings in C Language:
C Language doesn’t have any special datatype to represent the strings. Strings in C programming language are one-dimensional character arrays. All strings in the C language will terminate with the NULL ( \0) character.
We can create the strings in C by using the double quotes ( "). The strings are a sequence of characters that are enclosed within the double quotes ( ")
Here are a few examples of the Strings in C language:
"Welcome to SillyCodes.com"
"Leo Messi"
"Programming is Fun"
we can’t create strings using single quotes( '). The single quotes are used to represent the characters in C language. So 'v' is a single character ( Not a String ).
📢 Strings in C programming must end with the NULL ( \0) Character
As the strings are a character array, each character size is one byte, So the size of the string is equal to the number of characters in the string plus the NULL character.
The characters of the strings are stored in the continuous memory address.
Here is the graphical representation of the C Strings.
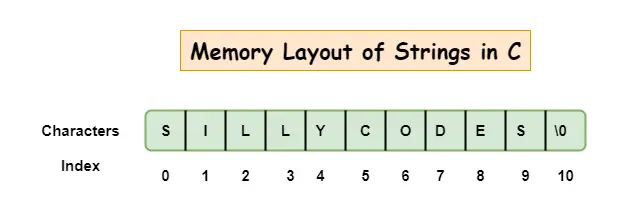
As you can see from the above memory layout diagram, Each character in the array is of one byte and all characters of the string are stored in continuous memory.
The NULL Character( \0) is a special character and its ASCII Value is equal to Zero(0).
Declaring Strings in C Language:
As specified earlier, The strings are character arrays. To create strings use the character arrays.
The Syntax for declaring the strings in c:
1 |
char string_name[string_size]; |
Here the string_name is the name of the string and string_size is the maximum number of characters string can hold including the NULL character. Let’s look at an example.
String Declaration Example:
1 |
char name[20]; |
In the above example, We have created a String called name with the size of 20 characters. So the name string can store a name with a max size of 19 letters (As the string needs to be terminated with the NULLcharacter)
Initialization of Strings in C:
Similar to the C Arrays and variables, We can initialize the String with the desired values.
We can initialize the strings in different ways, Let’s look at them
String initialization using the character Array:
1 2 3 4 5 |
// Example 1: char message[10] = {'W', 'e', 'l', 'c', 'o', 'm', 'e','\0'}; //Example 2: char message[] = {'W', 'e', 'l', 'c', 'o', 'm', 'e','\0'}; |
We can initialize the string using a character array, But we must need to end the character array with the NULL character. If we are initializing the array, Then specifying the string size is optional. (similar to Array initialization)
String Initialization using the String Literal:
We can use the string literals to initialize the string like below.
1 2 3 4 5 |
// Method 1: Specifiying size is optional char name[] = "Ross"; // Method 2: char name[8] = "Ross"; |
If we use the string Literals to initialize the string, Then the compiler automatically places the NULL character ( \0) at the end of the string.
So The above string has 5 characters R, o, s, s, \0.
Make sure to specify the string size as per your requirement properly.
📢If we initialize the string, Then the sizeis optional.
Accessing the String Elements in C:
As strings are character arrays, We can access the string elements (Characters) using the subscript
[ ] operation. Have a look at the following program.
1 2 3 4 5 6 |
// Initialize string char message[10] = "Welcome"; // Accessing String Elements char ch = message[0]; // the variable ch contains the character 'W' |
In the above example, We have initialized a string named message with the Welcome string literal.
Then to access the first element of the string, We used the subscript operator with the element index. which is message[0], and stored the result in the ch variable.
📢Similar to the Arrays, Strings are Also Zero Indexed. So the first element of the string will be stored at the index
Read and Print Strings in the C Programming:
Let’s look at how to read and print the strings in C programming language.
Reading the strings from the user:
We can use the scanf function with the %s format specifier to read the string values from the user.
