Palindrome Program in C language
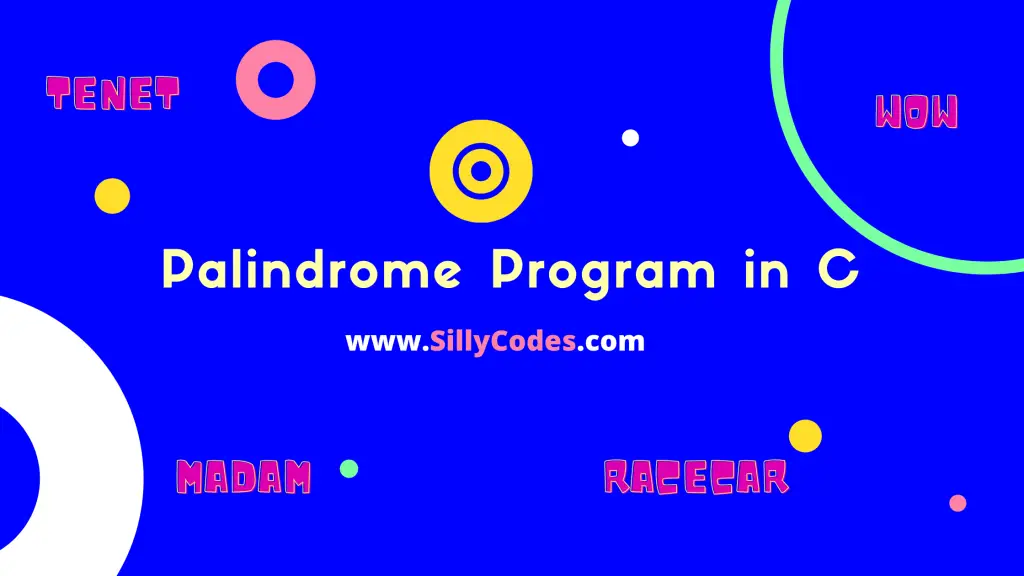
Introduction:
Write a C program to check or detect given string is Palindrome or not ( Palindrome Program in C ).
A palindrome is a word or other sequence of characters which reads the same backward as forward.
Examples of String Palindromes :
madam, wow, racecar, stats, etc.
Examples:
The program should accept a String as input and check if it is a Palindrome or not.
Here are few example expected input and outputs of program.
Example 1:
Input:
1 |
Enter String to test : racecar |
Output:
1 |
Given string racecar is a Palindrome |
The string racecar is palindrome, As we can create the same string in forward direction and backward direction.
Example 2:
Input:
1 |
Enter String to test : picture |
Output:
1 |
Given string picture is not a Palindrome |
Example 3:
Input:
1 |
Enter String to test : tenet |
Output:
1 |
Given string tenet is a Palindrome |
Algorithm to Check Palindrome Program :
- We are going to use two pointer/index method.
- One index will start from the beginning and goes in the forward direction and another index will starts from the end and comes in backwards direction.
- Forward index 'i' starts with index and Backward index 'j' starts from 'len-1' ( Here 'len'is the length of the string, So we are starting from the end of the string)
- At each iteration, We are going to check if the character at index 'i' and the character at index 'j' are same or not?
- If the string is palindrome then all characters at index 'i' and index 'j' need to be same ( Until ‘i'<‘j’ condition break), If any of the values at index 'i' and index 'j' are not equal then the string is not a Palindrome.
- If all the characters of 'i' and 'j' are equal until we reach the loop termination i.e ‘i'<‘j’, Then given number is a Palindrome.
