Standard Input (stdin), Output (stdout), and Error (stderr) Streams
- Standard Input (stdin), Output (stdout), and Error (stderr) Streams Overview:
- Standard Input or stdin stream:
- scanf function in C Language:
- Example to understand scanf function in C language:
- The getchar function in C:
- Program to understand the getchar function:
- gets function in C Language:
- Program to understand the gets function in C:
- Standard Output or Stdout:
- printf function in C language:
- printf function without format specifiers:
- printf with multiple format specifiers:
- putchar function in C:
- Program to understand the putchar function:
- puts function in C Programming:
- Program to understand the puts function:
- Standard Error or StdErr:
- Example program on stderr stream:
- Standard Input (stdin) Output (stdout) and Error (stderr) Conclusion:
- Tutorials Index:
- Related Articles:
- C Programs:
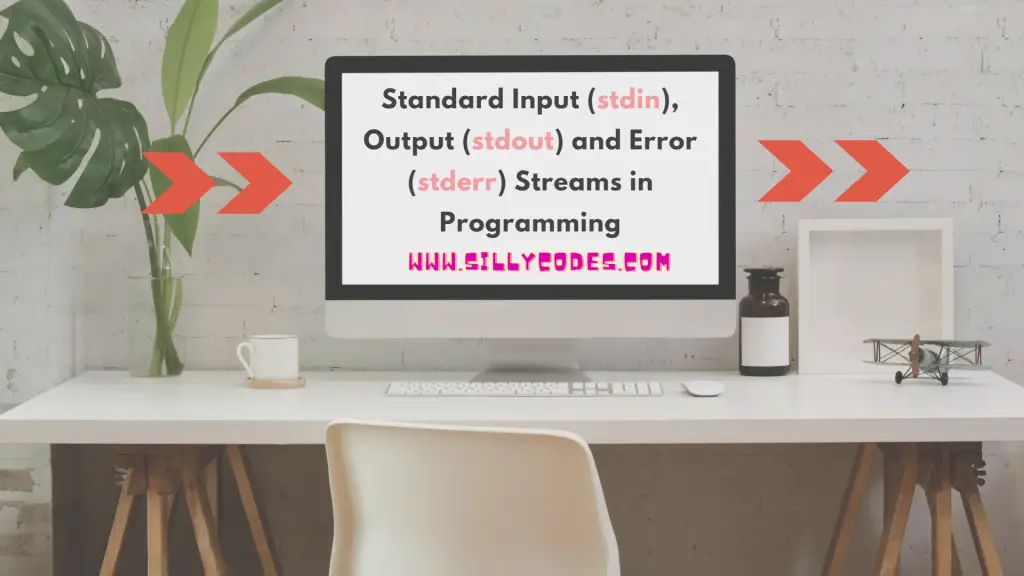
Standard Input (stdin), Output (stdout), and Error (stderr) Streams Overview:
So far we have looked at the basic building blocks of the C language and We also looked at the different Operators in C. In this article, We are going to look into the Standard Input (stdin) Output (stdout) and Error (stderr) Streams in C Programming Language.
In UNIX-like systems such as Linux, Everything is a file. So the input stream is also a file. This will be associated with a file descriptor.
A file descriptor is a number, which is associated with an open file.
Every program which is started will be associated with three open files ( Three streams).
- Standard Input ( stdin) – File descriptor value Zero (0)
- Standard Output ( stdout) – File descriptor value 1
- Standard Error ( stderr)- File descriptor value 2
Standard Input or stdin stream:
The standard input is input stream, Which is used to provide the input to your programs.
Programs get the standard input from the input devices like Keyboard. ( There are other options like using the < input redirection operator in Linux)
In the C programming language, We have many built-in library functions to get the input from the user. Here are a few of them.
- scanf
- getchar
- gets
scanf function in C Language:
The scanf function is used to read the input from the user. scanf function reads the input from the standard input stream and formats it as specified in the format string.
The scanf function is available as part of the stdio.h header file.
syntax:
1 |
int scanf(const char *formatString, ...); |
The scanffunction takes the variable number parameters as input.
We can also represent the above syntax as:
1 |
int scanf(const char *formatString, arg1, arg2, arg3, ...); |
The first argument of the scanf function is a format string, Which contains the desired format specifiers, If we want to read one Integer from the standard input (stdin) then we need to use the %d format specifier.
