Type of Functions in C Programming Language
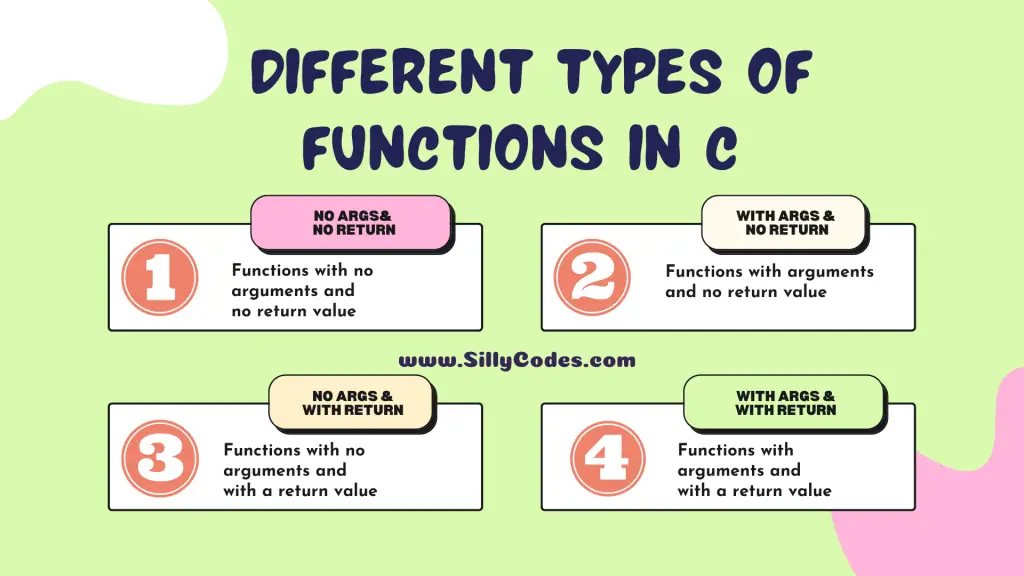
- Type of Functions in C Language Introduction:
- Pre-Requisite:
- Different Types of Functions in C Language:
- Type of Functions in C – Function with no Arguments and no Return value:
- Type of Functions in C – Function with Arguments and no Return value:
- Type of Functions in C – Function with no Arguments and with Return value:
- Type of Functions in C – Function with Arguments and with Return value:
- Which type of function you should use?
- Type of Functions in C Language Conclusion:
Type of Functions in C Language Introduction:
In our previous article, We have looked at the introduction to C functions and how to create and call functions. In this article, We are going to look at the Type of Functions in C programming language.
Pre-Requisite:
We need to know the basics of the functions. So Please go through the following article to learn more
Different Types of Functions in C Language:
- Functions with no arguments and no return value
- Functions with arguments and no return value
- Functions with no arguments and with a return value
- Functions with arguments and with a return value
Let’s look at them one by one.
Type of Functions in C – Function with no Arguments and no Return value:
Here is the syntax of the function without arguments and without return values
1 2 3 4 5 6 7 8 9 |
// Function without arguments and without return value. void function_name(void) {   // Function body   // statements   ......   ......   // statements } |
If you look at the above syntax, The above function ( function_name)
- Doesn’t have any arguments, We specified the argument_list as void. which means the function doesn’t accept any input values.
- Similarly, we specified the return type as void. So the function doesn’t return any value back to the caller.
Let’s look at a couple of examples for the Functions with no arguments and no return value.
Example 1 – Greet Function: Function without Arguments and without Return value:
Here is a program with a greet function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/*     Program: Function without arguments and without return value     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: greet     arguments_list : void     return_value : void */ void greet(void) {     printf("Welcome to SillyCodes \n"); }  /*     main() function */ int main() {     printf("Main Function \n");      // function call     greet();      return 0; } |
Here we have defined a function called greet. The greet function doesn’t take any input values (no arguments) and doesn’t return anything (no return type).
