Functions in C Language with Example programs
- Introduction:
- Functions in C Programming language:
- What are the Advantages of Functions:
- Different Types of Functions in C Language:
- Library Functions:
- User-Defined Functions in C:
- Elements of C Functions:
- Syntax of Functions in C programming language:
- Function Declaration:
- Function Definition:
- Function Call:
- Return Statement:
- Program to Understand the functions in C Programming Language:
- C Functions Program Explanation – How Function Call works:
- The main() function in C Programming Language:
- Conclusion:
- Functions Practice Programs:
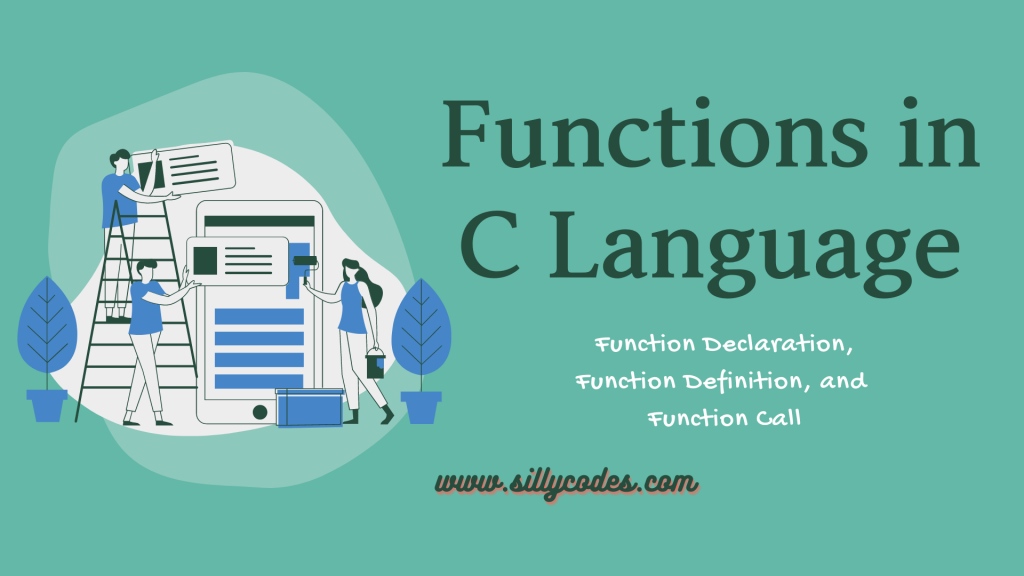
Introduction:
We have looked at the Decision Making statements and Loops in our earlier articles, In today’s article, We will look at the Functions in C language, different types of functions, and how to create and call functions in C programming language.
Functions in C Programming language:
A function is a collection of related statements that carry out a specific task. We also call the functions the subprogram.
The C Program is a collection of functions. So we can have one or more functions in the C program.
For example, If You have a large task to perform, Then it is a good idea to divide the large task into smaller and well-defined tasks and then complete them.
The same is true for the functions in C programming language as well, If you have a large task then divide it into multiple smaller tasks and create a function for each task, Instead of creating a single function that does all tasks.
📢 A function is a block of code, which does a specific task
If you are program contains only one function, Then that function must be the main() function.
What are the Advantages of Functions:
- Functions help us to Modularize our Programs. As functions allow us to divide the program into smaller and well-defined tasks, we can develop programs in a modular format.
- Functions help us to Reuse the Code. For instance, If we need to perform a particular task more than once, we can create a function for it and use it as many times as necessary. We have avoided writing the code many times(avoided code repetition) by creating and reusing the functions.
- Utilizing functions makes programming simpler. We can write smaller tasks (functions) easily and efficiently.
- We can increase the readability of our code by using functions. Since we can quickly get to the desired function rather than scrolling through the entire program.
- Functions improve the code debugging, Testing of code, and Maintenance of the code. As we can simply modify the specific function instead of changing the whole program.
- We can also store the functions in the library files and reuse them in other programs and share them with other programmers.
Different Types of Functions in C Language:
C Programming language has two different types of functions, They are
- Library Functions.
- User-Defined functions.
