Switch Statement in C Language with Example Programs
- Introduction:
- Switch Statement:
- Syntax of Switch statement:
- Program to understand switch statement – Arithmetic Calculator:
- Switch Statement Calculator Program Explanation:
- Switch statement to check character is vowels and consonants:
- Nested Switch Statement or Switch Case:
- Program to understand Nested Switch Statement in C:
- Exercise Program:
- Conclusion:
- Tutorials Index:
- Related Tutorials:
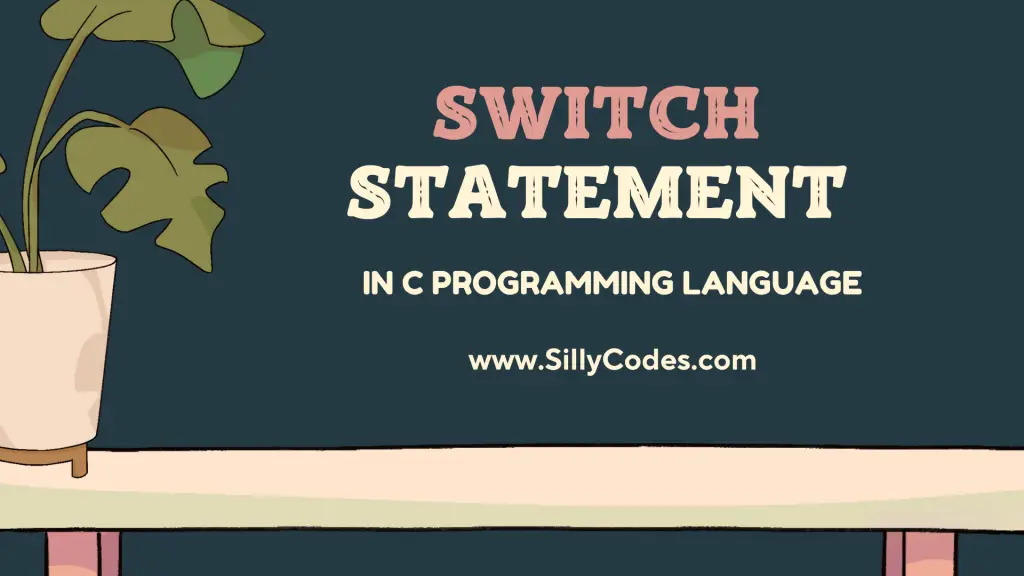
Introduction:
We have discussed the Nested-if-else and if else ladder in an earlier article. In today’s article, we will look at the Switch Statement in C language.
Switch Statement:
The Switch statement is a multi-decision statement. We can use the switch statement to compare the given expression (Switch condition) with multiple conditions(Cases) and execute the respective code block.
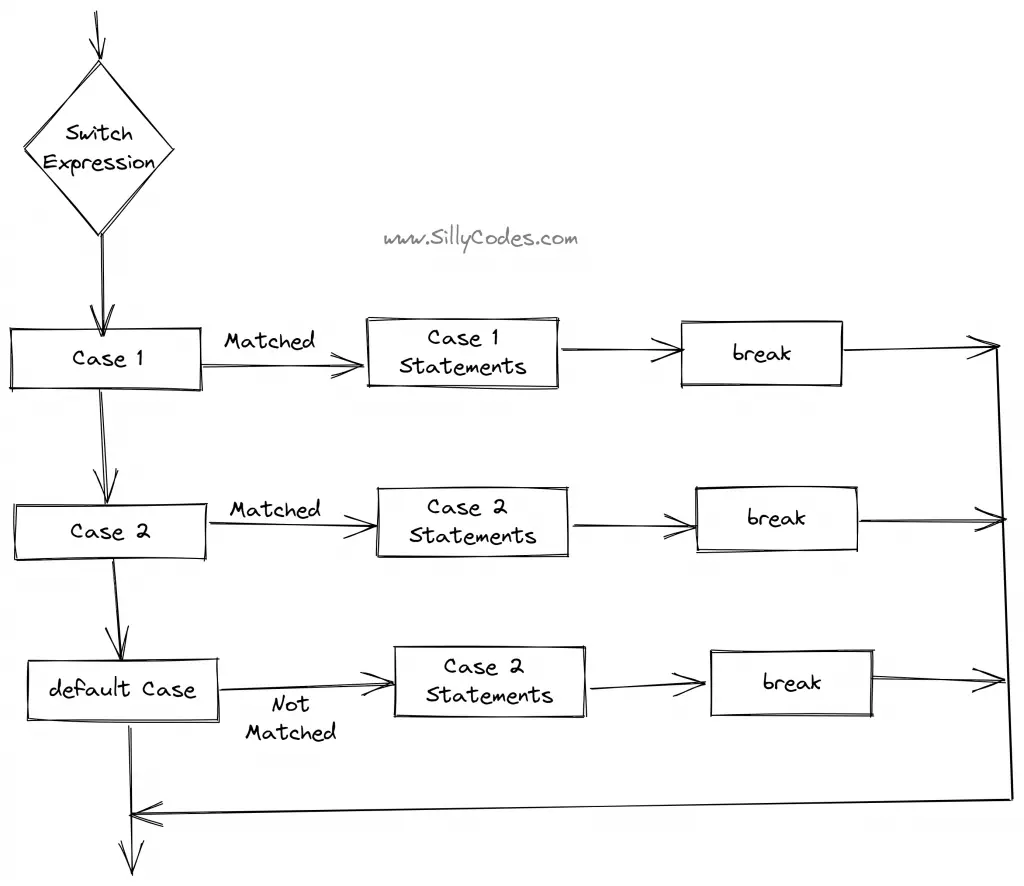
The switch statement is an alternative to the if-else ladder. but the readability of the switch statement is much better compared to if else ladder.
The switch statement is also called the Switch case.
Syntax of Switch statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
switch (expression) { case constant_expression_1: // If the given 'expression' is equal to 'constant_expression_1' // This block of code will be executed ...... ...... break; case constant_expression_2: // If the given 'expression' is equal to 'constant_expression_2' // This block of code will be executed ...... ...... break; case constant_expression_3: // If the given 'expression' is equal to 'constant_expression_3' // This block of code will be executed ...... ...... break; default: // If the 'expression' not matched any of the above 'cases' // Then 'default' case executed. ...... ...... break; } |
As you can see from the above syntax, If the given expression is evaluated against all case
constant_expression’s and if expression matches any constant_expression then that specific block of code will be executed.
For example, If the given expression matched the constant_expression_2 then The case 2 Code block up to the break statement will be executed.
The break statement is used to break out( or come out) of the switch case.
📢 The break statement is optional, But if you forgot to specify the break then the following cases are also executed.
The default case is optional. If the given expression is not matched any case then, Then the default case will be executed.
If the expression not matched any case and the switch doesn’t have default case, Then no action will be taken and program execution goes out of the switch statement.
📢 The switch expression must be of an integer or character type. (Float values are not allowed)
The Case Expression should be Integer or character constant.
When there are many options to pick from, we can use the switch statement or the if-else ladder. However, as the number of possibilities grows, the complexity of the if-else ladder also grows, reducing code readability and occasionally leading to confusion. Therefore, in suitable instances, it is advised to use the switch case rather than the if-else ladder.
To further understand the switch statement, let’s look at an example.
We’re going to use the switch statement to make an arithmetic calculator.
