Dereference Operator in C (*) – Indirection operator in C Language
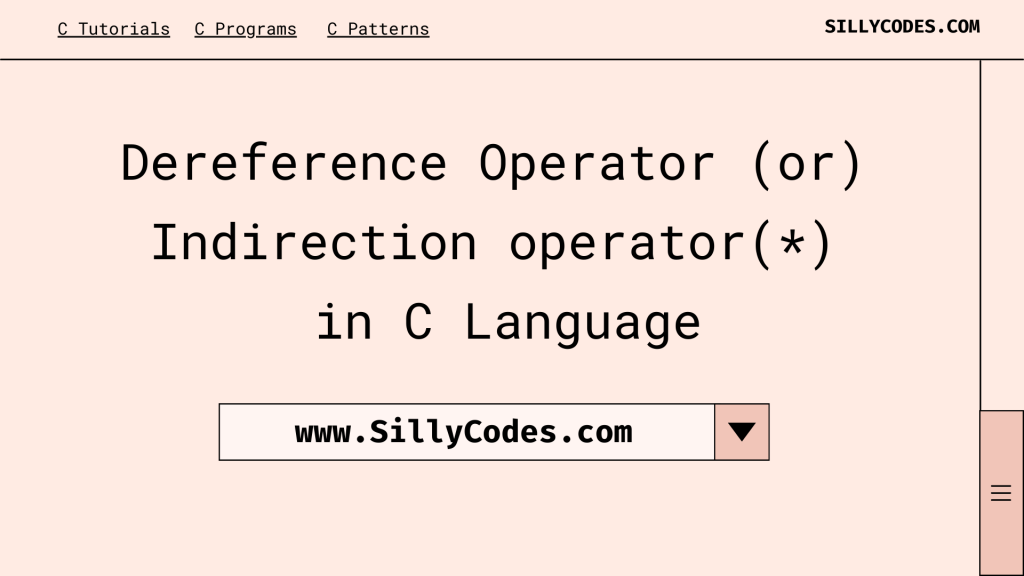
Introduction:
We have looked at the address of operator in c language in earlier posts, In today’s post we will look at the dereference operator in c programming language with an example program.
Prerequisites:
It is recommended to go through the Introduction to Pointers tutorial to better understand this article.
Dereference Operator in C Language:
So far we looked at how the pointer variable stores the address of another variable. We can access the value of the variable pointed by the pointer variable using the dereference operator (*).
The dereference operator is denoted by the Asterisk (‘*’) character. The dereference operator is also called as the indirection operator in C language.
📢 This Program is part of the Pointers Practice Programs series
Let’s say we have an integer variable mac with value 14
int mac = 14;
To store the address of the mac variable, We use an integer pointer like below.
int *macPtr = &mac;
Now, The macPtr holds the address of the mac variable.
The C language allows us to get the value of a variable by using its pointers. In this case, We can get the value of the mac variable by using the macPtr. To get the value of the variable using a pointer, We use the dereference operator i.e asterisk (*).
Use *macPtr to get the value of the mac variable.
printf("Value of mac is : %d\n", *macPtr);
Output:
Value of mac is : 14
📢 The dereference operator is also called as the indirection operator in C language.
Let’s look at an example to get the value of the variable indirectly using a pointer variable.
Get value of variable indirectly using pointer variable using dereferencing operator in C Language:
Here is the program to get the value of the integer variable using the pointer indirectly using indirection operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/*     Program to demonstrate the dereference operator in C     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int mac = 14;      // create a pointer variable 'macPtr'     // store the address of 'mac'     int *macPtr = &mac;      // value of 'mac'     printf("Value of mac variable: %d\n", mac);      // value of the pointer.     printf("Value of the pointer(macPtr): %p\n", macPtr);      // value variable pointed by 'macPtr' pointer     // i.e Get 'mac' value using 'macPtr' pointer     // We need to use the dereference operator. (`*`) with pointer     printf("Value of variable pointed by macPtr(*macPtr): %d\n", *macPtr);      return 0; } |
We have created an integer variable mac and assigned the value 14, Then we initialized a pointer variable macPtr and stored the mac variable address using address of operator(&)
Then we printed the value of the mac and the macPtr. The mac will contain the value 14 and macPtr will contain the memory address.
Finally, To indirectly access the value of the mac variable, We used the dereference operator or Indirection operator(*) with the macPtr pointer variable.
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 |
$ gcc get-value.c $ ./a.out Value of mac variable: 14 Value of the pointer(macPtr): 0x7ffc341e438c Value of variable pointed by macPtr(*macPtr): 14 $ |
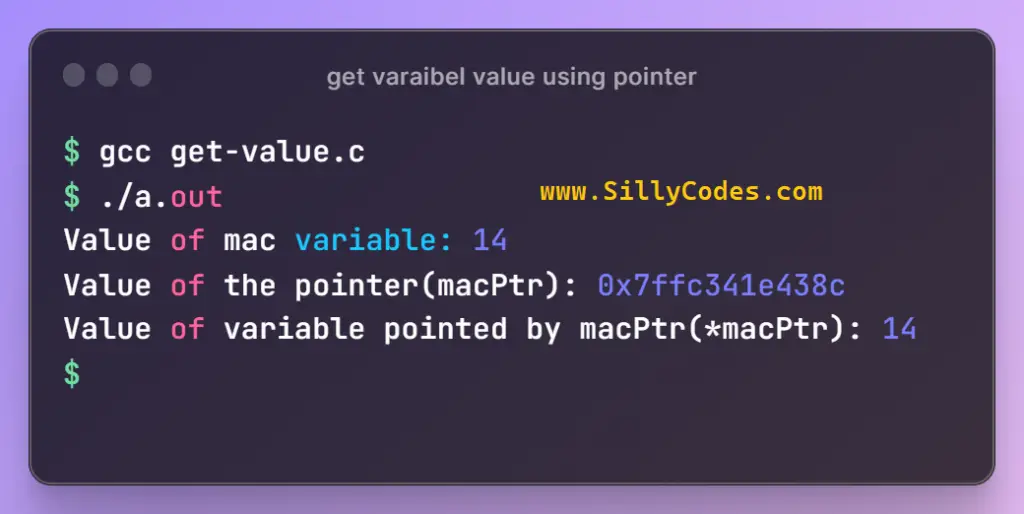
As we can see from the above output, We were able to get the value of the variable mac variable using the macPtr pointer variable using the dereference operator.