Here are the greet function details,
- function_name: greet
- arguments_list : void
- return_value : void
So To call the greet function, We don’t need to pass any arguments, We can simply call it using greet()
Program Output:
1 2 3 4 5 |
$ gcc function-no-args-no-return.c $ ./a.out Main Function Welcome to SillyCodes $ |
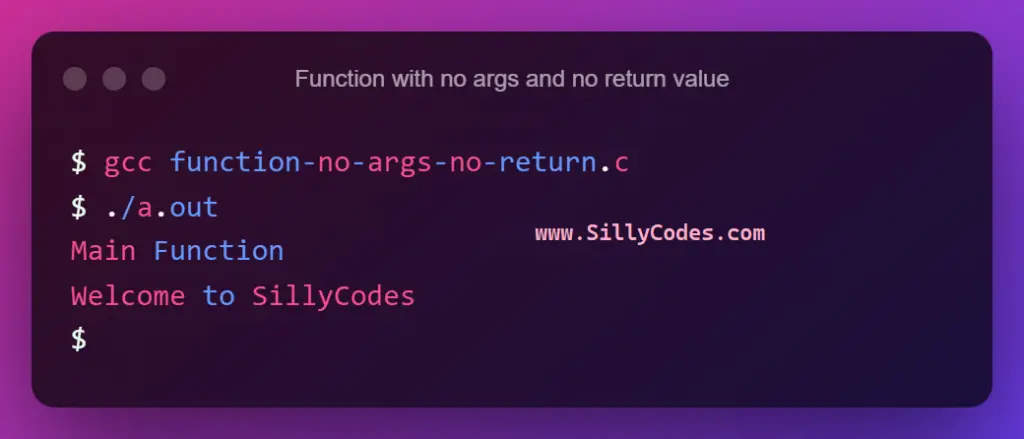
As you can see from the above output, The greet function displayed the message Welcome to SillyCodes and not returned any value.
Example 2: Sum of numbers Function with no Arguments and no Return value:
Let’s look at another program, Where we want to calculate the sum of the first 10 natural numbers and display the result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/*     Program: Function without arguments and without return value example 2     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: sum_of_numbers     arguments_list : void     return_value : void */ void sum_of_numbers(void) {     int i;     int sum = 0;      // calculate the sum of first 10 numbers     for(i=1; i<=10; i++)     {         sum += i;  // sum = sum + i;     }      // print the results     printf("Sum of first 10 natural numbers is %d \n", sum); }  /*     main() function */ int main() {     printf("Main Function \n");      // function call     sum_of_numbers();      return 0; } |
Here we have created a function named sum_of_numbers. This function is also a function with no arguments and no return value. So don’t accept and return anything.
Here are the sum_of_numbers function details
- function_name: sum_of_numbers
- arguments_list : void
- return_value : void
The function sum_of_numbers calculates the sum of natural numbers from 1 to 10 using for loop and displays the results.
📢 We have already covered the program to calculate the Sum of the first N natural number please go through the following program
Program Output:
1 2 3 4 5 |
$ gcc function-no-args-no-return-2.c  $ ./a.out Main Function Sum of first 10 natural numbers is 55 $ |
As you can see from the output, The program calculates the sum of the first 10 numbers.
Type of Functions in C – Function with Arguments and no Return value:
Here is the syntax of the function with arguments and without return values
1 2 3 4 5 6 7 8 9 |
// Function with arguments and without return value. void function_name(datatype1 arg1, datatype2 arg2, ...) {   // Function body   // statements   ......   ......   // statements } |
If you look at the above syntax,
- The function has the
argument_list i.e
(datatype1 arg1, datatype2 arg2, ..), So the function accepts the input values from the caller.
- Make sure to use the same datatypes you specified in the function declaration and function call.
- But the above function doesn’t have a return type, We have specified the return type as void. So the function doesn’t return any value back to the caller.
