Bitwise XOR Operator in C Language
- Introduction:
- Bitwise XOR Operator in C Language:
- Truth Table of Bitwise XOR Operator:
- How Bitwise XOR ( ‘^’ ) Operator Works?
- Example Program 1: Bitwise XOR Operator in C Language:
- Let’s Take another Example:
- Bitwise XOR Example 2:
- Invert bit using bitwise XOR Operator:
- Bitiwse XOR Operator to Swap the Numbers without extra space:
- Swap two number using XOR operator Example Walkthrough:
- Program : Swap two numbers without using third variable ( Bitwise XOR ):
- Program Output:
- Related Articles:
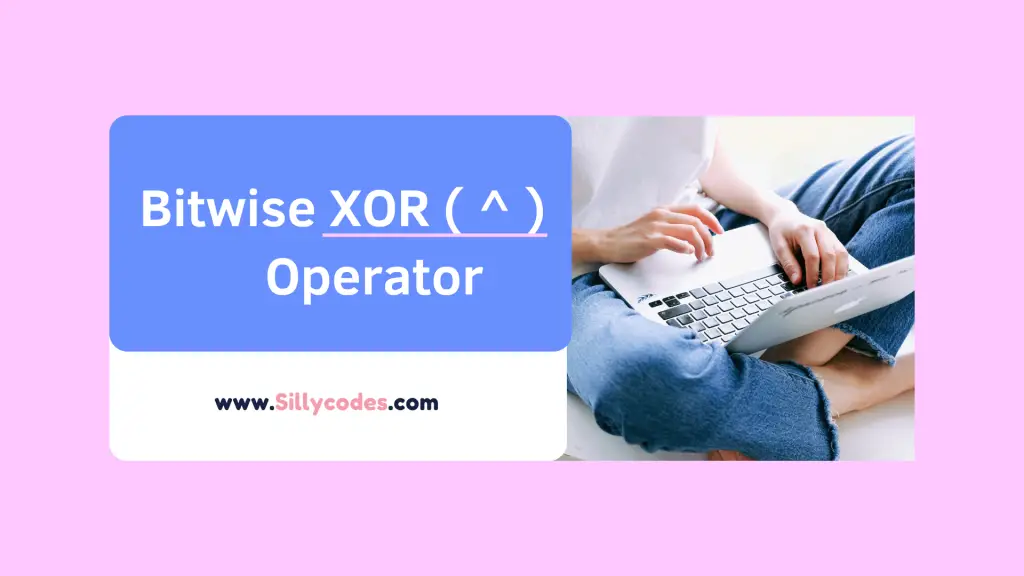
Introduction:
In the previous articles, We have discussed what are Bitwise Operators and Bitwise AND Operator, and Bitwise OR operators. In today’s article, we will learn about the Bitwise XOR operator in C Language.
📢 This Article is part of the Bitwise Operators Series
Bitwise XOR Operator in C Language:
The Bitwise XOR operator is Binary Operator and Takes two input values ( Binary sequences of two values ) and performs the Bitwise OR on each pair of bits in the given binary sequence.
In C Programming, The Bitwise XOR operator is denoted by the Carot ^symbol.
The result of the Bitwise XOR operation is False(0) only if both of the input bits are the same, i.e if both inputs are True(1) then the XOR output is False(0), Similarly, if both input bits are False(0) then XOR output is False(0)
Bitwise XOR Operator returns True(1), When both input bits are different.
So if your input is True(1) and False(0), Then XOR will output True(1) and vice-versa.
We can’t apply bitwise XOR to the Floating point data.
Truth Table of Bitwise XOR Operator:
Input bit 1 ( Xi ) | Input bit 2 (Yi) | Result ( Xi | Yi ) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Here Xi and Yi are the Pair of bits in the Binary sequence of X and Y values.
How Bitwise XOR ( ‘^’ ) Operator Works?
Let’s go through a step by step example, We will take two numbers X=4 and Y=8.
Now we need to apply the Bitwise XOR operator on variables X and Y. i.e X ^ Y.
To perform the bitwise XOR, First of all, we need to convert the values of X and Y into the Binary form or Base-2 form.
➡️ For making calculations easy, We are going to take only 8 bits for the integer value. But note that the Integer value will be stored in 32 bits in the memory.
The Binary Equivalent of the X or 4 is 0000 0100.
And Binary Equivalent of the Y or 8 is 0000 1000.
- Here is a C Program to convert an Integer number to the Binary sequence – Decimal to Binary conversion program in C (sillycodes.com)
The Left-most bit in the binary representation is MSB or Most significant bit and the Right-most bit is the LSB or Least Significant bit.
Now we need to apply the Bitwise OR operation on each pair of bits.
Here Pair of bits means MSB in the first number X and MSB in the second number Y, So on until we reach the LSB of X and LSB of Y.
➡️ Bitwise X ^ Y is:
X –> 0000 0100 (4 in Binary)
Y –> 0000 1000 (8 in Binary)
X ^ Y –> 0000 1100 ( X ^ Y = 12 )
So the result of the bitwise XOR operation of X and Y ( 4 | 8 ) is 12.