Program to understand switch statement – Arithmetic Calculator:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/* Switch Statement - Calculator Program */ #include <stdio.h> int main(void) { // Take two values from the user int num1, num2; printf("Enter two numbers: "); scanf("%d%d",&num1, &num2); // Take the operator from the user char op; printf("Enter the operator(+,-,*,/): "); scanf(" %c", &op); // We are going to check the given operator `op` with the below cases switch(op) { case '+': // if the expression 'op' matches the '+', This block of code will be executed // Addition operation is performed on given numbers printf("Addition of %d+%d = %d \n", num1, num2, num1+num2); break; case '-': // if the expression 'op' matches the '-', This block of code will be executed // subtraction operation is performed on given numbers printf("Subtraction of %d-%d = %d \n", num1, num2, num1-num2); break; case '*': // if the expression 'op' matches the '*', This block of code will be executed // Multiplication operation is performed on given numbers printf("Product of %d*%d = %d \n", num1, num2, num1*num2); break; case '/': // if the 'op' matches the '/', This block of code will be executed // Division operation is performed on given numbers printf("Division of %d/%d = %d \n", num1, num2, num1/num2); break; default: // The given expression 'op' is not matched any case. // default case will be executed. printf("Please enter valid Operator \n"); break; } return 0; } |
Program Output – Calculator:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc switch-statement.c $ ./a.out Enter two numbers: 20 10 Enter the operator(+,-,*,/): + Addition of 20+10 = 30 $ ./a.out Enter two numbers: 2000 1000 Enter the operator(+,-,*,/): - Subtraction of 2000-1000 = 1000 $ ./a.out Enter two numbers: 30 60 Enter the operator(+,-,*,/): * Product of 30*60 = 1800 $ ./a.out Enter two numbers: 30 10 Enter the operator(+,-,*,/): / Division of 30/10 = 3 $ ./a.out Enter two numbers: 20 10 Enter the operator(+,-,*,/): & Please enter valid Operator $ |
Switch Statement Calculator Program Explanation:
The above program will take the two numbers and desired operator from the user, And perform the selected operation.
We took the two numbers from the users num1 , num2, and the Operator op .
Then we used the switch statement to compare the given operator op against the Addition( +), Subtraction( -), Multiplication( *), and Division( /) cases.
1 2 3 |
Enter two numbers: 20 10 Enter the operator(+,-,*,/): + Addition of 20+10 = 30 |
In the above example, The user entered 20 and 10 as the input values and + as the operator ( op).
The switch expression op (operator) will be compared with all cases, If op matches any case, The block of code until the break will be executed, Here switch found the match at the first case +, and executed the case '+' code block.
Similarly, If we provide the Invalid operator, The default case will be executed.
Switch statement to check character is vowels and consonants:
We have earlier discussed that, If you don’t specify the break after each case, The subsequent case also executes. This often causes bugs but it is also useful to create operator OR like behavior.
To understand better lets look at the following program.
We are going to use the switch case to detect the Vowels and Consonants.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/* Program to detect Vowels or Consonants */ #include <stdio.h> int main(void) { // Take a alphabet from user char ch; printf("Enter alphabet(Lower case): "); scanf(" %c", &ch); // Switch will check the given alphabet with all cases switch(ch) { case 'a': case 'e': case 'i': case 'o': case 'u': // if you note, we have skipped the 'break' statement for above all cases // which means if 'ch' matches any of the above characters, The following printf will executes. printf("Given alphabet %c is Vowel \n", ch); break; default: printf("Given alphabet %c is consonant \n", ch); break; } return 0; } |
Program output:
We used gcc compiler to compile the code and renamed the executable file as checkVowels
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
$ gcc switch-vowels.c -o checkVowels $ ./checkVowels Enter alphabet(Lower case): a Given alphabet a is Vowel $ ./checkVowels Enter alphabet(Lower case): v Given alphabet v is consonant $ ./checkVowels Enter alphabet(Lower case): e Given alphabet e is Vowel $ ./checkVowels Enter alphabet(Lower case): m Given alphabet m is consonant $ ./checkVowels Enter alphabet(Lower case): u Given alphabet u is Vowel $ ./checkVowels Enter alphabet(Lower case): l Given alphabet l is consonant $ ./checkVowels Enter alphabet(Lower case): o Given alphabet o is Vowel $ |
As you can see from the code, We have intentionally not used break statement after the cases a, e, i , o
1 2 3 4 5 6 7 8 9 |
case 'a': case 'e': case 'i': case 'o': case 'u': // if you note, we have skipped the 'break' statement for above all cases // which means if 'ch' matches any of the above characters, The following printf will executes. printf("Given alphabet %c is Vowel \n", ch); break; |
Finally, we included the break statement for the case u.
