Function Pointers in C with Example Programs
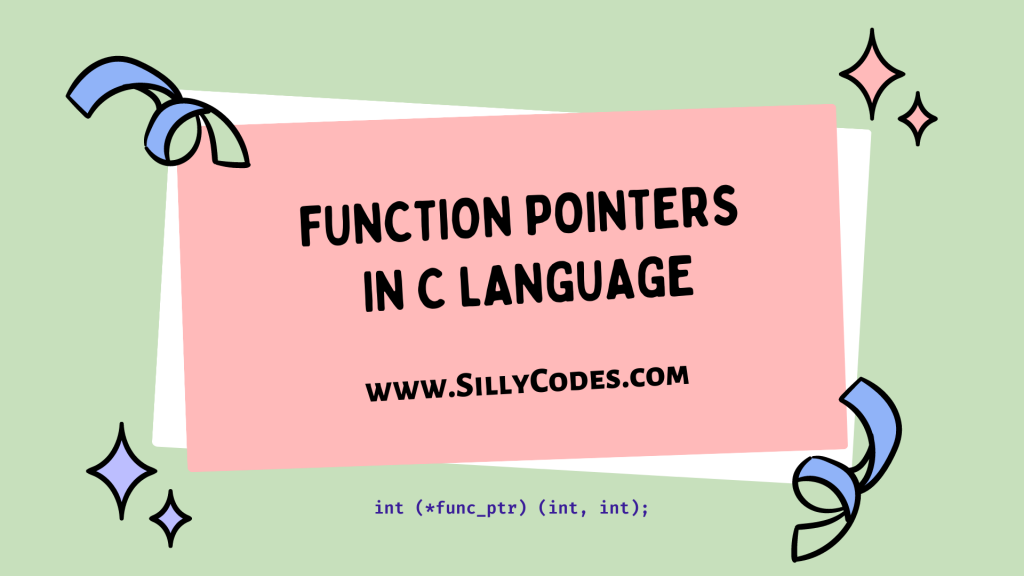
Introduction:
In previous articles, we looked at pointers in C and Double pointers in C with example programs. In this article, we will look at function pointers in c programming language with examples.
📢 Must Check: Pointers Practice Programs series
Function Pointers in C Language:
The function pointers are pointer variables, which are capable to store the memory address of a function.
Function pointers are used to create and use the callbacks and we can also pass a function as an argument to another function using the function pointers in C.
📢 Function pointers store the memory address of a function.
The Memory Address of a Function in C:
As we have discussed earlier in the introduction to pointers variables, Variables are stored in the memory and each memory cell has a memory address. Similarly, Every function will have a memory address.
We can get the memory address of a function by simply specifying the function name (without a function call ( ) ). As the function name also specifies the address of the function.
Let’s look at a program to get the address of a function.
Program to Get the address of a function:
In the following program, we have created a function addition and printed its address on the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/*     Program to understand function addresses in c     sillycodes.com */  #include<stdio.h>  /** * @brief  - Add two numbers and return result */ int addition(int num1, int num2) {     return num1+num2; }  int main() {     // declare two numbers     int num1, num2;      // print addresses of 'num1' and 'num2'     printf("Address of num1:%p and num2:%p\n", &num1, &num2);      // function address     printf("Function 'addition' address : %p\n", addition);      // we can also use address of operator ('&')     printf("Function 'addition' address(using '&' operator): %p\n", &addition);      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 |
$ gcc func-addr.c $ ./a.out Address of num1:0x7ffe02242890 and num2:0x7ffe02242894 Function 'addition' address : 0x5653f1791169 Function 'addition' address(using '&' operator): 0x5653f1791169 $ |
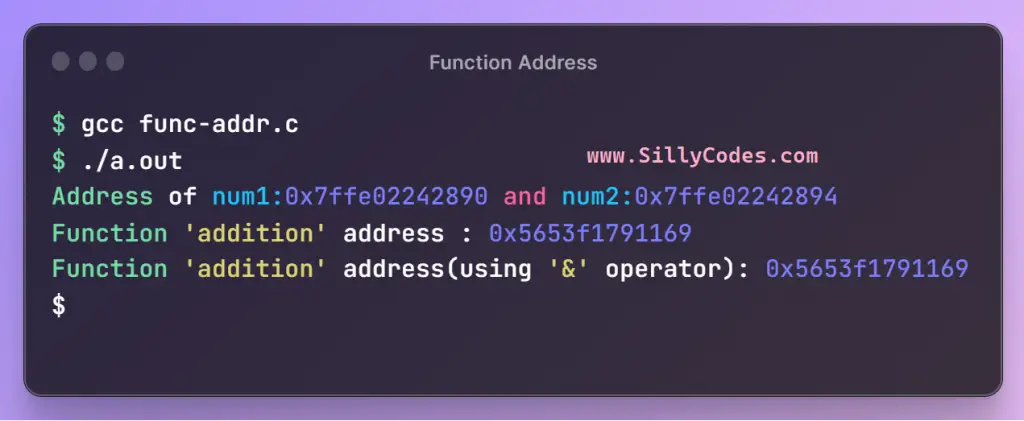
We have defined a function called addition to add two numbers num1 and num2. Then we printed the address( 0x5653f1791169) of the addition function on the console using the function name and address of operator(‘&’).
