Program to Count Number of Words in String in C (4 -Methods)
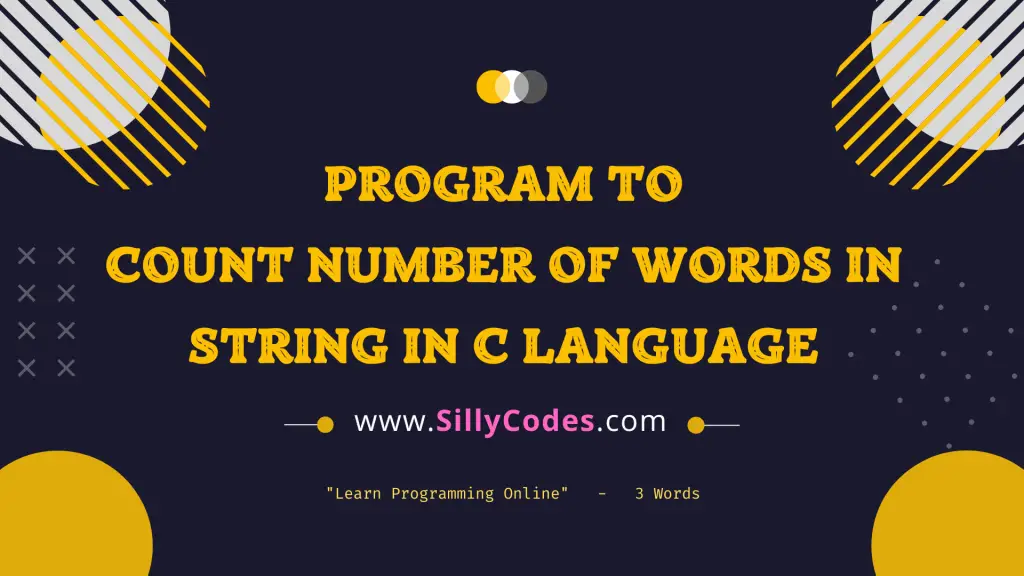
Program Description:
Write a Program to count number of words in string in c programming language. The program should accept a string from the user and count the total number of words in the given string.
We are going to look at a few methods to count a number of words in a string and Each program will be followed by a detailed step-by-step explanation with program output. We also provided instructions for compiling and running the program using your compiler.
📢 This Program is part of the C String Practice Programs series
Before looking at the programs, Let’s understand the excepted input and output of the program.
Example Input and Output:
Input:
Enter a string : Learn C Programming Online at SillyCodes.com
Output:
Number of words in the string is : 6
The input string contains six words.
Prerequisites:
Please go through the following Strings, Functions, and Arrays tutorials to better understand the program.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
We are going to look at Four Methods to count the number of words in a string
- Basic Method: Iterative method to count the number of words in a string. This program might fail if your string contains extra spaces.
- Improved Method: Calculates the word count. This program can handle extra spaces in the string.
- User-Defined Function: This is a modified version of Method-2, Which uses the C functions to count words in a string.
- Using strtok function: Counts the number of words in a string using the strtok string library function.
Let’s look at all these methods one by one.
Basic Method: Count Number of Words in String in C Program:
This is a basic form of calculating the words in a string. This method traverses through the input string and looks for the spaces. This method will fail if your string contains extra spaces. please look at the Improved method if you are string contains extra spaces.
Let’s look at the step by step instructions of the program.
Algorithm to count number of words in string basic method:
- Start the program by declaring the input string and let’s say it as str.
- Take the input string from the user and update the str string. we are using gets() function to read the string.
- Initialize a variable called nWords with Zero(0). This nWords contain the total number of words in the string.
- To count the number of words in a string, Iterate over the string and Increment the nWords whenever a space character occurs.
- Start the For Loop by initializing
i to
Zero(0). It will continue looping as long as the current character (
str[i]) is not a null character (
'\0'). At each iteration,
i is incremented by
1.
- For each iteration, Check if the str[i] is equal to space ( ' '). Use if(str[i] == ' '). We can check for tabs and newlines depending on our requirements or we can simply use the isspace() function from ctype.h header file.
- If the above condition is true, Then increment the nWords variable by 1. – i.e nWords++;
- Once the above loop is completed, Check if the i value is greater than Zero(0), If so increment the nWords by 1. ( Because we need to increment for the first word).
- Finally, Display the result on the console using printf() function.
