Bitwise OR Operator in C Programming Language
- Introduction:
- Bitwise OR Operator in C Language:
- Truth Table of Bitwise OR Operator:
- How Bitwise OR ( ‘|’ ) Operator in C Works? :
- Example Program : Bitwise OR Operator in C:
- Bitwise OR Example 2:
- Example Program 2: Bitwise OR Operator:
- Difference between the Logical OR and Bitwise OR Operators in C:
- Program: Bitwise OR Vs Logical OR operator:
- Set Nth Bit using the Bitwise OR operator
- Set Nth bit Program in C Language:
- Few Quick Tips on Bitwise OR Operator:
- Conclusion:
- C Tutorials Home:
- Related C Programs:
- Related Operators Tutorials:
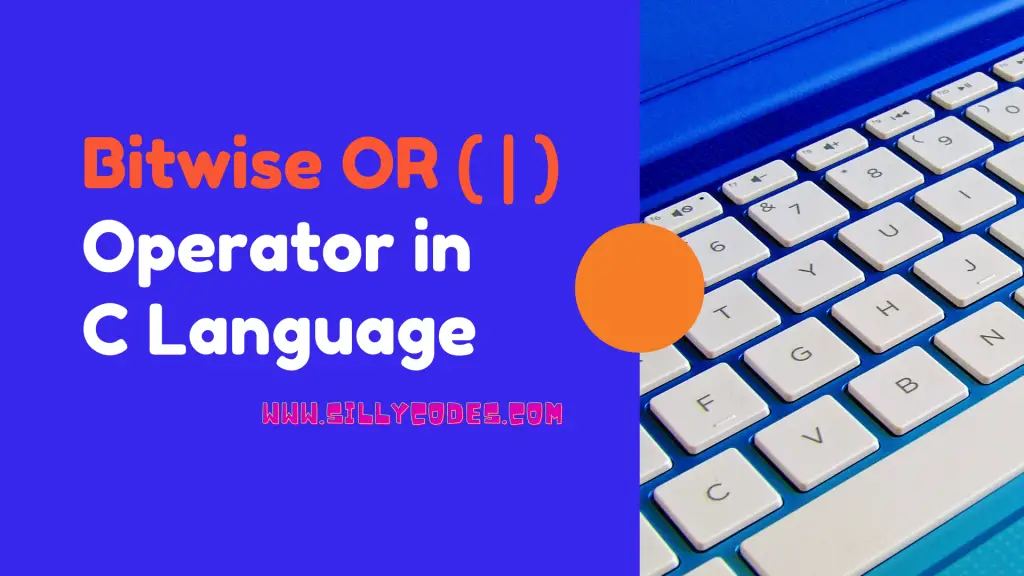
Introduction:
In the previous articles, We have discussed what are Bitwise Operators and Bitwise AND Operator. In today’s article, we will learn about the Bitwise OR operator in C Programming Language.
📢 This Article is part of the Bitwise Operators Series
Bitwise OR Operator in C Language:
The Bitwise OR operator is Binary Operator and Takes two input values ( Binary sequences of two values ) and performs the Bitwise OR on each pair of bits in the given binary sequence.
In the C Programming Language, The bitwise OR operator is denoted by the Vertical Bar or Vertical Line |.
The result of Bitwise OR operation is False(0) only if both of the input bits are False(0).
Bitwise Operator returns True(1), When at least one of the input bits are True(1)
Bitwise OR operator can be applied on Integer data values only. We can’t apply bitwise OR to the Floating point data.
Truth Table of Bitwise OR Operator:
Input bit 1 ( Xi ) | Input bit 2 (Yi) | Result ( Xi | Yi ) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
Here Xi and Yi are the Pair of bits in the Binary sequence of X and Y values.
How Bitwise OR ( ‘|’ ) Operator in C Works? :
Let’s go through a step-by-step example, We will take two numbers X=6 and Y=10.
Now we need to apply the Bitwise OR operator on variables X and Y. i.e X | Y.
To calculate the bitwise OR operator, First of all, we need to convert the values of X and Y into the Binary form or Base-2 form.
➡️ For making calculations easy, We are going to take only 8 bits for the integer value. But note that the Integer value will be stored in 32 bits in the memory.
