Pointer to array in C Programming Language
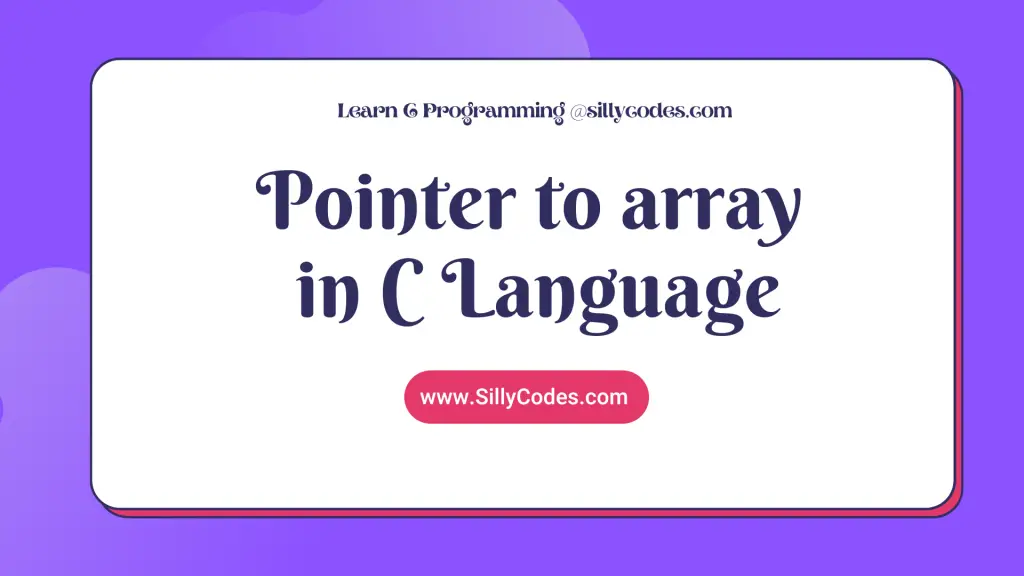
Introduction:
In an earlier article, we discussed the relationship between pointers and arrays. In today’s article, We will look at the pointer to array in c programming language.
📢 Recommended: Pointers Practice Programs series
What is Pointer to Array in C Language:
The C programming language allows us to declare a pointer that points to the entire array. This type of pointer is called Pointer to Array.
In earlier posts, we looked at the pointer to the array which points to the first element of the array.
1 2 3 4 |
int files[5]; Â // Pointer int *ptr = files; |
In the above example, The pointer variable ptr is pointing to the single element of the files array, that is the first element of the array ( 0th Index - files[0] element).
So if you increment the above pointer ptr, It will go to the next element in the array (i.e 1st Index - files[1] element).
Similarly, We can create a pointer variable, that points to the entire array instead of a single element. Let’s look at the syntax of the pointer to array in c.
Syntax of Pointer to Array in C:
data_type (*pointer_name) [size];
Here
- data_type is the pointer datatype.
- pointer_name is the name of the pointer
- size is the size of the array that the pointer going to point to.
The above pointer( pointer_name) can store the address of the array of data_type and size of size.
Let’s look at the example of Pointer to Array in C
Example of Pointer to Array in C Language:
Create an integer array called shares with the size 5.
1 2 |
// Array int shares[5] |
Now let’s create a pointer to an array called arrPtr.
1 2 3 4 5 |
// declare pointer to array int (*arrPtr) [5]; Â // store address of 'shares' in 'arrPtr' arrPtr = shares; |
The arrPtr pointer to an array will point to the entire array of shares[5] (unlike simple pointers). So the arrPtr can store the address of an integer array with 5 elements.
The pointer arithmetic is performed based on the base type of the pointer variable.
So If we increment the pointer to an array( arrPtr), The pointer to an array( arrPtr ) value will be incremented by 5 * Integer Size bytes. ( As the base type of arrPtr is Array of 5 integers)
📢The pointer to an array is used while working with multidimensional arrays.
Let’s look at an example program to understand the pointer to an array in C Programming Langauge.
Program to Understand Pointer to an Array in C Programming Language:
In the following program, We are going to declare an array( shares) with the size of 5 Integers. Then we will create two pointer variables, normalPtr and arrPtr.
The normalPtr is an integer pointer, which points to the 0th index of the shares array.
