Bitwise Operators in C Language ( |, &, ~, <<, >>, ^ Operators )
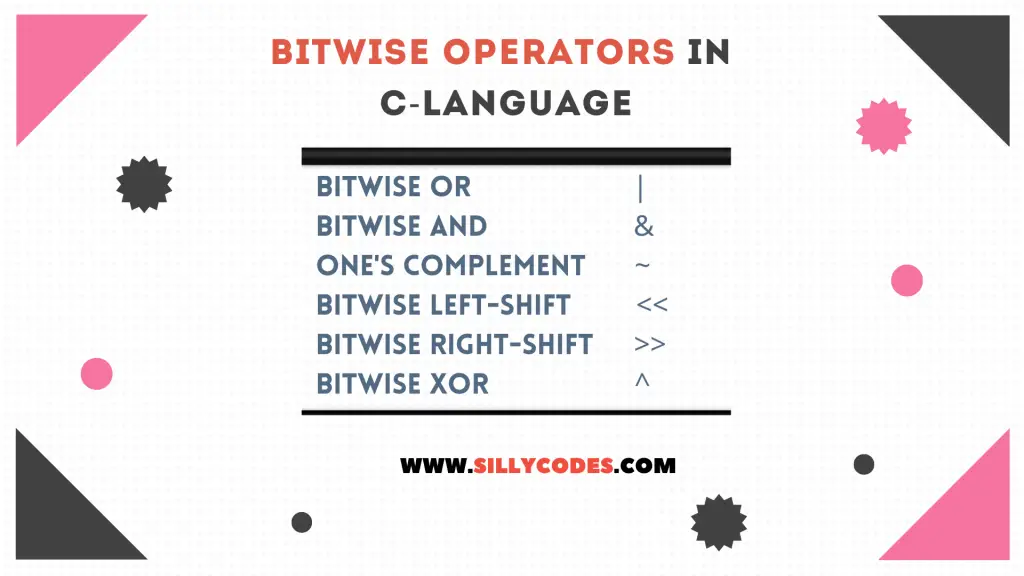
Introduction:
In our previous articles, We have discussed the Logical Operators and Conditional operators in C Language. In today’s article, We are going to look at the Bitwise Operators in C programming Language.
BitWise Operators in C Language:
Bitwise Operators are used for operations on Individual bits (Note that it is bits not Bytes. One Byte contains 8 bits).
So far we have looked at the other operators like Relational, Logical, etc. They all work on the Byte level.
The C language is capable of bit-level operation. It is one of the major advantages of the C language. As we are working at the bit level, We can modify or change the individual bits.
The bit-level operations are very fast in C, So generally Bitwise operators are much faster than the other operations.
The C programming language supports six Bitwise Operators.
- Bitwise OR Operator ( | )
- Bitwise AND Operator ( & )
- Bitwise one’s complement ( ~ )
- Bitwise Left shift Operator ( << )
- Bitwise Right Shift Operator ( >> )
- Bitwise XOR Operator ( ^ )
Bitwise OR Operator ( | ) in C Language:
The Bitwise OR Operator is a binary operator and works on two operands. It takes two input values ( Binary sequences of two values ) and performs the Bitwise OR on each pair of bits in the given sequence.
The output of bitwise OR operation is True (1) only if any of the input bits is True( 1 )
Bitwise Operator output is False or Zero, When both input bits are Zero(0).
Bitwise Operator is denoted by the Vertical line ( | ) in the C programming language.
Here is the truth table of the Bitwise OR operator
Truth Table of Bitwise OR Operator ( | ) in C :
Input bit 1 ( Xi ) | Input bit 2 (Yi) | Result ( Xi | Yi ) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Bitwise OR ( | ) Operator Example:
Let’s take X and Y and assume X=10 and Y=20.
Then the bitwise OR of X and Y ( X | Y ) is 30. Look at the following explanation.
For easy calculations, We are going to take only 8 bits for each number.
