do while loop in C Language with example programs and step-by-step walk-through.
- Introduction:
- do-while loop in C Language:
- Syntax of do while loop in C Language:
- Flow Diagram of do while Loop in C Programming:
- How do while loop works?
- The Sum of N digits program using the do while loop in C:
- Program Explanation: do while Loop in C Step by Step walk-through:
- Infinite loops using the do while loop in C programming:
- Avoiding Infinite do while loop in C:
- Difference between the while loop and do while loop in C Language:
- When to use do while loop:
- Menu-driven Calculator using Switch Case and Infinite do while loop in C language:
- Menu-driven Calculator program using do while loop:
- Menu-driven calculator Program Explanation:
- Exercise:
- Conclusion:
- Related Tutorials:
- Practise Programs using the loops in C language:
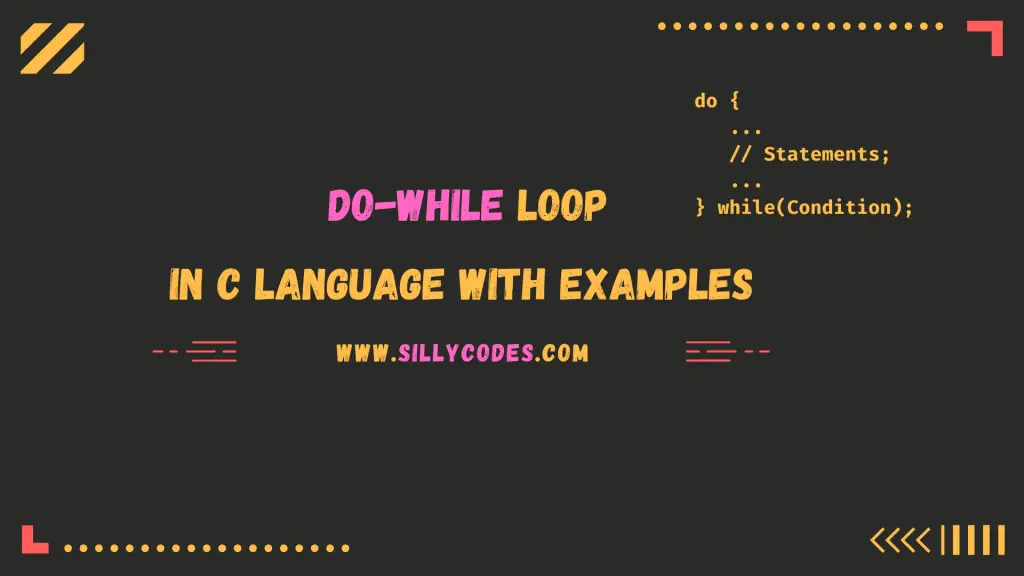
Introduction:
We have discussed about the while loop and for loop in c language in our earlier articles, In today’s article, We are going to look at the do while loop in C language with example programs. We also look at the step-by-step walk-through of the do while loop in C programming.
do-while loop in C Language:
The do while loop in C Programming is very similar to the while loop. But it has one difference, The body of the do while loop is executed at least once.
In the case of the while loop and for loop, The Condition is evaluated first, Once the condition returns True, Then only the body of the while or for loop executes.
But In the do while loop, The body of the do while loop is executed first, and then it checks the loop condition
📢 So, The body of the do-while loop is executed at least once. Even when the loop condition is False.