📢 This method will fail, If your input string contains extra spaces ( Leading, Trailing, or in between words)
Basic Method: Count the Number of words in a string in C Program:
Here is the program to count words in c.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
/*     program Count Number of words in string in c     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare input strings with 'SIZE'     char str[SIZE];     int i;      // Take the string from the user     printf("Enter a string : ");     gets(str);      // initialize 'nWords' with zero     int nWords = 0;      for(i = 0; i<strlen(str); i++)     { // For each character check if the present character is space         if(str[i] == ' ')         { // increment the 'nWords' counter             nWords++;         }     }      // only update the 'nWords' if string has atleast one character.     if(i)     {         // As we are starting the 'nWords' with zero.         nWords++;      }      // print the results     printf("Number of words in the string is : %d\n", nWords);     return 0; } |
Program Output:
Compile and Run the above program.
Test Case 1:
1 2 3 4 5 6 7 8 9 10 |
$ gcc count-words.c $ ./a.out Enter a string : The intelligent investor Number of words in the string is : 3 $ $ ./a.out Enter a string : Learn C Programming Online at SillyCodes.com Number of words in the string is : 6 $ $ |
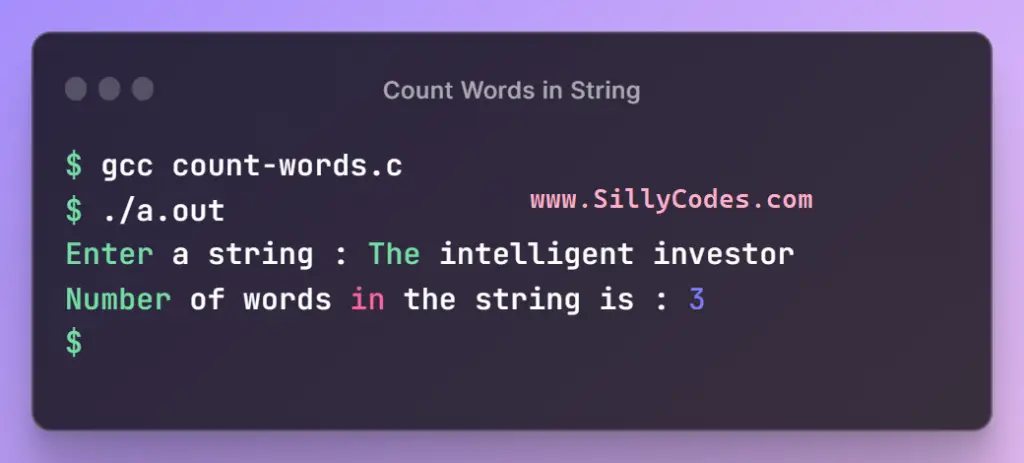
As we can see from the above output, The program is properly calculating the words in a string if the input string doesn’t have any extra spaces.
Test Case 2: String with extra spaces:
1 2 3 4 |
$ ./a.out Enter a string : Lot    of      spaces    tabs Number of words in the string is : 17 $ |
As the input string has extra spaces, The program generated incorrect results. To overcome this problem we need to use the following improved method.
Improved Method: Count Number of Words in String with extra spaces in C:
In this method, The program will count the number of words in a string, Even though the string has extra spaces.
We should be able to count the number of words for
- Normal string
- String with spaces in between the words
- String with trailing spaces
- String with leading spaces.
Let’s look at the program Algorithm/Explanation.
Count Number of words in a string with extra spaces Program Algorithm:
- As usual, Take the input string from the user and update the str string. Also initialize the nWords variable with Zero(0).
- To calculate the number of words in string, Traverse through the string character by character and increment the nWords only if the str[i] is not equal to space( ' ') and str[i+1] is equal to space( ' ') or NULL character.
- Start the for loop from
i = 0 and go till we reach the end of the string. i.e
for(i = 0; str[i]; i++)
- At Each Iteration, Check the if( str[i] != ‘ ‘ && (str[i+1] == ‘ ‘ || str[i+1] == ‘\0’) ) condition. If this condition is true, Then increment the nWords – i.e nWords++;
- Otherwise, Continue to the next iteration.
- Once the above Step 3 is completed, The nWords will have the total number of words in the input string.
- Display the nWords on the console.
Program to count words in string with extra spaces:
Let’s convert above algorithm into the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/*     program Count Number of words in string in c (works with extra spaces)     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare input strings with 'SIZE'     char str[SIZE];     int i;      // Take the string from the user     printf("Enter a string : ");     gets(str);      // initialize 'nWords' with zero     int nWords = 0;      // Iterage over the string.     for(i = 0; str[i]; i++)     {         // For each character check if the present character is space         // and next character is not a space or NULL.         if( str[i] != ' ' && (str[i+1] == ' ' || str[i+1] == '\0') )         {             // increment the 'nWords'             nWords++;         }     }      // print the results     printf("Number of words in the string is : %d\n", nWords);     return 0; } |
Program Output:
Compile the program using GCC(Any compiler)
$ gcc count-words-adv.c
Run the program.
