Decimal to Binary Conversion program in C
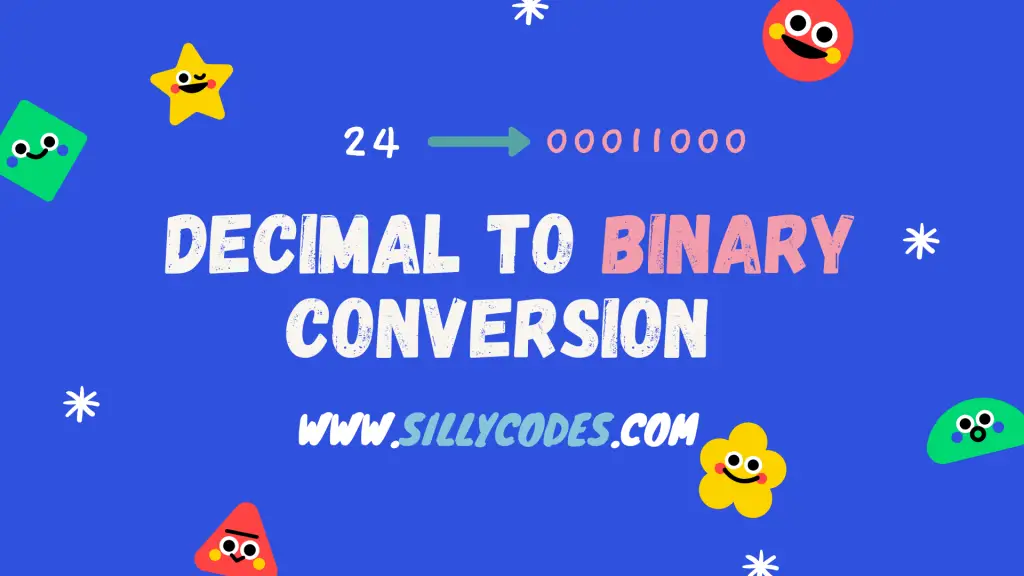
Program Introduction :
Write a C Program to convert a Decimal Number (Integer) to a Binary number ( Decimal to Binary Conversion).
Here are a couple of examples with expected input and output.
📢 Note: We are going to consider the size of the Input as 32 Bits (4 Bytes).
Example 1:
Input:
1 |
Enter a Number : 100 |
Output:
The decimal number 100 in Binary.
1 |
00000000000000000000000001100100 |
Example 2:
Input:
1 |
4535 |
Output:
1 |
00000000000000000001000110110111 |
Example 3:
Input:
1 |
16 |
Output:
1 |
00000000000000000000000000010000 |
Decimal to Binary Conversion C Program Logic:
We are going to use the Bitwise Operators to convert the given Decimal (Integer) number to Binary Number.
The main idea is to go to Each bit and check if the bit is Set or Clear. If the bit is set means the value of that bit is 1. Similarly, if the bit is Clear, Means the value of the bit is .
As we know the integer has 32 bits ( From Index 0 to Index 31 ) or 4 Bytes ( Integer size is compiler dependent, Let’s say 4 Bytes for this example ). We need to go to each bit and check if the bit is Set or Clear.
We will start from the Most Significant Bit (MSB) i.e 31st Index bit and loop until we reach the Least Significant Bit (LSB) i.e 0th index bit. At each Iteration check the bit value.
Algorithm to check if the Bit is Set or Clear:
We can check if the bit is set or clear by using the below bitwise operator logic.
1 |
(n & (1<<i) ) |
To check if the bit ( 'i'th bit) is Set or clear,
- Firstly, Left shift the number one (1) bit times (i times). i.e ( 1 << i ).
- Then perform bitwise and ('&') with the given number. i.e ( n & (1 << i) ).
- If the result of the above operation is 1, Then the Bit is Set. Otherwise, The Bit is Clear.
We will repeat the above steps for all the bits of the given Decimal (Integer) Number.
