Anagram Program in C – Check two strings are anagrams
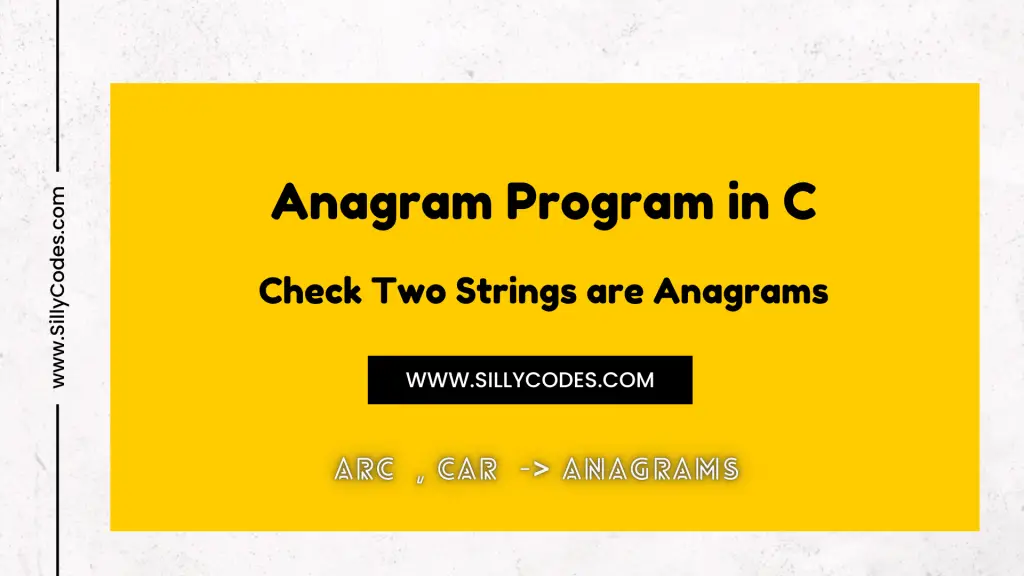
Program Description:
In an earlier article, We looked at how to Swap two strings in C. In today’s article, We will look at the anagram program in c language. The program should accept two strings from the user and check if the two strings are anagrams.
📢 This Program is part of the C String Practice Programs series
What is Anagram String:
An anagram is a word or phrase that is formed by rearranging the letters of another word or phrase.
All the original word letters are used exactly once.
An example of Anagram strings are:
State and Taste.
We can create the State string by rearranging the letters of the Taste string.
📢 The program is case-insensitive(Ignore case). So the uppercase characters and lowercase characters have the same meaning. (i.e V = v )
Let’s quickly look at the example input and output of the program.
Example Input and Output:
Input:
Enter the first string : Players
Enter the second string: parsley
Output:
ANAGRAMS! Given Strings are Anagrams
We are going to look at a few methods to check if the given strings are Anagrams and Each program will be followed by a detailed step-by-step explanation and program output. Then We also provided instructions for compiling and running the program using your compiler.
We will look at three different methods to check Anagram in C, They are
- Standard Method – Iterative method for Anagram program in C. Uses manual process to check the anagram. (Easy and straightforward Method)
- Using User-defined function – We are going to use a user-defined function to check the anagram.
- Advanced Method – In this method, We track the frequency of each character and check the anagram. ( Recommended Method)
Let’s look at each method in detail.
Algorithm for Anagram Program in C – Standard Method:
- Take two input strings from the user and read the strings using the gets function. Let the input strings as firstStr and secondStr
- Calculate the length of each string using the strlen() function and store the string lengths in len1 and len2 respectively.
- Check if the len1 and len2 are equal, If the two strings’ lengths are not equal, Then they are not anagrams. So display the error message and stop the program using return statement.
- As the program is case-insensitive, We need to convert the input strings to either lowercase or uppercase. We are converting the strings to lowercase using a custom strLower() function.
- The prototype of strLower() function is – char * strLower(char * str). Learn more about converting string from upper case to lower case at Convert Upper Case string to Lower Case Program.
- Call the strLower() function with two strings firstStr and secondStr.
- Once the above step 5 is completed, Two strings will be in Lower case. It will ease up our comparison.
- Now, We need to sort the strings. We are using the qsort() function from stdlib.h library. Call the qsort() function with each string to sort the strings in ascending order.