The arrPtr is a Pointer to an array of 5 integers ( int (*arrPtr)[5] ) and points to the entire array of shares.
To demonstrate that the arrPtr is pointing to the entire shares array, We perform the pointer increment operation on the above two pointers and display the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program to Understand Pointer to an Array in C Programming Language     sillycodes.com */  #include<stdio.h>  int main() {     // declare a array     int shares[5] = {40, 80, 20, 30, 99};      // declare normal pointer     // points to 0th index of 'shares' array     int *normalPtr = shares;      // declare pointer to an array     // points to entire array of 'shares'     int (*arrPtr)[5] = shares;      // print the details of both arrays     printf("value of normalPtr:%p \n", normalPtr);     printf("value of arrPtr:%p \n", arrPtr);      // increment normal pointer ('normalPtr')     normalPtr++;      // increment pointer to an array ('arrPtr')     arrPtr++;      // display the details again.     printf("value of normalPtr:%p (after increment)\n", normalPtr);     printf("value of arrPtr:%p (after increment)\n", arrPtr);      return 0; } |
Program Output:
Let’s compile and run the program. We are using the GCC compiler to compile the C program in this example.
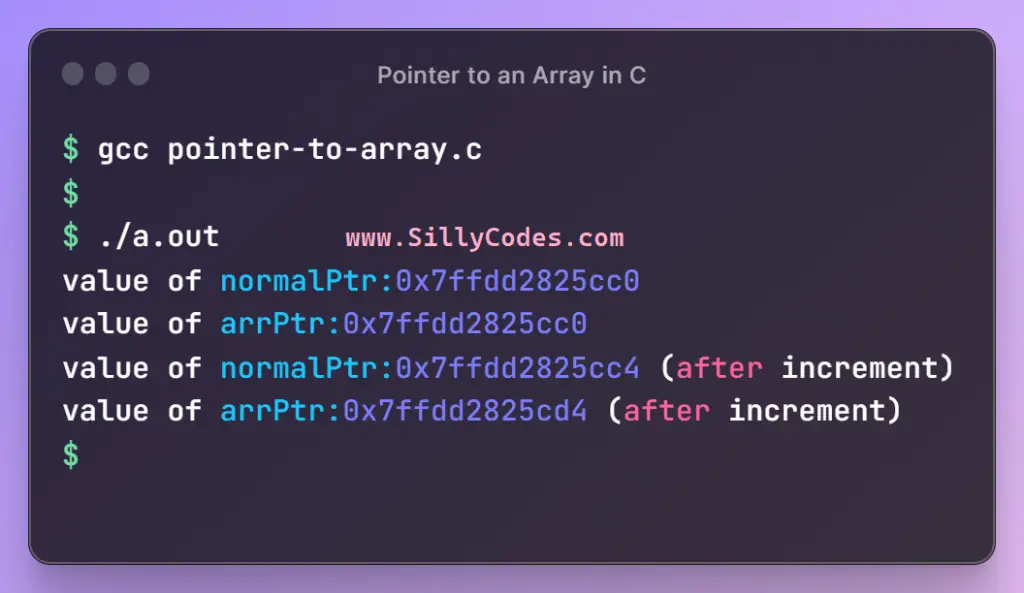
As you can see from the above output, Initially both the normal pointer normalPtr and the pointer to an array ( arrPtr) are pointing to the base address of the array. i.e 0x7ffdd2825cc0
Once we incremented the normal pointer( normalPtr) and the pointer to an array( arrPtr), The values of the pointers are changed.
The normalPtr value is increased by 4 Bytes ( Assuming the size of the integer is 4 Bytes)
But the value of the pointer to an array arrPtr is increased by 20 Bytes. which is equal to the size of the entire shares array ( 5 * 4 Bytes).
📢If we increment the pointer to an array, The value of the pointer to an array will be increased by the size of the entire array.
<strong>Before Increment - arrPtr - 0x7ffdd2825cc0</strong>
arrPtr++;
After Increment - arrPtr - 0x7ffdd2825cd4
Difference – 20 Bytes
📢The Pointers to an array are used extensively to traverse the multidimensional arrays. As we can simply move from one array to another array by performing the arithmetic operations on the pointer to an array.
Dereferencing the Pointer to an Array in C Programming Language.
The normal pointers are pointing to the first element of the array ( zeroth index – shares[0]), If we dereference the pointer, We will get the value of the 0th index element in the array. ( shares[0])
Similarly, The pointer to an array( arrPtr) is pointing to the entire array, so if we dereference the pointer to an array, We will get the array.