Example 1: Calculate Sum of Two Numbers using Function with Arguments and without Return value:
Write a C Program to calculate the sum of two numbers and display the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/*     Program: Function with arguments and without return value     Author: Sillycodes.com */  #include<stdio.h> void add(int n1, int n2);  /*     main() function */ int main() {     // Take the input from the user     int num1, num2;         printf("Enter two numbers: ");     scanf("%d%d", &num1, &num2);      // call the 'add' function with 'num1' and 'num2'     add(num1, num2);         return 0; }  /*     function_name: add     arguments_list : (int n1, int n2)     return_value : void */ void add(int n1, int n2) {     printf("sum of %d and %d is %d\n", n1, n2, n1+n2); } |
Here we have defined a function ( add). which calculates and displays the sum of two integer numbers.
The add function is a function with two arguments and no return value. So add function accepts two integers as input from the caller but doesn’t return anything.
Here are the add function prototype details.
- function_name: add
- arguments_list : (int n1, int n2)
- return_value : void
To call the add function, We need to pass two parameters (actual arguments) during the function call. Here is an example of add function call
add( 100, 200);
Here 100 and 200 are the actual arguments, Which are copied to the formal arguments of the add function
📢 Note, As we are calling the add function before defining it, So we have forward-declared the add function at the start of the program. void add(int n1, int n2);
Program Output:
1 2 3 4 5 |
$ gcc function-with-args-no-return-1.c $ ./a.out Enter two numbers: 20 50 sum of 20 and 50 is 70 $ |
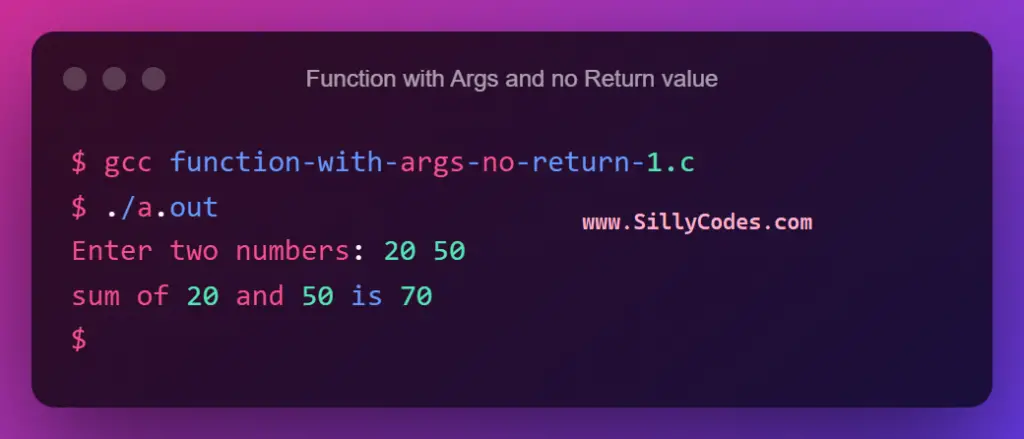
Example 2: Check Prime Number – Function with Arguments and no Return value:
C Program to check if the given number is a prime number.
The program accepts a number from the user and checks if it prime number using the function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
/*     Program: Check Prime - Function with arguments and without return value     Author: Sillycodes.com */  #include<stdio.h> void isPrime(int n);  /*     main() function */ int main() {     // Take the input from the user     int num1;         printf("Enter a positive numbers: ");     scanf("%d", &num1);      // call the 'isPrime' with one integer     isPrime(num1);         return 0; }  /*     function_name: isPrime     arguments_list : (int n)     return_value : void */ void isPrime(int n) {     int i, cnt = 0;     // Iterate through 2 to n/2     for(i=2; i<=n/2; i++)     {         // Check if 'i' is factor of 'n'         if(n%i == 0)         {             // 'i' is factor, Increment 'cnt' variable             // so 'n' is not prime             cnt++;             break;         }     }      // if the cnt is '0'. Then Number(n) is prime number     if(cnt == 0)     {         printf("%d is a Prime Number \n", n);     }     else     {         printf("%d is not a Prime Number \n", n);     } } |
Here we have defined a function ( isPrime). which checks if the given number is a prime number or not.