As the break statement does not exist in the above cases, It becomes like an OR operation. This means even if the user enters any of a, e, i, o, u alphabets, The following code block will be executed(till break).
And finally, we have the default case, If the user enters any other alphabet other than a, e, i, o, u, Then the default case will be executed.
Nested Switch Statement or Switch Case:
We can have Nested Switch statements in the C programming language.
Syntax of Nested Switch Statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
switch (expression) { case constant_expression_1: // If the given 'expression' is equal to 'constant_expression_1' // This block of code will be executed switch(nested_expression) { case nested_constant_expression: // if 'nested_expression` matches the `nested_constant_expression` // This block of code will be executed ...... ...... break; default: // If 'nested_expression' not matched any case // default case will be executed. ...... ...... break; } ...... ...... break; default: // If the 'expression' not matched any of the above 'cases' // Then 'default' case executed. ...... ...... break; } |
As you can see from the syntax above, We can have a Nested switch statement inside the Switch statement.
Here is the program to understand the Nested Switch statement.
Program to understand Nested Switch Statement in C:
We are going to write a Food Order-taking program. The program will ask if the user is Veg or Non-Veg.
Depending upon the user option, We will further present the customizations to the user.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
/* Switch Statement Example program */ #include <stdio.h> int main(void) { // Take two values from the user int number; int type; printf("Press 1 for Non-Veg, 2 for Veg: "); scanf("%d",&number); switch(number) { case 1: // User selected Non-Veg // Ask for the customizations printf("Press 1 for Chicken, 2 for Mutton, and 3 for Fish : "); scanf("%d", &type); switch(type){ case 1: printf("Your order Non-Veg - Chicken received \n"); break; case 2: printf("Your order Non-Veg - Mutton received \n"); break; case 3: printf("Your order Non-Veg - Fish received \n"); break; default: printf("Invalid Option \n"); break; } break; case 2: // User selected Veg // Ask for customizations printf("Press 1 Mashroom, 2 for Panner, and 3 for Salad : "); scanf("%d", &type); switch(type){ case 1: printf("Your order Veg - Mashroom received \n"); break; case 2: printf("Your order Veg - Panner received \n"); break; case 3: printf("Your order Veg - Salad received \n"); break; default: printf("Invalid Option \n"); break; } break; default: printf("Invalid Option - Please try again \n"); break; } return 0; } |
Food Order Program output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc nested-switch-statement.c -o nested-switch $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 2 Your order Non-Veg - Mutton received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 2 Press 1 Mashroom, 2 for Panner, and 3 for Salad : 1 Your order Veg - Mashroom received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 2 Your order Non-Veg - Mutton received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 1 Press 1 for Chicken, 2 for Mutton, and 3 for Fish : 3 Your order Non-Veg - Fish received $ ./nested-switch Press 1 for Non-Veg, 2 for Veg: 2 Press 1 Mashroom, 2 for Panner, and 3 for Salad : 3 Your order Veg - Salad received $ |
Here we used the two switch statements inside the main switch statement.
Sometimes having more nested switch statements can cause confusion, So Make sure you are properly using the break statement at the end of each case.
Exercise Program:
In the earlier posts, We looked at the Grade of the student’s program, But we used the if else ladder. You can try to re-write the Grade of the students program using the Switch statement.
Here is the link to Grade of the students program.
Conclusion:
We have discussed about the switch statement in C programming along with the Nested switch statements and how the break statement affects the flow of the program.
7 Responses
[…] Switch Statement in C Language […]
[…] Switch Statement in C Language […]
[…] Switch Statement in C Language […]
[…] Switch Statement in C Language […]
[…] Switch Statement in C with Example Programs […]
[…] Switch Statement in C Language with Example Programs […]
[…] is a Menu Driven calculator program, We are going to use the switch case and the Infinite do-while loop to create a calculator. So the program displays a Menu with the […]