The Binary Equivalent of the X or 6 is 0000 0110.
And Binary Equivalent of the Y or 10 is 0000 1010.
- Here is a C Program to convert an Integer number to the Binary sequence – Decimal to Binary conversion program in C (sillycodes.com)
The Left-most bit in the binary representation is MSB or Most significant bit and the Right-most bit is the LSB or Least Significant bit.
Now we need to apply the Bitwise OR operation on each pair of bits.
Here Pair of bits means, MSB in the first number X and MSB in the second number Y, So on until we reach the LSB of X and LSB of Y.
➡️ Bitwise X | Y is:
X –> 0000 0110 (6 in Binary)
Y –> 0000 1010 (10 in Binary)
X | Y –> 0000 1110 ( X | Y = 14 )
So the result of the bitwise OR operation of X and Y ( 6 | 10 ) is 14.
Here is the pictorial representation of the above Bitwise OR operation.
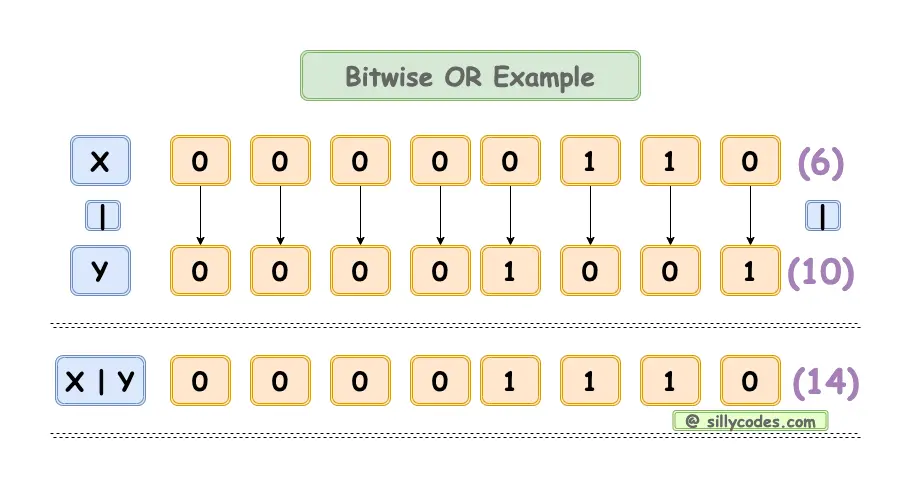
As we can see from the above graphical representation, The Bitwise OR operator perform on the Bit-Level. So only if both bits are False or Zero the resulting bit will be Zero.
So above problem X | Y or 6 | 10 is 14.
Let’s see a program to use the bitwise OR operator.
Example Program: Bitwise OR Operator in C:
We are going to apply the bitwise OR operator on the above values 6 and 10.
1 2 3 4 5 6 7 |
#include <stdio.h> int main() { // The bitwise OR of '6' and '10' is printf("The 6 | 10 is : %d \n", 6 | 10 ); return 0; } |
Program Output:
1 |
The 6 | 10 is : 14 |
As you can see the output of 6 & 10 is 14.
Bitwise OR Example 2:
Let’s look at one more example. Will take two numbers A=16 and B=15.
The Bitwise OR of A and B ( A | B ) is 31.
As we discussed earlier, We need to convert the given A and B values into the Binary representation.
Binary Equivalent of the variable A ( i.e 16 ) is 0001 0000
Similarly, The binary equivalent of variable B(i.e 15) is 0000 1111
Now we need to apply the Bitwise OR operator on variables A and B i.e A | B.
➡️ Bitwise A | B is:
A –> 0001 0000 (16 in Binary)
B –> 0000 1111 (15 in Binary)
A | B –> 0001 1111 ( A | B = 31 )
Here is the pictorial representation of above Bitwise OR calculation.
