Bitwise Right Shift Operator in C Programming Language ( >> )
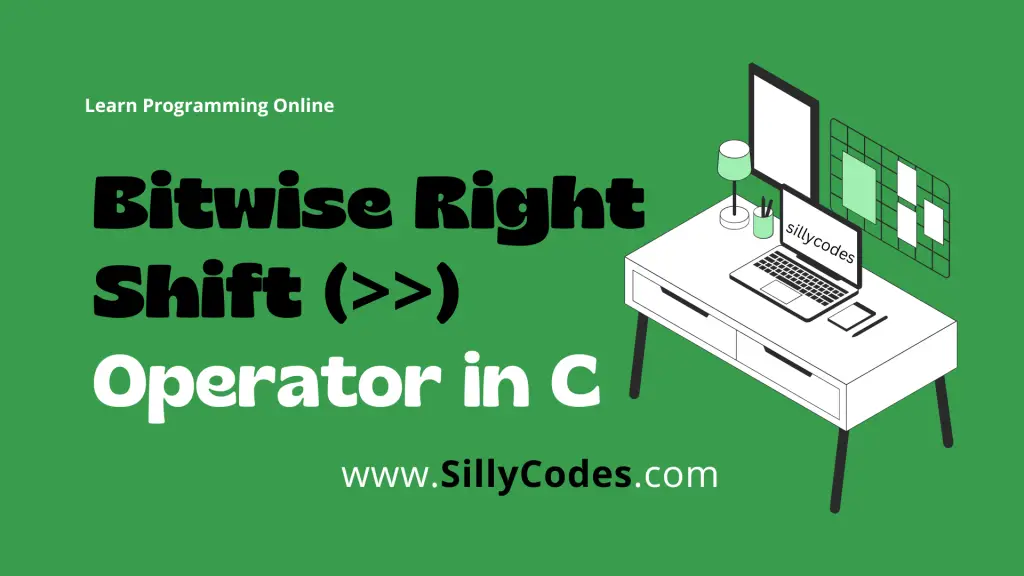
Introduction:
We have looked at the Bitwise XOR Operator in our earlier articles, In today’s article, we are going to look at the Bitwise Right Shift Operator in C language with Example Programs.
Bitwise Right Shift Operator in C ( >> ):
The Bitwise Right Shift Operator is used to move(shift) the specific number of bits in binary sequence in the right direction.
Here is the syntax of the Bitwise Right shift operator:
number >> number_of_positions_to_shift
Here
- number is the first operand.
- number_of_positions_to_shift is the second operand.
- The >> is the Bitwise right shift operator.
The Bitwise Right Shift Operator ( >> ) shifts the number(Binary sequence) right side by a number of positions given in the second operand ( number_of_position_to_shift).
📢 This program is part of Bitwise Operator in C language series.
Example to Understand the Bitwise Right Shift Operator:
Let’s take an example.
Example 1:
Shift one bit on the right side using the right shift operator.
20 >> 1
Here we are trying to right-shift the number 20 by one (1). As the bitwise operators work on the bit level, First we need to convert the decimal number 20 into the binary sequence.
The binary equivalent of the number 20 is 00010100
Now we need to shift the above binary sequence on the right side by one.
00010100 >> 1
which is equal to – 00001010 – Decimal Value 10.
We simply moved all bits to the right side once. Filling the 0 bit at the left side.
📢 So In Nutshell, the Right shift operator is shifting the first operand’s bits right side by the number of positions given in the second operand.
Here is the graphical representation of the above operation.
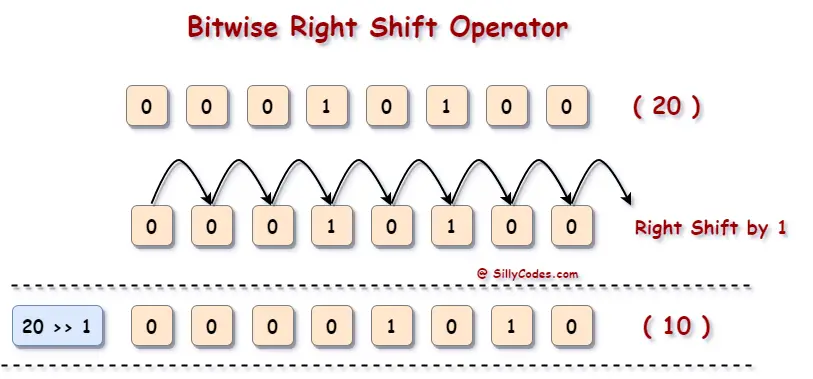
If you notice the final output, All the bits are right-shifted once.
Let’s look at another example.
Example 2:
Let’s shift the two bits right side by using the bitwise right shift operator.
24 >> 2
So above expression, Right Shifts the binary sequence of the number 24 by two positions.
The binary Equivalent of 24 is 00011000
Let’s shift two bits of the above sequence to the right side.
00011000 >> 2
Which is equal to 00000110 – Decimal value 6.
If you observe carefully, All the bits are moved right side by two times. And the Left side bits are filled with zeros.