The scanf() function reads the string up to the whitespace character. The whitespace character can be any character like Space ( ' '), Tab ( '\t'), New Line ( '\n'), and carriage return ( '\r'), etc.
📢Learn more about the white spaces at the Character Set of C Tutorial
Here is an example to read a string from the user using the scanf() function
1 |
scanf("%s", message); |
Printing the strings on the Console:
We use the printf function with the %s format specifier to print the string in the c programming language.
1 |
printf("Given Message is : %s \n", message); |
We can also use the puts() function to print the strings on the console.
1 |
puts(message); // prints string 'message' on console. |
Program to Read a string from user and print string on console:
Here’s an example program that uses the scanf() and printf() functions to read the user’s input string and print it back to the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/* Program to Read and Print String in C SillyCodes.com */ #include <stdio.h> int main() { // declare a string char message[100]; // Read the string from the user printf("Please enter a string : "); scanf("%s", message); // Print the string on the console printf("Given String is : %s \n", message); return 0; } |
Program Output:
Compile and run the program
1 2 3 4 5 |
$ gcc read-print-string.c $ ./a.out Please enter a string : Welcome Given String is : Welcome $ |
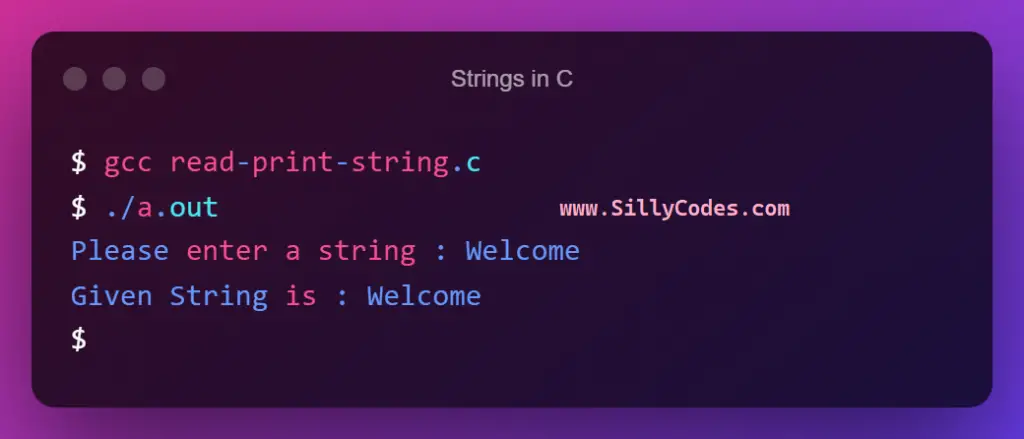
In the above program output, The user provided the input string as Welcome, We used the scanf function to read the Welcome string literal and stored it in the variable called message
scanf("%s", message");
Then We used the printf() function displays the message on the console like below.
printf("Given String is : %s \n", message);
As specified earlier, The scanf() function can only read the strings up to a Whitespace character, So if your string contains any spaces or tables, Then only the string up to the space or tab will be read by the scanf function.
Let’s look at this example
1 2 3 4 |
$ ./a.out Please enter a string : Welcome to Sillycodes.com Given String is : Welcome $ |
In the above output, The scanf function only read the string Welcome, and skipped the input string “ to Sillycodes.com”. This is happened due to the space character.
In order to read the complete line with spaces, We need to use the gets() function.
Reading a Line (string with spaces) using the gets() function:
The gets() function is helpful to read a line from the standard input. The reading stops if a new line or EOF is encountered.
📢The gets() function can read multiple strings. The space won’t stop the gets reading, Only the Newline or EOF stops.