Bitwise X|Y is:
X –> 0000 1010 (10 in Binary)
Y –> 0001 0100 (20 in Binary)
X|Y –> 0001 1110 (= 30)
Here is the graphical representation of Bitwise OR Operator
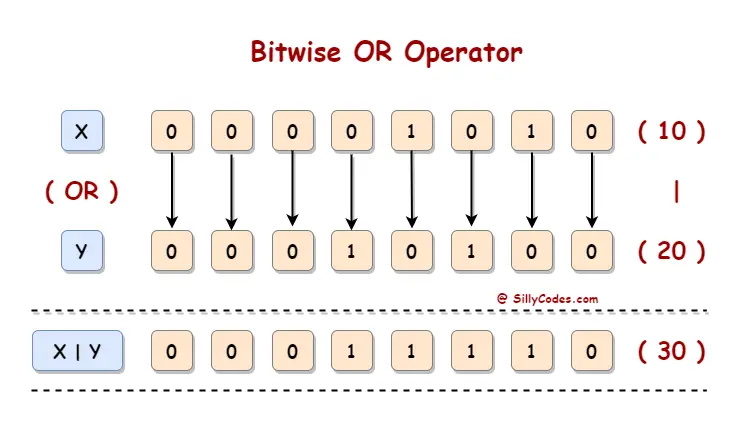
We have looked at the Bitwise OR operator in more detail in the following article, Please have a look at it.
Bitwise AND ( & ) Operator in C Language:
The Bitwise AND Operator also work on two operands. It takes two input values ( Binary sequences of two values ) and performs the Bitwise AND on each pair of bits in the given sequence.
The output of Bitwise AND operation is True(1) only if both of the input bits are True(1)
Bitwise Operator output is False or Zero, When any of the input bits are Zero(0).
Bitwise Operator is denoted by the Ampersand (&) symbol in the C programming language.
📢 Logical AND Operator is represented with the “Two-Ampersands ( && )” symbol, And the Bitwise AND Operator is represented with “One Ampersand (&)” Symbol
Truth Table of Bitwise AND Operator ( & ) :
Input bit 1 ( Xi ) | Input bit 2 (Yi) | Result ( Xi & Yi ) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Bitwise AND ( & ) Operator Example:
Let’s take a couple of numbers X = 8, Y = 7.
Then the bitwise AND of X and Y ( X & Y ) is 0 (Zero). Please have a look following explanation.
Bitwise X&Y is:
X –> 0000 1000 (8 in Binary)
Y –> 0000 0111 (7 in Binary)
X&Y –> 0000 0000 (= 0 )
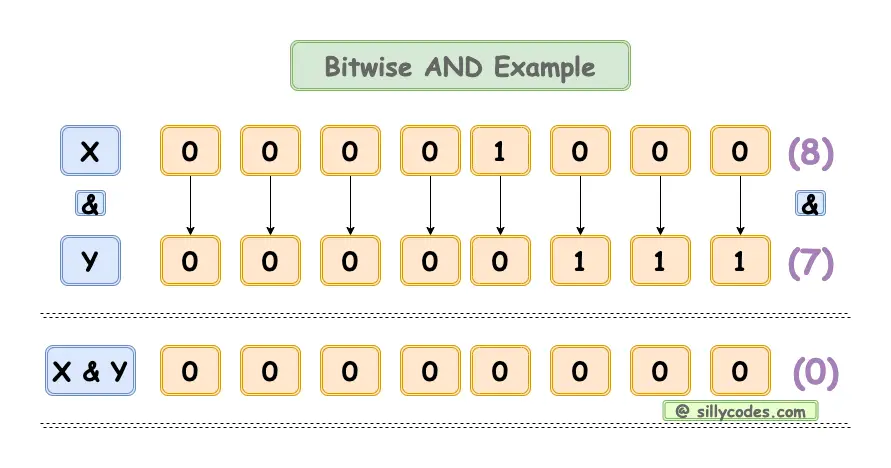
We have looked at the Bitwise AND Operator in detail in the following article, Please check out for more info.
