strlen in C language – C Library Functions
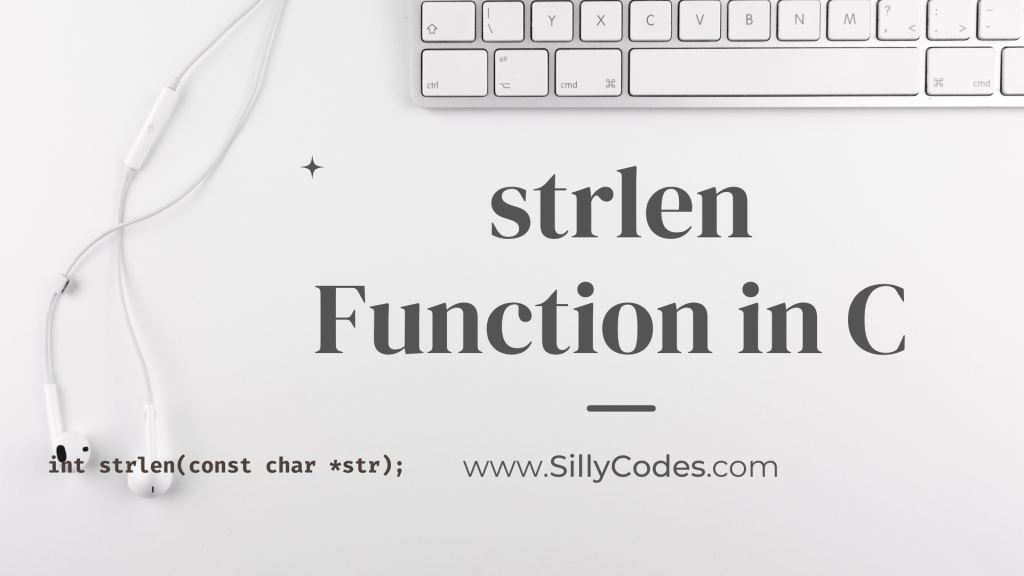
Introduction:
In our previous article, We looked at the strings in the C Language and how to read and print the string in C. In today’s article, We are going to look at the strlen library function.
The strlen function in C programming language:
As the name suggests, The strlen() function is used to calculate the length of the given string. The strlen (String length) function is available as part of the string.h header file.
As we already know, The C strings are terminated with the NULL characters ( \0) at the end.
For example,
char str[] = "hi";
If you check the size of the above string str, We will get the output as the 3. We have two characters ( “hi”) and the NULL characters which is added by the compiler.
But the strlen function only returns the actual length of the string without the NULL value. So if you call the strlen function with the “hi” string. The output will be 2.
📢 The strlen() function doesn’t consider the NULL character. It will only return the actual length (excluding the NULL character).
Let’s quickly look at the syntax of the strlen function.
Syntax:
Here is the syntax of the strlen (string length) function.
1 |
int strlen(const char *str); |
Parameters:
The strlen function takes a String ( const char *) as an argument. This is the input string.
Return Value:
The string length (strlen) function calculates the length of the given string str and returns the length as the int ( size_t – unsigned int)
📢 This Program is part of the C String Practice Programs series
Let’s look at a couple of examples to understand the strlen function.
Example 1: Program to understand the strlen in C:
The following program will prompt the user to provide a string and then calculate the length of the string using the strlen function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
/* program to understand the strlen function in c sillycodes.com */ #include<stdio.h> #include<string.h> // for 'strlen' function int main() { // declare a string char msg[100]; // Read the string from the user printf("Enter a string : "); scanf("%s", msg); int msg_len = strlen(msg); printf("Length of the given string is %d characters\n", msg_len); return 0; } |
📢We need to include the
string.h header file to access the
strlen function.
Related: Compilation stages in C Language
Program Output:
Compile and Run the program
1 2 3 4 5 |
$ gcc strlen-func.c $ ./a.out Enter a string : Welcome Length of the given string is 7 characters $ |
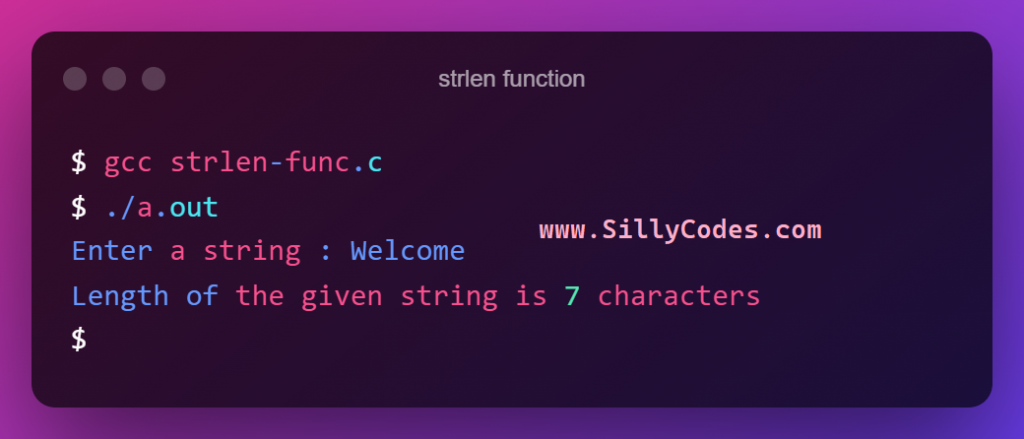
Program Explanation:
As you can see from the above program and output, We created a string named msg and updated it with the user input. Then we passed the msg as an argument to the strlen function to calculate the length of the msg string.