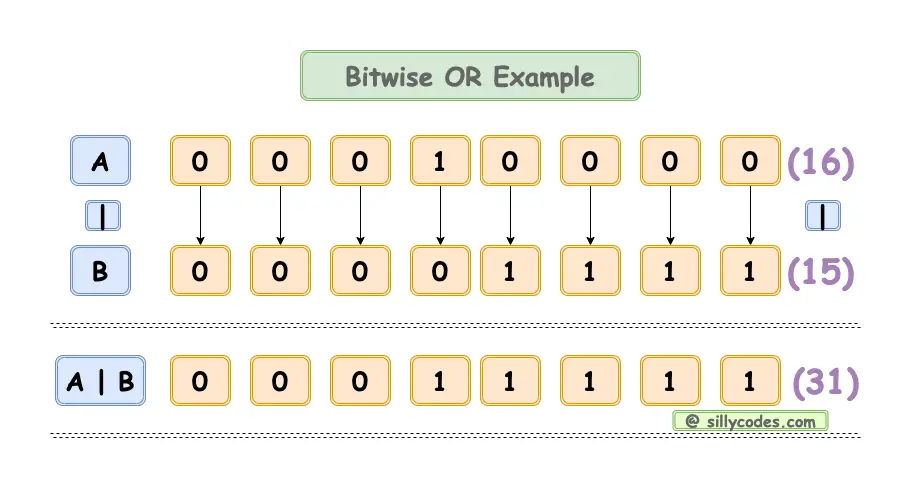
If any one of the input bits are True(1), Then the resulting bit will be 1.
let’s verify the above bitwise OR calculations using the C program.
Example Program 2: Bitwise OR Operator:
Applying the bitwise OR operation on values 16 and 15
1 2 3 4 5 6 |
#include <stdio.h> int main() { printf("The 16 | 15 is : %d \n", 16 | 15 ); return 0; } |
Program Output:
1 |
The 16 | 15 is : 31 |
Difference between the Logical OR and Bitwise OR Operators in C:
Don’t confuse with the bitwise OR with the Logical OR operator, The Logical OR Operator is denoted with Two Vertical Bars ( || ), While the Bitwise OR is denoted with a single Vertical bar ( | ) symbol.
Let us take an example to understand the difference between these operators.
Take two variable A and B and their values are A = 20 and B = 10.
Logical OR ( || ) Operator:
A || B ;
20 || 10 ;
The result is 1
The output of the above expression is one(1), Because both of the inputs ( 10, 20) are non-zero. So the output of the logical OR operation is One(1).
📢 Learn More about Logical Operators Here – Logical operators in C language ( &&, ||, and ! ) usage and Examples (sillycodes.com)
Bitwise OR ( | ) Operator:
A | B ;
20 | 10 ;
But unlike Logical OR, The Bitwise OR operates on Bit-Level, So we need to look at the binary representations of A and B. Then apply the Bitwise OR operation.
Here A and B are integer data. The size of the integer is 4 bytes i.e 32 bits (Let’s take full 32 bits for this example).
We need to apply the Bitwise OR on these 32 bits sequences of A and B ( because bitwise OR Operates on bits).
A ( 20 ) Binary Representation is 0000 0000 0001 0100
B ( 10 ) Binary Representation is 0000 0000 0000 1010
The bitwise OR of the above Example A | B that is 20 | 10
➡️ Bitwise A|B is:
0000 0000 0001 0100 ( A = 20 )
& 0000 0000 0000 1010 ( B = 10 )
————————
0000 0000 0001 1110 ( A|B = 30 )
————————
The result of A|B is 30
From the above example, It is clear that The Bitwise OR Operator works on the Bit-Level. While the Logical OR works on the Byte-Level.
Program: Bitwise OR Vs Logical OR operator:
The following program is going to demonstrate the differences between the Logical and bitwise OR operators.
Please go through the comments of the program for more details.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
/* Program to demonistrate the Difference between the Logical OR and Bitwise OR Logical OR Vs Bitwise OR */ #include <stdio.h> int main(void) { // Take two numbers int A,B; // I am assigning values A=20 and B=10 A=20; B=10; // To get the Logical OR of variable 'A' and Variable 'B', Use Logical OR '||' Operator // A || B ( Note we have two Vertical Bars here.) printf("Logical OR of A and B is %d || %d : %d \n", A, B, A||B ); // To get the Bitwise OR of variable 'A' and Variable 'B', Use Bitwise OR '|' Operator // A | B ( Note we have Only one Vertical Bar here.) printf("Bitwise OR of A and B is %d | %d : %d \n", A, B, A|B ); return 0; } |
- Use Double Vertical Bar for Logical OR Operation – A || B
- Use single Vertical Bar for Bitwise OR Operation – A | B
Program output:
1 2 |
Logical OR of A and B is 20 || 10 : 1 Bitwise OR of A and B is 20 | 10 : 30 |
Set Nth Bit using the Bitwise OR operator
One of the uses of the bitwise OR operator is, It can be used to set the bit at the specified position.