Bitwise One’s Complement Operator ( ~ ) in C:
The One’s Complement operator is a Unary Operator. It works on a single operand.
This Bitwise One’s Complement Operator Inverts the bits of the given number.
If the bit is One (1) Then One’s Complement will invert the bit and makes it Zero(0).
Similarly, If the bit is Zero(0), Then one’s complement will change the bit into One(1).
The Bitwise One’s Complement operator is Denoted by the Tilde ( ~ ) symbol in C programming.
Truth Table of Bitwise One’s Complement Operator:
Xi | ~Xi |
---|---|
0 | 1 |
1 | 0 |
Example:
Let’s take the number X = 10. Bitwise One’s complement of X ( ~X or ~10) is -11. Look at the below bit-level operation.
Bitwise ~X or ~10 is:
X –> 0000 1010 (10 in Binary)
~X –> 1111 0101 (Binary Equivalent of -11, Here X is signed Integer )
Here is the graphical representation of above bitwise one’s complement example.
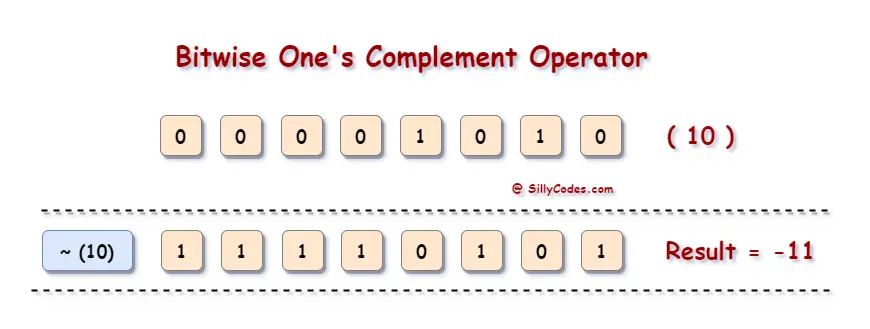
The bitwise One’s complement of any Signed Integer number ( n) is going to be a Negative n+1 Number ( -(n+1) ). In the above example, Bitwise One’s complement of 10 ( ~10 ) is equal to -(10+1) which is -11.
We have already looked at bitwise one’s complement operator in detail in the following article
Bitwise Left-Shift Operator ( << ) in C:
The Bitwise Lefshift operator is a binary operator and requires two operands.
The Bitwise Left-shift Operator is used to shift or move the bits of number by a specified number of positions in the left direction.
In the C programming, The bitwise Left-shift operator is denoted by << symbol.
Syntax of Left-shift Operator:
1 |
TargetNumber << NoOfPositions |
The bitwise Left shift operator ( << ) has two operands.
Leftside operand is the “Number to shift”, Rightside Operand is the “Number of positions to shift”
Example – Bitwise Left-shift Operator ( << ):
Let’s take an example number – X = 10. Now perform the bitwise left-shift operation on X.
We are going to left shift one position of X. The X << 1; is value 20.
Here is the explanation
Bitwise X << 1 is:
X –> 0000 1010 (Binary Equivalent of 10)
X << 1 –> 0001 0100 (Binary Equivalent of 20)
Here is the graphical representation of above bitwise Left shift operation
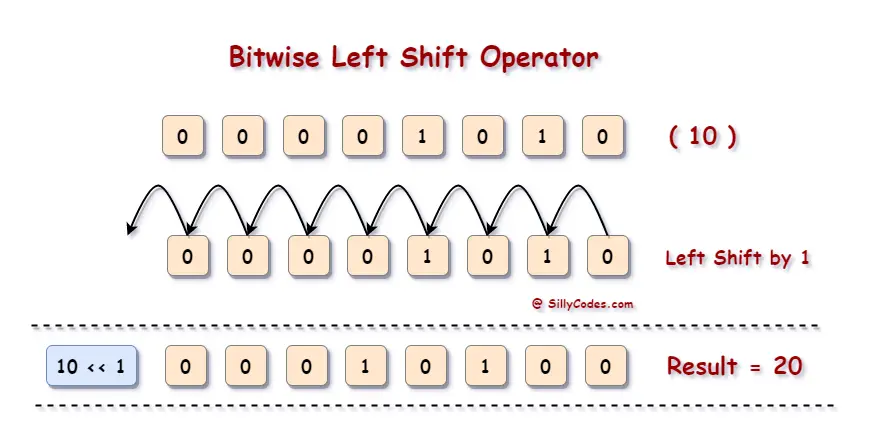
Whenever we left shift a number ( X) by 1 position, Then the result becomes 2 * X, So we can make any number double by left-shifting the number by one position.
