Program to demonstrate Address of Operator in C Language (&)
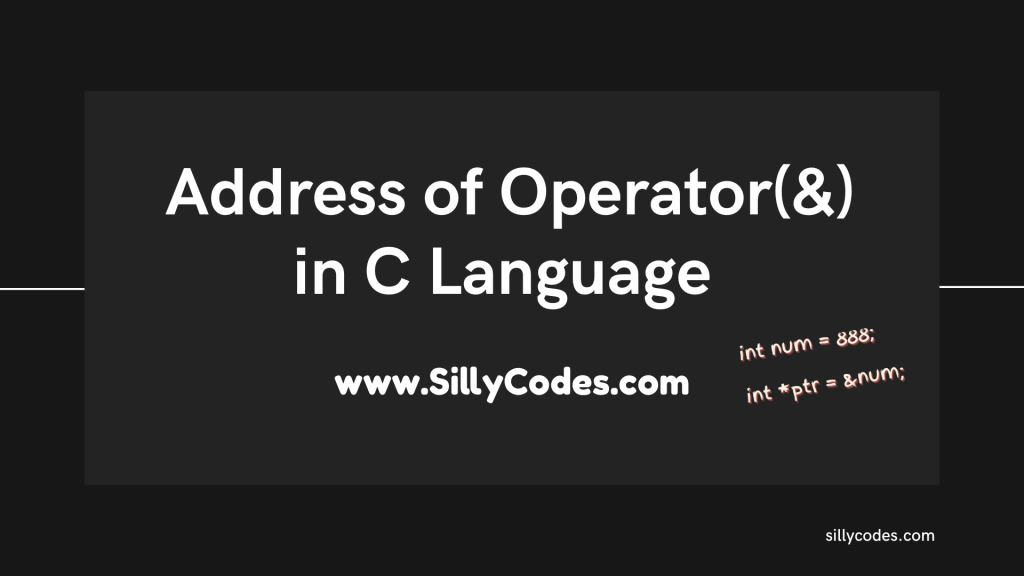
Introduction:
We have looked at the introduction to pointers in an earlier post. In today’s article, We will look at the address of operator in c programming language with example programs.
Address of Operator in C Language (‘&’):
The address of operator in C programming language is used to get the memory address of a variable.
As we know, each variable is stored at a specific memory address. We can use the address of operator to get that memory address.
The address of operator is denoted by using the ampersand & character. It is a unary operator, which means it operates on a single operand.
Syntax of Address of Operator (&):
1 |
&variableName; |
In order to get the memory address of a variable, Place the address of operator(ampersand &) before the variable name.
Example of Address of Operator usage:
Here is how we can use the address of operator to get the memory address of any variable
1 2 3 4 |
// initialize a variable int marks= 64; int *ptr = &marks // address of 'marks' variable |
We can get the marks variable address by using the address of the operator like &marks.
Also note that The address of a variable can only be stored in the pointer variable. So we used the integer pointer variable ptr to store the address of the marks variable.
Here is the graphical representation of how address of operator (&) works in C programming language.
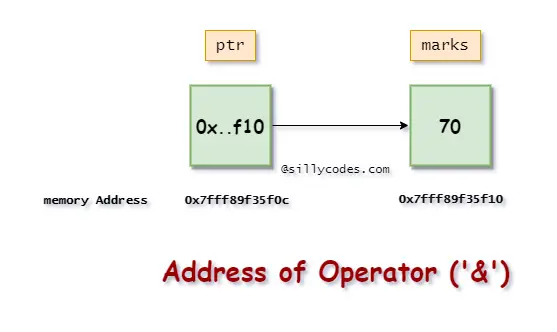
As we can see from the above image, The ptr variable is storing the address of marks variable, which we got using the address of operator(&)
📢 We have used the address of operator (&) many times while creating and practicing the C programs so far. If you notice, We always passed the variables with their addresses to the scanf function.
scanf(“%d%d”, &number1, &number2);
Let’s look at an example program to understand the address of operator in the c programming language.
Program to understand the address of operator(‘&’) in C Language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/* Program to demonstrate the address of operator in C language sillycodes.com */ #include<stdio.h> int main() { // Initialize a variable int marks = 70; // create integer pointer. int *ptr; // Update 'ptr' with the address of 'marks' // use address of operarot ('&') ptr = &marks; // 'marks' variable value printf("Value of marks: %d\n", marks); // get the address of 'marks' variable printf("Address of marks: %p\n", &marks); // value of 'ptr' pointer variable printf("Value of ptr(pointer) :%p\n", ptr); // Address of 'ptr' pointer variable printf("Address of ptr(pointer): %p\n", &ptr); return 0; } |
📢 This Program is part of the Pointers Practice Programs series
Program Output:
Let’s compile and Run the program using your favorite IDE. We are using the GCC compiler to run the program on Ubuntu Linux.
$ gcc address-of-operator-program.c
The above command will create an executable file named a.out in the current working directory ( present directory). Run the executable file using the following command from your terminal.
