Bitwise Left Shift Operator in C Language ( << ) with Programs
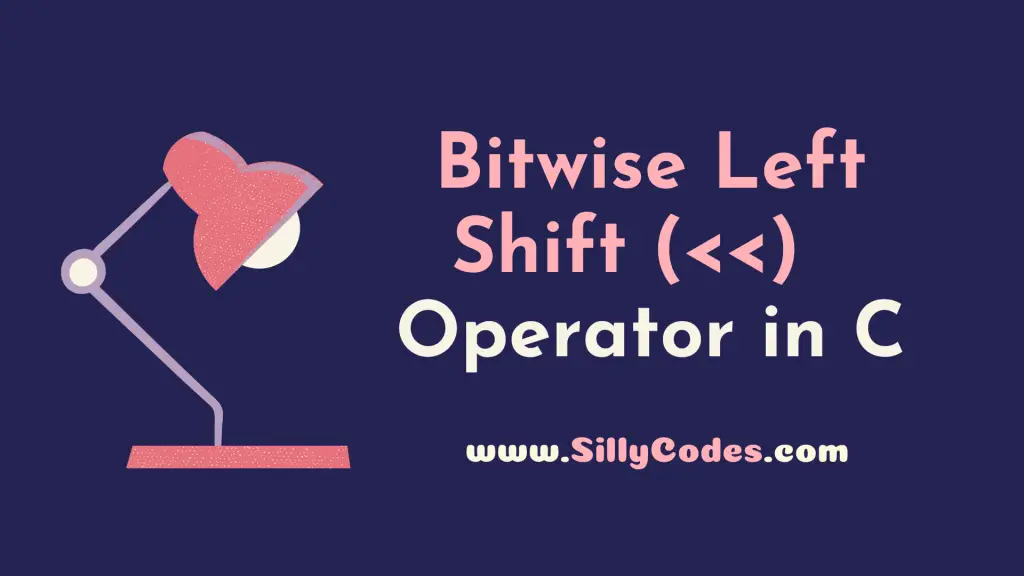
Introduction:
We have looked at the Bitwise XOR Operator and Bitwise Right Shift Operator in our earlier articles, In today’s article, we are going to look at the Bitwise Left Shift Operator in C language with Example Programs.
Bitwise Left Shift Operator in C ( << ):
The Bitwise Left Shift Operator is used to move(shift) the specific number of bits in binary sequence in the Left direction.
Here is the syntax of the Bitwise Right shift operator:
number << num_of_positions_to_shift
Here
- number is the first operand.
- num_of_positions_to_shift is the second operand.
- The << is the Bitwise left shift operator.
The Bitwise Left Shift Operator ( >> ) shifts the number(Binary sequence) Left side by a number of positions given in the second operand ( num_of_position_to_shift).
📢 This program is part of Bitwise Operator in C language series.
Example to Understand the Bitwise Left Shift Operator:
Let’s take an example.
Example 1:
Shift one bit on the left side using the left shift operator.
10 << 1
Here we are trying to left-shift the number 10 by one (1) position. As the bitwise operators work on the bit level, First we need to convert the decimal number 10 into the binary sequence.
The binary equivalent of the number 10 is 00001010
Now we need to shift the above binary sequence on the Left side by one.
00001010 << 1
which is equal to – 00010100 – Decimal Value 20.
We simply moved all bits to the left side by one position. Filling the 0 bit at the right side.
📢 So In Nutshell, the Bitwise Left shift operator is shifting the first operand’s bits left side by the number of positions given in the second operand.
Here is the image explaining the step-by-step movement of the bits
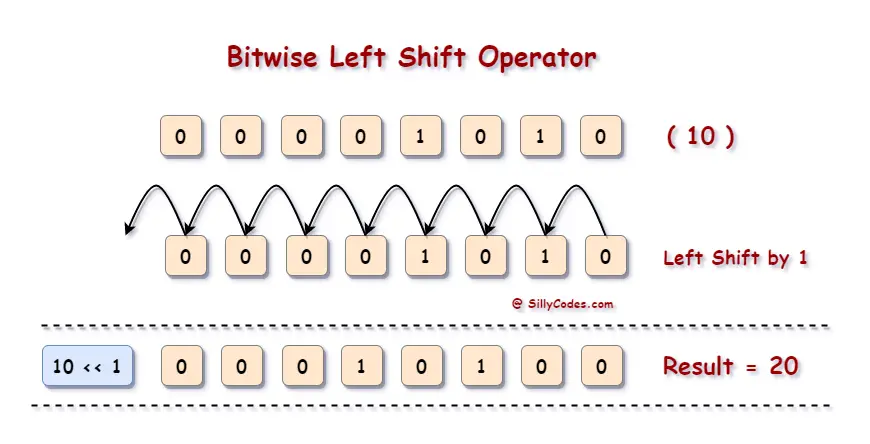
If you notice the final output, All the bits are Left-shifted by one position.
📢 To make our calculations easy, We are representing the integer numbers with 8 bits.
Let’s look at another example.
Example 2:
Let’s shift the two bits left side by using the bitwise left shift operator.
12 << 2
So above expression, Left Shifts the binary sequence of the number 12 by two positions.
The binary Equivalent of 12 is 00001100
Let’s shift two bits of the above sequence to the Left side.
00001100 << 2
Which is equal to 00110000 – Decimal value 48.
If you observe carefully, All the bits are moved Left side by two times. And the rightmost bits are filled with zeros.