📢 If we left shift a number(x) by ‘n’ positions, Then the result will be X * 2n ( 2 power n )
Similarly, If you left-shift any number ( X) by two positions then the result will be X * 22 or X * 4
Ex: 10 << 2 = 40
Here is the in-detail article on Bitwise Left shift Operator
▶️ Bitwise Left Shift Operator in C Language with Example Programs
Bitwise Right Shift Operator ( >> ) in C:
The Bitwise Right-shift operator also is a binary operator and works with two operands.
Bitwise Right-shift Operator is used to shift or move the bits of number by a specified number of positions in the Right Direction.
In C programming, The bitwise Right-shift operator is denoted by >> symbol.
Syntax of Bitwise Right-shift Operator:
1 |
TargetNumber >> NoOfPositions |
The bitwise Right-shift operator ( >> ) have two operands.
Leftside operand is the “Number to shift”, Rightside Operand is the “Number of positions to shift”
Example – Bitwise Right-shift Operator ( >> ) :
Let’s take an example number – X = 20. Now perform the bitwise right-shift operation on X.
We are going to right shift one position of X. The X >> 1; is value 10. Look at the following explanation.
Bitwise X >> 1 is:
X –> 0001 0101 (Binary Equivalent of 20)
X >> 1 –> 0000 1010 (Binary Equivalent of 10)
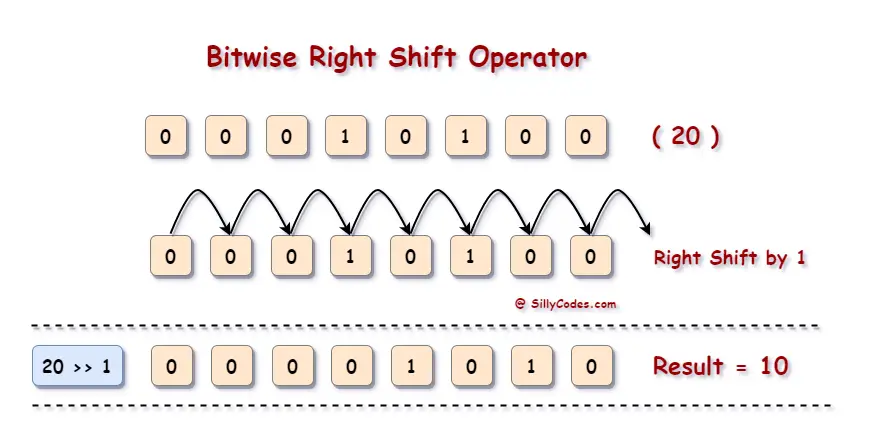
Right-shifting any number ( X) by one position gives us the result as the number/2 ( X/2 ), So we can make any number by half by just right-shifting it by one position.
📢 If we Right shift any number( X ) by ‘n’ positions, Then the result will be X / 2n ( 2 power n )
We have covered the bitwise right shift operator in detail in the following article,
Bitwise XOR Operator ( ^ ) in C Language:
It is a binary operator, Works on two Operands.
Bitwise XOR Operator takes two input values ( Binary sequences of two values ) and performs the Bitwise XOR on each pair of bits in the given sequence.
- The Bitwise XOR operator returns True only if one of the input is 1 ( Other must be 0)
- XOR Return False, If both the inputs are the same.