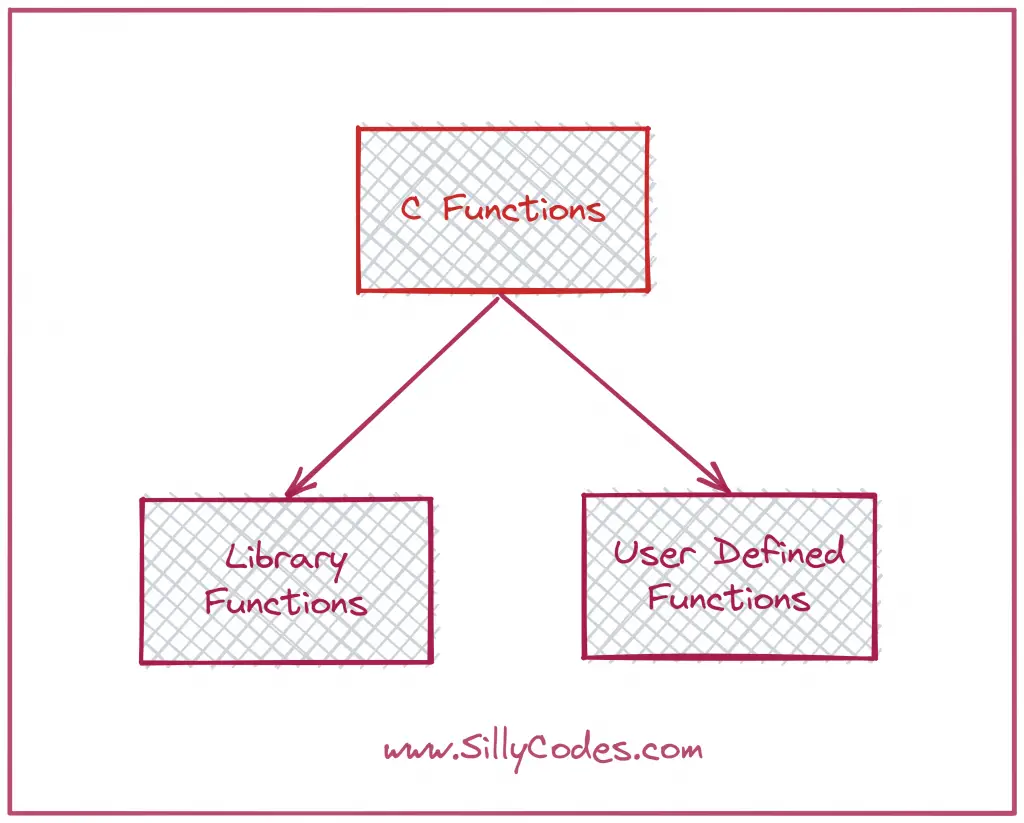
Library Functions:
The library functions are also called in-built functions or Predefined functions. The C Language (Compiler) comes with a few functions which help us to do certain tasks. These functions are called Library functions.
These library functions are present in the C library, We have used many predefined functions while writing the C Programs so far, for example, The functions like printf(), scanf(), pow(), and sqrt(), etc are part of the C library.
📢 The printf() and scanf() functions are used for standard input and standard output operations.
To use any library function, We need to add the required header file. For example, To use the standard input, and standard output functions like printf() and scanf(), We need to include the stdio.h header file.
Similarly, To use the pow() function, We need to include the math.h header file.
Please note, That the header files only contain the library functions declarations, The C library functions come in pre-compiled format, They will be linked to our program during the Linking process of the Compilation Process of the C program.
User-Defined Functions in C:
The C library comes with few general-purpose functions, There are many other functions that are not part of the C library. To overcome this problem. C Programming Language allows programmers to define their own functions to perform a specific task, These functions are called User-defined functions.
Elements of C Functions:
To define and use functions, We need to have the following three things
- Function Declaration
- Function Definition
- Function Call
Here is the syntax of the C Functions.