The isPrime function is a function with a single argument and no return value. So isPrime function accepts an integer from the caller but doesn’t return anything.
Here are the isPrime function prototype details.
- function_name: isPrime
- arguments_list : (int n)
- return_value : void
To call the program, Pass an integer variable like below.
isPrime(20);
or
isPrime(num1);
The isPrime function takes the num1 as input and calculates if the num1 is a prime number or not and displays the results on the console.
📢 We have already covered a few prime numbers checking programs, Please check them here.
Program Output:
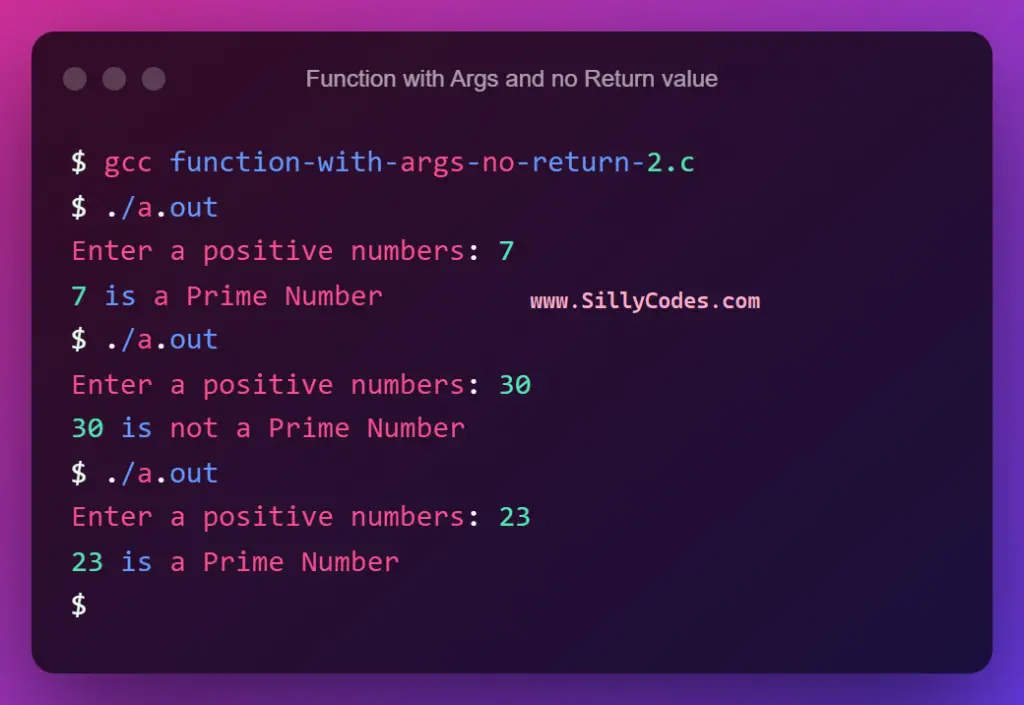
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc function-with-args-no-return-2.c $ ./a.out Enter a positive numbers: 7 7 is a Prime Number $ ./a.out Enter a positive numbers: 30 30 is not a Prime Number $ ./a.out Enter a positive numbers: 23 23 is a Prime Number $ |
Type of Functions in C – Function with no Arguments and with Return value:
Here is the syntax of the function without arguments and with return values
1 2 3 4 5 6 7 8 9 10 |
// Function without arguments and with return value. datatype function_name(void) {   // Function body   // statements   ......   ......   // statements   return <some-value>; } |
If you look at the above syntax,
- The function doesn’t accept any arguments, As we specified the argument_list as void.
- But the function does return a value. The has the return type as datatype. For example int, So it will return an Integer value to the caller.
Let’s look at a few examples of no-argument and return value functions.