Setting the Nth bit means, We are going to make the Nth bit as One(1).
Set Nth bit Logic :
The Setting the Nth bit means, If the Nth bit is 0, Then by using the set bit logic we can convert the Nth bit value from Zero(0) to One(1).
If the Nth bit already has the value One(1), Then Set bit logic won’t have any effect on the bit.
To Set the Nth bit, Use the following bitwise OR logic in C Language.
1 |
InputNumber |= ( 1 << bitPosition ) |
Here,
The
InputNumber is the number where we are setting the bit
The
bitPosition is the position of the bit (Nth bit) in the binary representation of
InputNumber
Set Nth Bit Example:
Let’s take a Number, InputNumber = 431. The binary equivalent of 431 is 110101111.
Now, let’s say you want to set the bit at position ‘4’ of above value 431.
📢 Here position will start from the end i.e from the Least significant bit (LSB). And position value starts with Zero(0) and up to the length of the Integer. So here we are trying to set the bit at the position 3, So it will be the 4th bit from the end.
To Set the 4th position bit of number 431, Use the below clear bit logic.
1 |
InputNumber |= (1 << 4) |
After this operation, The 4th position of the InputNumber(431) will be converted into 1. And the InputNumber becomes 447.
The binary sequence of 447 is 110111111
If you observe closely, The 4th position bit ( i.e 5th bit from the end) is Set or Modified to 1.
Let’s convert this into a C program.
Set Nth bit Program in C Language:
We are going to use the above set bit logic. The program is going to take the inputNumber from the user and The program also asks the user to provide the bitPosition to Set.
Once we got the user input, We will apply the above Set bit logic to Set the bit at the specified position.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
#include <stdio.h> int main() { int inputNumber; int bitPosition; // Take the 'inputNumber' from the User printf("Enter a Number : "); scanf("%d", &inputNumber); // Take the 'bitPosition' to Set from the user printf("Bit Position to Set : "); scanf("%d", &bitPosition); // Apply the 'Set Nth bit' logic on given 'inputNumber' inputNumber |= ( 1 << bitPosition ); // Print the Results after Setting the bit printf("Resulting Number after Setting the bit at %d position is : %d \n", bitPosition, inputNumber); return 0; } |
Program Output:
1 2 3 |
Enter a Number : 431 Bit Position to Set : 4 Resulting Number after Setting the bit at 4 position is : 447 |
As you can see from the above output, We got the desired output of 447.
The program set the bit at the 4th index from the end to 1. Which increased the value of inputNumber from 431 to 447.
Re-Run the above program with other examples and see the results.