Here is the graphical representation of How Bitwise XOR Operator works.
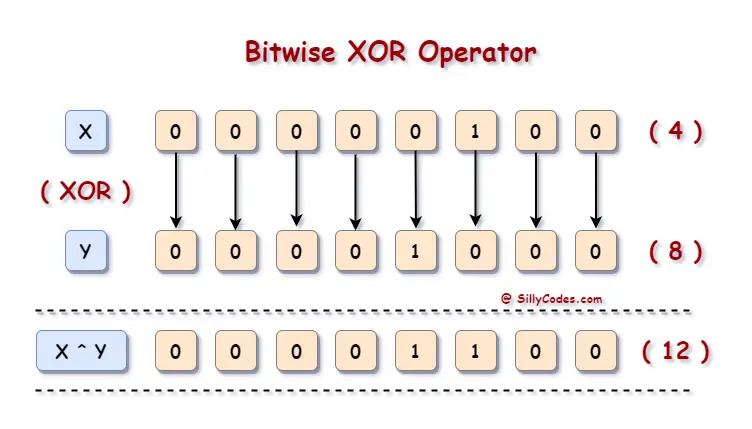
Let’s look at the program on the XOR operator.
Example Program 1: Bitwise XOR Operator in C Language:
In this program, we are going to perform the XOR operation on above inputs 4 and 8.
1 2 3 4 5 6 7 |
#include <stdio.h> int main() { // The bitwise XOR of '4' and '8' is 12 printf("The 4 ^ 8 is : %d \n", 4 ^ 8 ); return 0; } |
Program Output:
1 |
The 4 ^ 8 is : 12 |
As excepted, The output of XOR of 4 and 8 is 12.
Let’s Take another Example:
Let’s look at another example to make our understanding concrete. We will take two numbers a = 20 and b=15.
To Perform any bitwise operation, we need to convert the decimal numbers into a binary numbers.
Binary Equivalent of the a(i.e 21) is 0001 0101
Binary Equivalent of the b(15) is 0000 1111
➡️ Bitwise A ^ B is:
A –> 0001 0101 (21 in Binary)
B –> 0000 1111 (15 in Binary)
A ^ B –> 0001 1010 ( A ^ B = 26 )
The bitwise XOR of 21 and 15 ( 21 ^ 15 ) is 26.
📢 For the Bitwise XOR operation, If both bits are the same output will be False(0).
Bitwise XOR Example 2:
In this program, we are going to perform the XOR operation on the above inputs 21 and 15.
1 2 3 4 5 6 7 |
#include <stdio.h> int main() { // The bitwise XOR of '21' and '15' is '26' printf("The 21 ^ 15 is : %d \n", 21 ^ 15 ); return 0; } |
Program Output:
1 |
The 21 ^ 15 is : 26 |
As you can see, We got the excepted output of 26 by performing the XOR operation on 21 and 15.
Let’s look at how we can use the bitwise XOR Operator.
Invert bit using bitwise XOR Operator:
We can use the bitwise XOR operator to Invert or Toggle any bit of the given Number.
Let’s take number 10, The binary equivalent of the 10 is 1010
So our least significant bit is (Leftmost bit) index is 0. and The Rightmost bit index is 3 ( In the real world we will have 32 bits but for simplicity, we are using 4 bits)
Now we want to Invert the bit at the index 2. i.e We want to change the 2nd bit(from LSB) from 0 to 1.
Here is the logic to Invert the bit
1 2 |
// 'num' is the given number (i.e 10 ) num = num ^ (1 << bit) |
Here we are Left shifting the ‘1’ bit times. ( 1 << 2 which becomes ‘100’ in binary )
Then we are Performing XOR operation with the above result and given number
(num ^ ‘100’) which is equal to (‘1010’ ^ ‘0100’) = ‘1110‘
Which gives us ‘1110’(In binary), Which is equivalent of decimal 14
Here is the C program to Invert/Toggle any bit in a given number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include <stdio.h> int main() { // Input the number int num = 10; // Bit to Invert int bit = 2; printf("Before Inverting the bit : %d\n", num); // Here we are Left shifting the '1' bit times, ( 1 << 2Times = '100') // Then we are Performing XOR operation with above number and target number // (num ^ '100') => ('1010' ^ '0100') => '1110' // Which gives us '1110'(In binary), Which is equivalent of decimal 14 num = num ^ (1 << bit); printf("After Inverting the bit : %d\n", num); return 0; } |
Output:
1 2 3 4 5 |
$ gcc ./invert-bit.c $ ./a.out Before Inverting the bit : 10 After Inverting the bit : 14 $ |
This way we can Invert or Toggle any bit. The bitwise operations in the C are very fast. So whenever possible try to use the bitwise operators.
Bitiwse XOR Operator to Swap the Numbers without extra space:
Here is the logic to swap numbers using the bitwise XOR operator
1 |
a ^= b ^= a ^= b; |
The above statement is a complex statement, Which is the combination of the following statements.
