C Language Practice Programs [300 Programs]
List of C Language Practice Programs:
Here is the list of C Language practice programs we have covered so far. This list is constantly updated.
Basic C Programs
- C Program to perform all Arithmetic Operations
- C Program to calculate Size and Limits of all C Datatypes
- C Program to Swap Two numbers [With and without temporary variable ]
- C Program to Swap the Nibbles of Character
- C Program to Calculate Largest of two numbers using conditional operator
- C program to Convert temperature from centigrade to Fahrenheit
- C Program to understand Pre increment and Pre decrement Operators
- C Program to understand Type Conversion
- C Program to Print All ASCII Charcters and ASCII Values
- C Program to Calculate Area of Circle
- C Program to Calculate Area of Triangle
- C Program to calculate Perimeter and Area of Rectangle
- C program to calculate Simple Interest
- C Program to Find Roots of quadratic equation in C Language
- Program to Calculate Size of Datatypes in C
- C Program to understand float arithmetics
- C Program to Basic Input and Output of datatypes with Format specifiers
If else statement Practice Programs:
- C Program to check whether a number is Even or Odd
- C Program to check Positive or Negative number or Zero
- C Program to Print the Larger Number of Two Numbers
- C Program to check character is Alphabet or Number of Special Number
- C Program to Calculate the Minimum of Three Numbers
- C Program to find the Biggest number from Three Numbers (Maximum)
- C Program to calculate the Roots of a Quadratic Equation
- C Program to Calculate the Remainder and Quotient
- C Program to check given Year is Leap Year or Not
- C program to calculate the Grade of Student using Percentage
- C Program to find the Number of days in Month
- C Program to check character is Vowel or Consonant
- C Program to check character is Uppercase or Lowercase
- C Program to get day of week given week Number
Switch Case Practice Programs:
- C Program to Perform arithmetic Operations using Switch Statement
- C Program to Check the Sign of the Number ( Positive, Negative, or Zero) Using Switch Case
- C Program to find whether the alphabet is a Vowel or Consonant using Switch Case
- Enter the week number and print the day of week program Using Switch Statement
- C Program to calculate the Number of days in Month using the switch statement
- C Program to check Even or Odd Number using Switch Case
- C Program to find the maximum of two numbers using a switch case
- Must Practise: Menu Driven Calculator Program using Switch Case and Do while
C Loops Practice Programs:
- C Program to Print Numbers up to n ( user-provided number)
- C Program to Print Numbers in Reverse Order
- C Program to Calculate Sum of First N natural numbers
- C Program to Reverse a Number
- C Program to Calculate Sum of Digits of a Number
- C Program to Calculate Product of Digits of a Number
- C Program to Count the number of Digits of a Number
- C Program to Multiply without using Multiplication operator (*)
- C Program to generate Fibonacci Series up to a given number
- C Program to Print First n Fibonacci numbers
- C Program to get Nth Fibonacci number
- C Program to Check number is Prime Number or not?
- C Program to Check Prime Number using Square Root (Sqrt) Method
- C Program to Print Prime Numbers between Two numbers
- C Program to print First N Prime numbers
- C Program to generate Prime numbers up to N
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Calculate the Power of Number
- C Program to Check Number is Power of 2
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of number
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
- C Program to Generate Nth Armstrong Number
- C Program to Generate Multiplication Tables
Patterns Programs – ( Star, Number, Pyramid, Rhombus, Triangle, etc):
C Functions Practice Programs:
- C Program to Perform Arithmetic Operations using functions
- Add Two Numbers using Functions in C
- C Program to calculate Fibonacci Series using function
- C Program to find Factorial of a Number using functions
- C Program to find Minimum and Maximum numbers using Functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
Recursion Practice Programs in C:
- C Program to Calculate the Factorial of a Number using Recursion
- C Program to generate Fibonacci series using Recursion
- C Program to print first N Natural Numbers using Recursion
- C Program to calculate the sum of N Natural Numbers using Recursion
- C Program to calculate the Product of N Natural Numbers using Recursion
- C Program to Count the Number of digits in a Number using Recursion
- C Program to Calculate the Sum of Digits using Recursion
- C Program to Product the Number of digits in a Number using Recursion
- C Program to Reverse a Number using Recursion.
