Command line arguments in C programming with examples
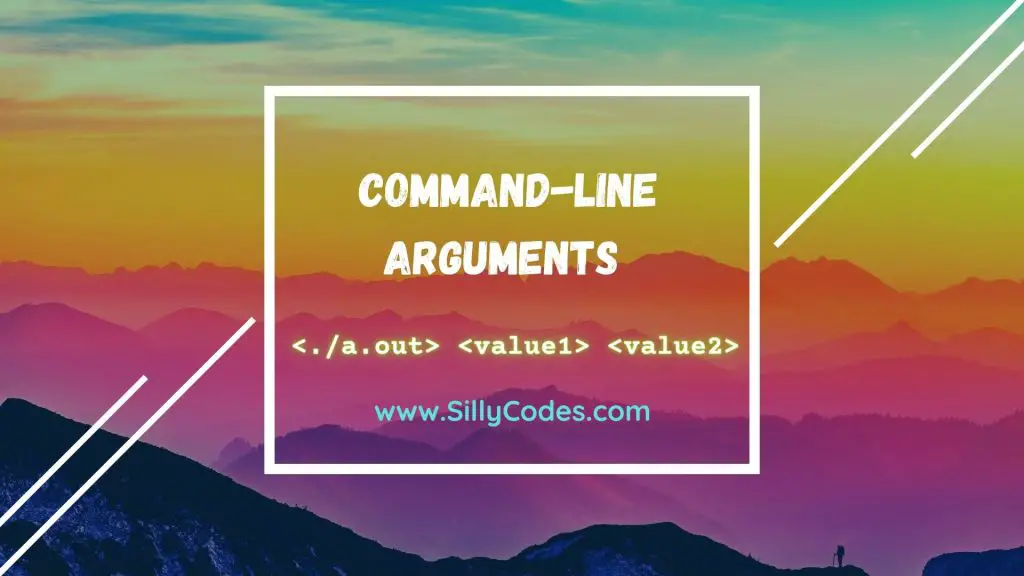
Introduction:
The Command line arguments are one of the ways to pass input to programs. In this article, We are going to discuss different ways to provide input to the program and We will learn what are command-line arguments and how to use them to provide input to the program. We will also look into examples of command line arguments to make understanding better.
Examples use of command line arguments:
We will pass two arguments at the command line along with the executable file.
Input:
1 |
$ ./a.out  10 20 |
output:
1 |
30 |
Note: Here ./a.outis our executable file. I am presently using linux so ./a.outis the executable file extension in linux. If you are in Windows OS, you are executable file will have .exe file extension.
Here is an another example
Input:
1 |
$ ./a.out  90 10 |
Output:
1 |
100 |
Command Line Arguments in C :
Before going to discuss about the command line argument. Let’s see how program gets the input from the user.
Generally, we use the scanf function to take the data or input from the user. Here is an example program to get data from user using the scanf function.
The scanf function to read the data from user:
1 2 3 4 5 6 7 8 9 10 11 12 |
#include <stdio.h> int main() {         int a, b;          printf("Enter values for a and b : ");         // Read user input, Save first value to variable 'a' and second value to variable 'b'         scanf("%d%d", &a, &b);          // Lets print the values of a and b varaible to console using printf         printf("Value of a:%d \t value of b:%d \n", a, b);         return 0; } |
In the above program, we asked user to provide two values. Once the user provides input, We are going to use scanf function to store the user input to variables a and b. The first value will be stored in variable 'a'and the second value will be stored in the variable 'b'.
Then we printed the stored values of variable 'a' and variable 'b'.
Output:
1 2 3 4 5 |
$ gcc scanf-test.c $ ./a.out Enter values for a and b : 10 20 Value of a:10Â Â Â Â value of b:20 $ |
1 |
In the above program we used scanf to read the data from the user and printf to display the output on the console. |
But scanf function is the not only way to provide the input to the program. We can also use the Command line arguments to pass the input to program.
Command line arguments to provide input to program
When we compile our code using GCC or any other compiler, We get the executable file that is a.out (In the Linux and Mac). And we are going to run this executable file using by specifying its path at the command line like below.
1 |
./a.out |
So here we are manually executing the program through the command line. The C language allows us to pass extra parameters along with the executable file while running/executing the program(executable file). These extra parameters are called command line arguments.
For example,
1 |
./a.out 10 20 |
In above example, ./a.out is our executable file and parameters 10 and 20 are command line arguments. The operating system will pass these parameters 10 and 20 to program while executing. So They are available for us to access from the code.
These variable 10 and 20 are stored in aspecial array called argument vector or "argv" and we also have one more variable called argument count or "argc". And argc contains the number of elements passed through the command line.
Note: The argc value contains the total number of parameters passed through the command line, argc value also includes the executable file name as well, In the above example, argc value will be 3 i.e ./a.out 10 20
Example Program: Add two integers given at command line:
Here is an example to add two integers which are passed at the command line.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include<stdio.h> #include <stdlib.h> int main(int argc, char *argv[]) {      //  Program requires two integer values along with the executable file.     // So check if the argc value is equal to 3.     // If argument counter is not equal to 3 then display the correct usage info. and return.     if(argc!=3)     {         printf("use <executable> <int1> <int2> \n");         return 0;     }      int a,b,c;      // The argv[1] and argv[2] are strings,     // So we need to convert them into the integers before Performing  the addition operation.     // We are going to use atoi() function ASCII to Integer conversion     // To use the atoi(), we need to include the stdlib.h headerfile.     a=atoi(argv[1]);     b=atoi(argv[2]);      // Now variable 'a' and 'b' are integers and we are good to perform addition.     c=a+b;      // Display the result.     printf("Sum of %d and %d is : %d\n", a, b, c);     return 0;  } |
In the above program, We are taking two integers from the user. The user need to pass those two integers at the command line while running the program.
Above program need three arguments, They are
1 |
<executable> <int1> <int2> |
So User needs to provide the executable file along with two integers to calculate the sum. If the user input is not matched with the expected input, We are going to display the error message with usage policy by specifying how to use the executable file. Like
1 |
printf("use <executable> <int1> <int2> \n"); |
If the user provides the executable with the two integer numbers, Then our argc check will pass and program continues.
Our command-line arguments are stored in the argv array. The First integer is stored at argv[1] and second integer stored at argv[2]
The file name in our case ./a.out is stored in the argv[0]
By default, all command-line arguments are of String format. So two integers passed at the command line also in the string format. So we need to convert them from string to integer. To do that we are going to use the atoi() function. which will perform the ASCII to Integer conversion.
The atoi()function is available as part of the stdlib.h headerfile. So we need to include the stdlib.h headerfile as well.
📢 The atoi() function is used to perform the ASCII to integer conversion. As all command-line arguments are strings we need to convert them to integer before performing the addition.
Once we convert the values to integer, We will perform the addition and display the result on to the console.
Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Compiling code using gcc compiler $ gcc command.c // Our executable is generated and the default executable file name is ./a.out // We added check to provide help info for how to use the executable. $ ./a.out use <executable> <int1> <int2> $ ./a.out 10 use <executable> <int1> <int2> Â // We got the output. $ ./a.out 10 20 Sum of 10 and 20 is : 30 Â // Another try $ ./a.out 877 455 Sum of 877 and 455 is : 1332 $ |
5 Responses
[…] Command line arguments in C programming with examples – SillyCodes […]
[…] Command-line arguments in C programming with examples – SillyCodes […]
[…] Command-line arguments in C programming with examples – SillyCodes […]
[…] Command line arguments in C programming with examples – SillyCodes […]
[…] Command-line arguments in C programming with examples – SillyCodes […]