1 2 3 |
a = a ^ b; b = b ^ a; a = a ^ b; |
Here is the explanation about above swap logic
- Step 1, We apply bitwise XOR on variable 'a' and variable 'b'. And assign the result to variable 'a'.
- Step 2: We will apply the bitwise XOR on variable 'b' and variable 'a'. And then assign the result to variable 'b'.
- Step 3: We will perform the bitwise XOR on variable 'a' and variable 'b'. And then assign the result to variable 'a'.
- After performing above three steps, The values of variable ‘a’and variable ‘b’ will be swapped.
Swap two number using XOR operator Example Walkthrough:
Let’s take two variables
'a'and
'b'.
value of
a=11, value of
b=22.
At First step, We will perform
a=a^b;
To apply a^b, We need to have variables 'a'and 'b' values in Binary. And then we will apply the bitwise XOR on them.
- Please note, Bitwise operators operates on the bit level, So we need to imagine the numbers in binary format to understand better.
- To convert Integer to Binary you can following program – Decimal (Integer) to Binary conversion program in C (sillycodes.com)
The Binary Value of a(i.e 11) is 00001011
The Binary value of b(i.e 22) is 00010110
1 2 3 4 5 6 7 |
a=a^b; (first operation) 00001011 (variable 'a' or 11 Binary Equivalent) 00010110 (Variable 'b' or 22 Binary Equivalent) --------- 00011101 (Result of (a ^ b) Operation ) -------- |
Now store above result to variable 'a'. So variable a become 00011101
Now let’s perform the second step b = b^a;
1 2 3 4 5 6 7 8 9 |
// The second step // Perform XOR of variable 'b' and variable 'a' b = b^a; 00010110 (Value of 'b') 00011101 (Value of variable 'a', Which we got from first step) -------- 00001011 (Result of (b ^ a). Store this result to variable 'b' ) -------- |
Store the Above (b^a) operation result into the variable 'b'. So Variable 'b' became 00001011
Now perform the third step a = a^b;
1 2 3 4 5 6 7 8 9 |
// XOR variable 'a' and Variable 'b' a = a^b; 00011101 ( Value of 'a', Which we got from step 1 ) 00001011 ( Value of 'b', Which we got from step 2 ) -------- 00010110 (Result of (a^b) and store result to variable 'a') -------- |
Save the result of above a^b operation to variable 'a'.
Now the variable 'a' became 00010110, Which is equal to Decimal value 22.
and the variable 'b' became 00001011, Which is equal to decimal value of 11.
As you can see the values of variable 'a' and variable 'b'are swapped. We are able to swap two numbers without using third number.
Program : Swap two numbers without using third variable ( Bitwise XOR ):
Let’s convert above XOR swap logic into the code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<stdio.h> int main() { // Take two variable, Two variable 'a' and 'b' for input int a, b; printf("Enter Two Numbers : "); scanf("%d%d", &a, &b); // Let's print the values of 'a' and 'b' before swapping printf("Before Swapping :: Values of a : %d \t b : %d \n", a, b); // In this method, We are going to use XOR operator to swap the numbers. a ^= b ^= a ^= b; // Another represntation of same logic // a ^= b; // b ^= a; // a ^= b; // More readable representation of above logic // a = a ^ b; // b = b ^ a; // a = a ^ b; // Now the numbers are swapped. // Let's print the values of 'a' and 'b' After swapping printf("After Swapping :: Values of a : %d \t b : %d \n", a, b); return 0; } |
Program Output:
We are using GCC compiler to compile the program on Ubuntu Linux.
📢. Learn More above compiling and running program following article – Hello World Program in C language – SillyCodes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Compilling program using GCC compiler venkey@Ubuntu$ gcc swap3.c // Run the Executable venkey@Ubuntu$ ./a.out Enter Two Numbers : 11 22 Before Swapping :: Values of a : 11 b : 22 After Swapping :: Values of a : 22 b : 11 // Example 2 venkey@Ubuntu$ ./a.out Enter Two Numbers : 555 444 Before Swapping :: Values of a : 555 b : 444 After Swapping :: Values of a : 444 b : 555 // Trail 3 venkey@Ubuntu$ ./a.out Enter Two Numbers : 33 999 Before Swapping :: Values of a : 33 b : 999 After Swapping :: Values of a : 999 b : 33 // Trail 4 venkey@Ubuntu$ ./a.out Enter Two Numbers : 10 20 Before Swapping :: Values of a : 10 b : 20 After Swapping :: Values of a : 20 b : 10 venkey@Ubuntu$ |
Related Articles:
- https://sillycodes.com/bitwise-operators-in-c-language/
- https://sillycodes.com/bitwise-or-operator-in-c-programming/
- https://sillycodes.com/bitwise-and-operator-in-c-programming/
3 Responses
[…] 📢 https://sillycodes.com/bitwise-xor-operator-in-c-language/ […]
[…] Bit-wise XOR ( ^ ) […]
[…] have looked at the Bitwise XOR Operator in our earlier articles, In today’s article, we are going to look at the Bitwise Right Shift […]