The arguments after the formatString are the variables, The data read from the stdin will be stored in these arguments. We need to specify the arguments as per the format string. If we want to read only one Integer from the stdin then format string will be %d and the following argument will be one variable
📢 The scanf function returns the number of items read.
Here is the scanf function example, Here we are reading an Integer from the standard input (stdin) and storing the value into the variable a
1 2 3 |
int a; // To store the value in the 'stdin' and store the value to the variable 'a' scanf("%d", &a); |
A couple of things to Note:
- We specified the formatString as %d ( Because we are expecting an Integer value)
- The argument &a contains the & ( address-of ). We need to pass the address to the scanf
We need to use the valid formatSpecifier in the formatString of the scanf function.
- %d for the Integer Values.
- %f for the Float values.
- %c for the Character data.
- %s for the string data, etc
📢 Here is the complete list of the format specifier in the C language https://sillycodes.com/format-specifiers-in-c-format/
Example to understand scanf function in C language:
Let’s look at an example. We want to write a program that takes two input numbers from the user and calculates the sum of given numbers.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
#include<stdio.h> int main() { int a, b; // Take two input numbers from the user printf("Enter two numbers: "); // Blocks the program to get input from user scanf("%d%d", &a, &b); // Add 'a' and 'b' int sum = a + b; printf("Sum of %d and %d is %d \n", a, b, sum); return 0; } |
Output:
We used gcc compiler to compile the code, learn more about compiling the c program at – https://sillycodes.com/compiling-c-program-in-linux-or-unix/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc ./invert-bit.c $ ./a.out Before Inverting the bit : 10 After Inverting the bit : 14 $ $ ./a.out Enter two numbers: 200 300 Sum of 200 and 300 is 500 $ $ ./a.out Enter two numbers: 7 9 Sum of 7 and 9 is 16 $ $ ./a.out Enter two numbers: 99 11 Sum of 99 and 11 is 110 $ |
There are other variants of the scanffunctions like fscanf, sscanf, etc which are used to read the data from the file and strings respectively.
The getchar function in C:
The getcharfunction is used to read a character from the standard input( stdin)
Syntax:
1 |
int getchar() |
The getchar function won’t take any parameters. It reads a character as an unsigned char and return it as int , In case of error it returns the EOF
Program to understand the getchar function:
In the following program, We are going to read a character from the stdin using the getchar function.
Getchar Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#include<stdio.h> int main() { // User input printf("Enter a Character: "); // Read provided character from 'stdin' and // store it into the character variable 'ch' char ch = getchar(); // Display the 'ch' printf("You Entered: %c \n", ch); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc ./getchar-prog.c $ ./a.out Enter a Character: V You Entered: V $ $ ./a.out Enter a Character: s You Entered: s $ ./a.out Enter a Character: 9 You Entered: 9 $ |
As you can see the getcharfunction read a character from the stdinand stored in to the Character variable ch
📢 The getchar in-built function is useful to read a single character from the standard input
We also have one more function called getcwhich is similar to the getchar
gets function in C Language:
The gets function is useful to read a line from the standard input. The reading stops if the new line or EOF is encountered.
📢 getscan read multiple strings. The space won’t stop the gets reading, Only the Newline or EOF stops.
Syntax:
1 |
char * gets(char *str) |
The gets function takes a buffer as an argument(Here str is the buffer) and the Data read from the standard input is stored in the provided buffer( str)
📢 The gets won’t do any Boundary-checks for the provided buffer.
So we need to be careful how much data we are reading from the stdin and Make sure to have enough capacity in the buffer
Program to understand the gets function in C:
Write a program to read a line from the stdin using the gets function.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include<stdio.h> int main() { // Define the buffer to store the data. char str[100]; printf("Enter some data: "); // 'gets' reads the data from the 'stdin' and // stores it in the variable 'str' gets(str); // Print the entered data out on the screen printf("You Entered: %s \n", str); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$gcc ./gets-prog.c $./a.out Enter some data: Sillycodes You Entered: Sillycodes $ $./a.out Enter some data: Lewis Hamilton You Entered: Lewis Hamilton $ $./a.out Enter some data: This is long string, Let's see You Entered: This is long string, Let's see $ $./a.out Enter some data: ANother Example You Entered: ANother Example $ |
As we can see, We can read multiple strings using the getsfunction.