- Learn more about sorting strings using qsort at Sort strings in Ascending order and descending order using Qsort() function
- qsort(firstStr, strlen(firstStr), sizeof(char), compare);
- qsort(secondStr, strlen(secondStr), sizeof(char), compare);
- Once the above operation is completed, The two strings will be sorted in ascending order. Now we need to Compare the firstStr and secondStr strings.
- We are using the strcmp library function to compare the strings. Call the strcmp function with two strings firstStr and secondStr.
- If the strcmp() function returns Zero(0), Then the two strings firstStr and secondStr are Anagrams. Otherwise, Strings are Not Anagrams.
- Display the result on the console.
Standard Method: Program to Check two strings are Anagrams in C:
Let’s convert the above algorithm into the C program which will check if the two strings are anagrams.
We have added extensive comments to the program for your convenience.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 |
/*     program check anagrams in c     sillycodes.com */  #include <stdio.h> #include <string.h> #include <ctype.h> #include <stdlib.h>  // Max Size of string const int SIZE = 100;   /** * @brief  - Convert the string to lower case string * * @param str  - input string * @return char* - lower case string * source code - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/ */ char * strLower(char * str) {     int i;         // Iterate over the string('str')     for(i = 0; str[i]; i++)     {         // use 'isupper()' library function to check if the 'ch' is upper case         if(isupper(str[i]))         {             // call the 'tolower' library function.             str[i] = tolower(str[i]);         }     }      // return converted string     return str; }  /** * @brief - Compare function for sorting strings in Ascending order * Learn more at  - https://sillycodes.com/sort-the-characters-of-a-string-in-c-sort-string-in-ascending-and-descending-order/ */ int compare(const void *a, const void *b) {     return *(char *)a - *(char *)b; }  int main() {      // declare two strings with 'SIZE'     char firstStr[SIZE], secondStr[SIZE];      // Take the first string from the user     printf("Enter the first string : ");     gets(firstStr);      // take the second str     printf("Enter the second string: ");     gets(secondStr);      // check the length of two strings     int len1 = strlen(firstStr);     int len2 = strlen(secondStr);     if(len1 != len2)     {         // length are not matched, So not anagrams         printf("NOT ANAGRAMS! Given Strings '%s' and '%s' are not anagrams\n", firstStr, secondStr);         return 0;     }      // convert strings to lower case characters     // use - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/     strLower(firstStr);     strLower(secondStr);      // Sor the two strings     // we are using - https://sillycodes.com/sort-the-characters-of-a-string-in-c-sort-string-in-ascending-and-descending-order/     // call the 'qsort()' function with 'firstStr' and 'compare' function     qsort(firstStr, strlen(firstStr), sizeof(char), compare);     // call the 'qsort()' function with 'secondStr' and 'compare' function     qsort(secondStr, strlen(secondStr), sizeof(char), compare);         // compare the strings using 'strcmp' function     int result = strcmp(firstStr, secondStr);      // If two strings are equal, Then both strings are anagrams     if(result == 0)     {         // Anagrams         printf("ANAGRAMS! Given Strings are Anagrams\n");     }     else     {         // Not Anagrams         printf("NOT ANAGRAMS! Given Strings are not Anagrams\n");     }      return 0; } |
We are using three extra header files, string.h, ctype.h, and stdlib.h header files.
Program Output:
Let’s compile and run the program using GCC compiler (Any compiler)
Test case 1:
Provide two strings and check if they are anagrams
1 2 3 4 5 6 |
$ gcc check-anagram.c $ ./a.out Enter the first string : arc Enter the second string: car ANAGRAMS! Given Strings are Anagrams $ |
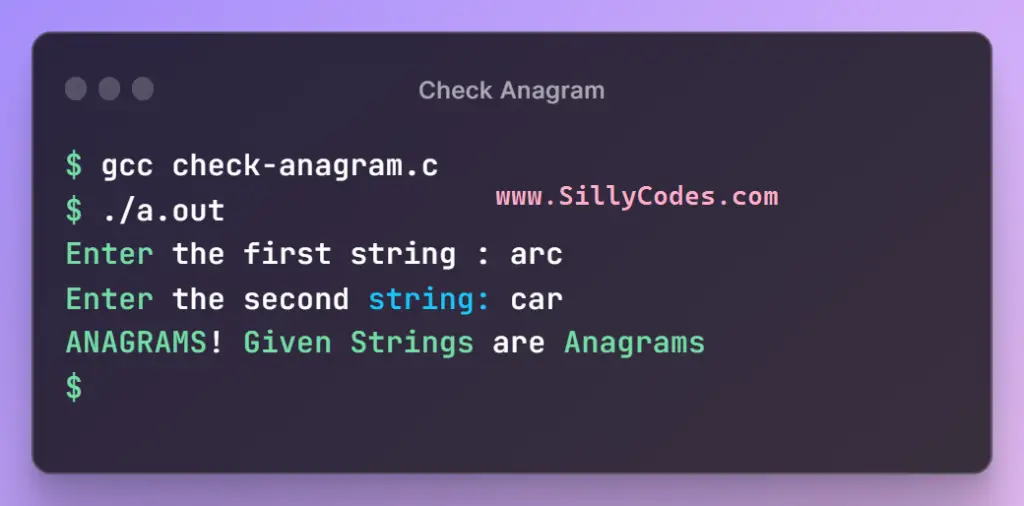
We can form the car string by rearranging the characters of the arc string, So the given strings arc and car are anagrams.