Decimal to Binary Conversion Program In C:
Note: Please go through the comments for understanding the code better.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include<stdio.h> int main() { int n,i; // Take the input from user and save it in 'n' variable printf("Enter any Number : "); scanf("%d",&n); // We are going to use Bitwise operators to get the Binary representation of number // We will loop through 31 to 0. ( Because Integer size is 32) // At Each Iteration, We will see if the bit is set or clear. // We will left shift the number '1' the bit('i') times // Perform bitwise and (&) with number ('n'). Which will gives us if the certain bit is 1 or 0 // If the bit is set, Display 1, Otherwise display 0 // By the end of the loop we will have the number in Binary format by using this simple bitwise technique for(i=31;i>=0;i--) { //printf("%d",((n>>i)&1) ? 1 : 0); // Check if the 'i'th bit is Set or Clear // Display 1 if the bit is Set // Display 0 if the bit is Clear printf("%d",(n&(1<<i)) ? 1 : 0); } printf("\n"); return 0; } |
Decimal to Binary Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Compiling the program using GCC Compiler venkey@venkey$ gcc int-to-binary.c // Run the executable. venkey@venkey$ ./a.out Enter any Number : 16 00000000000000000000000000010000 // Example 2 venkey@venkey$ ./a.out Enter any Number : 4535 00000000000000000001000110110111 // Example 3 venkey@venkey$ ./a.out Enter any Number : 12345 00000000000000000011000000111001 // Example 4 venkey@venkey$ ./a.out Enter any Number : 98 00000000000000000000000001100010 // Example 5 venkey@venkey$ ./a.out Enter any Number : 876534 00000000000011010101111111110110 |
As you can see from the above output, The binary representation of the decimal number is 32 bit long. And it is very hard to read. Let’s try to improve the readability of the output representation.
What if we add an extra space after every 8 bits. This can improve the readability of Binary numbers. So let’s change the above program to display the Output with spaces after every 8 bits.
Decimal to Binary ( Pretty Print Binary Number) :
We are going to add one extra condition to the above program to prettify the displaying binary number.
We will print extra space after every 8th bit. So that we will get 8 bit spaced binary numbers. Which definitely improves the readability.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#include<stdio.h> int main() { int n,i; // Take the input from user and save it in 'n' variable printf("Enter any Number : "); scanf("%d",&n); // We are going to use Bitwise operators to get the Binary representation of number // We will loop through 31 to 0. ( Because Integer size is 32) // At Each Iteration, We will see if the bit is set or clear. // We will left shift the number '1' the bit('i') times // Perform bitwise and (&) with number ('n'). Which will gives us if the certain bit is 1 or 0 // If the bit is set, Display 1, Otherwise display 0 // By the end of the loop we will have the number in Binary format by using this simple bitwise technique for(i=31;i>=0;i--) { //printf("%d",((n>>i)&1) ? 1 : 0); // Check if the 'i'th bit is Set or Clear // Display 1 if the bit is Set // Display 0 if the bit is Clear printf("%d",(n&(1<<i)) ? 1 : 0); // Add an extra space after every 8th bits. // Which will result into 8 bit spaced binary number if(i%8 == 0) printf(" "); } printf("\n"); return 0; } |
Output:
Now the output is an 8 Bit spaced binary number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
venkey@venkey$ gcc int-to-binary.c venkey@venkey$ ./a.out Enter any Number : 128 00000000 00000000 00000000 10000000 venkey@venkey$ venkey@venkey$ ./a.out Enter any Number : 65536 00000000 00000001 00000000 00000000 venkey@venkey$ ./a.out Enter any Number : 256 00000000 00000000 00000001 00000000 venkey@venkey$ ./a.out Enter any Number : 4096 00000000 00000000 00010000 00000000 venkey@venkey$ |
Conclusion:
In this article, We have discussed the Decimal to Integer conversion using the Bitwise operators.
Related Articles:
- C program to print all ASCII characters and ASCII values/numbers – SillyCodes
- C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes
- Armstrong Number Program in C Language – SillyCodes
10 Responses
[…] To convert Integer to Binary you can following program – Decimal (Integer) to Binary conversion program in C (sillycodes.com) […]
[…] Here is a C Program to convert an Integer number to the Binary sequence – Decimal to Binary conversion program in C (sillycodes.com) […]
[…] Here is a C Program to convert an Integer number to the Binary sequence – Decimal to Binary conversion program in C (sillycodes.com) […]
[…] Here is a C Program to convert an Integer number to the Binary sequence – Decimal to Binary conversion program in C (sillycodes.com) […]
[…] C Program to Convert Decimal Number to Binary Number […]
[…] C Program to Convert Decimal Number to Binary Number […]
[…] C Program to Convert Decimal Number to Binary Number […]
[…] C Program to Convert Decimal Number to Binary Number […]
[…] C Program to Convert Decimal Number to Binary Number […]
[…] C Program to Convert Decimal Number to Binary Number […]