Function Returning Pointer in C Programming Language
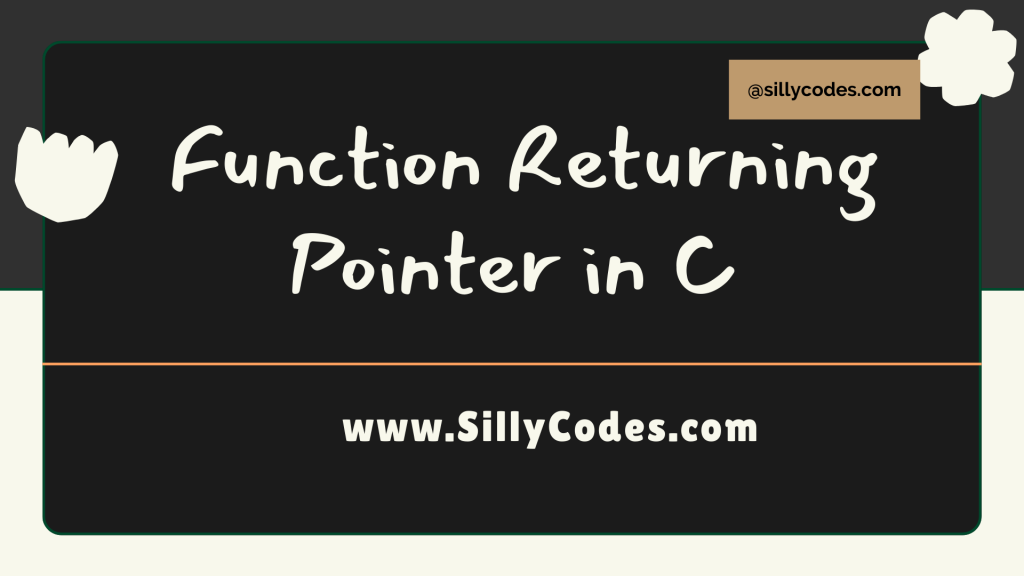
Function Returning Pointer in C:
Write a Program to demonstrate a function returning pointer in c programming language. As we have discussed earlier in the introduction to pointer and pointers and array articles, We can return a pointer variable from a function in C language. We are going to look at such an example in this article.
When we want to return multiple values or an array from a function, we can accomplish this by returning the array as a pointer variable.
We need to use a static variable(static array) inside the function so that it will be live throughout the life of the program.
Program Prerequisites:
Please go through the following pointers and array articles to better understand the program.
Let’s look at the step-by-step explanation of the program, where a function returns a pointer variable in c language.
Function Returning Pointer in C Program Explanation:
We are going to define a function called generateRandomArray() to generate an array with random integer numbers. This function takes the size as the input and generates an array with size number of random elements.
The generateRandomArray() function also returns the generated array as an integer pointer( int *) – i.e the generateRandomArray() function returns an integer pointer back to the caller.
Here is the prototype of the generateRandomArray() function
int * generateRandomArray(int size)
We use the rand() function from the stdlib.h header file to generate the random elements. Here is the syntax of the rand() function.
int rand(void)
We pass the time(0) to the srand() function(seed) to generate random numbers. The time(0) is equal to the number of seconds elapsed from the epoch ( 00:00:00 UTC, January 1, 1970.). If you don’t pass the random seed to the srand() function, Then there is a possibility of getting the same values every time you run the program.
srand(time(0));
Store the random values on a static integer array called randArray.
static int randArr[MAXSIZE];
Why We need to use a Static array:
The default storage class for local variables is automatic, and they will be destroyed once the function returns. Because we need our random array ( randArr ) to be available even after the generateRandomArray() function returns, So we must use a static integer array that will be alive throughout the program’s life.
Inside the main() function, Prompt the user to provide the desired size of array and update the input value in the size variable.
1 |
scanf("%d", &size); |
Finally, Call the generateRandomArray function with the size as the parameter. As the generateRandomArray() function returns an integer pointer, so store the return value in the integer pointer ptr.
ptr = generateRandomArray(size);
Print the array elements by iterating over the ptr using a for loop.
Program to demonstrate Function Returning Pointer in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
/*     Program to understand function returning pointer in c     www.sillycodes.com */  #include <stdio.h> #include <time.h> #include <stdlib.h>  // Max Size of Array #define MAXSIZE 100  /** * @brief - Generate a Random Array * * @param size - Desired Size of the array * @return int* - Generated Random Array */ int * generateRandomArray(int size) {     // create a static Array with 'MAXSIZE'     // static as we need 'randArr' to stay alive after this function returned.     static int randArr[MAXSIZE];      // seed the time(0) to rand function     srand(time(0));      // create random int and store in randArr     for(int i=0; i<size; i++){         randArr[i] = rand()%100+1;     }      // return the array     return randArr; }  int main() {       // create a pointer variable to hold array     int *ptr;      // desired size of array     int size;     printf("Enter the desired size for array(1-100): ");     scanf("%d", &size);      // check for array bounds     if(size >= 100 || size <= 0)     {         // Display error and return         printf("Invalid Array Size(%d), Please try again \n", size);         return 0;     }      // call the 'generateRandomArray()' function     ptr = generateRandomArray(size);      // print the random array     for(int i=0; i<size; i++)     {         printf("value of ptr[%d]=%d\n", i, ptr[i]);     }      return 0; } |
We have included detailed comments in the program for your convenience.
