Pointer to Pointer in C – Double Pointer in C
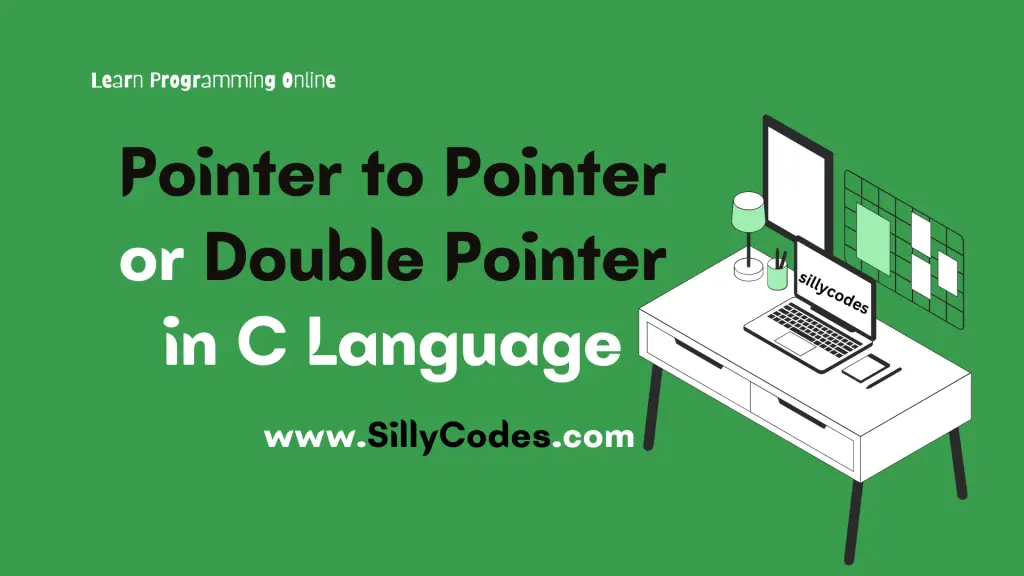
Introduction:
We have looked at the introduction to pointers, and pointer arithmetics in earlier articles. In today’s article, We will look at the pointer to pointer in c language or Double pointer in C programming language with Example Programs.
What is Pointer to Pointer or Double Pointer in C Language:
As we know a pointer is a special variable that holds the address of another variable.
1 2 3 4 5 |
// declare a integer 'num' int num = 99; Â // 'ptr' is integer pointer int *ptr = # |
The pointer is also a variable and it is stored somewhere in memory and it will also have a memory address. We can store this address of the pointer variable in another variable. This variable is called as the Pointer to Pointer variable or Double pointer in C language.
In simple terms, The double pointer is a pointer that stores the address of another pointer variable.
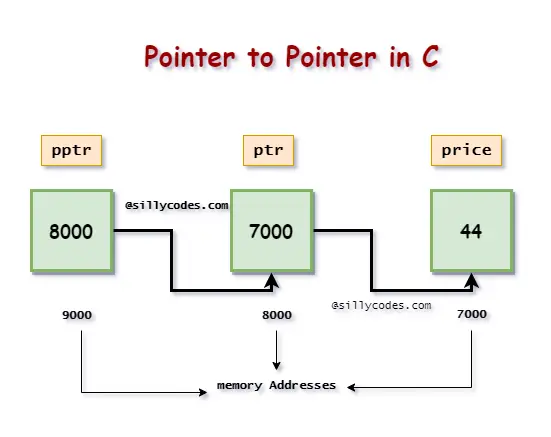
Syntax of double pointer or pointer to pointer in C language:
1 |
datatype **doublePointerName; |
Here
- The doublePointerName is the double-pointer or pointer to pointer.
- datatype is the data type of the variable pointed by the double-pointer.
Let’s look at a couple of examples of pointer to pointer variable to make our understanding concrete.
1 2 3 4 5 6 7 8 9 10 |
// declare a integer variable int price = 110; Â Â // Create Integer pointer variable pointing to 'price' int *ptr = &price; Â Â // create double pointer int **ptr = &ptr; |
In the above example, We Initialized an integer variable named price with value 110.
int price = 110;
Then Created an Integer pointer variable, That stores the address of the price variable.
int *ptr = &price;
Now, To store the address of the pointer variable *ptr, we need to create a pointer to pointer variable or double pointer variable. We can create a double pointer like below.
int **ptr = &ptr;
Here we created a double pointer variable pptr with an integer datatype, Which is storing the address of the pointer variable ptr.
If you notice, The double pointer has two Asterisks before the variable name ( int **ptr). This will let the compiler know that we are working with a double pointer.