When user entered Welcome as the input string, The strlen function calculated the length of the string and returned the result 7. ( Number of characters in the Welcome string – W, e, l, c, o, m, and e characters)
Example 2: Program to calculate the string(with special characters) length using strlen function:
The strlen() function will work on strings with special characters. So we can calculate the string length using strlen function, When the input string contains special characters.
1 2 3 4 5 6 7 8 |
// Read the string from the user printf("Enter a string : "); scanf("%s", msg); // calculate the string length using 'strlen' int msg_len = strlen(msg); printf("Length of the given string is %d characters\n", msg_len); |
Run the program and provide a string with special characters.
1 2 3 4 5 |
$ gcc strlen-func-special.c $ ./a.out Enter a string : @sillycodes.com Length of the given string is 15 characters $ |
As we can see from the above output, The given string @sillycodes.com contains two special characters ‘@’ character and ‘.’ full-stop character.
Here is another example
1 2 3 4 |
$ ./a.out Enter a string : #^&@*# Length of the given string is 6 characters $ |
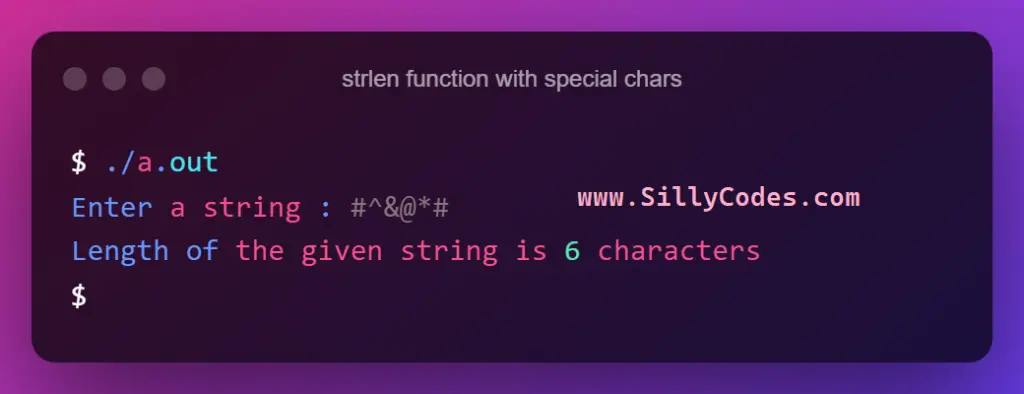
The strlen function properly calculated the size of the strings with special characters.
Example 3: Calculate the length of the string with spaces using strlen function:
Similarly to the above examples, We can use the strlen() function to calculate the length of the string with spaces.
One thing to note is the scanf() function only reads the input string up to the first whitespace characters. So we need to use the gets or fgets function to read the string from the user.
ℹ️ If you use the fgets() function, The newline character( \n) might also be read by the fgets function, So if you apply the strlen function on the string, You might get string length as actul_length+1
Let’s look at an example program to calculate the string with spaces.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* program to understand the strlen function in c when string contains the spaces sillycodes.com */ #include<stdio.h> #include<string.h> // for 'strlen' function int main() { // Initialize a string char msg[] = "Welcome to Sillycodes.com"; // call the 'strlen' function with 'msg' int msg_len = strlen(msg); // print the results printf("Length of the given string is %d characters\n", msg_len); return 0; } |
In the above program, We initialized a string called msg with the Welcome to Sillycodes.com string. Then we called the strlen(msg) function and passed the msg string to calculate the msg string length. Finally, Displayed the string length on the console using the printf() function.
Program Output:
Compile and run the above program.
1 2 3 4 |
$ gcc str-spaces.c $ ./a.out Length of the given string is 25 characters $ |
As we can see from the above output, The given string Welcome to Sillycodes.com contains few spaces and still the strlen() function able to calculate the size of the string.
Conclusion:
In this article, We have looked at the strlen function from the string.h header file. and We used the strlen() function to calculate the length of the string with the normal characters, special characters, and the string with spaces.
5 Responses
[…] strlen library function with example Programs […]
[…] earlier articles, We looked at the strlen function and strcpy functions. In today’s article, We will look at the strcat in C Programming language […]
[…] covered the strlen, strcpy, and strcat library functions in earlier posts. In today’s article, We will look at the […]
[…] have looked at the strlen, strcpy, strcat, and strcmp library functions in our earlier articles. In today’s article, We […]
[…] have covered the strlen, strchr, strcpy, strcat, and strcmp library functions in our earlier posts. Today’s post will […]