Continue Statement in C Language with Examples
- Introduction:
- Pre-Requisites:
- Continue Statement in C Language:
- Syntax of Continue statement:
- The flow diagram of the Continue Statement:
- Example program to understand the Continue statement in C language:
- Sum of Even Numbers Program Algorithm:
- Continue Statement with Different Loops:
- Continue statement with the for loop in C language:
- Program to print Odd numbers from 1 to 10 using Continue Statement.
- Continue Statement with the while loop in C:
- Continue Statement with the do while loop in C programming:
- Continue Statement with the Nested loops in C:
- Conclusion:
- C Tutorials Index Page:
- C Loops Practice Programs:
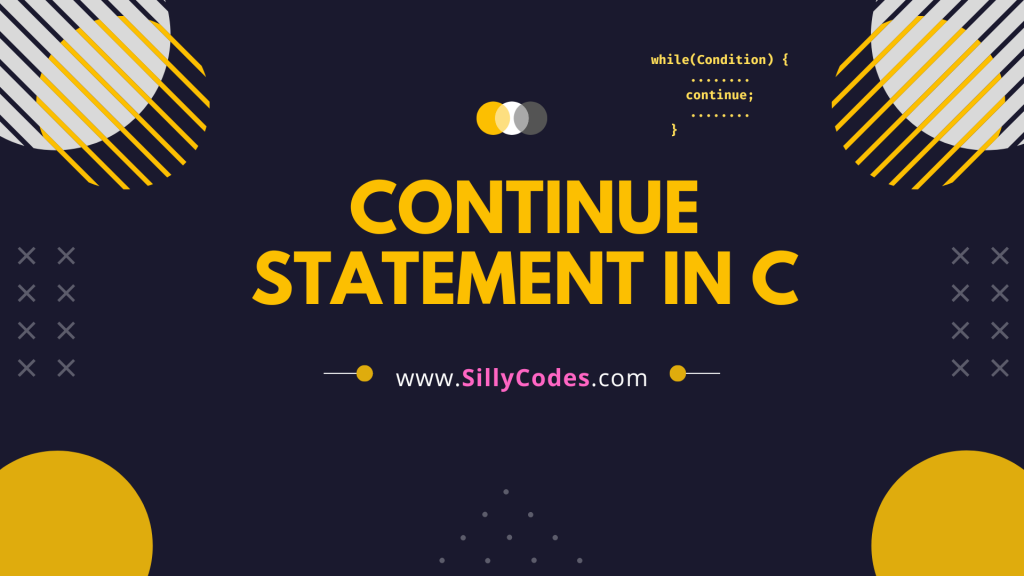
Introduction:
We have looked at the loops and break Statement in earlier posts. In today’s article, We are going to discuss about the Continue Statement in C language and how we can use it. Let’s get started.
Pre-Requisites:
It is recommended to have minimal knowledge of the loops in C. Please go through the following articles.
Continue Statement in C Language:
The Continue statement is used to skip the present loop iteration and takes the program control back to the loop condition for the next iteration.
The continue statement is kind of the opposite of the break statement. The break statement is used to terminate the loop completely and control goes out of the loop. The Continue statement is used to skip the current iteration but the loop won’t terminate, the loop will proceed to the next iteration of the loop.
We use the continue statement in the loops.
