Void Pointer in C Programming Language
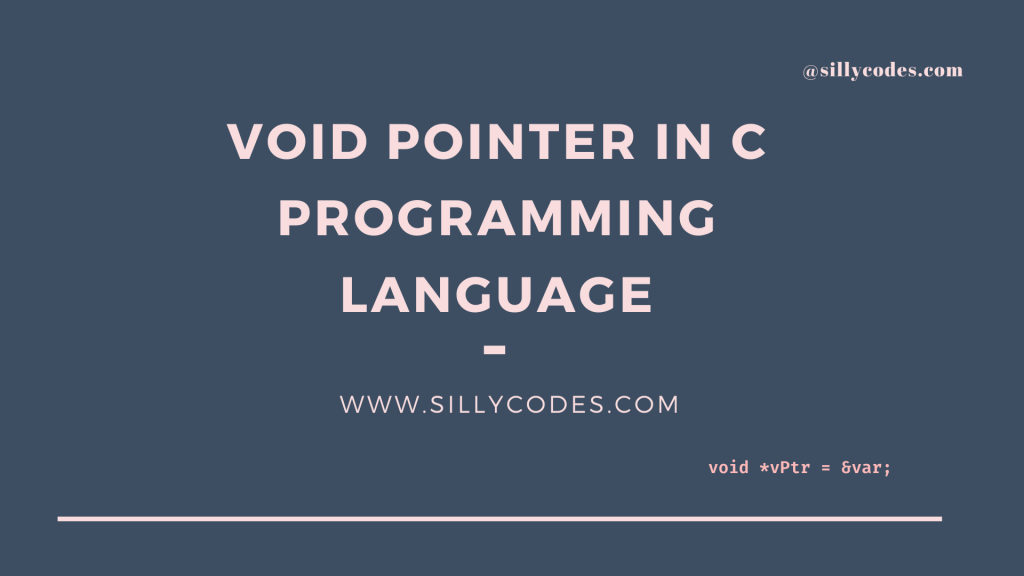
Introduction:
We have covered the C Pointers, Pointer Arithmetic, and Pointer to pointers in earlier articles. In today’s article, We will look at the void pointer in c programming language.
📢 Recommended: C Practice Programs Collection
Void Pointer in C Programming Language:
A pointer is a special variable, that can store the address of another variable. To declare the pointer variable we usually specify the pointer name with the pointer datatype.
int *ptr;
The data type of the pointer depends on the data the pointer going to point to ( Store the address).
If you want to store the address of the integer data, Then we create an integer pointer int *. Similarly, if you want to store the character data, then we will create a character pointer char *. and so on so.
Let’s say you have a character pointer chPtr.
char *chPtr;
Then you can’t use the character pointer to store any other datatype address other than the character data.
This is where the void pointer comes in handy. We can store the address of any datatype using the void pointer in c programming language. The void pointer can point to any type of data.
Declaring Void Pointer in C Language:
Let’s look at the declaration of the void pointer in c.
Syntax of a void pointer in c:
void *vPtr;
Here the void is the keyword in c and the vPtr is the void pointer.
Assign an address to void pointer in C Programming:
As specified earlier, We can assign any datatype addresses to the void pointer. so let’s assign the address of the integer value to the void pointer.
Create an integer variable, let’s say it is the edge variable.
int edge = 364;
Now, Create a void pointer vPtr
void * vPtr;
Let’s assign the address of the integer variable edge to the void pointer vPtr. Use the address of operator like below.
vPtr = &edge;
Now the void pointer vPtr is storing the address of the integer variable edge.
📢 Similarly, We can assign the void pointer to any other datatype pointer as well.
Let’s write a program to confirm if the void pointer is holding the correct address of the integer variable.
Program to demonstrate the void pointer in c language:
Here is the program to store the integer variable address using the void pointer in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
/* program to demonstrate the void pointer in c sillycodes.com */ #include<stdio.h> int main() { // create an integer variable int edge = 364; // declare a void pointer void *vPtr; // assign address of integer to void ptr. vPtr = &edge; // print the addresses. printf("Address of edge variabel (&edge) : %p\n", &edge); printf("Value of void pointer (vPtr): %p\n", vPtr); return 0; } |
In the above program, We have created an integer variable called edge and stored the value 364. Then we created a void pointer named vPtr. As a void pointer can store the address of another type of variable, We stored the integer variable edge address in a void pointer vPtr.
1 |
vPtr = &edge; |
Program Output:
Let’s compile and run the program using GCC compiler (Any of your favorite compilers).
