Program to Remove Consonants from String in C
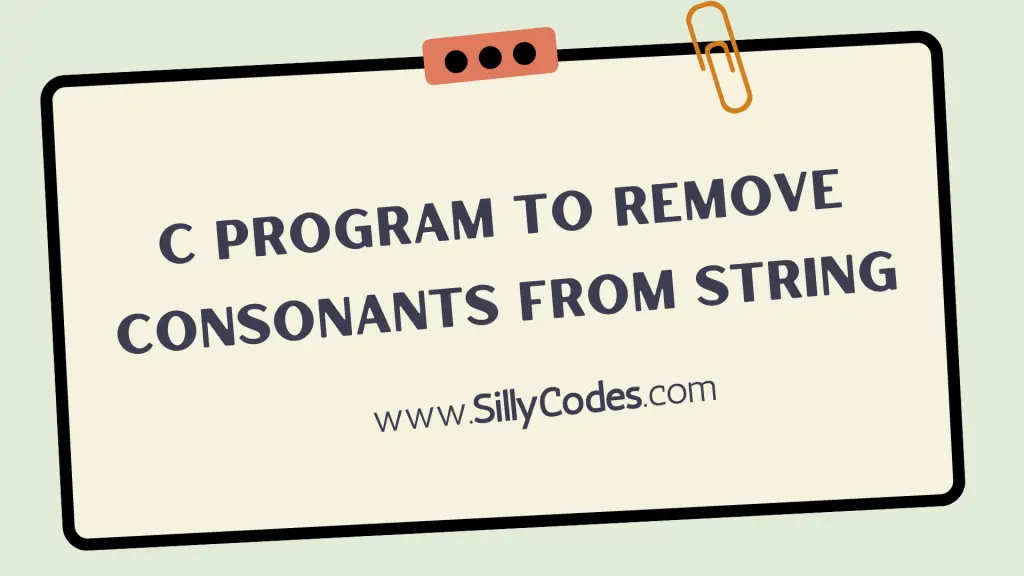
Program Description:
Write a Program to Remove Consonants from String in C programming language. The program should accept a string from the user and removes all consonants from the string and returns the results back to the user.
Other characters should not be altered by the program. If the input string contains spaces, numbers, or special characters, the program will keep them all and just remove the consonants.
📢 This Program is part of the C String Practice Programs series
We are going to look at different methods to Remove the consonants from a string in C Language. Each program will be followed by a detailed step-by-step explanation and program output. We also provided instructions for compiling and running the program using your favorite compiler.
Prerequisites:
It is recommended to know the basics of C-Strings, C-Arrays, and Functions in C to understand the following program better.
Example Input and Output:
Input:
Enter a string : Learn Programming Online
Output:
String before removing consonants :Learn Programming Online(Actual string)
String after removing consonants :ea oai Oie(resultant string)
The input string Learn Programming Online and the program removed all consonants and returned the ea oai Oie.
We will look at the two different methods to Remove Consonants from input string in C Language.
- Iterative Method
- Using User-defined Functions
Remove Consonants from String in C Program Explanation:
- Start the program by declaring the input string( inputStr) with the size of SIZE character. The SIZE is a global constant, which holds the max size of inputStr
- Declare two variables i and j. These two variables are used as indices. The i variable is used to iterate through the inputStr and the j variable is used to update the inputStr. We will call the variable i as the read index and the variable j as the write index. (‘i’-readIndex, ‘j’-writeIndex)
- Take the input string from the user and update the inputStr string using the gets function.
- To Remove Consonants from a string, Traverse through the inputStr and check all characters in the string and only update the character in the modified string if the character is not a consonant.
- Start the For Loop by initializing variables i and j with 0. It will continue looping as long as the current character (inputStr[i]) is not a NULL character (‘\0’).
- At each iteration, Inside the for loop, we check if the present character ( inputStr[i]) is a consonant. If it is not a consonant, Then it should be Vowel or digit or special character, etc. So Copy the character to the next position in the modified string ( inputStr[j]) at the write index j.
- Once the For loop is finished, Add the Terminating NULL character to the modified string inputStr at the write index ( j). – i.e inputStr[j] = '\0';
- Finally, Display the resultant string on the console using a printffunction.