1 2 3 4 5 6 |
$ ./a.out Value of marks: 70 Address of marks: 0x7fff89f35f0c Value of ptr(pointer) :0x7fff89f35f0c Address of ptr(pointer): 0x7fff89f35f10 $ |
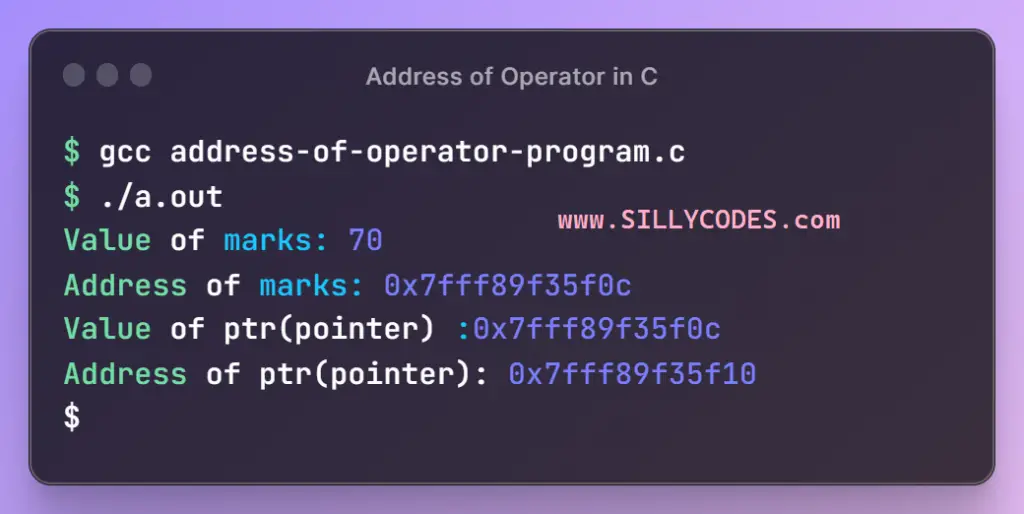
Address of Operator program explanation:
In the above program, We created an integer variable called marks and initialized it with the value 70
As we discussed earlier, The address of operator is used to get the memory address of a variable but inorder to store the memory address of variable, We need a pointer variable, So declare a pointer variable ptr to hold the address of the marks variable.
Then, we used the address of operator (&) operator to get the address of marks (&marks) and stored it in ptr variable.
ptr = &marks;
Finally, We displayed the values and address of the marks and ptr variable. which confirms the pointer variable is storing the address of the marks variable. i.e 0x7fff89f35f0c. This way we can simply get the address of a variable using the Address of operator (&) in C programming language.
Few Quick Notes on the Address of Operator in C ( & ):
There are a few caveats to using the address of operator, let’s look at them.
The address of Operator(&) can’t be used with constants or an expression.
For example,
1) We can’t use the address of operator on Constants like an integer constant.
1 2 |
// Invalid &44; |
The above example is invalid. As we are trying to get the address of an integer constant.
2) Similarly, We can’t use the address of operator on the expression. Please have a look at the following example.
1 2 3 |
int i = 10; int j = 20; int *test = &(i+j); |
Here we are using the address of operator on (i+j) expression, Which is Invalid and raises an error: lvalue required as unary ‘&’ operand
So we can’t use the address of operator on the expressions.
3) Use the address of operator with the appropriate variables and the elements of the arrays, strings, structures, etc.
1 2 3 |
// correct way int i = 100; int *iPtr = &i; |
Here the iPtr is a integer pointer variable, Which can store the address of the variable i.
Conclusion:
We have looked at the Address of Operator in C language (&) in C with an Example program. In the next tutorial, We will look at the dereference operator or indirection operator in C language.
Related Programs and Tutorials:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Functions in C Language with Example Programs
- Types of Functions in C
- Call by Value and Call by Address / Call by Reference in C Langauge
- Recursion in C Language with Example Programs and Call Flow
- Local Variable and Global Variables in C
- Variable Argument functions in C Language
8 Responses
[…] Finally, Call the sum(), subtract(), product(), moduloDivision(), and division() functions from the main() function and pass the num1 and num2 and result variables with addresses using the address of operator(‘&’) […]
[…] Program to Demonstrate Address of Operator (’&’) in C Language […]
[…] Call the swap() function and pass the number1 and number2 variables with their addresses. use the address of operator to pass the address of the number1and […]
[…] is recommended to go through the introduction to the pointers in C and how to use the address of operator and dereference operator in c to better understand the following […]
[…] Program to Demonstrate Address of Operator (’&’) in C Language […]
[…] We have defined a function called addition to add two numbers num1 and num2. Then we printed the address( 0x5653f1791169) of the addition function on the console using the function name and address of operator(‘&’). […]
[…] Program to demonstrate Address of Operator in C Language (&) […]
[…] Program to demonstrate Address of Operator in C Language (&) […]