Pointers and Arrays in C Language with Example Programs
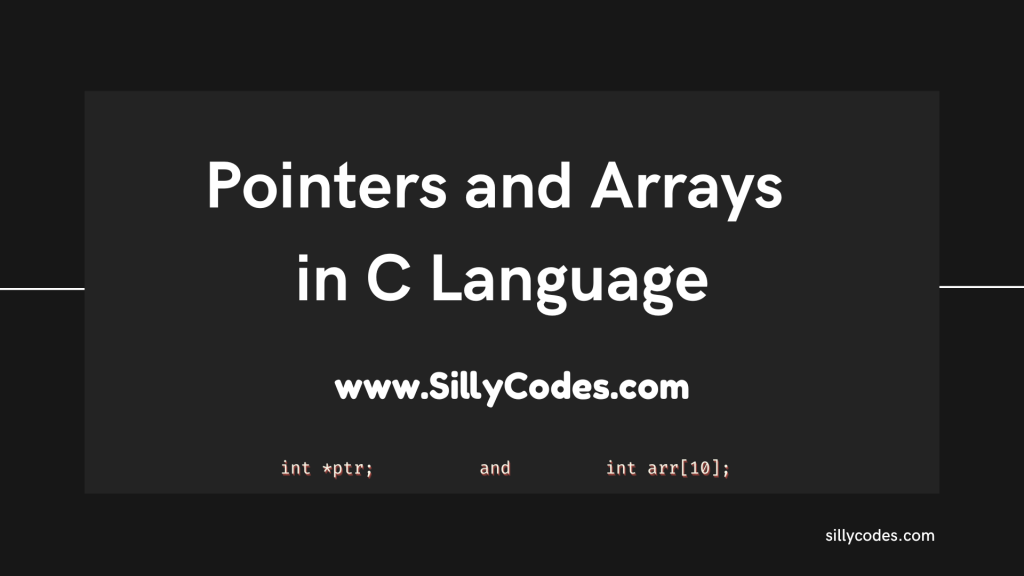
Introduction:
We have looked at the Introduction to pointers and pointer to pointer variable in earlier posts. In today’s article, We will look at the relationship between the pointers and arrays in c programming language.
Arrays in C Langauge:
As we have discussed in the arrays article, The C Array is a collection of data items of a similar datatype. The Array elements are stored in the contiguous memory.
For example, Let’s create an array named cars that store the information of 5 cars.
int cars[5] = {20, 50, 80, 90, 110};
The First element( 20) of the array will be stored at Zeroth Index and the second value( 50) at the 1st index, and so on, The last value is stored at the n-1th index. (where n is the size of the array).
To access the elements of the array we use the subscript [ ] operator with the element index.
So we can say,
cars[0] = 20
cars[1] = 50
cars[2] = 80
cars[3] = 90
cars[4] = 110
How Arrays in C programming stored in the Memory:
Now, Let’s look at how the arrays are stored in the memory.
int cars[5] = {20, 50, 80, 90, 110};
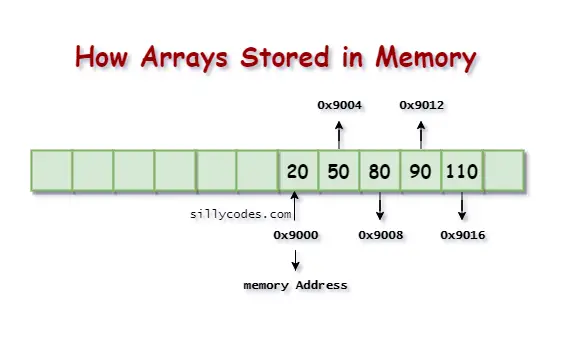
Let’s say the array’s starting memory address is 0x9000 and the integer size is 4 Bytes.