1 2 3 4 5 |
$ ./a.out Enter the first string : listen Enter the second string: silent ANAGRAMS! Given Strings are Anagrams $ |
The input strings listen and Silent are anagrams, So the program properly calculated and displayed the strings as anagrams.
Test Case 2: Two Word Anagrams or Check if the phrases are anagrams.
1 2 3 4 5 6 |
// Two word anagram $ ./a.out Enter the first string : the eyes Enter the second string: They See ANAGRAMS! Given Strings are Anagrams $ |
As we can see from the above output, The program is able to check the anagrams of phrases or sentences. The Given phrases the eyes and They See are Anagrams.
Test Case 3: Negative Case
1 2 3 4 5 |
$ ./a.out Enter the first string : programming Enter the second string: learn NOT ANAGRAMS! Given Strings are not Anagrams $ |
In this example, We have provided the strings as programming and learn. As we can see these two strings are of different length and doesn’t form the other string, So they are Not Anagrams.
Anagram Program in C using user-defined functions:
In the above program, We have used two custom functions like strLower and compare but the logic to check anagram strings is written inside the main function. So let’s move this code to a separate function So that we can simply call the check anagram function from the main() function.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the rewritten version of the anagram program in C. In this program, We defined a custom function called isAnagram to check anagram strings.
The prototype of the isAnagram() function is
int isAnagram(char * firstStr, char * secondStr);
This function takes two strings as formal arguments, They are firstStr and secondStr. It checks if the given strings are Anagrams.
The isAnagram() function returns One(1) if the two strings are Anagrams, Otherwise, it returns Zero(0).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 |
/*     program check anagrams in c using user defined function     sillycodes.com */  #include <stdio.h> #include <string.h> #include <ctype.h> #include <stdlib.h>  // Max Size of string const int SIZE = 100;  // function declaraion int isAnagram(char * firstStr, char * secondStr);   /** * @brief  - Convert the string to lower case string * * @param str  - input string * @return char* - lower case string * source code - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/ */ char * strLower(char * str) {     int i;         // Iterate over the string('str')     for(i = 0; str[i]; i++)     {         // use 'isupper()' library function to check if the 'ch' is upper case         if(isupper(str[i]))         {             // call the 'tolower' library function.             str[i] = tolower(str[i]);         }     }      // return converted string     return str; }  /** * @brief - Compare function for sorting strings in Ascending order * Learn more at  - https://sillycodes.com/sort-the-characters-of-a-string-in-c-sort-string-in-ascending-and-descending-order/ */ int compare(const void *a, const void *b) {     return *(char *)a - *(char *)b; }  int main() {      // declare two strings with 'SIZE'     char firstStr[SIZE], secondStr[SIZE];      // Take the first string from the user     printf("Enter the first string : ");     gets(firstStr);      // take the second str     printf("Enter the second string: ");     