Here is the output of the program for different input values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
venkey@ubuntu$ ./a.out Enter a Number : 0 Bit Position to Set : 4 Resulting Number after Setting the bit at 4 position is : 16 venkey@ubuntu$ venkey@ubuntu$ ./a.out Enter a Number : 0 Bit Position to Set : 1 Resulting Number after Setting the bit at 1 position is : 2 venkey@ubuntu$ venkey@ubuntu$ ./a.out Enter a Number : 0 Bit Position to Set : 0 Resulting Number after Setting the bit at 0 position is : 1 venkey@ubuntu$ venkey@ubuntu$ ./a.out Enter a Number : 10 Bit Position to Set : 6 Resulting Number after Setting the bit at 6 position is : 74 venkey@ubuntu$ ./a.out Enter a Number : 20 Bit Position to Set : 4 Resulting Number after Setting the bit at 4 position is : 20 venkey@ubuntu$ ./a.out Enter a Number : 762 Bit Position to Set : 2 Resulting Number after Setting the bit at 2 position is : 766 venkey@ubuntu$ ./a.out Enter a Number : 765432 Bit Position to Set : 14 Resulting Number after Setting the bit at 14 position is : 781816 venkey@ubuntu$ venkey@ubuntu$ |
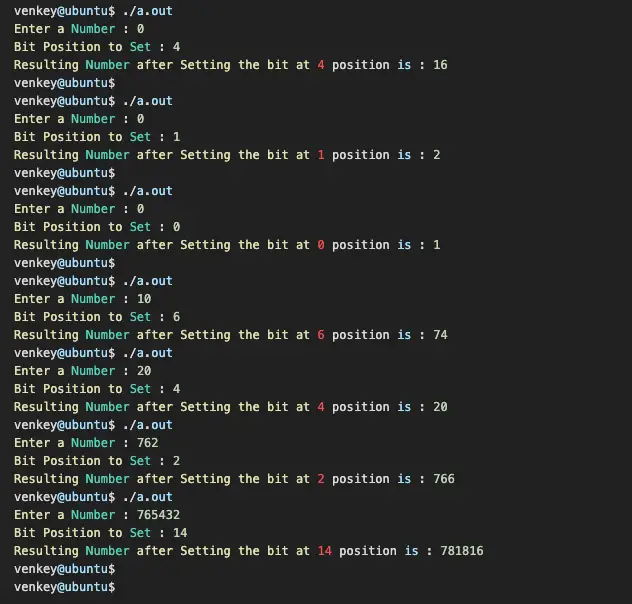
Few Quick Tips on Bitwise OR Operator:
Bitwise OR operator with Zero :
If you apply the bitwise OR on a Number(X) with the Zero, Then the result will the Number(X).
Bitwise OR with Zero Example program:
1 2 3 4 5 6 |
#include <stdio.h> int main() { printf("Result is : %d \n", 0 | 44); return 0; } |
Output:
1 |
Result is : 44 |
As you can see Applying the bitwise OR on the Zero(0) and 44, resulting as 44.
Bitwise OR Operator with Negative One (-1) :
If we apply the Bitwise OR on any value and the -1, Then the result will be always -1
This is due to the fact that Negative One (-1) is represented as the all Ones in Binary format. So applying the bitwise OR with all 1’s will results in all 1’s. Which is Negative One(-1).
Negative One (-1) in Binary – 1111 1111 1111 1111
Let’s look at an example program.
Bitwise OR with Negative One(-1):
1 2 3 4 5 6 |
#include <stdio.h> int main() { printf("Result is : %d \n", -1 | 431); return 0; } |
Output:
1 |
Result is : -1 |
Explanation:
The Bitwise OR of values -1 and 431 is -1, Because the binary Equivalent of -1 is all ones. Please look at the following explanation.
➡️ Bitwise OR ( -1 | 431 )
-1 = 1111 1111 1111 1111 (Binary Equivalent)
431 = 0000 0001 1010 1111 (Binary Equivalent)
Result = 1111 1111 1111 1111 ( -1 | 431 = -1 )
So Bitwise OR of –1 and any value results in -1.
Few More examples:
-1 | 1000 –> -1
-1 | 1 –> -1
321 | -1 –> -1
Conclusion:
In this article, We have discussed the Bitwise OR operator and the Truth table of Bitwise OR. How the bitwise OR operator works in C language and also looked at a few examples and programs.
Related Articles:
- https://sillycodes.com/bitwise-and-operator-in-c-programming/
- https://sillycodes.com/bitwise-xor-operator-in-c-language/
C Tutorials Home:
- Start Here – Step by step tutorials to Learn C programming Online with Example Programs – SillyCodes
- Operators in C Language | Different type of Operators in C language (sillycodes.com)
5 Responses
[…] Bit-wise OR ( | ) […]
[…] Bit-wise OR ( | ) […]
[…] 📢 Bitwise OR ( | ) Operator in C programming Language – SillyCodes […]
[…] the previous articles, We have discussed what are Bitwise Operators and Bitwise AND Operator, and Bitwise OR operators. In today’s article, we will learn about the Bitwise XOR operator in C […]
[…] Bitwise OR Operator ( | ) […]