Program to understand the double-pointer or pointer to pointer in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/*     Program to understand the pointer to pointer or double pointer in C     www.SillyCodes.com */ #include <stdio.h> int main() {     // declare integer     int price = 110;      // create integer pointer     // to store address of price     int *ptr = &price;      // create double pointer or pointer to pointer     // to store the address of ptr     int **pptr = &ptr;      // detals of 'price' variable     printf("Value of price variable : %d\n", price);     printf("Address of price variable: %p\n", &price);      // value and address of pointer variable     printf("Value of ptr variable(pointer): %p\n", ptr);     printf("Address of ptr variable(&pointer): %p\n", &ptr);      // value and address of doubel pointer variable     printf("Value of pptr variable(pointer to pointer): %p\n", pptr);     printf("Address of pptr variable(double pointer): %p\n", &pptr);      return 0; } |
please look at the program explanation section below for a detailed explanation.
Program Output:
Let’s compile and run the program using the GCC compiler. (Any of your favorite compilers)
1 2 3 4 5 6 7 8 9 |
$ gcc double-pointer.c $ ./a.out Value of price variable : 110 Address of price variable: 0x7ffc7eb45a54 Value of ptr variable(pointer): 0x7ffc7eb45a54 Address of ptr variable(&pointer): 0x7ffc7eb45a58 Value of pptr variable(pointer to pointer): 0x7ffc7eb45a58 Address of pptr variable(double pointer): 0x7ffc7eb45a60 $ |
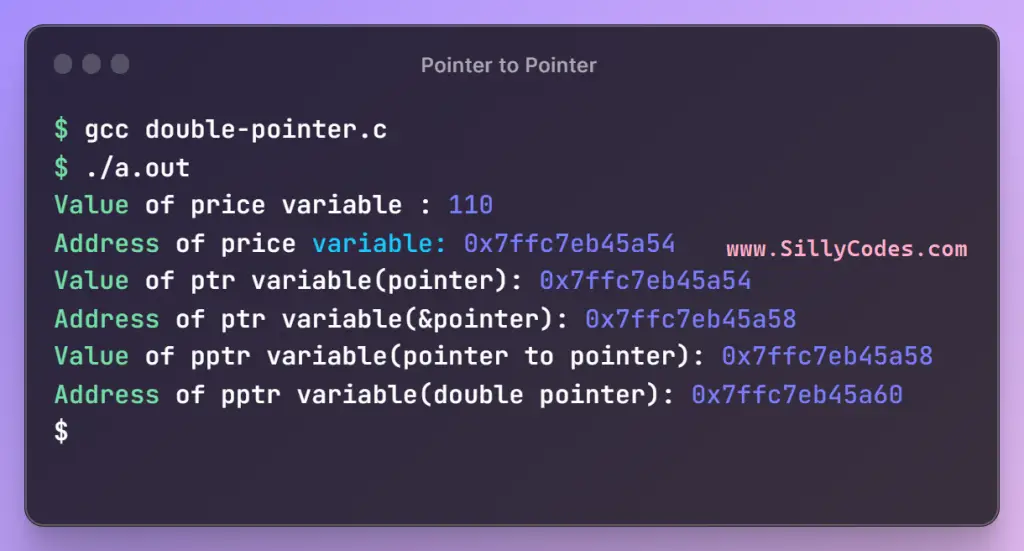
Pointer to Pointer Program Explanation in C:
Here is the graphical representation of how the above integer variable, pointer variable, and a pointer to pointer(Double pointer) variables addresses are mapped.
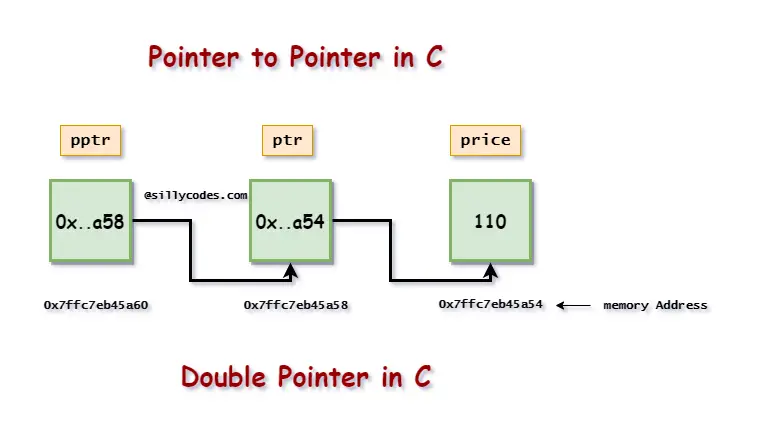
As we can see from the above output and graphical representation of the double-pointer or pointer to pointer variable,
- The price variable is stored at the memory address 0x7ffc7eb45a54 and the value of the price variable is 110.
- The pointer variable ptr is storing the address of the price variable, So the value of the integer pointer ptr is equal to the price variable address i.e 0x7ffc7eb45a54. The ptr variable also has its own memory address which is equal to 0x7ffc7eb45a58 ( &ptr )
- Finally, We created the pointer to the pointer variable
pptr ( i.e double pointer variable) which is used to store the address of the pointer variable
ptr.
- So the value of the double-pointer pptr is equal to the address of the ptr. That is 0x7ffc7eb45a58.
- Again, The double-pointer variable pptr also has its own memory address which is equal to the 0x7ffc7eb45a60.