Let’s look at the graphical representation of the above bitwise Left shift operation. ( 12 << 2)
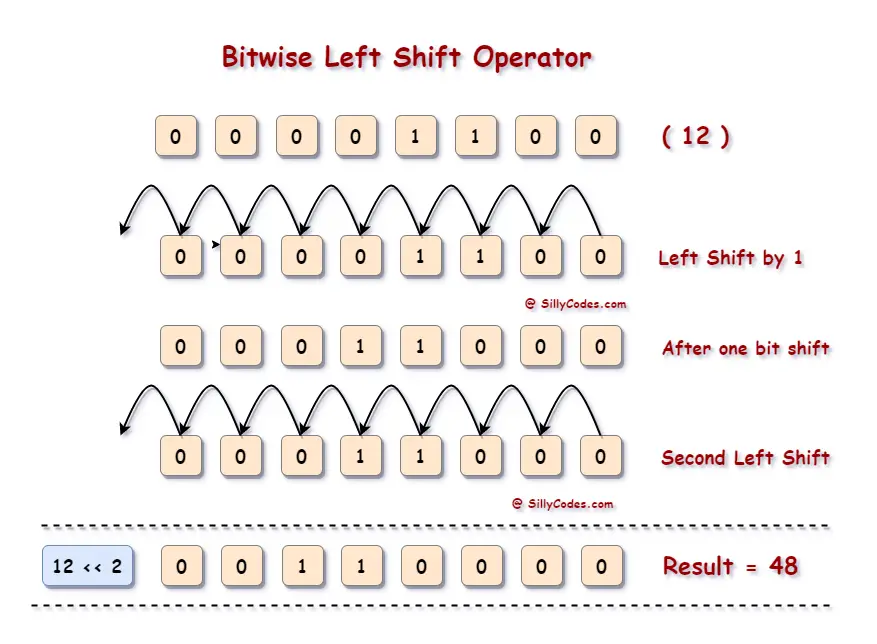
Uses of Bitwise Left Shift Operator ( << ):
If you observe the above two examples, Whenever we Left-shift the number by one bit, The Original number is increased and becomes exactly double (as we shifted only one position).
In the first example, The 10 << 1, became 20, which is 10*2 = 20.
In the second example, The 12 << 2, which became 48, which is equal to 12 * 22 = 12 * 4 = 48.
Similarly, If you shift 20 << 2, Which will be equal to 80. (20*22 = 20 * 4 = 80)
📢 So whenever we shift the number, ‘n’ bits(positions) on the Left side, The Original number increased by number * (2n)
So whenever you want to increase a number in (2n) terms, You can use the bitwise Left shift operator. As these operations are performed at bit-level, These operations are performed fast in C language.
We can also use Bitwise operators to SET a Bit, CLEAR a bit, and Invert a Bit. We are going to look at these examples in our upcoming posts.
Program to Understand the Bitwise Left shift operator in C language:
Let’s write a program to see how we can use the bitwise Left shift operator in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* Program to understand bitwise Left shift operator in C */ #include<stdio.h> int main() { // Left shift the number 512 by 1 printf("512<<1 is equal to %d \n", 512 << 1); // Left shift 64 by two positions printf("64<<2 is equal to %d \n", 64 << 2); // Left shifting 30 by 4 bits printf("30<<4 is equal to %d \n", 30 << 4); // Left shift 2 by 10 bits printf("2<<10 is equal to %d \n", 2 << 10); return 0; } |
Program Output:
We are going to use the GCC compiler to compile and run the program. Learn more about C Program compilation in Linux using GCC
1 2 3 4 5 6 7 |
$ gcc left-shift.c $ ./a.out 512<<1 is equal to 1024 64<<2 is equal to 256 30<<4 is equal to 480 2<<10 is equal to 2048 $ |
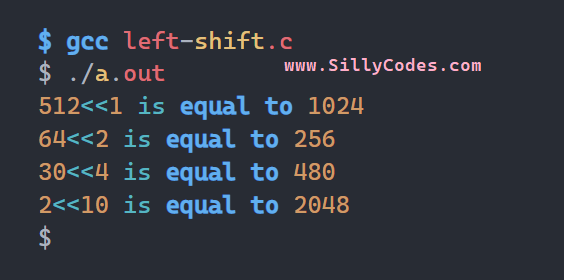
Bitwise Left shift Program in C Explanation:
We have performed four Bitwise Left shift operations in the above program. Let’s look at them one by one.
512 << 1:
The first Left shift operation is 512 << 1.
As we discussed earlier, Whenever we Left -shift the bits of any number by one position. The number becomes double (number * 21 ). So here also the number 512 became double, which is 1024.
Here is the step-by-step calculation.
512 << 1
512 * 2^1
512 * 2
1024
64 << 2:
As we are Left shifting the 64 number by 2 positions, The number will be increased by four times of the original number (22) which is equal to 256.
Here is the step-by-step calculation
64 << 2
64 * 2^2
64 * 4
256
30 << 4:
As we are Left shifting the number 30 by 4 positions, The number will be increased by 16 times of the original number (24) which is equal to 480.
Here is the step-by-step calculation
30 << 4
30 * 2^4
30 * 16
480
2 << 10:
In the last expression, We moved the number 2 by 10 times in the Left side direction. So the number 2 will be increased by 210 times.
2 << 10
2 * 2^10
2 * 1024
2048
Conclusion:
In this article, We have looked at the bitwise Left shift operator, We also explained how the bitwise lift shift operator works with example programs.
2 Responses
[…] Bitwise Left shift Operator ( << ) […]
[…] Bitwise Left Shift Operator ( << ) […]