Standard Output or Stdout:
The standard Output stream is used to display the data from the computer to the Output devices such as Consoles.
In the C programming language, We have a few built-in library functions to get the output data from the program. Here are a few of them.
- printf
- putchar
- puts
printf function in C language:
The printf function is used to display the formatted output on the output devices(Console).
printf function reads the data from the standard output stream and formats it as specified in the format string.
The printf function is available as part of the stdio.h header file.
Syntax:
1 |
int printf(const char *formatString, ...); |
The print function takes the variable number parameters as input.
We can also represent above syntax as:
1 |
int printf(const char *formatString, arg1, arg2, arg3, ...); |
The first argument of the printf function is format string, Which contains the string with desired format specifiers.
The arguments after the formatString are the variables, The values of these arguments will be replaced in the formatString to create the final Output string.
📢 The printf function returns the number of characters written on to the console.
Here is an printf function example, Here we are printing the value of the Interger num on to the Console using the printf function.
1 2 3 4 |
int num = 10; // This will create the string 'The Given Number is 10 " printf("The given Number is %d \n", num); |
The formatString is an character string, Which if needed can contains the required format specifiers to get the desired formatted output. In the above example, The value of num i.e 10 is replaced in the formatString
We need to use the valid formatSpecifier in the formatString
- %d for the Integer Values.
- %f for the Float values.
- %c for the Character data.
- %s for the string data, etc
There are other format specifiers like %p, %e, etc we can use in the printffunction.
Lets look at the different types of printf functions
printf function without format specifiers:
If the formatString doesn’t contain any format specifiers then it will be printed as is on to the console.
1 2 |
printf("Hello World"); // "Hello World" |
This is simple printf function, We don’t have any format specifiers inside the formatString So whatever present in the formatString will be written onto the console.
In the above example, The string Hello World will be printed on the output device (As we don’t have any format specifiers).
printf with multiple format specifiers:
The printffunction can have multiple format specifier. Which helps us to format the output string.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 |
#include<stdio.h> int main() { char name[32] = "Alice"; int age = 24; float weight = 55.6; // 'printf' with mutliple format specifiers printf("Name is: %s, Age: %d, and weight: %f \n", name, age, weight); return 0; } |
Output:
1 2 3 4 5 6 |
$ gcc ./printf-prog.c // Output $ ./a.out Name is: Alice, Age: 24, and weight: 55.599998 $ |
In the above program, We have multiple format specifiers like %s, %d, and %f The first format specifier %s is replaced by the variable name, and second one %d is replaced by integer variable age, and The thrid format specifier %f is replaced by the variable weight
putchar function in C:
The putcharfunction is useful to display a character on to the output device.
Syntax:
1 |
int putchar(int ch); |
The putchar function takes a argument ch and writes it out. The argument is written as the unsigned char
On the success, putchar returns the ch as the int . On error it will return EOF
Program to understand the putchar function:
Write a program to display a character variable onto the console using the putchar function.
Example Program:
1 2 3 4 5 6 7 8 9 10 |
#include<stdio.h> int main() { // Take a Character char ch = 'V'; // display the value of 'ch' on console putchar(ch); return 0; } |
Output:
1 2 3 4 |
$ gcc ./putchar-prog.c $ ./a.out V $ |
puts function in C Programming:
The puts function is used to print a line or string on the console
📢 The puts can read multiple strings. The space won’t stop the puts
Syntax:
1 |
int puts(const char *buf) |
The puts function takes a character buffer as an argument (Here buf is the buffer) and prints the characters of buf on to the output device.
The puts() function returns an integer value, Which is the number of characters written to the console.
📢 The puts function adds a Trailing newline to the output.
Program to understand the puts function:
Use the puts function to display a sentence on the console.
Program:
1 2 3 4 5 6 7 8 9 10 11 |
#include<stdio.h> int main() { // Define the buffer char buf[32] = "Learn Programming"; // Print the 'buf' on the console using the 'puts' puts(buf); return 0; } |
Output:
1 2 3 4 |
$ gcc ./puts-prog.c $ ./a.out Learn Programming $ |
Standard Error or StdErr:
The stderr or standard error stream is the default stream for the error messages. Which used to display the error messages.