Syntax of Functions in C programming language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// header files  // function declaration return_type function_name ( argument_list);   int main() {   ....   // statements   .....   // function call   result = function_name (argument_list);   .....   // statements; }   // function definition return_type function_name (argument_list) { // Function_body return <some-expression>; } |
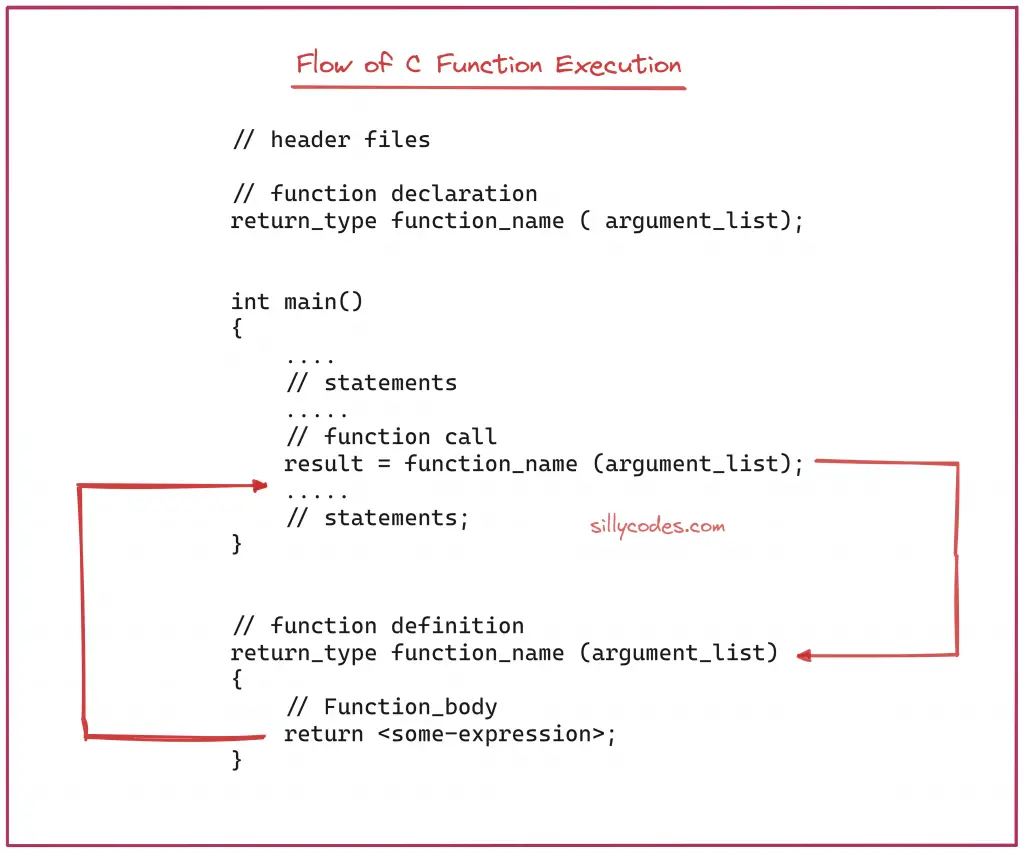
Let’s look at them one by one.
Function Declaration:
The Declaration is also called as the function Prototype. The calling function needs to know the syntax of the called function, So we need to use the function declaration to let the compiler know about the function-related details like name, etc.
Syntax of a function declaration:
return_type function_name(arguments list);
The function declaration contains the
- Function name (
function_name)
- The name of the user-defined function. Can be any C Identifier.
- The function name within the program must be unique. C language won’t support function overloading.
- Arguments List (
arguments_list)
- The argument list specifies the number of arguments that the function accepts and what are the datatypes of these arguments.
- These arguments are called formal arguments. and used to provide input to the function.
- The function can take any number of arguments. ( or won’t take any arguments at all )
- Here is an example of arguments_list – ( datatype1 arg1, datatype2 arg2, ….)
- Return Type (
return_type) :
- The data type of the return value of the function.
- There will be instances where you’ll want to send data back to the caller. To achieve this, use the return statement with the desired value.
- If the function doesn’t return any value, Then we can use the void as the return type
The function declaration tells the compiler that there is a function, Which is going to be defined later in the program, But here is the syntax or prototype of the program. So if any other function calls this function (don’t complain 😃), match the function call with the provided function declaration.
The function declaration is also called as the Forward declaration.
If the called function is defined before using it, Then we don’t need to explicitly declare the function. ( No need to do the function declaration)
📢 Function declaration ends with a semicolon (;).
Function Definition:
The function definition is the block of code, Which contains the statements to execute. The function definition is an important part of the function, As it contains the actual code to accomplish the specified task.
Here is the syntax of the function Definition:
1 2 3 4 5 6 7 |
return_type  function_name (arguments_list) {     // function body     // statements     // statements     return <some_value>; } |
The function definition contains two parts
- Function header
- Function Body.
Function header:
In the above syntax, The first line, i.e return_type function_name (arguments_list) is called as the function header.
The function header contains the function_name, arguments_list, and return_type.
If you notice, The function header looks very similar to the function declaration except that the function declaration contains the semicolon( ;) at the end, But the function header won’t contain the semicolon at the end. ( Specifying the variable name is not mandatory in the function declaration, so that is another difference).