Example 1: Calculate the product Numbers – Function with no Arguments and with Return value
Write a C Program Calculate the product of the first 5 (1 to 5) numbers and return the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/*     Program: Function without arguments and with return value     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: prod     arguments_list : void     return_value : int */ int prod(void) {     int i;     int product = 1;      for(i=1; i<= 5; i++)     {         product *= i;     }      // return the 'product'     return product; }  /*     main() function */ int main() {     int result;         // call 'prod' function to get product of numbers 1-5     result = prod();      // print the results     printf("Product of 1-5 numbers is : %d\n", result); } |
Here we have defined a function ( prod). which calculates to the product of numbers from 1 to 5.
The prod function is a function without arguments and with a return value. So prod function doesn’t accept any arguments from the caller but returns an integer back.
Here are the prod function prototype details.
- function_name: prod
- arguments_list : void
- return_value : int
To call the prod function, we use
result = prod();
The prod function calculates the product and returns the results and we are storing the results in result variable.
Program Output:
1 2 3 4 |
$ gcc function-no-args-with-return-1.c $ ./a.out Product of 1-5 numbers is : 120 $ |
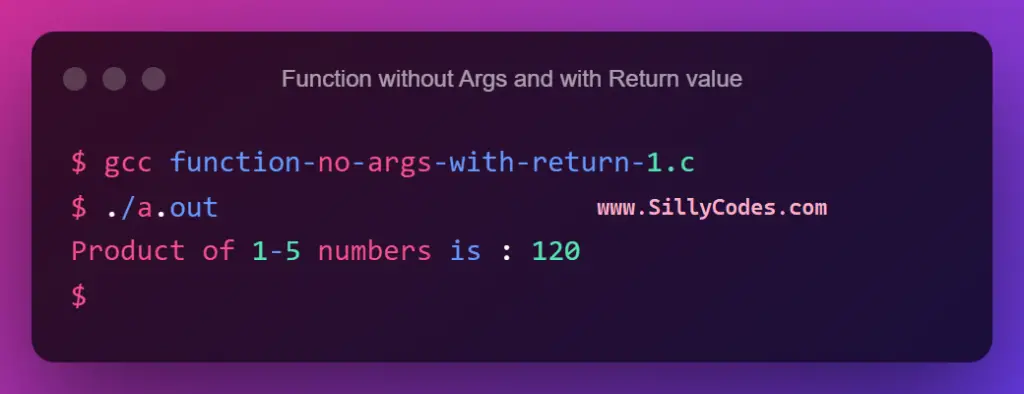
Example 2: Sum of Odd Number – Function with no Arguments and with Return value:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
/*     Program: Function without arguments and with return value     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: sumOfOddNumbers     arguments_list : void     return_value : int */ int sumOfOddNumbers(void) {     int i, oddSum = 0;         for(i=1; i<= 10; i++)     {         // Only add Odd values         if(i%2 == 1)             oddSum += i;     }      // return the 'oddSum'     return oddSum; }  /*     main() function */ int main() {     int res;         // call 'sumOfOddNumbers' function     res = sumOfOddNumbers();      // print the results     printf("Sum of Odd numbers from 1 to 10 is : %d\n", res); } |
In this program, We have created a function called sumOfOddNumber which takes no values but returns an integer, which is the sum of all Odd numbers from number 1 to 10.
Here are the sumOfOddNumbers function prototype details.