Syntax of Continue statement:
1 |
continue; |
Here is the syntax of the continue statement with the while loop
1 2 3 4 5 6 |
while(condition) { // statements ... continue; ... } |
As you can see from the above syntax, As soon as the continue statement is executed, control goes back to the while condition and starts executing the next iteration (if the while condition is still true)
The flow diagram of the Continue Statement:
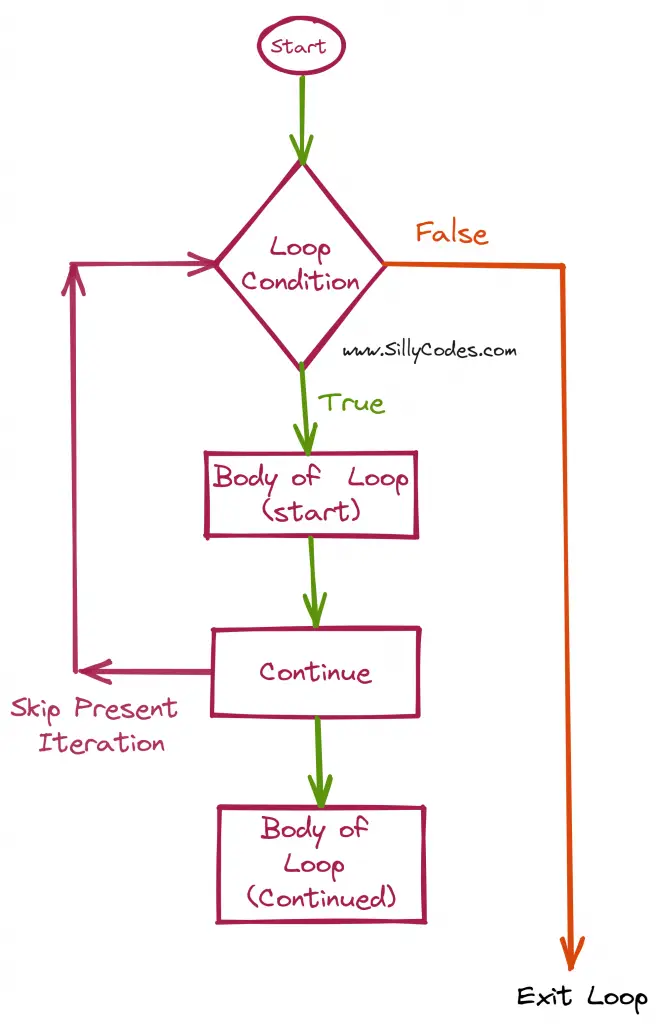
Here is another graphical representation of the continue statement flow.
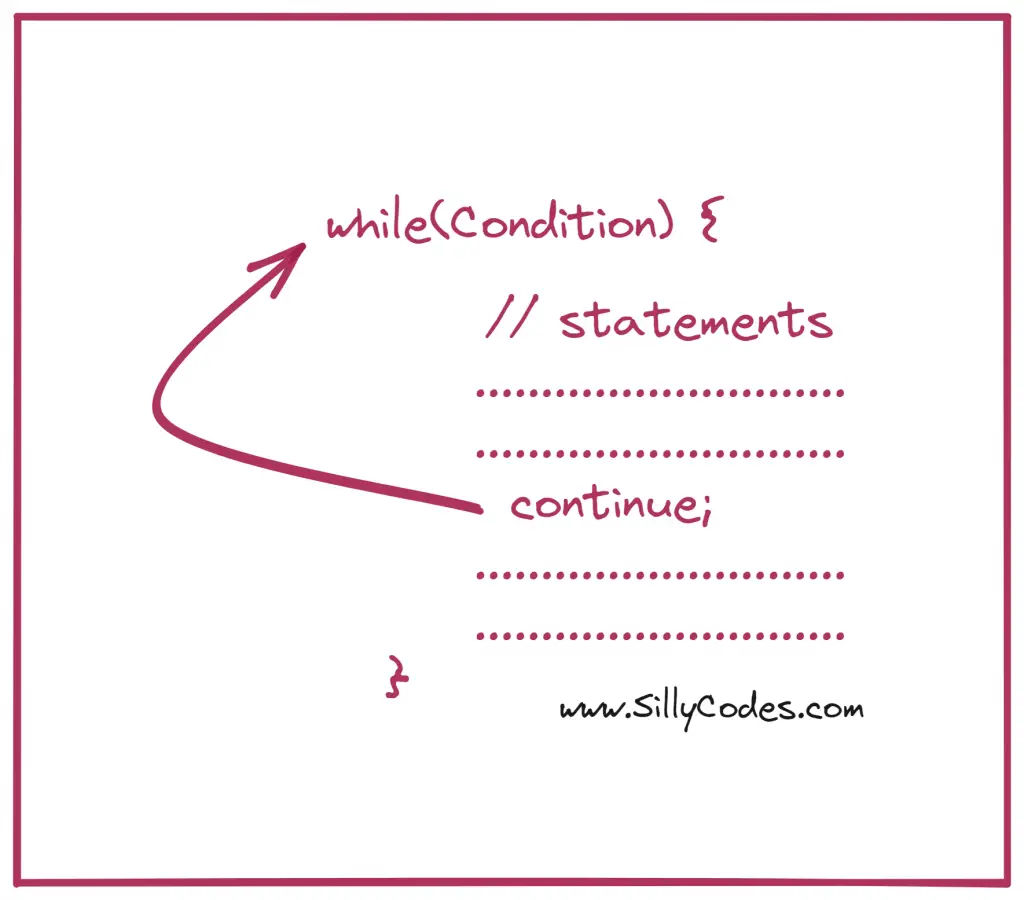
Example program to understand the Continue statement in C language:
Let’s write a C Program Sum of Even Numbers from 1 to 20.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/* Program : Sum of Even numbers from 1 to 20. Author : Sillycodes.com */ #include<stdio.h> int main() { // 'counter' is our loop counter int counter; int sum = 0; // we store sum so far here. for(counter = 1; counter <= 20; counter++) { // If the 'counter' is ODD Number // Then we no need to perform the addition // so we will skip the iteration and goes for next iteration // check if the number is ODD using modulus operator if (counter % 2 == 1) { // ODD Number // skip the iteration using the 'continue' statement continue; } // If we reached here, Means we have the 'Even' Number sum = sum + counter; } printf("Sum of Even Numbers from 1 to 20 is : %d \n", sum); return 0; } |
Program Output:
1 2 3 4 |
$ gcc continue-statement.c $ ./a.out Sum of Even Numbers from 1 to 20 is : 110 $ |
Sum of Even Numbers Program Algorithm:
- We have used two variables counter and sum. The counter variable is used to iterate from 1 to 20 and the sum variable is used to store the sum so far. The sum variable will be initialized with zero
- We use the for loop to iterate from 1 to 20 with the help of a counter variable. The counter variable starts with the value 1 and for each iteration counter value will increase by 1.