Function Body:
The Function body contains the actual code statements and the function body is enclosed by curly statements ( { ... } ). The function body also contains a Return statement, Which helps the function to send data back to the caller ( The parent function, which is called this function).
The Return statement is optional. We will look at the return statement in detail in the next section.
A function definition can be placed anywhere in the function. but make sure to add the forward declaration, So that the compiler knows the prototype of the function.
Function Call:
So far, We have looked at the function declaration and function definition, But how to use the function? To use the function, We need to call the function. We can call the function from anywhere in the program. A function can be called by specifying the function name and argument list like below.
Here is the syntax of the function call in C:
1 2 3 4 5 |
function_name(arguments_list);  or  result = function_name(arguments_list);    // if your function returns any value |
The function_name and arguments_list in the function call must match the function_name and argument_list in the function definition and function declaration.
If the function returns any value, We can get the return value by using the following syntax
result = function_name(arguments_list);
The variable result contains the returned value.
The argument_list, which is passed here is called actual arguments.
Whenever the function call is executed, The program control jumps to the called function (here function_name function) and once the called function is completed, The program control comes back to the caller function (the function from where we called the other function).
Return Statement:
The return statement is used to return a value from the function to the caller ( Parent function ). The data type of the return value must match the datatype specified in the function declaration and function definition.
Syntax of return statement:
1 2 3 4 5 |
return <some_expression>;  // has return type  or  return;    // no return type. |
The Return statement is used inside of a function. The return statement terminates the function execution and program control will be sent back to the caller function (parent function) with the return value ( if the return statement has the return value).
Program to Understand the functions in C Programming Language:
Let’s look at a practical example to understand the C functions. Let’s look at a program to perform addition and subtraction on two numbers using the C functions.
The following program accepts two integers from the user and calculates the sum and subtraction.
📢 We have added a lot of comments to explain the program logic
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/*     C Program to understand the C Functions     Author: Sillycodes.com */  #include<stdio.h>  /* Function Declaration */ int add(int num1, int num2);  /*     Subtract function definition     Function_name: subtract     arguments_list: (int num1, int num2)     return_type: int */ int subtract(int num1, int num2) {     return num1 - num2; }  int main() {     int num1, num2, sum, sub;     printf("Enter two numbers : ");     scanf("%d%d", &num1, &num2);      // Call add function to calculate the sum     // 'add' function is defined after the call     // so we need to declare it.     sum = add(num1, num2);         // Call subtract function to calculate the subtraction     // As we are defining the subtract before using it     // We no need to declare it explicitly.     sub = subtract(num1, num2);      printf("Sum: %d and Subtract: %d\n", sum, sub);      return 0; }  /*     add function definition     Function_name: add     arguments_list: (int num1, int num2)     return_type: int */ int add(int num1, int num2) {     return num1 + num2; } |
Program Output:
Compile and run the program using your favorite compiler. We are using the GCC compiler here.
1 2 3 4 5 |
$ gcc c-functions-example.c $ ./a.out Enter two numbers : 20 10 Sum: 30 and Subtract: 10 $ |
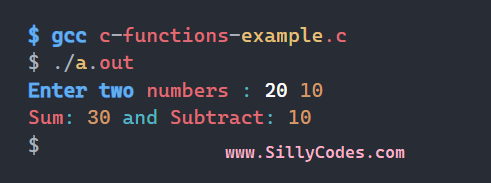
C Functions Program Explanation – How Function Call works:
We have defined two functions named add and subtract in the above program. Let’s look at them one by one.
The add function Flow:
Function Definition:
The add function takes two integer numbers as input and returns an Integer value. So we specified the following syntax
1 2 3 4 |
int add(int num1, int num2) { Â Â Â Â return num1 + num2; } |
The details of addfunction prototype details:
- Function_name: add
- arguments_list: (int num1, int num2)
- return_type: int
Function Declaration ( Forward Declaration):
As we defined the add function after making the call, So the compiler doesn’t know about the add function prototype, So we need to specify the prototype using the function declaration. So we have declared the add function at the start of the program using
1 2 |
/* Function Declaration */ int add(int num1, int num2); |
Function Call:
Finally, We called the add function using
1 |
sum = add(num1, num2); |
Note that, We have passed two integer values num1and num2to the program and as the add function returns an integer value, We stored it in variable sum.