Similar to the standard output stream( stdout) , The stderr messages are by default displayed on the output devices(Console)
This two streams stdout and stderr are useful to differentiate between the normal logs and errors.
In the real world, The standard output stdout messages are redirected to a file. The Standard error stderr messages, which are kind of fatal messages So are displayed on to console.
We can send the error messages to Standard error stream by using the fprintf function. We need to specify the stderr as the first argument. The fprintf expects a FILE * file pointer as a first argument.
Example program on stderr stream:
In the following we are going to use the fprintf along with the standard error stream file pointer stderr , So we will pass stderr as first argument and the data in the second argument character string ( formatString) is going to be written into the stderr
1 2 3 4 5 6 |
#include <stdio.h> int main() { fprintf(stderr, "Error - Something went wrong!!\n"); return 0; } |
Output:
1 2 3 4 |
$ gcc ./stderr-ex.c $ ./a.out Error - Something went wrong!! $ |
By default, the standard error stderris displayed on to the output console.
Standard Input (stdin) Output (stdout) and Error (stderr) Conclusion:
We have looked at the Standard Input (stdin) Output (stdout) and Error (stderr) streams in the C programming language. The Functions like printf puts are acts as the standard output functions similarly The functions like scanf gets are used for standard input.
37 Responses
[…] Standard Input (stdin), Output (stdout), and Error (stderr) Streams […]
[…] Print the result on the console using printf function. […]
[…] 📢 The printf() and scanf() functions are used for standard input and standard output operations. […]
[…] Print the results on the console using the printf function. […]
[…] Print the result on the standard output i.e console. […]
[…] Display the results on the console using printf function. […]
[…] the numbers array. Use a For Loop to iterate over the array and take the input from the user using standard input and output functions ( printf and scanf) and update the array […]
[…] Use a For loop to Iterate through the array and update the array elements using the scanf function. […]
[…] Define another function called display() to print the array elements on the standard output. […]
[…] Use a For Loop to iterate over the array and update the numbers[i] with the scanf function […]
[…] Use a For Loop to iterate over the array from to size-1 and accept the user input and update the &number[i] element using scanf function. […]
[…] The display() function is used to print the array elements on the Standard Output […]
[…] The display() function is used to print the array elements on the Standard Output […]
[…] Display the Results( Maxtrix-Z) using loops and printf function. […]
[…] Similar to scanf we can use the printf function with %s format specifier to print the strings on the standard Output […]
[…] Finally, We printed the original string ( src) and Destination string ( dest) on the console. […]
[…] If the subStr is not found in the mainStr, Then the return value res will contain the NULL. So display the “NOT FOUND” message on the console […]
[…] the user to provide the string for str and read it using the gets function. (The gets function won’t check the boundaries, so use it with […]
[…] Read the two strings from the user and update str1 and str2 respectively. […]
[…] Display the results on the console. […]
[…] the user to input a string and store it in the inputStr string. We are using the gets function. use it with caution as it won’t check […]
[…] the user to provide the input string and read it using the gets function and update the inputStr string with the given […]
[…] the user for the input string and read it using the gets function and update the inputStr […]
[…] the input string from the user using the gets function and store it in the inputStr […]
[…] the input string from the user using the gets function and store it in the inputStr […]
[…] string and character to search from the user and update the inputStr and ch variables. We use the gets function to read the […]
[…] Prompt the user to provide the input string( inputStr) and Read it using the gets function. […]
[…] character to search from the user and update the inputStr and ch variables respectively. We use the gets function to read the […]
[…] character to search from the user and update the inputStr and ch variables respectively. We use the gets function to read the […]
[…] the input string from the user and update the str string. we are using gets() function to read the […]
[…] two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the […]
[…] two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the […]
[…] two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the […]
[…] the two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings. The gets() function doesn’t do the boundary checks so be cautious while […]
[…] Take the two integer values from the user and store them in the variables num1 and num2 using scanf function.i.e use the address of […]
[…] user to provide the input string and update the inputStr character array/string. We are using the gets function to read the string from the […]
[…] for loop and store the values in the allocated memory. We then printed the integer variable on the console using another for […]