C Program to change case of alphabet | Change Case Program in C
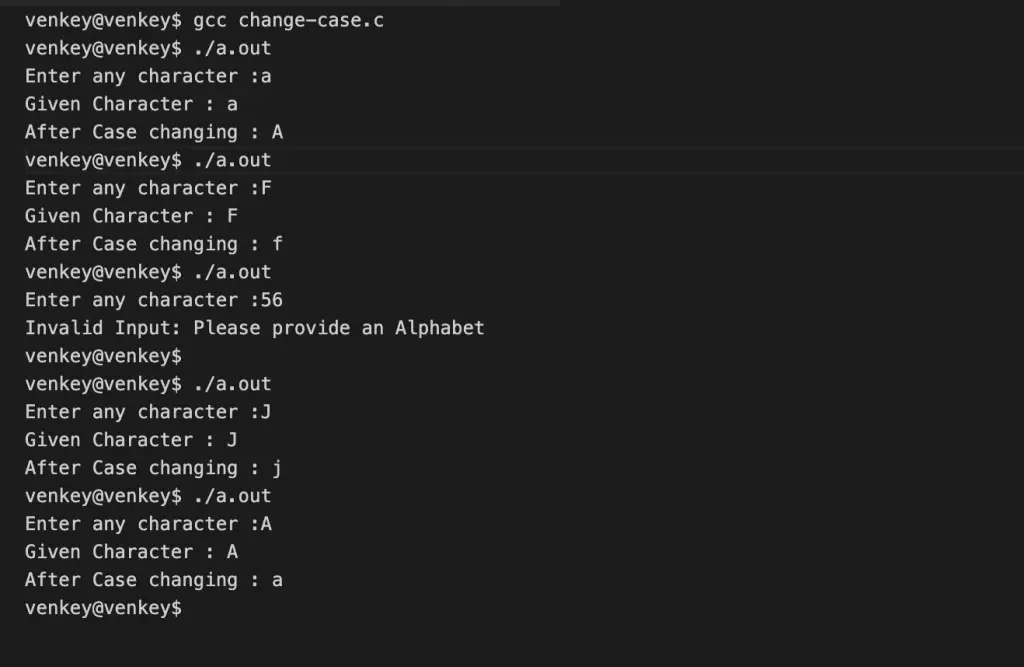
Program Introduction:
Write a C Program to change case of alphabet. The program shall take one alphabet as input and change the case of that alphabet.
If the user input is Upper case alphabet then program should convert it to Lower case alphabet.
If the user input is Lower case alphabet, Then Program should convert it to Upper case alphabet.
Here are couple of expected inputs and output
Example 1 :
User provided upper case ‘A’ alphabet. Our program should return lower case equivalent.
Input:
1 |
'A' |
Output:
1 |
'a' |
Example 2:
User can also provide lower case alphabet, Then we should return Upper case alphabet
Input:
1 |
'v' |
Output:
1 |
'V' |
Example 3:
If user input is not an alphabet, Then display an Error message and usage info.
Input:
1 |
100 |
Output:
1 |
"Invalid Input: Please provide an Alphabet" |
Program Logic: Change Case of the Alphabet:
As we discussed in the above description, Program will take any kind of input. Like Upper case alphabet, Lower Case alphabet, or even numeric value.
We are going to use the climit.h library to check the input type(alphabet or not) and Case of the alphabet (Is it lower-case, is it upper-case)
To change the case of alphabet, We are going to use one ASCII value trick, Which can invert the case of alphabet.
The ASCII value of Capital ‘A’ is 65, and the Lower case ‘a’ ASCII value is ’97’. Similarly ‘B’ ASCII Value is 66 and ‘b’ ASCII value is 98. The difference between the Lower case alphabet ASCII Value and Upper case alphabet ASCII value is 32.
📢 We have discussed all characters and their ASCII values in this post. C program to print all ASCII characters and ASCII values/numbers – SillyCodes
So, The Idea is If you want to change the case from the Upper Case alphabet to the Lower case alphabet. Then we need to add integer value 32 to the given character. By adding the integer value 32 to the Upper case alphabet will result in the Lower case alphabet.
Similarly, If you want to change the case from the Lower Case alphabet to the Upper case alphabet. Then we need to Subtract the value 32 to the given character. By removing the 32 from lower case character, will modify the character to Upper case character.
Change Case of Alphabet Program Algorithm:
- First of all, We will take the input from the user.
- Once we got the input, We will check if the given input is a valid character or not.
- We are going to use the
isalpha() function from the
ctype.hlibrary of C-Language.
- The isalpha() function takes one parameter. if the given parameter is character then It will return a non-zero integer. Otherwise (Not alphabet), The isalpha() will return zero (0)
- If the isalpha()function return value is zero then we will display the error message saying "Invalid Input: Please provide an Alphabet"
- If the isalpha() function returns a non-zero value, Means given input is character.
- Then we are going to check the input character case. So we will check the case of the alphabet using the
isupper()function.
- The isupper() function is used to check if the given alphabet is Upper case or not. If the given character is an Upper case character then isupper() will returns Non-zero integer. Otherwise, it will return the Zero (0) value.
- Once we got the case of the character, Then we need to change the case.
- To change the Case from Upper case to lower case, We will add the integer 32 to the given character.
- Similarly, To convert Lower case to upper case character, We need to subtract the value 32 from the character.
Here is the program for the above algorithm.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
#include<stdio.h> #include<ctype.h> int main() {     char ch;      // Take the input from the user and save it to 'ch' variable     printf("Enter any character :");     scanf("%c",&ch);      // Check if the given input is Alphabet or not.     // isaplha() function returns Non-zero for alphabet, Zero for non-alphabet     if(isalpha(ch))     {         // Given number is Alphabet.         // Check if the alphabet is Upper case or Lower case.         // We will use isupper() function, Which return Non-zero for Upper case         // Zero for Lower case         if(isupper(ch))         {             // Given Character is upper-case             // So we need to Add 32 to given alphabet.             // Ascii Values of A is 65 and a = 97, similarly B=66,b=98 so difference is 32              printf("Given Character : %c \n",ch);             printf("After Case changing : %c \n",ch+32);         }         else         {             // Given Character is Lower-case             // So we need to Substract 32 to given alphabet.             // Ascii Values of A is 65 and a = 97, similarly B=66,b=98 so difference is 32              printf("Given Character : %c \n",ch);             printf("After Case changing : %c \n",ch-32);         }     }     else     {         // Given Input is not a valid Alphabet. So display error message         printf("Invalid Input: Please provide an Alphabet \n");     }     return 0; } |
Program Change case of alphabet Output:
We are using gcc compiler in linux operating system.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
// Compile the program using GCC compiler venkey@venkey$ gcc change-case.c  // Run the executable file venkey@venkey$ ./a.out Enter any character :A Given Character : A After Case changing : a  // Example 2 venkey@venkey$ ./a.out Enter any character :B Given Character : B After Case changing : b  // Example 3 venkey@venkey$ ./a.out Enter any character :o Given Character : o After Case changing : O  // Example 4 venkey@venkey$ ./a.out Enter any character :g Given Character : g After Case changing : G  // Example 5 venkey@venkey$ ./a.out Enter any character :567 Invalid Input: Please provide an Alphabet venkey@venkey$ |
Conclusion:
In this article, We discussed about the case changing program using the ASCII value change.