Syntax of do while loop in C Language:
1 2 3 4 5 |
do { .... // Body of do-while loop .... } while( Condition ); |
Flow Diagram of do while Loop in C Programming:
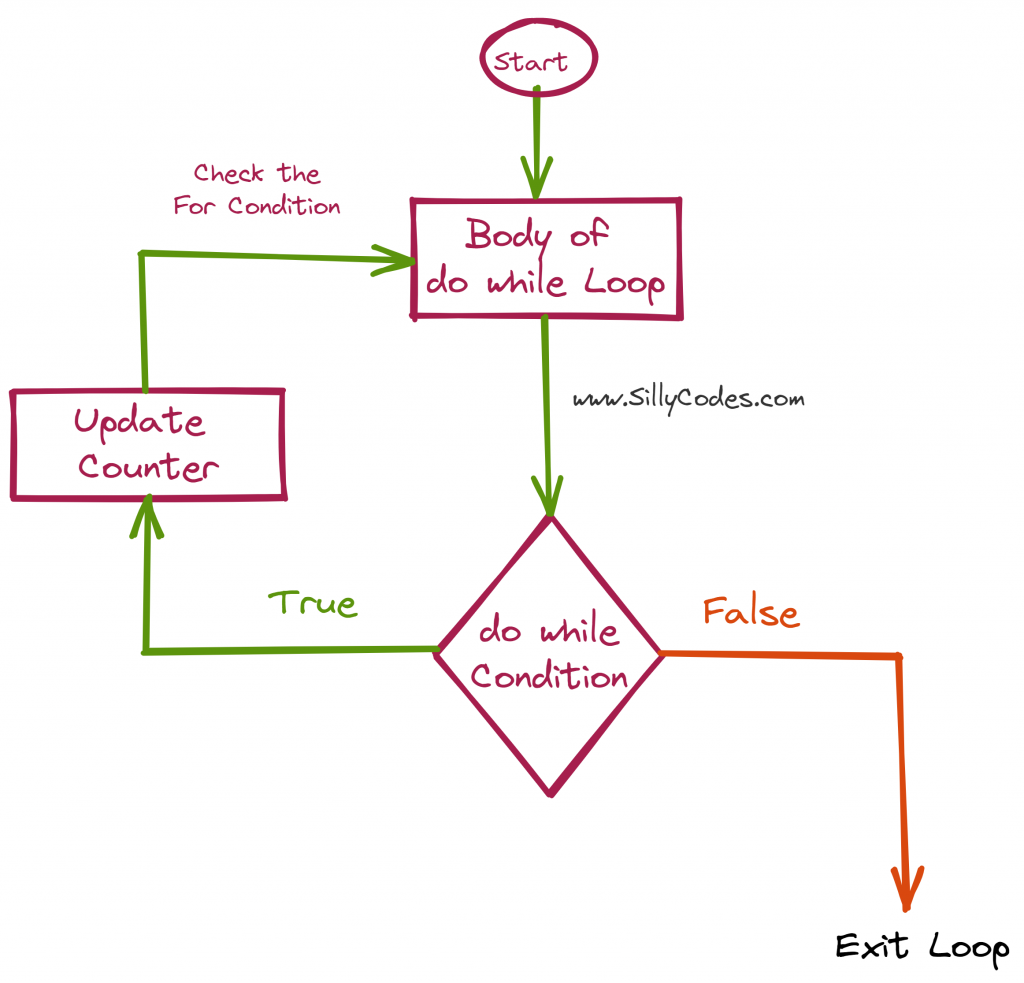
How do while loop works?
As you can see from the above syntax, The do-while loop starts with the do keyword, Followed by the body of the loop and then the loop condition.
In the first Iteration, do while won’t check the loop Condition, It executes the body of do while loop, Once the body of the loop is executed, We will check the loop Condition.
If the Condition returns True, Then the body of the do while loop is executed again, This process continues until the loop Condition becomes False.
Once the loop Condition became False, Then do while loop will terminate and control comes out of the loop to execute the subsequent statements in the program.
📢 The do while loop needs to end with the semicolon (;). Make sure to add the semicolon at the end of the loop.
Let’s look at a program to understand the do while loop.
The Sum of N digits program using the do while loop in C:
We are going to use the do while loop to calculate the sum of the N (user-provided number) numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* Program: Calculate Sum of Given Number */ #include<stdio.h> int main() { int total, num; // 'i' is our loop counter int i=1; // 'sum' is the variable where we maintain the sum so far. int sum = 0; // Take the input from the user printf("How many numbers you want to add: "); scanf("%d", &total); do { // Take the numbers from User printf("Enter a number: "); scanf("%d", &num); // Add the `num` to `sum` sum += num; // sum = sum + num; // Increment the counter - 'i' i++; } while(i<=total); // Loop Condition // Print the sum printf("Sum : %d\n", sum); return 0; } |
The Sum of digits Program output:
1 2 3 4 5 6 7 8 9 10 |
$ gcc do-while.c $ ./a.out How many numbers you want to add: 5 Enter a number: 10 Enter a number: 20 Enter a number: 30 Enter a number: 40 Enter a number: 50 Sum : 150 $ |
Program Explanation: do while Loop in C Step by Step walk-through:
First of all, We are asking the user for the number of digits the user wants to add.( How many numbers you want to add: ) and save the results in total variables ( Let’s say total = 5 here)
Then the program control reaches the do while loop.
First Iteration:
- Execute the Body:
- As we learned earlier, The do while loop first executes the body of loop.
- So we displayed the Enter a number: prompt on the console and waited for user input. Once the user provided the input, We will store the value in num variable.
- Then We are adding the
num value to
sum
- By default, The value of sum is , and In the above example, The User entered 10.
- So we added sum = sum + num which is sum = 0 + 10 . Sum became 10.
- After that, we incremented our loop control variable i by 1. So our counter variable i becomes 2
- Check Condition:
- Finally, We are going to check the do while loop Condition ( i<=total), Which is 2 <= 5, This condition will be evaluated as True. So the Loop will continue.
Second Iteration:
- Execute the Body:
- We go back to the start of the loop, We display the Enter a number: prompt on the console and wait for user input. Once the user provided the input, We will store the value in num variable.