gets(secondStr);      // call the isAnagram() function and pass two strings     int result = isAnagram(firstStr, secondStr);      // If two strings are equal, Then both strings are anagrams     if(result == 1)     {         // Anagrams         printf("ANAGRAMS! Given Strings are Anagrams\n");     }     else     {         // Not Anagrams         printf("NOT ANAGRAMS! Given Strings are not Anagrams\n");     }      return 0; }  /** * @brief  - Check if two strings are anagram * * @param firstStr - first string * @param secondStr - second string * @return int      - returns `1` if two strings are anagrams, Otherwise returns `0` */ int isAnagram(char * firstStr, char * secondStr) {     // check the length of two strings     int len1 = strlen(firstStr);     int len2 = strlen(secondStr);     if(len1 != len2)     {         // length are not matched, So not anagrams         return 0;     }      // convert strings to lower case characters     // use - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/     strLower(firstStr);     strLower(secondStr);      // Sor the two strings     // we are using - https://sillycodes.com/sort-the-characters-of-a-string-in-c-sort-string-in-ascending-and-descending-order/     // call the 'qsort()' function with 'firstStr' and 'compare' function     qsort(firstStr, strlen(firstStr), sizeof(char), compare);     // call the 'qsort()' function with 'secondStr' and 'compare' function     qsort(secondStr, strlen(secondStr), sizeof(char), compare);         // compare the strings using 'strcmp' function and return it's value     return !strcmp(firstStr, secondStr);    // note, we have '!' at the start  } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 |
$ gcc anagram-func.c $ ./a.out Enter the first string : State Enter the second string: Taste ANAGRAMS! Given Strings are Anagrams $ |
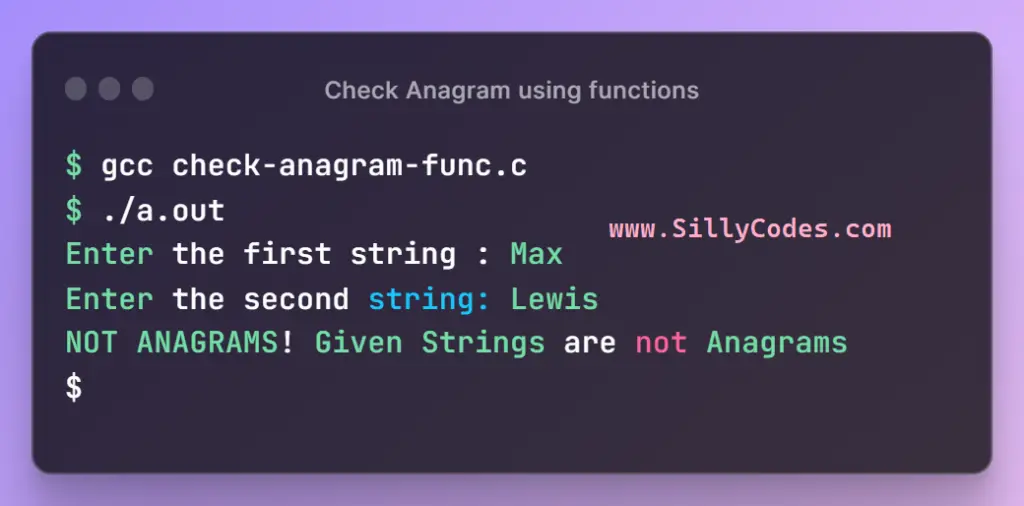
As we can see from the above output, The isAnagram function is properly checking the anagrams.
Algorithm for Anagram Program in C – Advanced Method:
Now, Let’s look at another method, Where we use the character frequencies to check if the two strings are anagrams.
Here is the algorithm of the Anagram program in C (Advanced Method)
- Take two strings from the user and Convert them to lowercase using the custom strLower() function.
- Inside the isAnagram() function, Create a frequency array with the ASCIISIZE(256) to store the frequencies of each character in strings.
- Then, Traverse through the two strings and update the frequency array. – Loop should look like this for(i=0; firstStr[i] && secondStr[i]; i++)
- Check if any of the string is not reached the NULL, Which means the two strings’ lengths are different. So the strings are not anagrams. Return Zero(0).
- Then, Go through the frequency array and check if any character frequency is greater than zero. This means The characters from two strings are not matched, So the strings are not anagrams. If we found any character with greater than zero frequency (>0), Then Strings are not anagrams. Return Zero(0).
- If we iterated over the frequency array successfully and not found any mismatch, Then the given strings firstStr and secondStr are Anagrams. Return One(1).
- Call the isAnagram() function from the main function and pass the two input strings. and display the results.