Let’s look at a program to demonstrate the dereference operator on a pointer to an array in C Programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/*     Program to Understand Dereference of Pointer to an Array in C     sillycodes.com */  #include<stdio.h>  int main() {     // declare a array     int shares[5] = {40, 80, 20, 30, 99};      // declare normal pointer     // points to 0th index of 'shares' array     int *normalPtr = shares;      // declare pointer to an array     // points to entire array of 'shares'     int (*arrPtr)[5] = shares;      // print the details of both arrays     printf("value of normalPtr:%p \n", normalPtr);     printf("value of arrPtr:%p \n", arrPtr);      // dereference pointers     printf("value of *normalPtr = %d\n", *normalPtr);     printf("value of *arrPtr = %p\n", *arrPtr);      return 0; } |
The normalPtr and arrPtr are storing the address of the shares array. while the normalPtr is a normal integer pointer, The arrPtr is a pointer to an array.
Program Output:
Compile the program.
$ gcc pointer-to-array-derefence.c
Run the program.
1 2 3 4 5 6 |
$ ./a.out value of normalPtr:0x7ffebc06d380 value of arrPtr:0x7ffebc06d380 value of *normalPtr = 40 value of *arrPtr = 0x7ffebc06d380 $ |
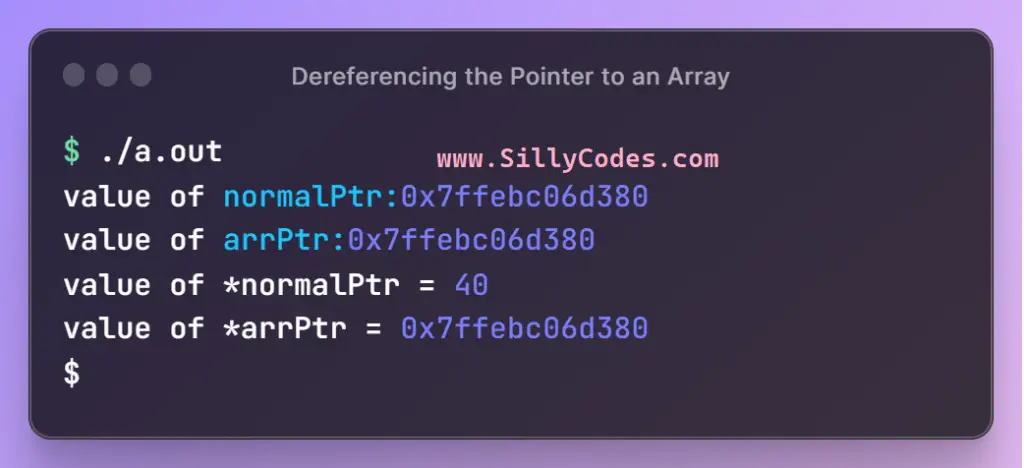
As we can see from the above program output,
- Dereferencing the normal pointer *normalPtr returned the value of the 0th index element of the shares array. that is equal to 40.
- Similarly, If we apply the dereference operator on the pointer to an array *arrPtr, We got the address of the entire array. That is equal to 0x7ffebc06d380 ( value of *arrPtr = 0x7ffebc06d380)
We can further confirm that we got the entire array by using the sizeof() operator on the above-dereferenced values. ( Example : sizeof(*arrPtr))
Pointers with 2D Arrays in C Language :
The Pointers to an array are used extensively to traverse the multidimensional arrays. As we can simply move from one array to another array by performing the arithmetic operations on the pointer to an array.
📢Do note that, Each element of the Two Dimensional arrays (2D array) is a one-dimensional array.
Let’s look at a program to traverse a Two-dimensional array using a pointer to an array.
Pointers to an array to access the elements of the 2 Dimensional Array in C Programming Language:
In the following program, We have created a 2-Dimensional array (2D Array) called arr with the 3 rows and 3 columns and initialized it with the values.
1 2 3 4 5 6 |
// create a 2D Array int arr[3][3] = { Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â {11, 22, 33}, Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â {44, 55, 66}, Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â {77, 88, 99} Â Â Â Â Â Â Â Â Â Â Â Â Â Â }; |
Then we created a pointer to an array called arrPtr, The arrPtris a pointer to an array of 3 Integer elements. and initialized the pointer to an array arrPtrwith the 2D Array arr.