Not only we can access the variable value using the pointer variable, We can also change the value of the variable using the pointer variable. Let’s look at another example program to change the variable value using pointer.
Modify Variable Value Indirectly using pointer with dereference Operator in C:
Here is the syntax to change the value of the variable using the pointer variable.
*PointerName = newValue;
Here the pointerName is the pointer which is pointing to a variable(non-constant). The newValue is the new value of the variable.
Here is the program to change/modify the variable value indirectly using the pointer variable in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/*     Program to change value of variable using the dereference operator in C     sillycodes.com */  #include<stdio.h>  int main() {     // create a variable     int bull = 88;      // create a pointer variable 'bullPtr'     // store the address of 'bull'     int *bullPtr = •      // value of 'bull'     printf("Value of bull variable: %d\n", bull);      // value of the pointer.     printf("Value of the pointer(bullPtr): %p\n", bullPtr);      // value variable pointed by 'bullPtr' pointer     // i.e Get 'bull' value using 'bullPtr' pointer     // We need to use the dereference operator. (`*`) with pointer     printf("Value of variable pointed by bullPtr(*bullPtr): %d\n", *bullPtr);      // update the 'bull' using 'bullPtr' pointer     *bullPtr = 44;      // After update     printf("After Update: Value of 'bull' variable: %d\n", bull);      return 0; } |
Program Output:
Compile the program using your compiler.
$ gcc update-value.c
Run the program.
1 2 3 4 5 6 |
$ ./a.out Value of bull variable: 88 Value of the pointer(bullPtr): 0x7fffe8c0984c Value of variable pointed by bullPtr(*bullPtr): 88 After Update: Value of 'bull' variable: 44 $Â Â |
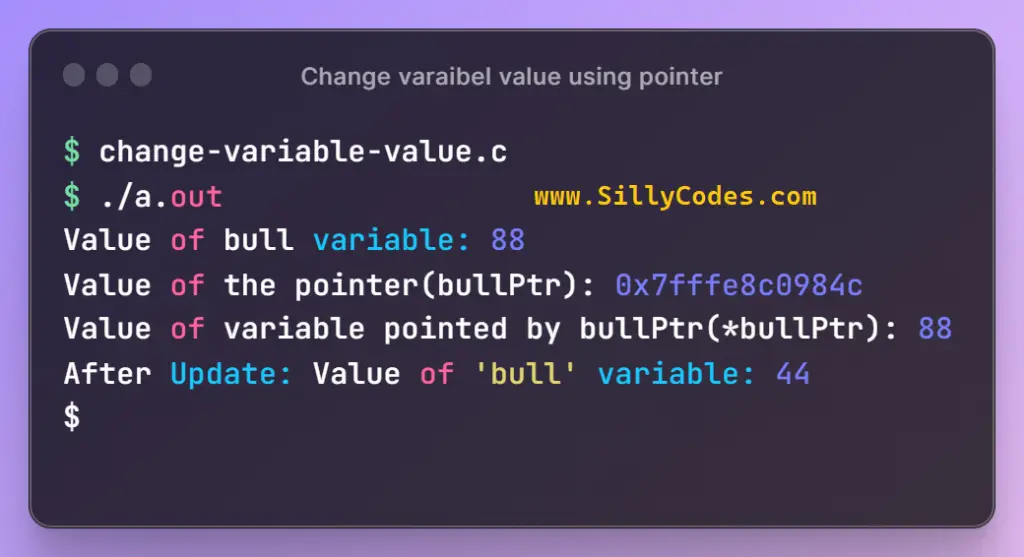
As we can see from the above output, The value of the bull variable is changed to 44.
Program Explanation – Change the value of a variable using the pointer:
We have defined an integer variable bulland the pointer variable bullPtr. Initially the bull variable has the value 88 and the pointer variable bullPtr storing the address of the bull variable.
int bull = 88;
int *bullPtr = •
Finally, we used the dereference operator (*) to modify/update the value of the bull variable using the bullPtr pointer variable like below.
*bullPtr = 44;
This changes the value of the bullvariable and updates it with the number 44. So the new value of the bull variable is 44 (which is modified indirectly using the pointer variable bullPtr).
Related Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
5 Responses
[…] Program to Understand the Dereference or Indirection Operator in C Language […]
[…] using pointers. To access the array’s elements, we will use standard pointer notation (by dereferencing the pointer) as well as pointer subscript […]
[…] using a pointer. To access the string elements, we will use standard pointer notation (by dereferencing the pointer) as well as pointer subscript […]
[…] Dereference Operator in C (*) – Indirection operator in C Language […]
[…] Dereference Operator in C (*) – Indirection operator in C Language […]