- For each iteration,
- We will check if the counter is an even or odd number. As our program is to calculates the sum of the even numbers, We don’t really worry about the odd numbers so we can skip the odd numbers.
- So First check inside the for loop is to verify if the counter value is even or odd number. We used the Modulus operator ( counter % 2 == 1 ) to check the number. If the above condition returns true then the counter is an Odd number. If the counter % 2 == 1 becomes false then the counter is an Even number.
- If the counter is an odd number, Then we no need to add the value to the sum, So we can skip this iteration. To do that, We can use the continue statement. which skips the present iteration and proceeds to the next iteration. ( Make sure to increment the loop control variable i.e counter)
- If the counter is an Even number, Then we add the counter value to the existing sum value using the sum = sum + counter statement, and control goes to the next iteration. ( Make sure to increment the counter variable)
- This process continues until the counter reaches the value 21. Once the counter reached 21 the for loop condition counter <= 20 becomes false. and the loop will be terminated.
- Once the loop is terminated, we are printing the result of the sum using the printf function.
📢 Here we used the continue statement in c programming to skip the iteration where the counter value became an Odd number. This way we can take advantage of the continue statement to skip the unnecessary iteration based on our program logic.
Continue Statement with Different Loops:
Now Let’s look at how we can use the continue statement with different loops.
- continue statement with for loop
- continue statement with a while loop
- continue statement with do while loop
Continue statement with the for loop in C language:
We have already used the for loop in the above Sum of Even Numbers program.
Syntax:
1 2 3 4 5 6 7 8 |
for ( init; condition; update) { // statements ... ... continue; ... ... } |
Let’s write a program to print the Odd numbers from 1 to 10 using the continue statement in c programming.
Program to print Odd numbers from 1 to 10 using Continue Statement.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
/* Program : Display odd numbers from 1 to 10. Author : Sillycodes.com */ #include<stdio.h> int main() { // 'i' is our loop counter int i; for(i = 1; i <= 10; i++) { // check if the 'i' is Even or Odd if( i%2 == 0) { // Even Number, Skip Iteration // go to next iteration continue; } printf("%d ", i); } // print newline printf("\n"); return 0; } |
Program Output:
1 2 3 4 |
$ gcc continue-for-loop.c $ ./a.out 1 3 5 7 9 $ |
Here we used the continue statement to skip the iteration, So whenever we got an Even value for the counter (i.e i), We skipped the iteration using the continue statement and went to the next iteration.
If the i value is an Odd number, Then only we proceeded further and printed the value onto the console.
Continue Statement with the while loop in C:
As specified earlier we can use the continue statement with any loop Here is an example of the while loop and the continue statement.
Let’s write a program to add all Odd numbers from 1 to 10 using the continue statement. We have looked at the Sum of Even number the program above using the for loop, This program is the opposite of that program.