Then the first element of the array(cars[0]) will be stored at the memory address 0x9000, the Second element will be stored at the 0x9004, similarly, all other elements will be stored in the consecutive memory address. The final element will be stored at the index 0x9016 memory address.
cars[0] = 0x9000
cars[1] = 0x9004
cars[2] = 0x9008
cars[3] = 0x9012
cars[4] = 0x9016
Let’s look at the program to get the values and addresses of the array elements.
Program to get the values and address of the array elements:
We use the address of operator(&) to get the address of array elements (&marks[i]).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
/*     Program to get array element values and addresses     sillycodes.com */  #include <stdio.h>  // Size of Array #define SIZE 5  int main() {      // create an array     int marks[SIZE] = {77, 55, 44, 88, 99};      printf("Get Array Elements Values and Addresses\n");      // Iterate over the array     for(int i=0; i<SIZE; i++)     {         // print array element and address         printf("Value of marks[%d]=%d and Address of &marks[%d]=%p\n", i, marks[i], i, &marks[i]);     }      return 0; } |
Program Output:
Let’s compile and run the program.
Compile the program.
$ gcc array-values-addrs.c
Run the executable file.
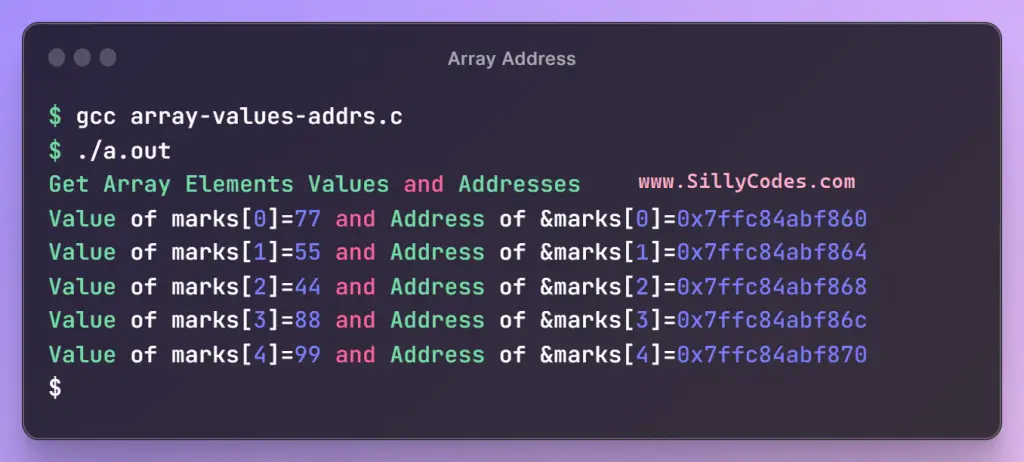
If you observe addresses carefully, The array elements are stored in contiguous memory and each element occupied 4 Bytes.
Now, We know how arrays are stored in memory. Let’s go ahead and see how arrays and pointers are related in the C programming language.
Pointers and Arrays in C:
We can get the address of the array by using the address of operator (&) with the first element of the array ( arr[0])
1 2 3 4 |
int scores[5]; Â // pointer int *ptr = &scores[0]; |
Similarly, We can get the address of the second element in the array using the &scores[1], and so on so.
In C Programming language, The arrays and The pointers are closely related and an array name can be used as a pointer to the first element of the array.
As the array name also holds the memory address of the first element of the array. So we directly assign the array name to the pointer variable to store the address of the array.
1 2 3 |
int scores[5] = {60, 70, 80, 90, 100}; Â int *ptr = scores; |
So the ptrwill point to the first element in the array (i.e 0th Index element) and the ptr+1 will point to the second element in the array ( 1st Index), and so on and so.
📢As we discussed in the pointers arithmetic tutorial, The ptr+1 will give us the next element in the list.