Check Palindrome Program in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
#include<stdio.h> #include<string.h> int main() { // Declare the desired variables char str[20]; int i,j,len, cnt; cnt = 0; // Take the input string from the user and store it in 'str' variable. printf("Enter String to test : "); scanf("%s", str); // Check the length of the string len = strlen(str); // Here is the main logic of the program. // We are going to use two pointer/index method. // One will start from the begining and goes forward and another will starts from the end and comes backwards. // One index 'i' starts with 0 and another index 'j' starts from 'len-1' // At each iteration, We are going to check if the index 'i' value and index 'j' value are same // If the string is palindrome it need to be same, If any of the values at index 'i' and index 'j' are not equal then the string is not a Palindrome // If all the values are equal until we reach the loop termination i.e 'i'<'j', Then given number is a Palindrome. for(i=0,j=len-1; i<j; i++,j--) { // Check if the value at index'i' and 'j' are same // If same then continue // If not same means the number is not a Palindrome, Increase the cnt and break out the loop if(str[i] != str[j]) { cnt++; break; } } // Check if the 'cnt' is 0, // If the 'cnt' is 0, Means all the values at 'i' and 'j' are same. // which means the given number is Palindrome. if(cnt == 0) printf("Given string %s is a Palindrome\n", str); else printf("Given string %s is not a Palindrome\n", str); return 0; } |
Palindrome Program Output:
We are using the GCC compiler on the Ubuntu Linux system. You can use your favourite IDE or Editor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// Compile the program using the 'gcc' compiler venkey@venkey $ gcc palindrome.c // Run the executable file './a.out' venkey@venkey $ ./a.out Enter String to test : wow Given string wow is a Palindrome // Example 2 venkey@venkey $ ./a.out Enter String to test : racecar Given string racecar is a Palindrome // Example 3 venkey@venkey $ ./a.out Enter String to test : f1race Given string f1race is not a Palindrome // Example 4 venkey@venkey $ ./a.out Enter String to test : tenet Given string tenet is a Palindrome // Example 5 venkey@venkey $ ./a.out Enter String to test : picture Given string picture is not a Palindrome // Example 6 venkey@venkey $ ./a.out Enter String to test : noon Given string noon is a Palindrome venkey@venkey $ |
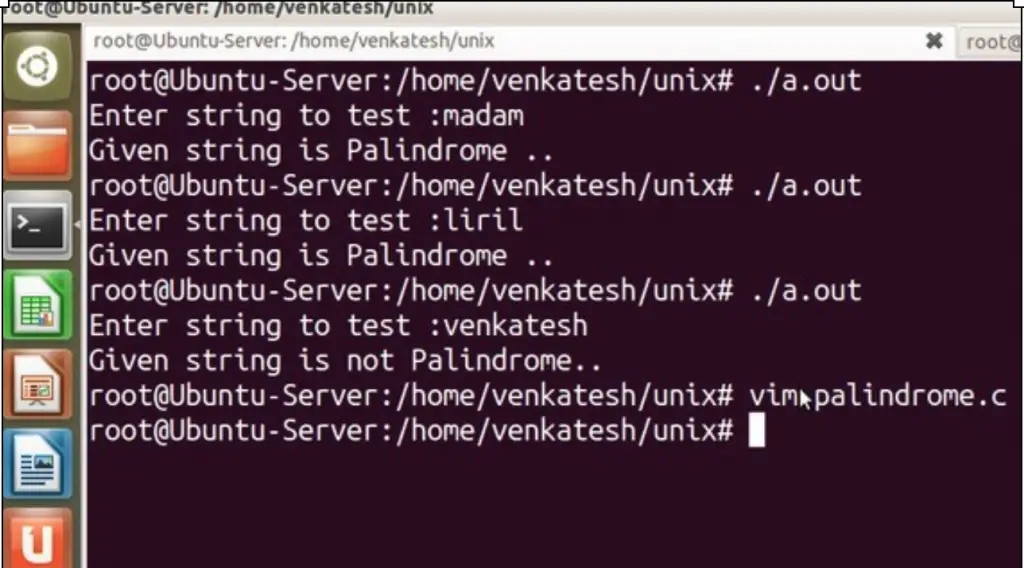
Conclusion:
We have discussed about the logic to detect or check the string palindromes using the C programming Language.
Related Reading:
- Start Here – Step by step tutorials to Learn C programming Online with Example Programs – SillyCodes