Use the following condition to confirm the current character is not a consonant
1 2 3 |
(!isalpha(inputStr[i]) || inputStr[i] == 'a' || inputStr[i] == 'e' || inputStr[i] == 'i' || inputStr[i] == 'o' || inputStr[i] == 'u' || inputStr[i] == 'A' || inputStr[i] == 'E' || inputStr[i] == 'I' || inputStr[i] == 'O' || inputStr[i] == 'U') |
We are using the isalpha() function from the ctype.h header file.
Program to Remove Consonants from String in C Language:
Let’s convert the above algorithm into the C Program, Which will remove all consonants in the given string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
/*     program to Remove Consonants in a string in C     sillycodes.com */  #include<stdio.h> #include<ctype.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a inputString with 'SIZE'     char inputStr[SIZE];     int i, j;      // get the string from the user     printf("Enter a string : ");     gets(inputStr);      printf("String before removing consonants :%s(Actual string)\n", inputStr);      // Iterate over the string     // maintain two indices 'i'-readIndex, 'j'-writeIndex     for (i = 0, j = 0; inputStr[i]; ++i)     {         // If character is not a alphabet. Keep it in string. Copy it to writeIndex('j') position in the modified string.         // If character is Vowel, Keep it in string - Copy it to writeIndex('j') position in the modified string.         // If it is not a Vowel. Skip the character i.e continue to next step         if (!isalpha(inputStr[i]) ||                 inputStr[i] == 'a' || inputStr[i] == 'e' || inputStr[i] == 'i' || inputStr[i] == 'o' || inputStr[i] == 'u' ||                 inputStr[i] == 'A' || inputStr[i] == 'E' || inputStr[i] == 'I' || inputStr[i] == 'O' || inputStr[i] == 'U')         {             // present character is not a Consonant - So keep it in the string.             inputStr[j++] = inputStr[i];         }      }         // Add the Terminating NULL Character at the end of inputStr string.     inputStr[j] = '\0';      // Print Results     printf("String after removing consonants :%s(resultant string)\n", inputStr);      return 0; } |
📢 Don’t forget to include the ctype.h header file.
Program Output:
Let’s compile and run the program using GCC compiler (Any compiler)
Test Case 1: Normal characters
1 2 3 4 5 6 |
$ gcc remove-consonants.c $ ./a.out Enter a string : Learn Programming Online String before removing consonants :Learn Programming Online(Actual string) String after removing consonants :ea oai Oie(resultant string) $ |
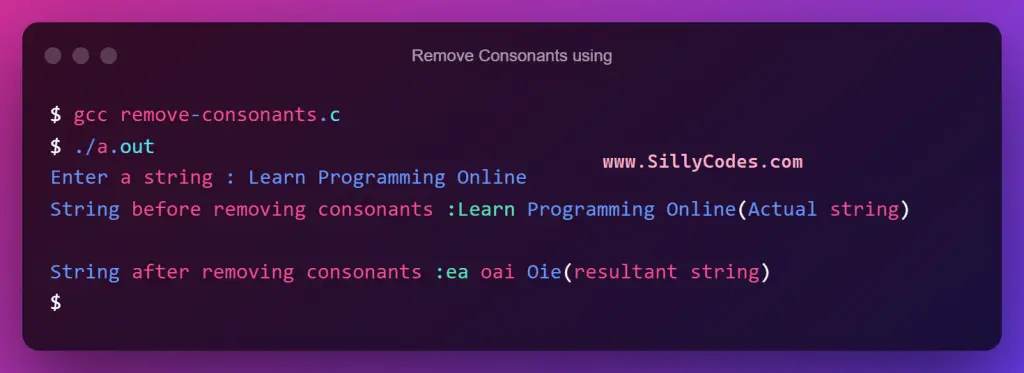
1 2 3 4 5 |
$ ./a.out Enter a string : sillycodes.com String before removing consonants :sillycodes.com(Actual string) String after removing consonants :ioe.o(resultant string) $ |
The Program is properly removing the consonants from the string. For example, if the input string is sillycodes.com, Then the program removed all consonants and returned the string ioe.o
Test Case 2: If the input string contains the numbers and the special characters,
1 2 3 4 5 6 |
// With Numbers and Special character $ ./a.out Enter a string : Test 1723 STRING ?#$& 1 String before removing consonants :Test 1723 STRING ?#$& 1(Actual string) String after removing consonants :e 1723 I ?#$& 1(resultant string) $ |
In this case, the input string (Test 1723 STRING ?#$& 1) contains the numbers and the special characters, and program removed the consonants and doesn’t change the special characters and numbers.