In summary,
ptr points to the address of the 0th element in the array – i.e &scores or &scores[0]
ptr+1 points to the address of the 1st element in the array – i.e &scores[1]
ptr+2 points to the address of the 2nd element in the array – i.e &scores[2]
ptr+3 points to the address of the 3rd element in the array – i.e &scores[3]
ptr+4 points to the address of the 4th element in the array – i.e &scores[4]
We can get the value of the array element pointed by the pointer ptr by using the dereference operator/indirection operator ( *) like below.
To get the value of the 0th element use *ptr – i.e 60
To get the value of 1st element use *(ptr+1) – i.e 70
Similarly, To get the value of 2nd element use *(ptr+2) – i.e 80
To get the value of 3rd element use *(ptr+3) – i.e 90
To get the value of 4th element use *(ptr+4) – i.e 100
We can also use the subscript notation [ ] like below to get the array values using the pointer variable.
*(ptr+1) = ptr[1]
*(ptr+2) = ptr[2]
…
…
*(ptr+i) = ptr[i]
Let’s look at the program to understand the relationship between the arrays and pointers in the c programming language.
Accessing Array elements using Pointer in C Language – Array and Pointers in C:
In the following program, we are going to access the integer array marks elements using a pointer variable ptr.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
/*     Program to understand pointers with Arrays     sillycodes.com */  #include <stdio.h>  // Size of Array #define SIZE 5  int main() {      // create an array     int marks[SIZE] = {77, 55, 44, 88, 99};      // create a pointer and store address of marks     int *ptr = marks;      // use pointer to get values of array     for(int i=0; i<SIZE; i++)     {         // use *(ptr+i) to get the values of array elements         printf("Value of marks[%d]=%d \n", i, *(ptr+i));     }      return 0; } |
Program Output:
Let’s compile and run the program using GCC.
1 2 3 4 5 6 7 8 |
$ gcc array-pointers.c $ ./a.out Value of marks[0]=77 Value of marks[1]=55 Value of marks[2]=44 Value of marks[3]=88 Value of marks[4]=99 $ |
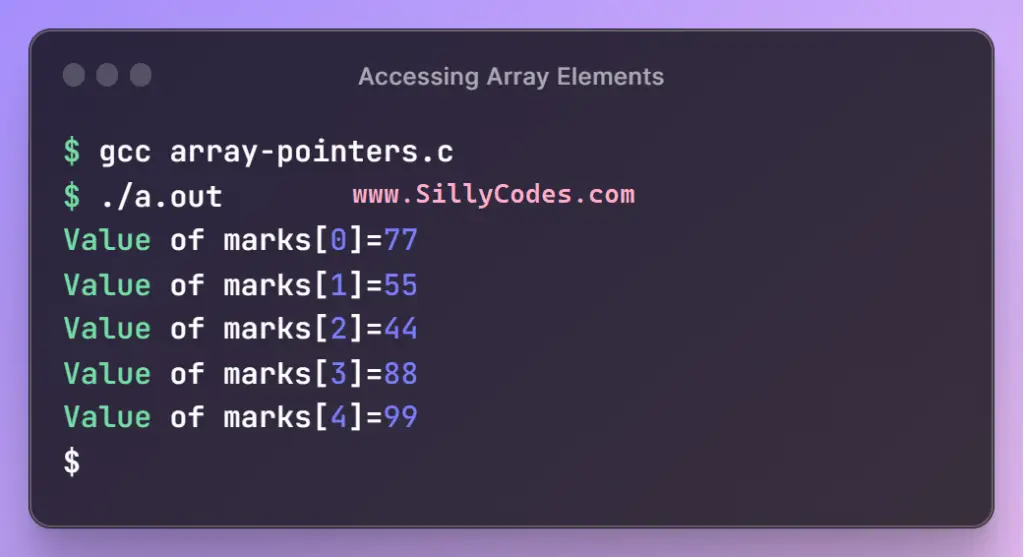
As we can see from the above program and output, We started the program by creating an integer array named marks, Then we stored the address of the marks array in the integer pointer ptr.
int *ptr = marks;
Note that, We used the marks array. As the array name also holds the memory address of the first element of the array.