- function_name: sumOfOddNumbers
- arguments_list : int
- return_value : void
Program Output:
1 2 3 4 |
$ gcc function-no-args-with-return-2.c $ ./a.out Sum of Odd numbers from 1 to 10 is : 25 $ |
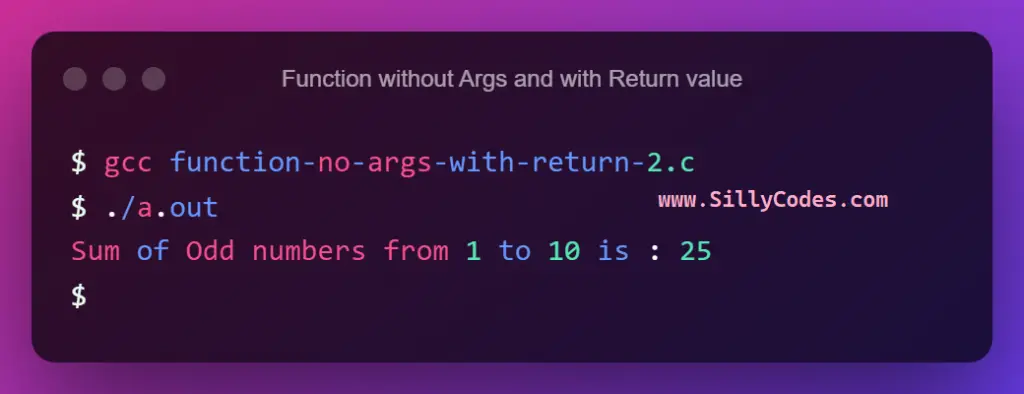
Type of Functions in C – Function with Arguments and with Return value:
Here is the syntax of the function with arguments and with return values
1 2 3 4 5 6 7 8 9 10 |
// Function with arguments and with return value. datatype function_name(datatype1 arg1, datatype2 arg2, ...) {   // Function body   // statements   ......   ......   // statements   return <some-value> } |
If you look at the above syntax,
- The function has the
argument_list i.e
(datatype1 arg1, datatype2 arg2, ..), So the function accepts the input values from the caller.
- Make sure to use the same datatypes you specified in the function declaration and function call.
- The function also returns a value back to the caller. Here we have specified the return data type as
datatype, It can be any valid C data type like
int,
float,etc.
- If you use the int as the return type, Make sure to return the integer value from the function. Similarly, if you use the float datatype, return the float data.
Example 1 – Number of Digits – Function with Arguments and with Return value:
C Program to calculate the number of digits in a given number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/*     Program: Number of Digits in Number using functions             Function with arguments and with return value     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: noOfDigits     arguments_list : int     return_value : int */ int noOfDigits(int num) {     int i;     int digitCnt = 0;      for(i = num; i > 0; i = i/10) {         // increment the number of digits count (digitCnt)         digitCnt++;     }      // return the 'digitCnt'     return digitCnt; }  /*     main() function */ int main() {     int number, res;     printf("Enter a Positive Number: ");     scanf("%d", &number);         // call 'noOfDigits' function     res = noOfDigits(number);      // print the results     printf("Number of digits in %d Number is : %d\n", number, res); } |
Here we have defined a function ( noOfDigits). which calculates the number of digits in a given number.
The noOfDigits function is a function with a single argument and a single return value. So noOfDigits function accepts an integer as input from the caller and returns an Integer back.
