Pattern 21 : Hollow inverted right angle triangle star pattern using for loops
Write a C program to print below inverted triangle star pattern using for loops:
C Program to print the Hollow inverted triangle pattern using the loops.
Here is the sample output of the program when the input size is 5.
Sample output :
Enter Number : 5
* * * * *Â
* Â Â *Â
* Â *Â
* *Â
*Â
Seems few browsers are not displaying the pattern properly, So here is the expected output in image format.
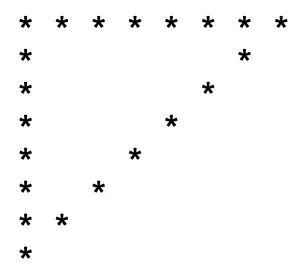
Note : This post is part of my series on Star Pattern programs in C language, Check out all program HERE.
Hollow inverted triangle pattern Program :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include<stdio.h> int main() {     int i,j,k,n;      printf("Enter Number : ");     scanf("%d", &n);         for(i=1; i<=n; i++)     {         for(j=1; j<=n; j++)         {             if(j <= (n+1-i))             {                 if(i==1 || j==1 || j == (n+1-i) )                     printf("* ");                 else                     printf("  ");             }         }         printf("\n");     }     return 0; } |
Hollow inverted triangle pattern Program Output:
![]() |
Output of hollow triangle |
You may also like:
- Basic star pattern program (The square pattern with stars).
- Triangle star pattern program.
- Triangle star pattern program with Spaces.
- Pyramid star pattern.
- Reverse Pyramid program.
- Diamond Star pattern program.
- star pattern program.
- K-Star pattern program.
- Empty Rhombus program.
- X-Star pattern program
More C programs:
- Program to calculate Maximum of three numbers.
- What are Prime Number and C program to Check given number is Prime or Not
- Check given Number is Prime or not Using Square Root(sqrt) Function.(Efficient way)
- C Program to generate prime numbers between two numbers
- C Program to generate first N prime numbers.
- C program to Calculate percentage of student.
- C Program to check given year is the leap or not.
- C Program to convert Temperature.
- C program to understand type conversation.
- Finding Largest of two numbers using the conditional operator in C.
- C program to calculate the simple Interest,
- C program to understand Size of Operator.
- 200+ C Programs.
C Tutorials with simple Examples:
- Arithmetic Operators with Examples.
- Arithmetic operators priority and it’s Associativity.
- Modulus Operator and Hidden Concepts of Modulus Operator.
- Precedence Table or Operators Priority Table.
- Assignment Operator, Usage, Examples
- Increment Operator and Different types of Increment operators Usage with Examples.
- Decrement Operator and Different types of Decrement operators with Examples.
- Logical or Boolean Operators.