In the C Language, The Bitwise XOR Operator is denoted by the Caret ( ^ ) Symbol.
Bitwise XOR Operator Truth Table:
Xi | Yi | Xi ^ Yi ( Xi XOR Yi ) |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 0 |
Example on Bitwise XOR operator:
Let’s take two numbers X = 10 and Y = 5, The Bitwise XOR of X and Y ( X ^ Y ) is 15.
Here is the explanation
Bitwise X^Y is:
X –> 0000 1010 (Binary Equivalent of 10)
Y –> 0000 0101 (Binary Equivalent of 5)
X^Y –> 0000 1111 ( X ^ Y = 15 )
Here is the graphical representation of above calculations
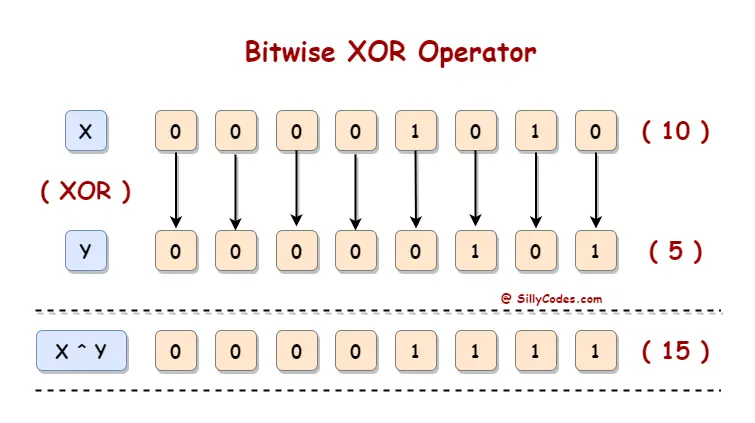
We have looked at the Bitwise XOROperator in detail in the following article, Please check out for more info.
Few Characteristics of Bitwise Operators in C language :
- Don’t compare the bitwise operators and Logical Operators. Logical Operator operates on the Byte-Level and Bitwise Operators operates at the Bit-Level.
- It is important to differentiate between the Bitwise AND ( & ) Bitwise OR ( | ) operators symbols and Logical AND (&&) and Logical OR ( || ) Operators symbols. The bitwise operator’s symbol only has one character, while the logical operators consists of two characters.
- Bitwise Operators only with the Integer data values. We can’t use Bitwise operators on Float data.
- Bitwise operators are faster than normal operators, so it’s recommended to use them whenever possible.
Tutorials Index :
- Start Here – Step by step tutorials to Learn C programming Online with Example Programs – SillyCodes
Related Posts :
- Arithmetic Operators with Examples.
- Arithmetic operators priority and it’s Associativity.
- Modulus Operator and Hidden Concepts of Modulus Operator.
- Precedence Table or Operators Priority Table.
- Assignment Operator, Usage, Examples
- Increment Operator and Different types of Increment operators Usage with Examples.
- Decrement Operator and Different types of Decrement operators with Examples.
- Logical or Boolean Operators.
9 Responses
[…] Bit-wise Operators. […]
[…] Bit wise Operators in C Language – SillyCodes […]
[…] 📌 Learn more about bitwise operators at the following article – Bit wise Operators in C Language – SillyCodes […]
[…] are going to use the Bitwise Operators to convert the given Decimal (Integer) number to Binary […]
[…] Bit-wise Operators. […]
[…] our previous article, We have looked at what are Bitwise Operators and different Bitwise Operators. We briefly looked into them. This article will look at the Bitwise AND Operator in C Language in […]
[…] the previous articles, We have discussed what are Bitwise Operators and Bitwise AND Operator. In today’s article, we will learn about the Bitwise OR operator in […]
[…] the previous articles, We have discussed what are Bitwise Operators and Bitwise AND Operator, and Bitwise OR operators. In today’s article, we will learn about […]
[…] Bitwise Operators in C Language ( Bitwise OR, AND, XOR, left shiftt, Right Shift) […]