Let’s look at the graphical representation of the above bitwise right shift operation. ( 24 >> 2)
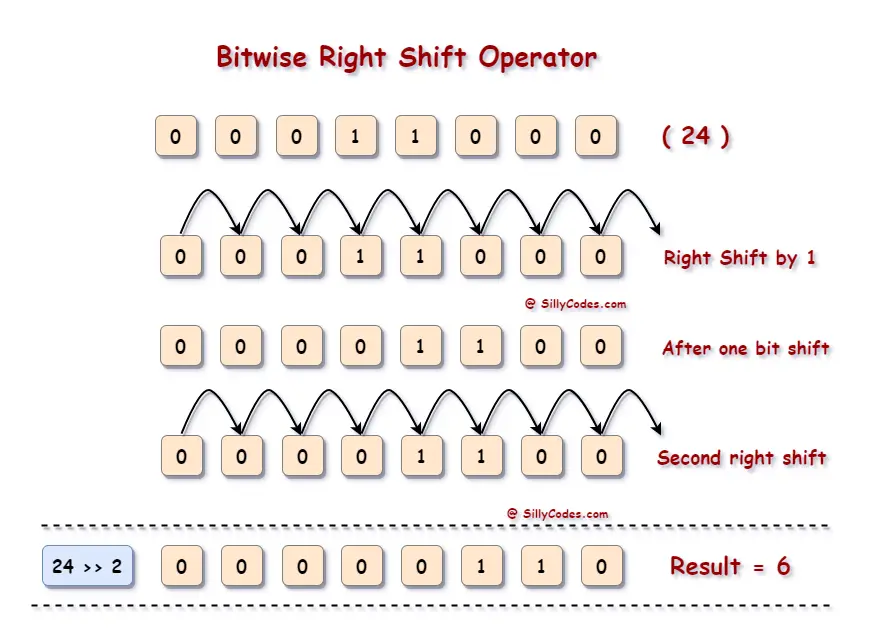
Uses of Bitwise Right Shift Operator ( >> ):
If you observe the above two examples, Whenever we right-shift the number by one bit, The Original number is reduced and becomes exactly half (as we shifted only once).
In the first example, The 20 >> 1, became 10, which is 20/2 = 10.
In the second example, The 24 >> 2 became 6, which is equal to 24/22 = 24/4 = 6.
Similarly, If you shift 40 >> 2, Which will be equal to 10. (40/22 = 40/4 = 10)
📢 So whenever we shift the number, ‘n’ bits(positions) on the right side, The Original number reduces by number / (2n)
So whenever you want to reduce a number in (2n) terms, You can use the bitwise Right shift operator. As these operations are performed at bit-level, These operations are performed fast in C language.
We can also use Bitwise operators to SET a Bit, CLEAR a bit, and Invert a Bit. We are going to look at these examples in our upcoming articles.
Program to Understand the Bitwise Right shift operator in C language:
Let’s write a program to see how we can use the bitwise right shift operator in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* Program to understand bitwise right shift operator in C */ #include<stdio.h> int main() { // If you right shift the number 32 by 1 printf("32>>1 is equal to %d \n", 32 >> 1); // Right shift 20 by two positions printf("20>>2 is equal to %d \n", 20 >> 2); // Right shifting 128 by 4 bits printf("128>>4 is equal to %d \n", 128 >> 4); // Right shift 1024 by 8 bits printf("1024>>8 is equal to %d \n", 1024 >> 8); return 0; } |
Program Output:
We are using the GCC compiler to compile the program.
1 2 3 4 5 6 7 |
$ gcc right-shift.c $ ./a.out 32>>1 is equal to 16 20>>2 is equal to 5 128>>4 is equal to 8 1024>>8 is equal to 4 $ |
Bitwise Right shift Program in C Explanation:
We have performed four Bitwise Right shift operations in the above program. Let’s look at them one by one.
32 >> 1:
The first right shift operation is 32 >> 1.
As we discussed earlier, Whenever we right-shift the bits of any number by one position. The number becomes half ( number / 21 ). So here also the number 32 became half, which is 16.
Here is the step-by-step calculation.
32 >> 1
32 / 2^1
32 / 2
16
20 >> 2:
In the second right shift operation, We looked at 20 >> 2.
So the number will be reduced to one-fourth of the original number ( number / 22) which is equal to 5.
Here is the step-by-step calculation
20 >> 2
20 / 2^2
20 / 4
5
128 >> 4:
In the Third right shift operation, we right-shifted the number 128 by 4 positions. So the number will be reduced by 24 times.
Here is the step-by-step evaluation.
128 >> 4
128 / 2^4
128 / 16
8
1024 >> 8:
In the last expression, We moved the number 1024 by 8 times in the right side direction. So the number 1024 will be reduced by 28 times.
1024 >> 8
1024 / 2^8
1024 / 256
4
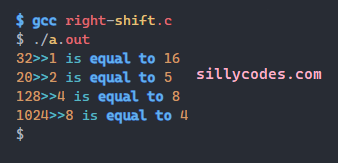
Conclusion:
In this article, We have looked at the bitwise Right shift operator, We also explained how the bitwise Right shift operator works with example programs. In the next tutorial, We are going to look at the Bitwise Left shift operator.
3 Responses
[…] our previous tutorials, We have looked at the Bitwise Right shift and Left shift operators. In today’s article, We are going to look at the Bitwise One’s […]
[…] Bitwise Right Shift Operator ( >> ) […]
[…] Bitwise Right Shift Operator ( >> ) […]