Then we used a for loop to iterate over the array elements and printed the elements using the pointer ptr with Index(i) (i.e *(ptr+i))
Get Array Values using the Pointer subscript notation:
Similarly, We can also use the pointer variable with the subscript notation [ ] to get the array elements. Here is the program to demonstrate the same.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/*     Program to understand pointers with Arrays     sillycodes.com */  #include <stdio.h>  // Size of Array #define SIZE 5  int main() {      // create an array     int marks[SIZE] = {77, 55, 44, 88, 99};      // create a pointer and store address of marks     int *ptr = marks;      printf("Get Array Elements using Pointer Subscript Notation\n");         // use pointer to get values of array     for(int i=0; i<SIZE; i++)     {         // We can also use subscript notation ptr[i]         printf("Value of marks[%d]=%d \n", i, ptr[i]);     }      return 0; } |
Program Output:
Here is the output of the program.
1 2 3 4 5 6 7 8 9 |
$ gcc array-pointers-subscript.c $ ./a.out Get Array Elements using Pointer Subscript Notation Value of marks[0]=77 Value of marks[1]=55 Value of marks[2]=44 Value of marks[3]=88 Value of marks[4]=99 $ |
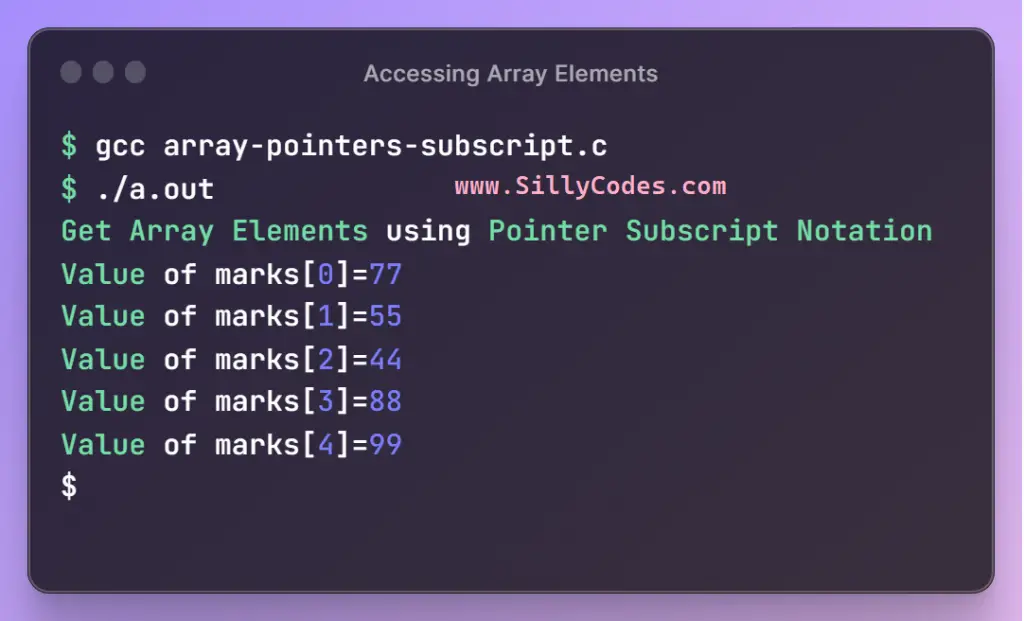
As we can see from the above output, we are able to get the array elements values using the subscript notation(ptr[i])
Passing An array to function using a pointer:
Let’s look at how to pass an array as a pointer to a function. The function should accept the input array as a pointer variable, which means the function’s formal argument should be a pointer variable.