- Then We are adding the value of
num and
sum
- The present sum value is 10, and In the above example, The User entered 20 for the second value.
- So we added sum = sum + num which is sum = 10 + 20. So Sum became 30.
- After that, we incremented our loop control variable i by 1. So our counter variable i becomes 3
- Check Condition:
- Finally, We will check the do while loop Condition ( i<=total), Which is 3 <= 5, This condition will be evaluated as True. So the Loop will continue.
Third Iteration:
- Execute the Body:
- We go back to the start of the loop, We display the Enter a number: prompt and wait for user input. Once we got the input, We will store the value in num variable.
- Then We are adding the value of
num and
sum
- The present sum value is 30, and In the above example, The User entered 30
- So we added sum = sum + num which is sum = 30 + 30. So Sum became 60.
- After that, we incremented our loop control variable i by 1. So our counter variable i becomes 4
- Check Condition:
- Finally, We will check the do while loop Condition ( i<=total), Which is 4 <= 5, This condition will be evaluated as True. So the Loop will continue.
Fourth Iteration:
- Execute the Body:
- We go back to the start of the loop, We display the Enter a number: prompt and wait for user input. Once we got the input, We will store the value in num variable.
- Then We are adding the value of
num and
sum
- The present sum value is 60, and In the above example, The User entered 40
- So we added sum = sum + num which is sum = 60 + 40. So Sum became 100.
- After that, we incremented our loop control variable i by 1. So our counter variable i becomes 5
- Check Condition:
- Finally, We will check the do while loop Condition ( i<=total), Which is 5 <= 5, This condition will be evaluated as True. So the Loop will continue.
Fifth Iteration:
- Execute the Body:
- We go back to the start of the loop, We display the Enter a number: prompt and wait for user input. Once we got the input, We will store the value in num variable.
- Then We are adding the value of
num and
sum
- The present sum value is 100, and In the above example, The User entered 50
- So we added sum = sum + num which is sum = 100 + 50. So Sum became 150.
- After that, we incremented our loop control variable i by 1. So our counter variable i becomes 6
- Check Condition:
- Finally, We will check the do while loop Condition ( i<=total), Which is 6 <= 5, This condition will be evaluated as False.
- So the Loop will Terminate and Program control comes out of the do while loop.
Final Output: Sum : 150
📢 As you can, We always execute the body of the do while loop first, and then we check the do while loop condition
Infinite loops using the do while loop in C programming:
Any do while loop can become an Infinite loop if the do while loop condition never becomes False. So the loop will run forever.
The Infinite loops can occur, when we forgot to Increment or Decrement the loop counter (control variable).
Here is an example of an Infinite loop using the do while loop.
Infinite do while Loop example:
We are going to write a program to print the numbers 1 to 5 on the console using the do while loop. We are using the counter variable as a loop control variable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
/* Program: Display numbers using do while loop */ #include<stdio.h> int main() { // 'counter' is our loop counter int counter = 1; do { // print the number 'counter' on to console. printf("%d ", counter); // We forgot to increment the 'counter' // which creates the infinite do while loop } while(counter <= 5); // print the newline for proper formatting. printf("\n"); return 0; } |
Program Output:
1 2 3 |
$ ./a.out 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 // Press CTRL + C to terminate the loop |
In the above program, We have forgot to increment the counter inside the do while loop, so the counter variable value stayed at 1 forever.
So at each iteration, we execute the body of the do while loop and check the condition, which is counter ≤ 5 , As the counter value stayed at 1, The condition never becomes false, So do-while runs forever hence creating an Infinite do while loop.
That is the reason our output continuously prints the 1’s.
Avoiding Infinite do while loop in C:
To solve the Infinite loop , Simply increase the counter variable inside the do while loop.
Here is the code without the Infinite loop.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
/* Program: Display numbers using do while loop */ #include<stdio.h> int main() { // 'counter' is our loop counter int counter = 1; do { // print the number 'counter' on to console. printf("%d ", counter); // Increment the 'counter' counter++; } while(counter <= 5); // print the newline for proper formatting. printf("\n"); return 0; } |
Program Output:
1 2 3 4 |
$ gcc dowhile-infinite.c $ ./a.out 1 2 3 4 5 $ |
Infinite loop with always True condition while (1):
We can create a infinite loop by specifying the do while loop condition as the 1 or any valid expression which always returns True.
Here is an example.
1 2 3 4 5 6 7 8 9 10 11 12 |
/* Program: Infinite do while loop */ #include<stdio.h> int main() { do { // This is going to run forever printf("Loop Executing..."); } while(1); // This condition is always returns True. return 0; } |
Difference between the while loop and do while loop in C Language:
The main difference between the while loop and do while loop is, The Body of the do while loop executes at least once. So you are guaranteed to execute the body of the loop even when the do while loop condition fails.