Now we know how to access the function addresses, let’s look at how to declare a function pointer and assign the function address to the function pointer.
How to Declare Function Pointers in C Language:
We can create a function pointer by function prototype that pointer going to store. Here is the syntax of the function pointer.
Syntax of Function Pointer in C:
1 2 |
// function pointer return_type (*function_name)(argument_list); |
Example of function pointer:
1 2 |
// function pointer example int (*func_ptr) (int, int); |
Here we have declared a function pointer named func_ptr. The func_ptr points to a function with two integer arguments and returns an integer value.
let’s look at another example.
1 |
char (*random_ptr) (char *) |
The random_ptr is a function pointer, that can store the address of a function that returns a character( char) and accepts a character pointer( char *) as an argument.
Assigning functions to a function pointer:
Now that we know how to declare a function pointer, Let’s look at how we can assign a function to a function pointer.
Let’s say we have a function called product which takes two integers as input and returns an integer variable.
1 2 3 4 5 |
// product function definition. int product(int number1, int number2) { Â Â Â Â return number1*number2; } |
Declare a function pointer called product_ptr to hold the address of the product function.
1 2 |
// declare a function pointer int (*product_ptr)(int, int); |
As discussed earlier, The function name also specifies the address of the function, We can simply assign the product function product_ptr function like below.
1 2 |
// Assign 'product' function to 'product_ptr' product_ptr = product; |
Now, The product_ptr function pointer is now storing the address of the product function.
Calling a function using the Function Pointer in C Programming:
We can call the function product using the function pointer product_ptr by dereferencing the function pointer like below.
Syntax to call the function using a function pointer:
1 2 |
// call the function using function pointer. int result = (*product_ptr)(number1, number2); |
Note that we passed the two integer numbers number1 and number2 as the arguments to the function pointer and The product function returns an integer variable and we stored the return value in the result variable.
Let’s combine all the above operations and create a program to declare, assign and use the function pointers in C.
Program to understand the function pointer in C programming language:
The following program demonstrates how to declare a function pointer, assign a function to a function pointer, and finally call a function using a function pointer in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/*     Program to understand function pointer in c programming language     sillycodes.com */  #include<stdio.h>  /** * @brief  - Function calculate the product of given numbers * * @param number1 - number1 * @param number2 - number2 * @return int    - results - product of number1 and number2 */ int product(int number1, int number2) {     return number1*number2; }  int main() {     // declare two numbers     int number1, number2;      // Take two values from the user     printf("Enter two integers: ");     scanf("%d%d", &number1, &number2);      // declare a function pointer     int (*product_ptr)(int, int);      // Assign 'product' function to 'product_ptr'     product_ptr = product;      // call the 'product' function using 'product_ptr' function pointer     int result = (*product_ptr)(number1, number2);      // display the results     printf("Product of %d*%d is : %d\n", number1, number2, result);      return 0; } |
Program Output:
Let’s compile the program using your favorite compiler. We are using the GCC compiler in this example.
$ gcc function-pointer.c
Test Case 1:
The above command generates an executable file called a.out. Run the executable file using the following command.
1 2 3 4 |
$ ./a.out Enter two integers: 20 30 Product of 20*30 is : 600 $ |
As we can see from the above output, The function pointer product_ptr is properly storing the function address and we are able to get the product by dereferencing the function pointer.
Let’s look at another example.