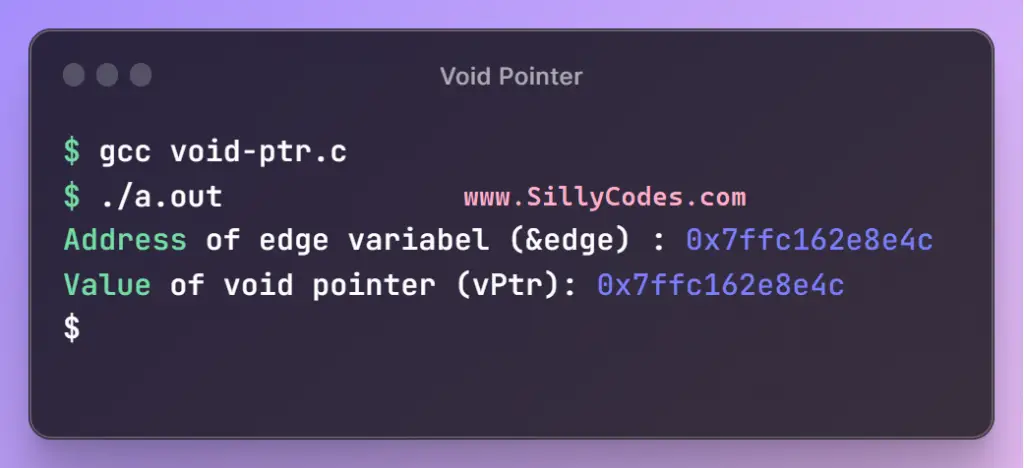
Now we know that the void pointer is able to store the address of another data type variable. let’s look at how we can get the value using the void pointer in c.
Get the value of a variable using a void pointer in C Programming:
We typically dereference a pointer variable to obtain the value stored by the pointer variable. However, dereferencing a void pointer in c will not produce the desired results. We also need to type case the void pointer to the appropriate datatype before performing the dereference operation on it.
For example, If you want to get the value pointed by the above void pointer variable vPtr. First of all, we need to typecast the vPtr to integer data type ( as vPtr is pointing to the integer variable edge). Then we can perform the dereference operation using the indirection/dereference operator.
So To get the value pointed by the void pointer, typecase the pointer and dereference it like below.
*(int *) vPtr;
Here the (int *) is the typecast operation. We are typecasting the void pointer to the integer pointer. Finally, performed the dereference operation on the type casted pointer.
Let’s look at the program to get the variable value through the void pointer.
Program to Get variable value through the void pointer in C Programming Language:
Here is the program to Access an integer variable value through the void pointer.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/* program to get variable value through void pointer in c sillycodes.com */ #include<stdio.h> int main() { // create an integer variable int edge = 364; // declare a void pointer void *vPtr; // assign address of integer to void ptr. vPtr = &edge; // normally getting the value printf("Value of the edge is : %d\n", edge); // get the value of the 'edge' using the vPtr printf("Value of edge using void pointer : %d\n", *(int *)vPtr ); return 0; } |
At Line 23 in the above program, We used the *(int *)vPtr operation to get the value of the integer variable through the void pointer. We typecasted the void pointer to the integer pointer, Then applied the indirection operation.
📢 Don’t forget the parenthesis while performing the typecast operation. – *(int *)vPtr
Program Output:
Compile the program.
$ gcc get-value-using-void-ptr.c
Run the program.
1 2 3 4 |
$ ./a.out Value of the edge is : 364 Value of edge using void pointer : 364 $ |
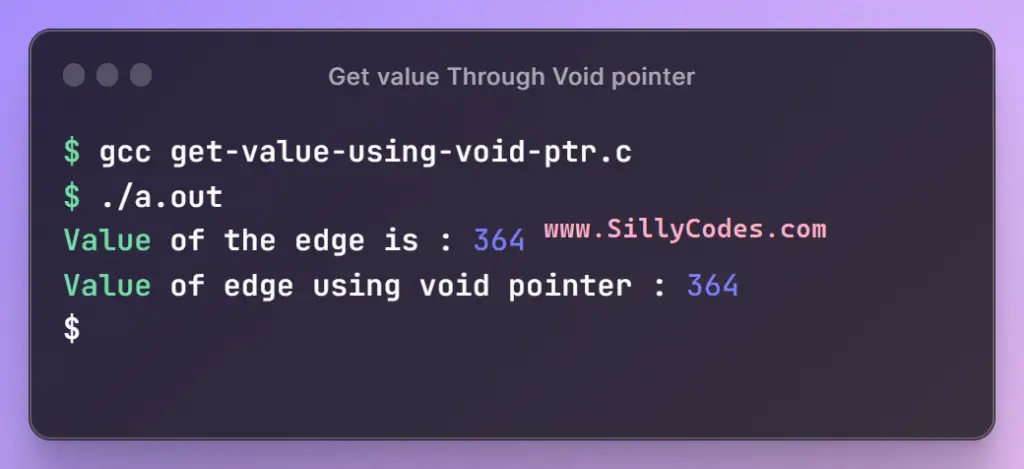
As we can see from the above output, We are able to get the value of the integer variable edge using the void pointer vPtr. ( Typecast + dereference)
Related Articles:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Program to perform Arithmetic Operations using Pointers in C
- Swap Two Numbers using Pointers in C Language
- Pointers and Arrays in C Language with Example Programs
- Accessing Array Elements using Pointers in C
- Print String Elements using Pointers in C Language
- Function Returning Pointer in C Programming Language
- Function Pointers in C with Example Programs
3 Responses
[…] have looked at the void pointer in c in an earlier article. In today’s article, We will look at the wild pointer in c programming […]
[…] void * – The realloc() function also returns the void pointer. […]
[…] Void Pointer in C Programming Language […]