And of course, there are some syntactical differences between the while and do while. But at the core, Both loops achieve the same functionality Repeating a piece of code multiple times based on a condition
When to use do while loop:
There will be cases where you want to execute certain statements regardless of loop Condition, Then we can use do while loop.
For example, if You want to write a Menu-driven program, Where you display the instructions to the user based on user input, You take the decision. In such cases, You need to display the instructions on whether the Condition is True or False.
Here is an example program, Menu-Driven Calculator using the do while loop in C programming( In fact, Infinite do loop).
Menu-driven Calculator using Switch Case and Infinite do while loop in C language:
- This is a Menu-driven calculator program, So the program displays a Menu with the Options.
- Menu Options are the operations you want to perform, Like 1 for Addition, 2 for subtraction, etc.
- The Program waits for the user’s input, User can provide any numeric option based on Menu.
- After that, the program prompts the Two Number to carry out the chosen operation.
- Once the operation is complete, Program displays the result on the console.
- The program will display the Menu once more to accommodate the user’s new request.
- If the user selects the Invalid option, Program will display an Error message and presents the Menu again.
- The User can exit the program by specifying Option 6
Menu-driven Calculator program using do while loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
/* Program: Calculate Sum of Given Number */ #include<stdio.h> int main() { int num1, num2; int result; int in; do { printf("\n***** Calculator *****\n"); printf("1. Addition\n"); printf("2. Substraction\n"); printf("3. Multiplication\n"); printf("4. Division\n"); printf("5. Modulo Division\n"); printf("6. Exit\n"); printf("Select a Number (1-5): "); scanf("%d", &in); // If 'User' Enters 6 - Stop the program. if(in == 6) { printf("Thank you for trying! Bye! \n"); break; } // Take two numbers from the user printf("Enter Two Numbers: "); scanf("%d%d", &num1, &num2); switch (in) { case 1: printf("Addition of %d + %d = %d \n", num1, num2, num1+num2); break; case 2: printf("Subtraction of %d - %d = %d \n", num1, num2, num1-num2); break; case 3: printf("Product of %d * %d = %d \n", num1, num2, num1*num2); break; case 4: printf("Division of %d / %d = %d \n", num1, num2, num1/num2); break; case 5: printf("Division of %d %% %d = %d \n", num1, num2, num1%num2); break; default: printf("Invalid Option, Please select the valid Options\n"); break; } } while(1); return 0; } |
Output: Menu Driven Calculator Demo:
We used the GCC compiler to compile the program learn more at the following URL.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
$ gcc menu-calculator.c $ ./a.out ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 3 Enter Two Numbers: 10 20 Product of 10 * 20 = 200 ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 1 Enter Two Numbers: 50 50 Addition of 50 + 50 = 100 ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 2 Enter Two Numbers: 764 29 Subtraction of 764 - 29 = 735 ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 10 Enter Two Numbers: 10 20 Invalid Option, Please select the valid Options ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 5 Enter Two Numbers: 20 3 Division of 20 % 3 = 2 ***** Calculator ***** 1. Addition 2. Substraction 3. Multiplication 4. Division 5. Modulo Division 6. Exit Select a Number (1-5): 6 Thank you for trying! Bye! $ |
Menu-driven calculator Program Explanation:
- As you can see from the above output, We display the Menu first and then ask for the user input ( Input for Operation to perform and Two Operands)
- Based on user input, We perform the arithmetic operation.
- We used the Infinite do while loop to display the Menu. We used while (1) condition to create the Infinite loop
- We also used the Switch statement to match user input with options.
- If the user enters 1, The switch case with 1 will be executed and the program will display the addition of given numbers
- If the user enters an Invalid Option (Like the options which are not present in the above menu). The default case of the Switch statement will be executed. Which displays the error message Invalid Option, Please select the valid Options on the console.
- If the user selects the Option 6, Then the if statement will be executed as the if condition if ( in == 6 ) evaluated as True. So We will break out of the loop.
Exercise:
Re-Write the above Menu driven calculator program using the If..else ladder instead of switch statement.
Conclusion:
This is a very long article, To summarise, We have discussed the do while loop in C language with example programs. We also looked at the step-by-step walk-through of the do while loop.
Then we learned about Infinite loops using the do while loop, How to create and avoid Infinite loops.
Finally, we have written a Menu-driven calculator program by using the Switch statement and Infinite do while loop in C programming language.
Hope you enjoyed the article. We will discuss the break and continue statements in our next article.
12 Responses
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] do while loop in Language with example programs […]
[…] have looked at the while loop, for loop, and do while loop in our earlier articles, In today’s article we will learn about the break statement in c language […]
[…] do while loop in Language with example programs […]
[…] If we need to find any element in the array, Then we need to traverse the array using a C loop (for loop, while loop, and do while loop). […]