Test Case 2:
1 2 3 4 |
$ ./a.out Enter two integers: 40 50 Product of 40*50 is : 2000 $ |
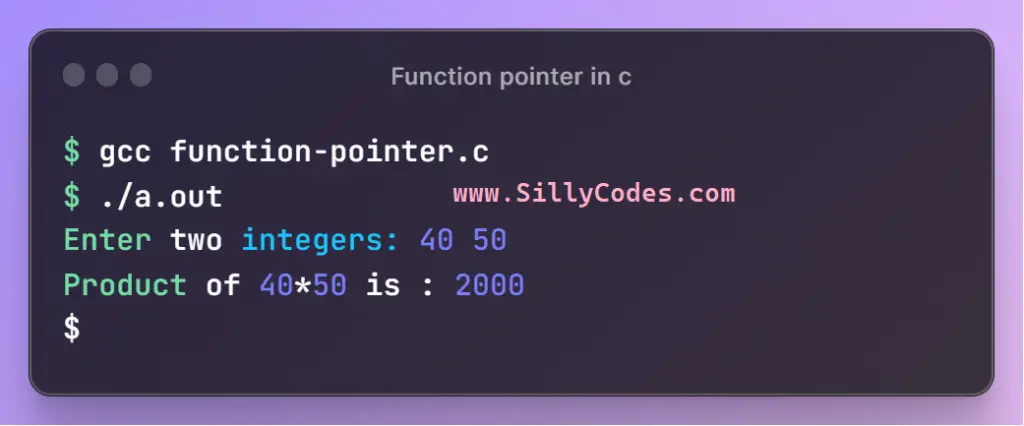
The program is properly calculating the product of the given two integers.
Function pointer vs Function declaration in C:
If you observe the above function pointer declaration, It is very similar to the function forward declaration.
📢 Please learn more about function forward declaration in the following article.
For example, If we have a function called addition with the following function definition
1 2 3 4 5 |
// function definition. int addition(int a, int b) { Â Â Â Â return a+b; } |
We can forward declare the above function using the following function declaration.
1 2 |
// function declaration int addition(int, int); |
Let’s create a function pointer to store the address of the addition function. We can create a function pointer like below.
1 2 |
// function pointer int (*addition_ptr) (int, int); |
As we are creating the pointer variable, we need to specify the asterisk symbol( *) before the pointer name.
The parentheses are also needed otherwise, it will become the function declaration.
Let’s look at why we need parentheses. If you forgot to specify the parentheses, We end up creating a function declaration instead of the function pointer.
1 2 |
// Incorrect function pointer - parentheses missing int * addition_ptr (int, int);Â Â Â Â Â Â // function declaraion. |
In the above example, We haven’t specified the parentheses while creating the function pointer, So it became a function declaration of a function returning an integer pointer ( int *).
📢 The precedence of the parenthesis ( ) is higher than the asterisk *, Please look at the Operators Precedence and Associativity Table to understand the operator precedencies in the c programming language.
An Array of Function pointers in C :
An array of function pointers is an array and each element of the array is a function pointer. When you have a collection of functions that all carry out the same operations and you want to select one of them at runtime based on a criterion, an Array of function pointers can be used.
We can declare an array of function pointers by specifying the size of the array like below.
1 2 |
// array of function pointers int (*func_ptr_arr[5])(int, int); |
Here we have defined an array of function pointers func_ptr_arr with the size of 5 elements. Each element of the array is a function pointer, that returns an integer value and takes two integers as arguments.
We can assign the functions to the array of function pointers like below.
1 2 3 4 5 |
func_ptr_arr[0] = function1; func_ptr_arr[1] = function2; func_ptr_arr[2] = function3; func_ptr_arr[3] = function4; func_ptr_arr[4] = function5; |
Here the function1, function2, function3, function4, and function5 are functions that take two integer values and return an integer value.
Finally, We can call the functions using the array of function pointers by dereferencing the function pointer like below.