Note that, the randArr is a static array.
Program Output:
Let’s compile and run the program using your favorite compiler. We are using the GCC compiler in this example.
Compile the program.
$ gcc function-return-pointer.c
Run the program.
Test Case 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter the desired size for array(1-100): 10 value of ptr[0]=35 value of ptr[1]=56 value of ptr[2]=53 value of ptr[3]=60 value of ptr[4]=92 value of ptr[5]=88 value of ptr[6]=75 value of ptr[7]=53 value of ptr[8]=54 value of ptr[9]=15 $ |
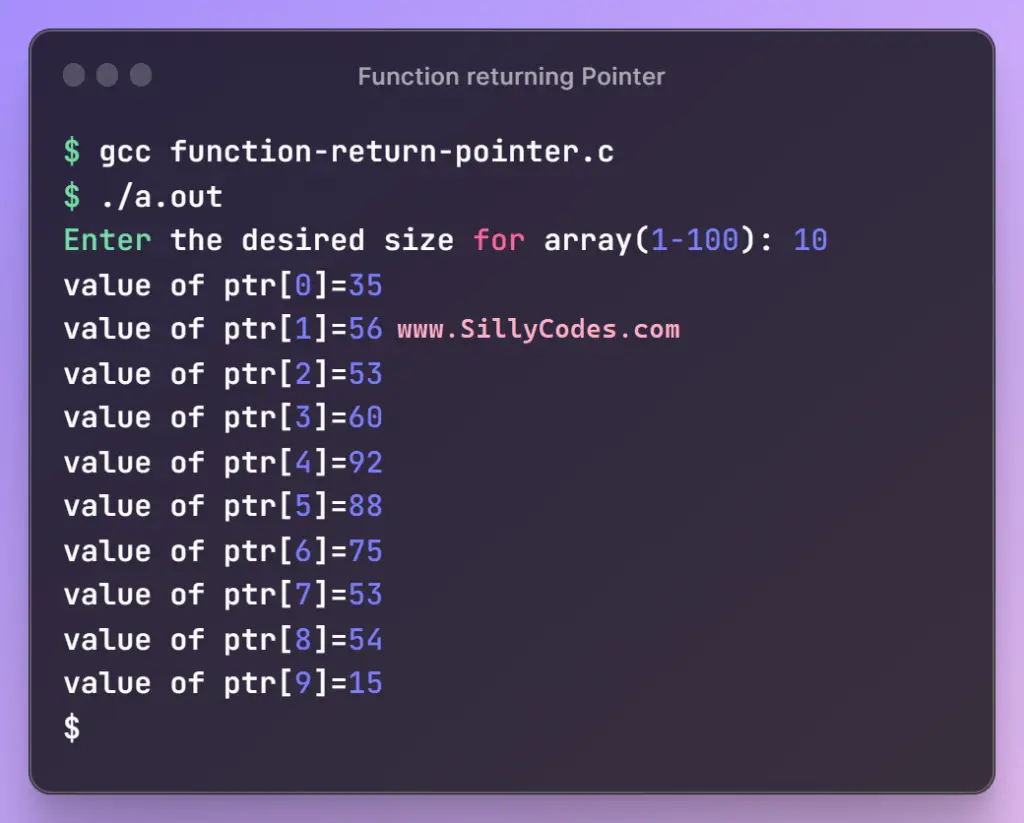
The user entered the desired array size as 10 and the program generated an array with ten random elements.
Let’s look at another example.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter the desired size for array(1-100): 5 value of ptr[0]=77 value of ptr[1]=31 value of ptr[2]=16 value of ptr[3]=27 value of ptr[4]=12 $ |
As expected the generateRandomArray() function generated an array with 5 random numbers, stored them in randArray and returned to main() function as a pointer variable.
Test Case 2:
Let’s look at the boundary checks (i.e Negative cases).
1 2 3 4 5 |
// negative test case $ ./a.out Enter the desired size for array(1-100): 500 Invalid Array Size(500), Please try again $ |
As we have defined the MAXSIZE of the array as 100, The program generates an error message if the number is out of bounds.
#define MAXSIZE 100
Please increase the above MAXSIZE variable to a desired value.
2 Responses
[…] Function Returning Pointer in C Programming Language […]
[…] size_t is an unsigned integer type. So The malloc() function allocates the size number of bytes and returns a pointer to the allocated block of memory. The returned pointer is a void pointer, so we need to typecast it […]