We can extend the pointer-to-pointer concept further, Let’s say, If you want to store the address of the pptr variable(pointer to pointer), Then we need to create the pointer to pointer to pointer (triple pointer) variable ( int ***ppptr). Which can store the address of the double pointer pptr.
This pointer-to-pointer notion can be expanded indefinitely. But in practice, we usually use up to the double-pointer.
How to get values from pointer to pointer/double pointer in C:
Now, Let’s look at how to get the values from the pointer to pointer variable.
To get the value from the pointer variable, We use the dereference operator or indirection operation ( *). Similarly, To get the value pointed by the pointer to pointer variables we need to use two dereference operators( ** – Two asterisks)
Let’s say we have an integer variable mars with value 4
int mars = 4;
Here are the pointer and double pointers which are storing the address of mars and marsPtr respectively.
int *marsPtr = &mars;
Double pointer
int **marsPPtr = &marsPtr;
Now, The marsPPtr is a pointer to a pointer(aka double pointer). To get the value of the mars, We need to dereference two times.
The first dereference operation on the double pointer( marsPPtr) will give the value of the pointer( marsPtr )
The value of marsPtr is equal to *marsPPtr
Similarly, The two times dereferencing the double pointer( marsPPtr) will give us the value of the original variable(i.e mars )
The value of mars is equal to **marsPPtr;
📢 To get the value pointed by the double pointer or pointer to pointer, We need to dereference two times. ( ** )
Let’s look at an example program on getting the value from the double pointer in c
Program to Get the value from pointer to pointer or double pointer in C ( use double dereference):
In the following program, We have created three variables in the above program
- mars integer variable, which holds the value 4
- Pointer variable marsPtr, which is storing the address of the mars
- Double pointer or pointer to pointer variable marsPPtr, which is storing the address of the marsPtr
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program to get value from double pointer in c     www.SillyCodes.com */ #include <stdio.h> int main() {     // declare integer called 'mars'     int mars = 4;      // create integer pointer     // to store address of mars     int *marsPtr = &mars;      // create double pointer or pointer to pointer     // to store the address of marsPtr     int **marsPPtr = &marsPtr;      // detals of 'mars' variable     printf("Value of mars variable : %d\n", mars);      // value of pointer variable     printf("Value of marsPtr variable(pointer): %p\n", marsPtr);      // value of doubel pointer variable     printf("Value of marsPPtr variable(pointer to pointer): %p\n", marsPPtr);          // get value of 'marsPtr' using 'marsPPtr'     printf("Value of marsPtr using double pointer(*marsPPtr):%p\n", *marsPPtr);      // get value of 'mars' using 'marsPPtr'     printf("Value of mars using double pointer(**marsPPtr):%d\n", **marsPPtr);      return 0; } |
Program Output:
Compile the program.
$ gcc double-pointer-dereference.c
Run the program.
1 2 3 4 5 6 7 |
$ ./a.out Value of mars variable : 4 Value of marsPtr variable(pointer): 0x7fffa777a644 Value of marsPPtr variable(pointer to pointer): 0x7fffa777a648 Value of marsPtr using double pointer(*marsPPtr):0x7fffa777a644 Value of mars using double pointer(**marsPPtr):4 $ |
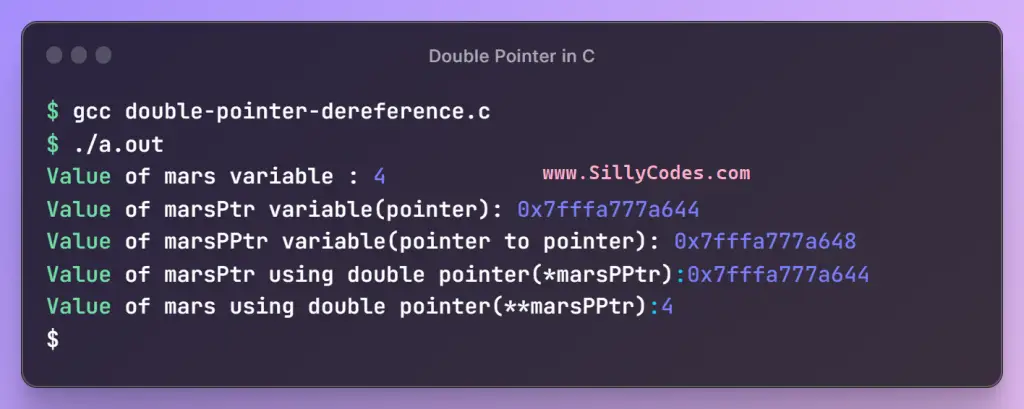
As we can see from the above program and program output, To get the values of mars and marsPtr using the double pointer, Then
- Dereference once to get the value of the marsPtr using double pointer – *marsPPtr
- Dereference twice to get the integer mars value – **marsPPtr
📢In a nutshell, We can get the value of the mars variable by dereferencing the pointer to pointer(Double pointer) marsPPtr twice.
2 Responses
[…] Pointer to Pointer in C – Double Pointer in C […]
[…] Double Pointer (Pointer to Pointer) in C Language […]