C Program to check character is alphabet or number or a Special character
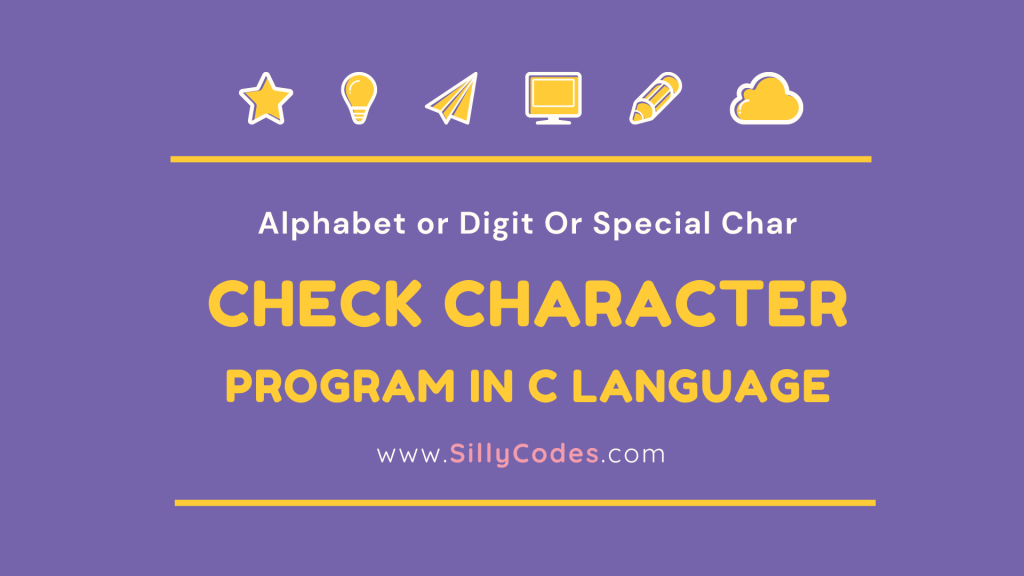
Check Character Program :
Write a C Program to Check character is alphabet or number or a special character. The program should accept a character from the user and detect if the given character is Alphabet or Digit or Special Character.
Here are the example input and excepted output of the program.
Example 1
Input:
1 |
Enter character to test : 4 |
Output:
1 |
4 is Digit.. |
Example 2:
Input:
1 |
Enter character to test : M |
Output:
1 |
M is alphabet.. |
Example 3:
Input:
1 |
Enter character to test : & |
Output:
1 |
& is special symbol.. |
Algorithm – Check character is alphabet or Digit or a Special character :
Here is the algorithm of the character detection program.
- The program should take one character as input from the user. We are going to store it in the c varaible.
- Then We need to check if the given character is Alphabet, Digit, or a special character.
- We are going to use the
ctype.h library for checking the character. The
ctype.h library contains the functions like
isalpha() and
isdigit().
- The isalpha(ch) function takes Integer (which will be converted to ASCII value upon passing ) as input. Returns a non-zero integer value if the given character ch is Alphabet.
- Similarly, The isdigit(ch) function takes Integer as input. and Returns the non-zero Integer value if the given value is a digit.
- By using the isalpha() and isdigit() functions we will check if the given input is an alphabet or Digit.
- If the given number is neither alphabet nor Digit, Then it must be a Special character.