Remove Consonants from String using a user-defined function in C:
In the above program, We have written the entire program inside the main function. It is not a recommended method, Let’s rewrite the above program to use a function to remove the consonants from the string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits of using the functions in programming.
Here is the program to remove the consonants from a string using a user-defined function in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/*     program to Remove Consonants in a string in C using Functions.     sillycodes.com */  #include<stdio.h> #include<ctype.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Function Remove the Consonants from the 'str' (input string) * * @param str - input string */ void removeConsonants(char *str) {     int i, j;      // Iterate over the string     // maintain two indices 'i'-readIndex, 'j'-writeIndex     for (i = 0, j = 0; str[i]; ++i)     {         // If character is not a alphabet. Keep it in string. Copy it to writeIndex('j') position in the modified string.         // If character is Vowel, Keep it in string - Copy it to writeIndex('j') position in the modified string.         // If it is not a Vowel. Skip the character i.e continue to next step         if (!isalpha(str[i]) ||                 str[i] == 'a' || str[i] == 'e' || str[i] == 'i' || str[i] == 'o' || str[i] == 'u' ||                 str[i] == 'A' || str[i] == 'E' || str[i] == 'I' || str[i] == 'O' || str[i] == 'U')         {             // present character is not a Consonant - So keep it in the string.             str[j++] = str[i];         }      }         // Add the Terminating NULL Character at the end of str string.     str[j] = '\0'; }  int main() {      // declare a string with 'SIZE'     char str[SIZE];     int i, j;      // get the string from the user     printf("Enter a string : ");     gets(str);      printf("String before removing Consonants :%s(Actual string)\n", str);      // call the removeConsonants() function with 'str'     removeConsonants(str);      // Print Results     printf("String after removing Consonants :%s(resultant string)\n", str);      return 0; } |
We have defined a function named removeConsonants to remove the consonants from the string.
The Prototype of the removeConsonants() function. – void removeConsonants(char *str). This function takes the input string as the argument and removes all consonants from it.
Call the removeConsonants() function from the main() function and pass the input string( inputStr) to Remove the consonants from the string.
1 2 |
// call the removeConsonants() function with 'str' removeConsonants(str); |
As we are passing the string as the address, All the changes made in the removeConsonats function will be reflected in the main() function.
Program Output:
Compile and Run the Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc remove-consonants-func.c $ ./a.out Enter a string : mastering yourself is true power String before removing Consonants :mastering yourself is true power(Actual string) String after removing Consonants :aei oue i ue oe(resultant string) $ $ ./a.out Enter a string : sillycodes.com String before removing Consonants :sillycodes.com(Actual string) String after removing Consonants :ioe.o(resultant string) $ // with numbers and special characters $ ./a.out Enter a string : This includes numbers 123874 ?@&#*( special chars String before removing Consonants :This includes numbers 123874 ?@&#*( special chars(Actual string) String after removing Consonants :i iue ue 123874 ?@&#*( eia a(resultant string) $ |
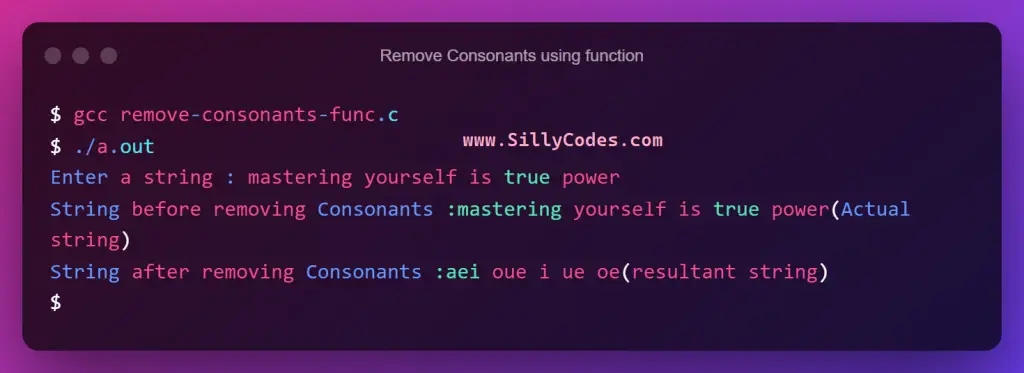
As we can see from the above examples, The removeConsonants() function is properly removing the consonants from the input string.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
1 Response
[…] C Program to Remove Consonants from a String […]