1 2 3 |
// declare pointer to an array // 'arrPtr' pointing to the array of 3 integers int (*arrPtr)[3] = arr; |
Finally, We traversed the 2D array using the pointer to an array arrPtr and displayed the elements of the 2D array by dereferencing the pointer to an array arrPtr
Here is the program to access the values of the 2D Array using a pointer to an array in C Programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program to pointer to 2d array in C     sillycodes.com */  #include<stdio.h>  int main() {     // declare a array     int arr[3][3] = {                     {11, 22, 33},                     {44, 55, 66},                     {77, 88, 99}                 };      // declare pointer to an array     // 'arrPtr' pointing to the array of 3 integers     int (*arrPtr)[3] = arr;      printf("Accessing Elements of 2D array using pointer to an array : \n");     // iterate over the 2d array and print values     for(int i = 0; i<3; i++)     {         for(int j=0; j<3; j++)         {             // print element by dereferencing two times.             printf("%d ", *(*(arrPtr+i)+j) );                         // we can also simply use the two subscripts like below             // printf("%d ", arrPtr[i][j]);         }          printf("\n");     }      return 0; } |
Note, that we used the pointer expression *(*(arrPtr+i)+j)to print the values of the 2D array. As we are using the 2-Dimentional array, we need to dereference twice to get the values.
1 |
printf("%d ", *( *(arrPtr+i)+j) ); |
We can simply use the subscript notation twice like below to get the values of the 2 Dimensional array.
1 |
printf("%d ", arrPtr[i][j]); |
Program Output:
Let’s compile and Run the program.
1 2 3 4 5 6 7 |
$ gcc pointer-to-2d-array.c $ ./a.out Accessing Elements of 2D array using pointer to an array : 11 22 33 44 55 66 77 88 99 $ |
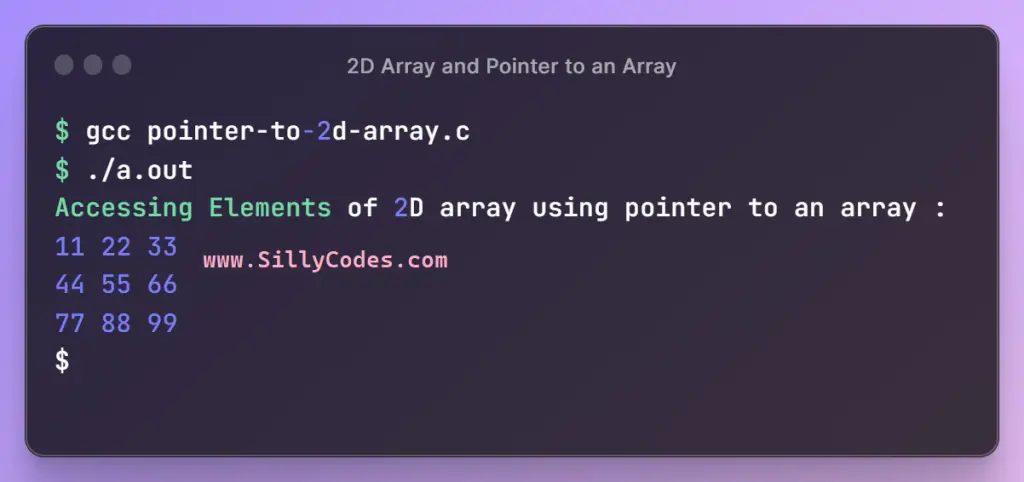
As we can see from the above output, We are able to access the elements of the 2D Array arr using the pointer to an array arrPtr.
📢Similarly, We can use the pointer to arrays to access the elements of the 3-Dimentional arrays as well.
Conclusion:
We looked at the Pointer to array in C programming language, How to Dereferencing the Pointer to an Array. We also looked at the Pointers with 2-Dimentional arrays and How to store and access the 2D Arrays using the Pointer to an entire array in C language.
Related C Programming Tutorials:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Pointer to Pointer in C – Double Pointer in C
- Pointers and Arrays in C Language with Example Programs
- Accessing Array Elements using Pointers in C
- Print String Elements using Pointers in C Language
- Function Returning Pointer in C Programming Language
- Function Pointers in C with Example Programs
1 Response
[…] Pointer to Array in C Programming Language […]