Advanced Method to Check Anagrams in C Langauge:
Here is the program to check two strings are anagrams in the C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 |
/*     program check anagrams in c using user defined function     sillycodes.com */  #include <stdio.h> #include <string.h> #include <ctype.h>  // Max Size of string #define SIZE 100  // ASCII Values size #define ASCIISIZE 256  // function declaraion int isAnagram(char * firstStr, char * secondStr);  /** * @brief  - Convert the string to lower case string * * @param str  - input string * @return char* - lower case string * source code - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/ */ char * strLower(char * str) {     int i;         // Iterate over the string('str')     for(i = 0; str[i]; i++)     {         // use 'isupper()' library function to check if the 'ch' is upper case         if(isupper(str[i]))         {             // call the 'tolower' library function.             str[i] = tolower(str[i]);         }     }      // return converted string     return str; }  int main() {      // declare two strings with 'SIZE'     char firstStr[SIZE], secondStr[SIZE];      // Take the first string from the user     printf("Enter the first string : ");     gets(firstStr);      // take the second str     printf("Enter the second string: ");     gets(secondStr);      // call the isAnagram() function and pass two strings     int result = isAnagram(firstStr, secondStr);      // If two strings are equal, Then both strings are anagrams     if(result == 1)     {         // Anagrams         printf("ANAGRAMS! Given Strings are Anagrams\n");     }     else     {         // Not Anagrams         printf("NOT ANAGRAMS! Given Strings are not Anagrams\n");     }      return 0; }  int isAnagram(char* firstStr, char* secondStr) {         int i;     // create a 'frequency' array to store the frequencies and initialize with 'zeros'     int frequency[ASCIISIZE] = {0};      // convert strings to lower case characters     // use - https://sillycodes.com/convert-string-from-uppercase-to-lowercase-in-c-language/     strLower(firstStr);     strLower(secondStr);         // Iterate over the two arrays     // increment the character frequency for firstStr     // decrement the character frequency for secondStr     for(i=0; firstStr[i] && secondStr[i]; i++)     {         // increment frequency for firstStr         frequency[firstStr[i]]++;          // decrement frequency for secondStr         frequency[secondStr[i]]--;     }      // check if any of the string is not reached the NULL.     if (firstStr[i] || secondStr[i])     {         // String lengths are not equal. so not anagrams         return 0;     }      // Go throgh the frequency array and check if any frequency is greater than zero     for (i = 0; i < ASCIISIZE; i++)     {         // check character frequency         if (frequency[i])         {             // frequency difference, so Not anagrams             return 0;         }     }      // strings are anagrams     return 1; } |
Program Output:
Let’s compile and Run the above program.
1 2 3 4 5 6 |
$ gcc anagram-advanced.c $ ./a.out Enter the first string : School Master Enter the second string: The Classroom ANAGRAMS! Given Strings are Anagrams $ |
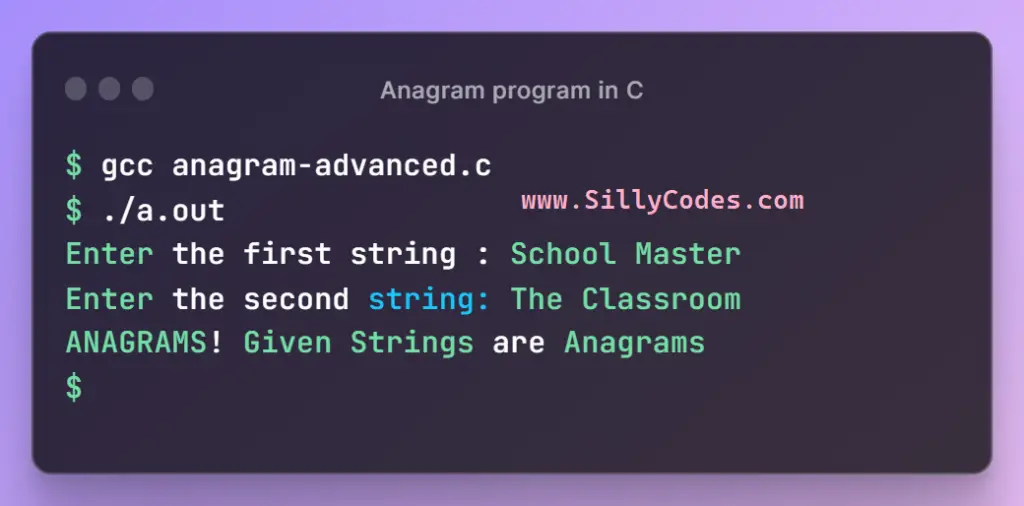
As we can see from the above output, The two input sentences School Master and The Classroom are Anagram strings.
1 2 3 4 5 |
$ ./a.out Enter the first string : Active Enter the second string: Passive NOT ANAGRAMS! Given Strings are not Anagrams $ |
The strings Active and Passive are not anagrams. The program is properly detecting the anagram strings.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
- C Program to Find All Occurrences of a character in string
1 Response
[…] Anagram Program in C – Check two strings are anagrams […]