Here is a program to read a line from the user ( string with spaces)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* Program to Read and Print String in C SillyCodes.com */ #include <stdio.h> int main() { // declare a string char message[100]; // Read the string from the user printf("Please enter a string : "); // scanf("%s", message); gets(message); // Print the string on the console printf("Given String is : %s \n", message); return 0; } |
Program Output:
1 2 3 4 5 |
$ gcc read-print-string.c $ ./a.out Please enter a string : Welcome to SillyCodes.com Given String is : Welcome to SillyCodes.com $ |
Now we are able to read the complete line from the user. We have looked at the gets function in detail in the Standard Input and Standard Output Functions in C Program.
📢The getsfunction won’t do any Boundary-checks for the provided buffer. So it is advised to use it with caution or you can use functions like fgetsand getlinefunctions to read the string from the user.
Using fgets() function to read the strings with white spaces.
1 2 |
// read input from the stdin fgets(message, sizeof(message), stdin); |
Passing String to Functions:
We can pass the string to a function as an Array of characters or as a pointer to characters.
Here is a program to pass the string to function as an Array of characters
📢Related: Functions in C Language
Example Program: How to Pass Strings to Functions in C Language:
The following program will prompt the user to provide the name and calls a function called greetUser with the name as the parameter. ( Passing string name to function greetUser)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/* Program to Read and Print String in C SillyCodes.com */ #include <stdio.h> /** * @brief Greet the user with 'name' * * @param name - 'name' of the user */ void greetUser(char name[]) { // Print the string on the console printf("Hi %s, Welcome to SillyCodes.com\n", name); } int main() { // declare a string char name[100]; // Read the string from the user printf("Enter your name : "); scanf("%s", name); //fgets(name, sizeof(name), stdin); // call the 'greetUser' pass 'name' string greetUser(name); return 0; } |
The greetUser() function takes a character array ( char name[]) as a formal argument. Then it will display a welcome message on the console.
Program Output:
Let’s compile and run the program and observe the output.
1 2 3 4 5 |
$ gcc string-func.c $ ./a.out Enter your name : Joey Hi Joey, Welcome to SillyCodes.com $ |
As you can see from the above output, the user entered the nameas the Joey and the main() function called the greetUser(name) function with the name, and greetUser() function received the name as the character array and printed the welcome message on the screen.
String Functions:
The C Programming language comes with a few string manipulation and utility functions. Here are a few string functions in the C.
Function Name | Description |
---|---|
strlen() | To calculate the length of the string |
strcpy() | To copy a string to another string |
strcmp() | To compare two strings |
strcat() | To concatenate two string |
strchr() | To search for the first occurrence of a character in the given string |
strstr() | To search for the first occurrence of a string in the given string |
We are going to look at the above string functions in detail in the upcoming articles.
Conclusion:
We have looked at the Strings in C programming language and how to declare, Initialize and modify strings with example programs. We also learned about how to read and print the strings in C programming and finally, we looked at how to pass strings to the functions.
19 Responses
[…] our previous article, We looked at the strings in the C Language and how to read and print the string in C. In today’s article, We are going to look at the strlen […]
[…] have looked at the introduction to strings in C and strlen library function in earlier articles, In today’s article, We will look at the strcpy […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] a Program to Copy String in C programming language. The program should accept a string (character array) from the user and copy it to new […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] Strings in C Language – Declare, Initialize, and Modify Strings […]
[…] is recommended to know the C-Strings, C-Arrays, and Functions in C to better understand the following […]
[…] is recommended to know the basics of the C-Strings, C-Arrays, and Functions in C to better understand the following […]
[…] have looked at the Strings in C language in earlier articles, In today’s article, We will learn about the Pointers in C programming. We […]
[…] is recommended to know the basics of the C-Strings, C-Arrays, and Functions in C to better understand the following […]
[…] Strings in C language – How to Create and use strings in C […]
[…] C Strings – How to Create and Use Strings in C Programming Language […]
[…] far we have used static memory allocation to create variables, arrays, strings, […]
[…] have looked at the Strings and Pointers in earlier articles. In today’s article, We will look at the dynamic memory […]