1 2 3 4 5 |
// calls the function1 int res = (*func_ptr_arr[0])(44, 63); Â // call the function3 int res = (*func_ptr_arr[2])(33, 1); |
Program to understand the Array of Function Pointers in C Language:
Let’s look at a program to uses the array of function pointers in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 |
/*     Program to understand Array of function pointer in c programming language     sillycodes.com */  #include<stdio.h>  #define SIZE 4  /** * @brief  - Function calculate the product of given numbers * * @param number1 - number1 * @param number2 - number2 * @return int    - result - product of number1 and number2 */ int product(int number1, int number2) {     return number1*number2; }  /** * @brief  - Function calculate the addition of given numbers */ int add(int number1, int number2) {     return number1+number2; }  /** * @brief  - subtract given numbers */ int subtract(int number1, int number2) {     return number1-number2; }  /** * @brief  - Division of given numbers */ int division(int number1, int number2) {     return number1/number2; }  int main() {     // declare two numbers     int number1, number2;      // Take two values from the user     printf("Enter two integers: ");     scanf("%d%d", &number1, &number2);      // declare a function pointer     // array of function pointers     int (*func_ptr_arr[SIZE])(int, int);       // assign the functions to function pointers.        func_ptr_arr[0] = add;     func_ptr_arr[1] = subtract;     func_ptr_arr[2] = product;     func_ptr_arr[3] = division;       // Iterate over the array of function pointers     for(int i=0; i<SIZE; i++)     {         // call the 'function' using 'function pointer'         int res = (*func_ptr_arr[i])(number1, number2 );                 // print result         printf("%d\n", res);     }      return 0; } |
Program Output:
Let’s compile and run the program.
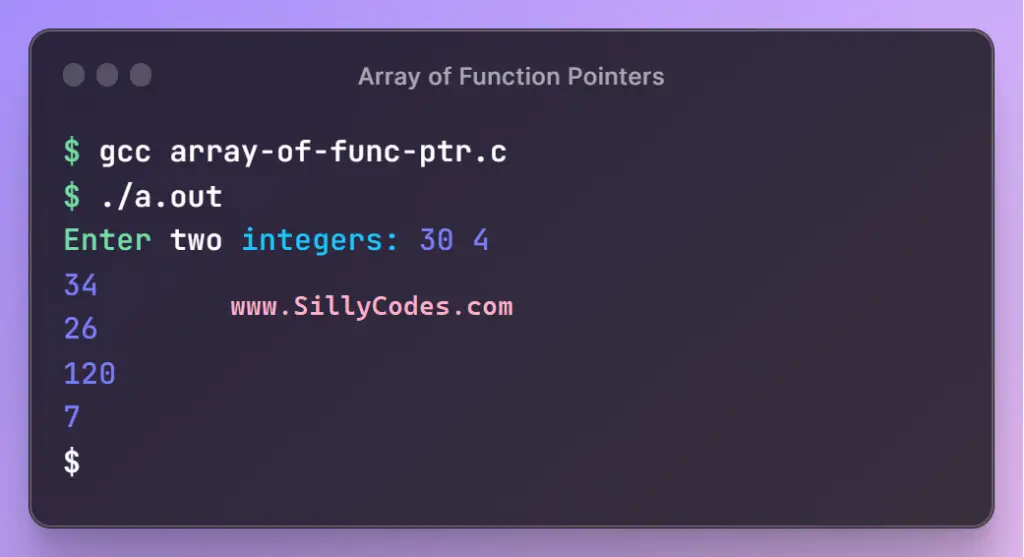
As we can see from the above output, The program performed all arithmetic operations using the array of function pointers.
Function Pointers uses in C Programming:
In C, function pointers are used to create and use callbacks, and we can also use function pointers to pass a function as an argument to another function.
One example of function pointer usage in the c language is with the signal() function in Linux OS.
1 2 3 4 5 6 7 |
// signal man page  #include <signal.h>  typedef void (*sighandler_t)(int);  sighandler_t signal(int signum, sighandler_t handler); |
Here the sighandler_t is a function pointer.
We also use the function pointer in qsort() function to sort arrays, and strings.
1 2 |
void qsort (void* string, size_t num, size_t size, Â Â Â Â Â Â Â Â Â Â Â Â int (*compare)(const void*,const void*)); |
The compare function is a function pointer.
Conclusion:
We have looked at the function pointers in C programming language in detail. We covered how to declare a function pointer, how to assign a function to a function pointer, and finally how to call a function through the function pointer to get the desired results.
Related Articles:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Pointer Arithmetic in C Language
- Pointers and Arrays in C with Example Programs
- Program to Demonstrate Address of Operator (’&’) in C Language
- Program to Understand the Dereference or Indirection Operator in C Language
- Size of Pointer variables in C Language
- Program to Add Two Numbers using Pointers in C
- Program to perform Arithmetic Operations using Pointers in C
- Swap Two Numbers using Pointers in C Language
- Accessing Array elements using pointers in C language
- Print the string elements using pointers in C Language
1 Response
[…] Function Pointers in C with Example Programs […]