The subtract function flow:
Function Definition:
The subtract function also takes two integers as input and subtracts the provided integers and returns the results back to the caller using the return statement
Here is the definition of the subtract function
1 2 3 4 |
int subtract(int num1, int num2) { Â Â Â Â return num1 - num2; } |
The details of subtract function definition:
- Function_name: subtract
- arguments_list: (int num1, int num2)
- return_type: int
Function Declaration:
We have defined the subtract function before calling it from the main() function. So we no need to do the forward declaration or function declaration. So we skipped the declaration of subtract function.
📢 The function declaration is not needed if the function is defined before the function call.
Function Call:
We called the subtract function from the main() function.
1 |
sub = subtract(num1, num2); |
We have passed the two integers num1 and num2 and stored the return value in the variable sub.
The main() function in C Programming Language:
The main() function in the C programming language is a special type of function. The C program execution always starts with the main() function and we call all other functions from the main() or its child functions() ( which are called from the main() function).
If you have only one function in your program, That function must be the main function. If you have multiple functions one of them must be the main function.
By default main function returns an Integer value. The operating system(start-up code) invokes the main function and we can send the exit status of the program back to the operating system using return or exit() function. The Normal program exit is represented by sending(returning) the integer value and any non-zero exit code is treated as an abnormal program exit.
The program compilation starts from Top to Bottom. But the C Program execution starts with the main functions and ends with the main() function.
Conclusion:
We have introduced the Functions in C programming language, We looked at different types of functions and how to declare, define, and call functions. Finally, We went through a practical example of Functions.
In the next tutorial, We are going to look at the Different Types of Functions in the C Programming Language.
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- Arithmetic Operations using Functions
- Add Two Numbers using Function
- 100+ C Practice Programs
- C Tutorials Index
48 Responses
[…] Functions in C language with example programs […]
[…] Functions in C language […]
[…] Functions in C Language with Example Programs […]
[…] our previous article, We have looked at the introduction to C functions and how to create and call functions. In this article, We are going to look at the Type of […]
[…] Functions in C Language […]
[…] Functions in C Language […]
[…] Functions in C Language […]
[…] Functions in C Language with Example Programs […]
[…] have looked at the Introduction to C Functions and Types of C functions in our earlier posts. In today’s article, We are going to look at Call […]
[…] is recommended to know the basics of the Functions in C Language, Recursion in Programming, and Relational […]
[…] Functions in C Language with Examples and call flow […]
[…] Functions in C language with Example Programs […]
[…] Functions in C Language […]
[…] How to Define, Declare and call functions in C Langauge with Example Programs […]
[…] Functions in C Langauge – Declare, Define, and Call functions […]
[…] have looked at the Functions in C and Recursion in C in our earlier articles, In today’s article, We are going to look at the […]
[…] is recommended to know the basics of the Functions in C language and Recursion in C […]
[…] Functions in C Language with Example Programs […]
[…] is required to know the Arrays and Functions in C language to better understand the following […]
[…] Functions in C – How to Declare, Define, and Call Functions […]
[…] are going to use arrays and functions, So it is a good idea to know about Arrays in C, Functions in C, and Passing Arrays to […]
[…] Functions in C Language […]
[…] Functions and How to Create and Use Functions in C with Examples […]
[…] Functions in C Langauge with Example Programs […]
[…] Functions in C Language […]
[…] Functions in C programming – how to create and use functions […]
[…] Check if the array size is out of bounds (max size is 100 – change this if you want to use large arrays), If the array is out of bounds, Display an error message and terminate the program using the return statement. […]
[…] Display the FOUND message on the console and stop the program using the return statement […]
[…] Functions in C with Examples […]
[…] is recommended to know the basics of the C Arrays, Functions in C, and Mutli-dimentional Arrays or Matrices in C […]
[…] Functions in C […]
[…] Functions in C Language with Example Programs […]
[…] Functions in C – Declare, Define, and Call Functions […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Check if the len1 and len2 are equal, If the two strings’ lengths are not equal, Then they are not anagrams. So display the error message and stop the program using return statement. […]
[…] the main() function, Call the swap() function and pass the number1 and number2 variables with their addresses. […]
[…] function also returns the generated array(pointer to the generated array – int *) back to the […]
[…] Functions in C – How to Create and Use functions in C […]
[…] Functions in C […]
[…] Functions in C […]
[…] can allocate the memory using functions like malloc(), calloc(), and realloc(). Similarly, We can de-allocate the memory using the free() […]