We have defined a function called printArray to print the array elements. The printArray() function takes two formal arguments, an integer pointer *scorePtr and array size size (We can also use the SIZE macro as well).
void printArray(int *scorePtr, int size)
The printArray() function uses a for loop to traverse through the array and prints the array elements using the scorePtr pointer variable. ( We are using the pointer with a subscript notation to access the array elements).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     Program pass array to function using pointers in C.     www.sillycodes.com */  #include <stdio.h>  // Size of Array #define SIZE 5  /** * @brief  - print array pointed by 'scorePtr' pointer * * @param scorePtr - 'scorePtr' integer pointer * @param size  - size of array pointed by 'scorePtr' pointer */ void printArray(int *scorePtr, int size) {     printf("Array elements are : \n");      // Iterate over the array using '*scorePtr' pointer     for(int idx=0; idx<SIZE; idx++)     {         // print array element using subscript notation         printf("Value of scorePtr[%d]=%d\n", idx, scorePtr[idx]);     }      return; }  int main() {      // create an array     int score[SIZE] = {108, 154, 99, 212, 38};      // call printArray() with 'score' array and SIZE     printArray(score, SIZE);      return 0; } |
Finally, Call the printArray() function from the main() Function and pass the scorearray and its size(i.e SIZE).
Program Output:
Compile the program.
$ gcc passing-array-to-func.c
The above command generates the executable file named a.out. Run the executable file using ./a.out command.
1 2 3 4 5 6 7 8 |
$ ./a.out Array elements are : Value of scorePtr[0]=108 Value of scorePtr[1]=154 Value of scorePtr[2]=99 Value of scorePtr[3]=212 Value of scorePtr[4]=38 $ |
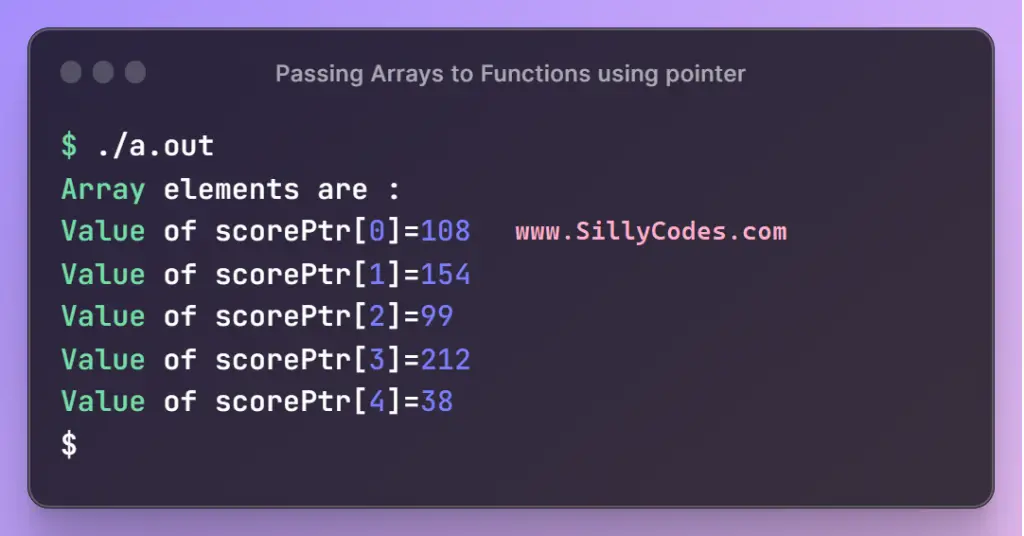
As you can see from the above output, The printArray() function printed the array elements on the console.
4 Responses
[…] pointer in c programming language. As we have discussed earlier in the introduction to pointer and pointers and array articles, We can return a pointer variable from a function in C language. We are going to look at […]
[…] an earlier article, we discussed the relationship between pointers and arrays. In today’s article, We will look at the pointer to array in c programming […]
[…] Pointers and Arrays in C […]
[…] Pointers and Arrays in C Language with Example Programs […]