- C Program to check whether the number is Palindrome using Recursion
- C Program to Calculate LCM using Recursion.
Arrays Practice Programs in C Language:
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
- Linear Search in C Language with Example Programs
- C Program to Count Frequencies of Each Element in the Array
Matrix Practice Programs in C ( 2D-Array Programs):
- C Program to Read and Print Matrix or 2D Array
- C Program to demonstrate How to Pass 2D Array to Functions
- C Program to Multiplication of two Matrices
- C Program to Add Two Matrices
- C Program to Subtract Two Matrices
- C Program to Calculate the Transpose of Matrix
- C Program to Check Two Matrices are Equal
- C Program to Check Multiplicability of Two Matrices
- C Program to Check a Sparse Matrix
- Matrix and Scaler Multiplication Program in C
- Program to Check Identity Matrix in C Language
- Program to Check Symmetric Matrix in C
- Print Diagonal Elements of Matrix in C Language
- Sum of Diagonal Elements of Matrix in C Language
String Programs in C Language:
- C Program to Calculate Length of the String
- C Program to Copy a String to New String
- C Program to Concatenate Two Strings
- C Program to Compare Two Strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Count Number of Words in a String
- C Program to Implement Custom atoi() function
- Anagram Program in C – Check two strings are anagrams
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
- C Program to Find All Occurrences of a character in string
- C Program to Count All Occurrences of character in string
- C Program to Swap two strings
- C Program to Search for substring in a String
- C Program to Find Last Occurrence of Substring/Word in a String
- C Program to Find All Occurrences of Substring in a String
- C Program to Count All Occurrences of Substring in a String
- Custom strstr() function implementation in C
String Library Functions:
- strlen library function in C
- strcpy library function in C with Example Programs
- strcat library function in C
- strcmp library function in C
- strchr library function in C
- strstr library function in C
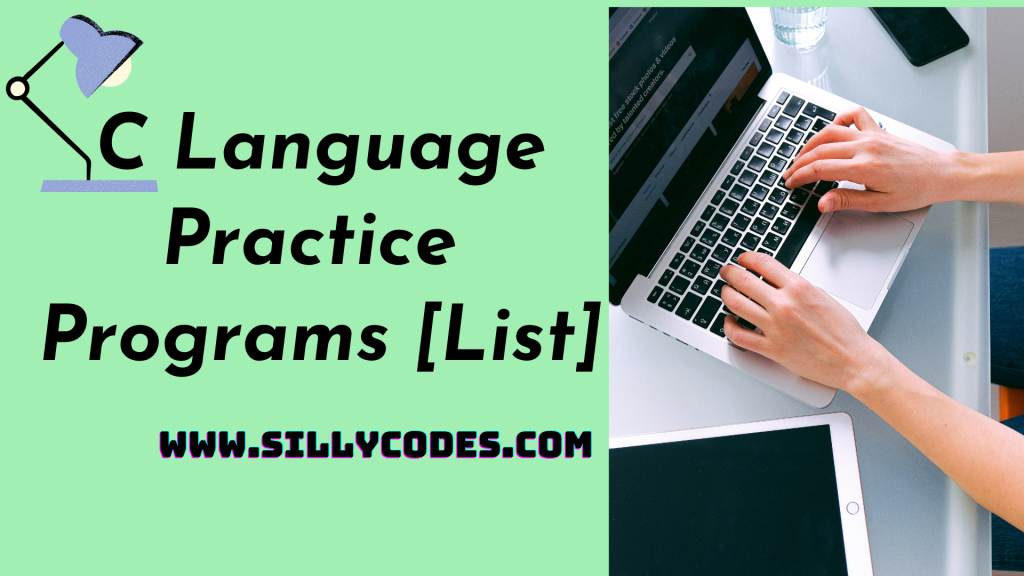
44 Responses
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]
[…] C Programs […]