Test Case 1: Normal String
1 2 3 4 |
$ ./a.out Enter a string : Programming is Fun Number of words in the string is : 3 $ |
The input string Programming is Fun contains three words.
Test Case 2: String with Extra Spaces:
1 2 3 4 |
$ ./a.out Enter a string : Lot    of    extra  spaces  between    words Number of words in the string is : 6 $ |
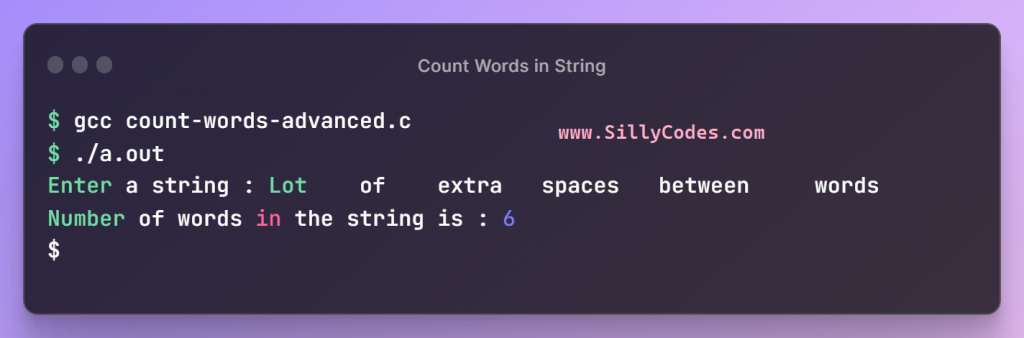
In this case, The input string Lot      of      extra      spaces        between      words has many extra spaces in between the words. Still, the program is able to calculate the number of words in the string properly and returned the result as 6.
Test Case 3: String with Leading Spaces:
1 2 3 4 |
$ ./a.out Enter a string :        leading    spaces    string Number of words in the string is : 3 $ |
In this method, The input string has the leading spaces, The program properly counted the number of words and returned the result.
Test Case 4: String with Trailing Spaces:
1 2 3 4 |
$ ./a.out Enter a string : trailing    spaces    string        Number of words in the string is : 3 $ |
The input string trailing      spaces    string has spaces in between the words and also the trailing spaces. The program counted the number words and returned the result as the 3.
As we can see from the above test cases, The program is providing the desired results.
User-defined Function: Program to Count Number of words in a string using functions in C Language:
Let’s move the above word counting logic from the main() function to a separate function. So that we can reuse the code.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the modified program, Where we defined a function called countWords to count the number of words in a string.
This function takes a string as the input and counts the total number of words in the string and returns the result back to the caller.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/*     program Count Number of words in string in c using function     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  /** * @brief  - count number of words in string * * @param str - input string * @return int - returns number of words in string */ int countWords(char * str) {     // initialize 'nWords' with zero     int nWords = 0;     int i;      // Iterage over the string.     for(i = 0; str[i]; i++)     {         // For each character check if the present character is space         // and next character is not a space or NULL.         if( str[i] != ' ' && (str[i+1] == ' ' || str[i+1] == '\0') )         {             // increment the 'nWords'             nWords++;         }     }      // return the 'nWords'     return nWords; }  int main() {      // declare input strings with 'SIZE'     char str[SIZE];      // Take the string from the user     printf("Enter a string : ");     gets(str);      // call the 'countWords()' function with inputstring.     int wordCnt = countWords(str);         // print results     printf("Number of words in the string is : %d\n", wordCnt);      return 0; } |
The prototype of the countWords function
int countWords(char * str)
Program Output:
Let’s compile and run the program and observe the output.
