Operators in C Language | Different type of Operators in C language
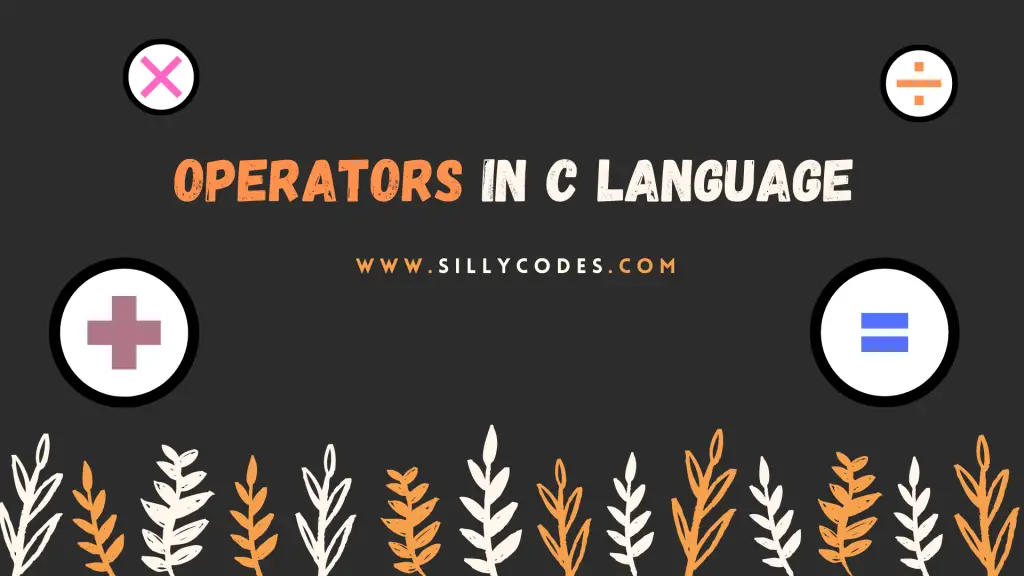
Introduction:
In our previous article, We have discussed Keywords in C-Langauge. In todays article, We will discuss about the Operators in C programming language. and we will also look into different types of operators of c-language with examples.
Operators in C Language:
An Operator is a symbol that tells the compiler to perform specific task. For example, The addition operator plus ( '+' ) used to perform the addition operation.
All Operators operate on the Operands.
What are Operands :
Operands are data elements on which operator acts. Let’s take an example.
sum = 10 + 20;
Here the '+' ( Plus ) is an Addition Operator
and numbers 10 and 20 are the operands.
C Programming Language supports different types of Operators. Different operator takes different number of operands to perform its task.
Depending upon the number of operands, The Operators are Classified into 3 types.
- Unary Operators
- Binary Operators
- Ternary Operators
Unary Operators:
Unary operators act on single Operand. These unary Operators need only one operand to perform the operation.
Few examples of Unary Operators:
- One’s Compliment Operator ( ~ )
- Increment ( Post / Pre ) Operators ( ++ )
- Decrement ( Pre / Post ) Operators ( -- )
- Unary Plus ( + )
- Unary Minus ( - )
Binary Operators:
The Operators which need two operands to perform its operation are called Binary Operators.
Here are few examples of Binary Operators in C Language.
- Addition Operator ( + )
- Subtraction Operator ( - )
- Multiplication Operator ( * )
- Division Operator ( / )
- Modulus Operator ( % )
Ternary Operators:
Ternary Operators need three Operands to accomplish it’s task.
For example, C Language have Condition Operator which uses three Operands to perform its operation.
- Conditional Operator ( : ? )
Classification of Operators in C Programming Language:
The above classification is based on the number of Operands. But we also have other classification like Role based classification. All C Operators falls under several categories based on their role, Which are
- Arithmetic Operators.
- Assignment Operators.
- Increment Operator and Pre and post Increments
- Decrements Operators and Pre and post decrements
- Relational Operators.
- Conditional Operator.
- Logical Operators
- Comma Operator.
- Sizeof Operator.
- Bitwise Operators.
- Precedence and Associativity of Operators
Here are few examples of above Operators.
Arithmetic Operators:
These Operators are used to perform the Arithmetic Operations like Addition, Subtraction, Multiplication, etc.
Here is the list of arithmetic operator in C programming Language
Operator | Description | Syntax | Example |
---|---|---|---|
Addition ( + ) | Adds two or more numbers | a + b | >
200 + 100 300 |
Subtraction ( - ) | Subtracts two or more numbers | a – b | >
200 - 100 100 |
Multiplication ( * ) | Multiplies two or more numbers | a * b | >
200 * 100 20000 |
Division ( /) | Divides left side operand by right operand | a / b | >
200 / 100 2 |
Modulus ( %) | Calculate the reminder of division of two operands | a % b | >
25 % 10 5 |
Assignment Operators ( Compound Operators ) in C :
The Assignment Operators used to assign values to Variables.
The equal = is the Assignment operator.
int x = 10 // assignment operation ( Variable Initialisation )
In above statement, We have created a variable called x and assigned the value 10 to it using assignment Operator ( = )
The C programming language also supports Compound assignment operators to perform the assignments along with other arithmetic operations.
For example,
x = 100 // Simple assignment
x += 200 // compound operation
In the above example, x += 200is a Compound Assignment Operation. Which is equal to x = x + 200
Here is the list of Compound Assignment Operations.
Operator | Syntax | Description | Example |
---|---|---|---|
Assignment ( = ) | a = b | Creates variable 'a' and assigns value 'b'to it. | >
a = 10 a value is 10 |
Compound Addition (
+= ) or Add and Assign | a += b | First, Evaluate the addition of
a+b then assigns result to
a step 1: a+b step 2 : a = a+b //result of a+b will be assigned to a | >
a = 10 > a += 10 a value is 20 |
Compound Subtract (
-= ) or Subtract and Assign | a -= b | First, Evaluate the a-b then assign the result to a | >
a = 10 > a -= 10 a value is |
Compound product (
*= ) or Multiply and Assign | a *= b | First, Evaluate the a*b then assign the result to a | >
a = 10 > a *= 10 a value is 100 |
Compound division (
/= ) or Division and Assign | a/= b | First, Evaluate the a/b then assign the result to a | >
a = 10 > a /= 10 a value is 1 |
Compound Modulus (
%= ) or Modulus and Assign | a %= b | First, Evaluate the a%b then assign the result to a | >
a = 10 > a %= 10 a value is |
For above example, We took a variable a and assigned the value 10. Then performed compound addition, compound subtraction, etc operations with variable a and value 10 and displayed the result values. ( i.e a values)
Please go through the above operators links for learning more about each operator.
Conclusion:
In this article, We have discussed about the operators in C language and different types of Operators.
2 Responses
[…] Operators […]
[…] our previous article, We have looked at the Operators and different types of Operators in C language. In todays article, We will learn about the Constant in C language and categories of C […]