Check character is alphabet or number or a Special character Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include<stdio.h> #include<ctype.h> int main() { // Create a character variable 'ch' char ch; // Take the input character from user // Store it in the 'ch' variable printf("Enter character to test : "); scanf(" %c", &ch); // We will check if the given character 'ch' is alphabet // by using the 'isalpha()' function // The 'isalpha()' function is available in the ctype.h library // 'isalpha()' function takes character as input and return 'true' if it is alphabet. if(isalpha(ch)) //is alpha available in ctype.h { printf("%c is alphabet..\n",ch); } else if(isdigit(ch)) { // The 'isdigit()' function also available in the 'ctype.h' library. // 'isdigit()' function takes one character as input and returns true if the given character is Digit. printf("%c is Digit..\n",ch); } else { // If the given character is not a 'alhpabet' and 'Digit' then it must be special character. printf("%c is special symbol..\n",ch); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// Compilling the program using the gcc compiler venkey@venkey$ gcc check-alpha.c // Run the Executable file venkey@venkey$ ./a.out Enter character to test : 1 1 is Digit.. venkey@venkey$ ./a.out Enter character to test : A A is alphabet.. venkey@venkey$ ./a.out Enter character to test : v v is alphabet.. venkey@venkey$ ./a.out Enter character to test : ? ? is special symbol.. venkey@venkey$ ./a.out Enter character to test : * * is special symbol.. venkey@venkey$ ./a.out Enter character to test : Q Q is alphabet.. venkey@venkey$ ./a.out Enter character to test : 4 4 is Digit.. venkey@venkey$ |
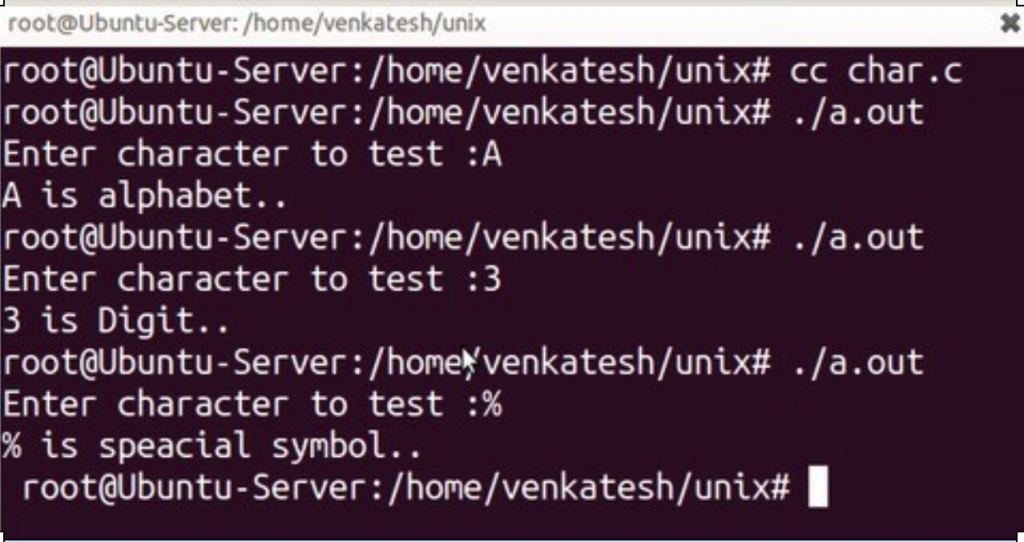
Method 2: character is alphabet or number or a special character Program:
In the previous method, We used the in-built functions like isalpha and isdigit. In this method, we are going to check the alphabet and digit manually.
Logic to check the alphabet:
We are going to compare the ASCII characters. To be an alphabet, the given character must be in between the a and z ( upper case and Lower case). So we can use the following logic to check the alphabet
(ch >= ‘a’ && ch <= ‘z’) || (ch >= ‘A’ && ch <= ‘Z’)
If the above logic returns True, Then the given number is Alphabet.
Logic to check the Digit:
Here also we will compare the ASCII character. Use the following condition to check if the character ch is Digit.
ch >= ‘0’ && ch <= ‘9’
If this condition is True, Then input character ch is Digit.
Check character C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#include<stdio.h> int main() { // Create a character variable 'ch' char ch; // Take the input character from user // Store it in the 'ch' variable printf("Enter character to test : "); scanf(" %c", &ch); // We will check if the given character 'ch' is alphabet // Manually comparing the 'ch' with ASCII characters // if the 'ch' is betwen the ASCII character 'a' and 'z' (Lower and Upper Case) if( (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { printf("%c is alphabet..\n",ch); } else if(ch >= '0' && ch <= '9' ) { // Manullay comparing the ASCII characters // 'ch' should be greater than or equal to '0' and lessthan or equal to 9 to be Digit printf("%c is Digit..\n",ch); } else { // If the given character is not a 'alhpabet' and 'Digit' then it must be special character. printf("%c is special symbol..\n",ch); } return 0; } |
Program Output:
We used GCC compiler to compile and run the program on Linux operating system. Learn more about the C Program compilation in following article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc alpha-digit.c $ ./a.out Enter character to test : M M is alphabet.. $ ./a.out Enter character to test : v v is alphabet.. $ ./a.out Enter character to test : 8 8 is Digit.. $ ./a.out Enter character to test : 0 0 is Digit.. $ ./a.out Enter character to test : ] ] is special symbol.. $ ./a.out Enter character to test : ? ? is special symbol.. $ |
2 Responses
[…] C Program to check character is Alphabet or Numbre or Special Character […]
[…] C Program to check character is Alphabet or Number of Special Number […]