Here are the noOfDigits function prototype details.
- function_name: noOfDigits
- arguments_list : (int num)
- return_value : int
Call the function using,
res = noOfDigits(number);
Here we are passing a number (i.e variable number) to the function during the function call, And the function returns an Integer variable, Which will be stored in the res variable.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc function-with-args-with-return-1.c $ ./a.out Enter a Positive Number: 12345 Number of digits in 12345 Number is : 5 $ ./a.out Enter a Positive Number: 485 Number of digits in 485 Number is : 3 $ ./a.out Enter a Positive Number: 1 Number of digits in 1 Number is : 1 $ ./a.out Enter a Positive Number: 45892123 Number of digits in 45892123 Number is : 8 $ |
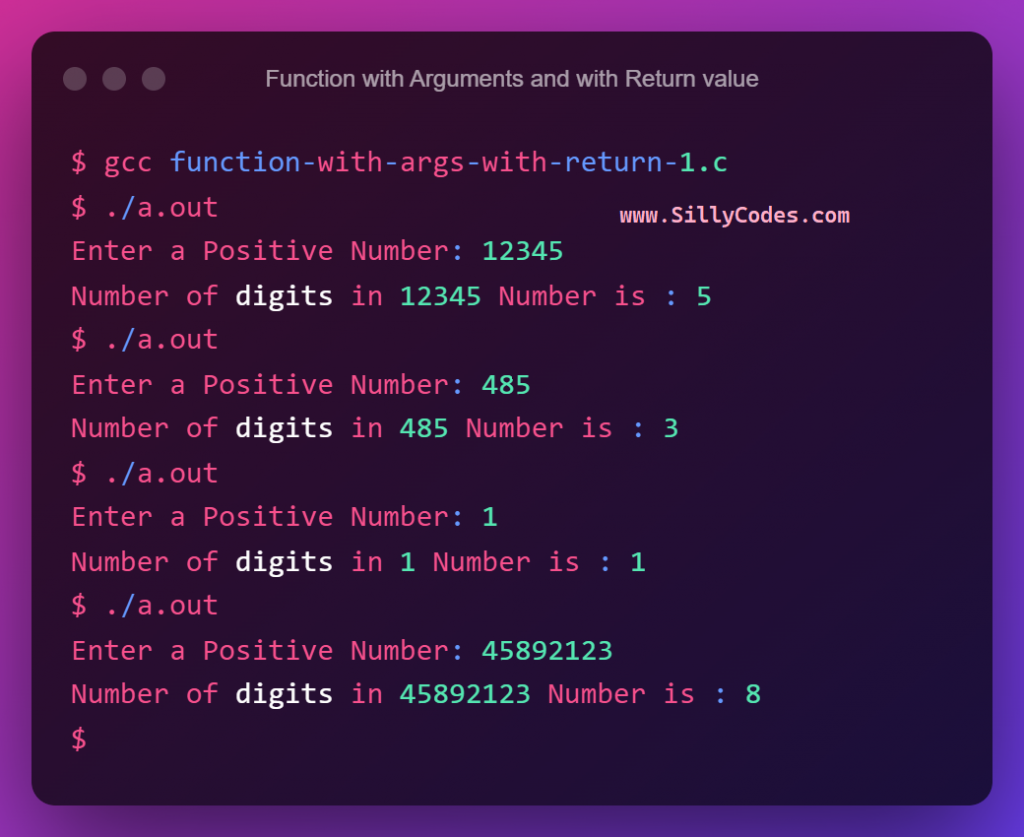
As we can see from the output, The program is calculating the number of digits in the given number and returning back to the main() function, Then we are displaying the results using the printf() function.
Example 2: Factorial of Number – Function with Arguments and With Return value:
Write a C Program to calculate the factorial of a number using functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
/*     Program: Factorial of a Number using functions             Function with arguments and with return value     Author: Sillycodes.com */  #include<stdio.h>  /*     function_name: factorial     arguments_list : int     return_value : int */ int factorial(int n) {     int fact = 1, i;      // Factorial of '0' is '1'     if(n == 0)         fact = 1;     else     {         for(i=1;i<=n;i++)         {             fact = fact*i;         }     }      // return the 'fact'     return fact; }  /*     main() function */ int main() {     int number, result;     printf("Enter a Positive Number: ");     scanf("%d", &number);         // call 'factorial' function     result = factorial(number);      // print the results     printf("Factorial of %d Number is : %d\n", number, result); } |
Here we have defined a function named factorial to calculate the factorial of the given number.
The factorial function takes an integer value and calculates the factorial and returns the value(Integer) back to the main() function.
Here are the factorial function prototype details.