1 2 3 4 5 6 7 8 9 |
$ gcc countWords-func.c $ ./a.out Enter a string : Learn    Programming    Online Number of words in the string is : 3 $ $ ./a.out Enter a string :            count    words  in    string    Number of words in the string is : 4 $ |
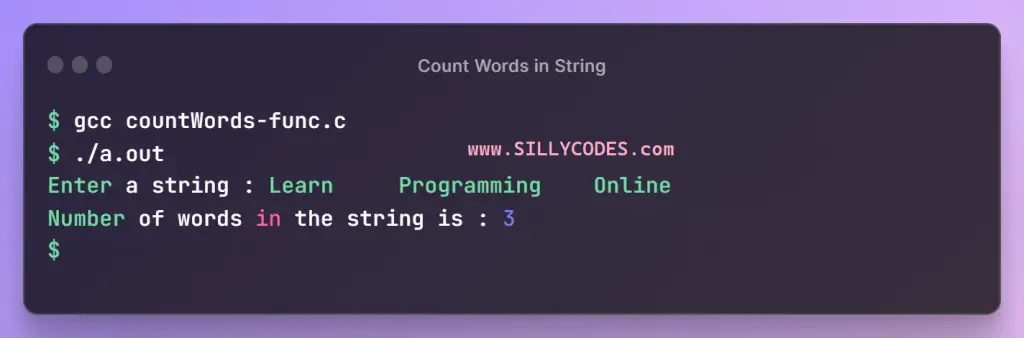
As we can see from the above input and outputs, The program is giving the desired results.
strtok library function: Count Number of Words in a String in C using strtok function:
We can also use the strtok() library function to count the number of words in a string. This method demonstrates the same.
The syntax of strtok function is
char * strtok(char * str, const char * delimiter)
The strtok() function parses the input string str into the sequence of tokens based on the delimiter.
The first call to strtok() function should be input string str and delimiter. But for the subsequent calls to strtok() that parses the same string the string( str) should be NULL.
Now, We know how strtok() function is used to split the string into the tokens. Let’s use this strtok() function to count the number of words in the string.
Call the strtok() function with input string and delimiter space( " "). Note this space is a string, not a character.
The strtok() function returns a character pointer. store it in ptr. Then use a while loop to make subsequent calls to strtok() function and increment the wordCnt variable.
The wordCntvariable will be initialized with zero and will be incremented by 1 at each iteration of the above while loop.
Here is the program to count number of words in a string using the strtok() function in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
/*     program Count Number of words in string in c using function     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare input strings with 'SIZE'     char str[SIZE];      // Initialize 'wordCnt' with zero.     int wordCnt = 0;      // Take the string from the user     printf("Enter a string : ");     gets(str);      char* ptr = strtok(str, " ");      while(ptr)     {         wordCnt++;         ptr = strtok(NULL, " ");     }      // print results     printf("Number of words in the string is : %d\n", wordCnt);      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 8 9 |
$ gcc count-words-strtok.c $ ./a.out Enter a string :      Deleted    code    is      debugged    code    Number of words in the string is : 5 $ $ ./a.out Enter a string : Who built pyramids? Number of words in the string is : 3 $ |
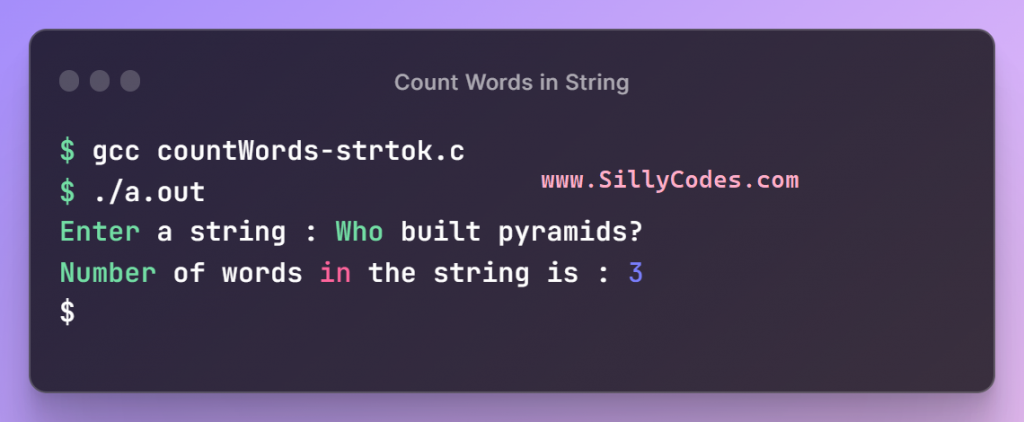
As we can see from the above output, The input string Who built pyramids? contains three words and strtok() function is able to properly count the number of words in the string.
Related String Programs:
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
- C Program to Find All Occurrences of a character in string
- C Program to Count All Occurrences of character in string
- C Program to Remove First Occurrence of character from string
- C Program to Remove Last Occurrence of character from string
- C Program to Remove All Occurrences of character from string
2 Responses
[…] C Program to Count Number of Words in a String […]
[…] C Program to Count Number of Words in a String […]