Sum of Odd Numbers using Continue Statement and while loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
/* Program : Sum of Odd numbers from 1 to 10. Author : Sillycodes.com */ #include<stdio.h> int main() { // 'counter' is our loop counter int counter = 1; int sum = 0; // we store 'sum' so far here. while (counter <= 10) { // If the 'counter' is Even Number // Then we no need to perform the addition // so we will skip the iteration and goes for next iteration // check if the 'counter' is Even number using modulus operator if (counter % 2 == 0) { // 'counter' is an Even Number // skip the iteration using the 'continue' statement // don't forgot to 'increment' the 'counter' counter++; continue; } // If we reached here, Means we have an 'Odd' Number sum += counter; // sum = sum + counter; // Increment the loop control variable. counter++; } // end of while loop printf("Sum of Odd Numbers from 1 to 10 is : %d \n", sum); return 0; } |
Program Output:
1 2 3 4 |
$ gcc continue-while-loop.c $ ./a.out Sum of Odd Numbers from 1 to 10 is : 25 $ |
As you can see from the above program, We used the continue statement to skip the iterations where the counter(i.e i) is even number.
📢 Make sure to increment the counter variable i.e i before using the continue statement. If you forgot to increment the counter variable, then we might get the Infinite loop.
Continue Statement with the do while loop in C programming:
Similar to other loops, We can use the continue statement in do while loop and skip the specific iteration.
Let’s look at an example program. We want to print the numbers from 90 to 100 except the 93 and 97 numbers.
Do While loop with Continue Statement Example Program (skip numbers):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/* Program : Print numbers using do while and continue Author : Sillycodes.com */ #include<stdio.h> int main() { // 'i' is our control variable int i = 90; do { // check if the 'i' is equal to '93' or '97' // if 'i' matches these numbers, then skip iteration if (i == 93 || i == 97) { // skip the iteration using the 'continue' statement // don't forgot to 'increment' the 'i' (loop control variable) i++; continue; } // print the number printf("%d ", i); // Increment the loop control variable. i++; } while (i <= 100); // Print New Line printf("\n"); return 0; } |
Program Output:
1 2 3 4 |
$ gcc continue-do-while.c $ ./a.out 90 91 92 94 95 96 98 99 100 $ |
As you can see from the above output, We haven’t printed the values 93 and 97. and printed the remaining numbers from the 90 to 100.
Here also, We used the continue statement to skip the loop iteration, whenever the i value is equal to either of 93 or 97 numbers.
Continue Statement with the Nested loops in C:
If we use the continue statement in the nested loops, Then only the Inner-most loop iteration will be skipped. It won’t affect the OuterLoop iterations.
Please have a look at the following program.
Nested loops flow with Continue Statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/* Program: Nested Loops and Continue Author: Sillycodes.com */ #include<stdio.h> int main() { int i, j; // Outerloop start for(i=1; i<=5; i++) { printf("OuterLoop - i : %d \n", i); // InnerLopp Start for(j=1; j<=5; j++) { // skip the iteration if the 'j' is Even. // This will skip the iteration of InnerLoop Only. if(j%2 == 0) { continue; } printf("InnerLoop - j : %d \n", j); } } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
$ gcc continue-nested-loops.c $ ./a.out OuterLoop - i : 1 InnerLoop - j : 1 InnerLoop - j : 3 InnerLoop - j : 5 OuterLoop - i : 2 InnerLoop - j : 1 InnerLoop - j : 3 InnerLoop - j : 5 OuterLoop - i : 3 InnerLoop - j : 1 InnerLoop - j : 3 InnerLoop - j : 5 OuterLoop - i : 4 InnerLoop - j : 1 InnerLoop - j : 3 InnerLoop - j : 5 OuterLoop - i : 5 InnerLoop - j : 1 InnerLoop - j : 3 InnerLoop - j : 5 $ |
Program Explanation:
As you can see from the above output, We have a continue statement in the body of the InnerLoop. We are checking if the InnerLoop counter (i.e j) is even or odd number. If j value is an even number, Then we are skipping that specific iteration of InnerLoop using the continue statement.
Please note that, the OuterLoop iterations are not skipped due to the InnerLoop continue statement.
📢 The InnerLoop continue statement won’t affect the iterations of the OuterLoop.
Conclusion:
We covered the Continue statement in C language with examples in today’s article. We also discussed the various uses of the continue statement. Finally, we explored the nested loops and the continue statement.
4 Responses
[…] Continue Statement in C Language […]
[…] loop starts by initializing i to 0. It will continue looping as long as the current character ( inputStr[i]) is not a NULL character ( ''). At […]
[…] a For loop by initializing variable i with 0. It will continue looping as long as the current character ( inputStr[i]) is not a NULL character ( […]
[…] of the character in input string. The For loop starts by initializing variable i to 0. It will continue looping as long as the present character ( inputStr[i]) is not a NULLcharacter ( […]