- function_name: factorial
- arguments_list : (int num)
- return_value : int
To call the factorial function, We used
result = factorial(number);
Here the number is the user input and the main() function passed number to the factorial function, Once the factorial function is completed and returns the return value, It will be stored in the result variable.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc function-with-args-with-return-2.c $ ./a.out Enter a Positive Number: 10 Factorial of 10 Number is : 3628800 $ ./a.out Enter a Positive Number: 5 Factorial of 5 Number is : 120 $ ./a.out Enter a Positive Number: 1 Factorial of 1 Number is : 1 $ ./a.out Enter a Positive Number: 7 Factorial of 7 Number is : 5040 $ |
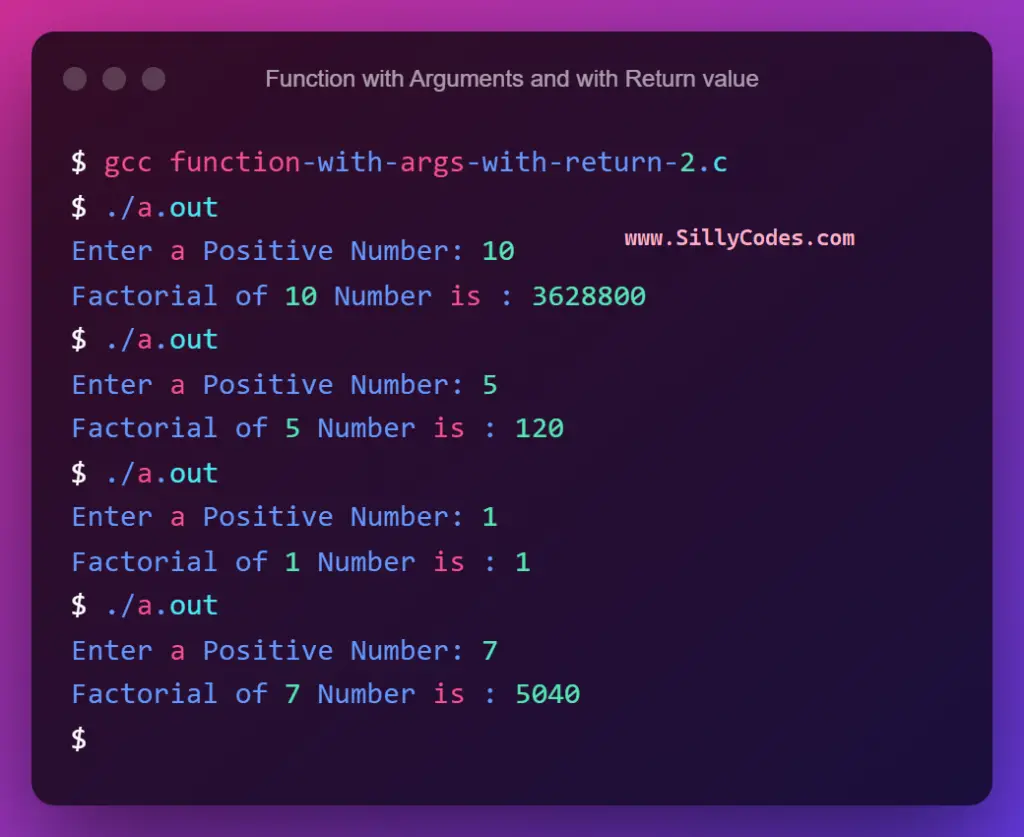
As you can see from the above output, The program is giving appropriate results.
Which type of function you should use?
It depends upon your requirement, If you need to send the data to the function and get the data back from the function, Then use the function with arguments and with the return type. Similarly, You don’t really need a return value but need to send some data to the function, Then use a function with arguments and without return type.
So Which type of function to use purely depends upon your use case.
Type of Functions in C Language Conclusion:
We have discussed about the Type of Functions in C language with example programs. We looked at the variables of functions like The function without arguments and without a return value, The function with arguments and without a return value, The function without arguments and with a return value, and The function with arguments and with a return value.
Related Functions Program:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- Arithmetic Operations using Functions
- Add Two Numbers using Function
- 100+ C Practice Programs
- C Tutorials Index
5 Responses
[…] have looked at the Introduction to C Functions and Types of C functions in our earlier posts. In today’s article, We are going to look at Call by Value and Call by […]
[…] Types of Functions in C […]
[…] Types of Functions in C […]
[…] Types of Functions in C […]
[…] a function pointer called product_ptr to hold the address of the product […]