Program to calculate the Remainder and Quotient in C Language
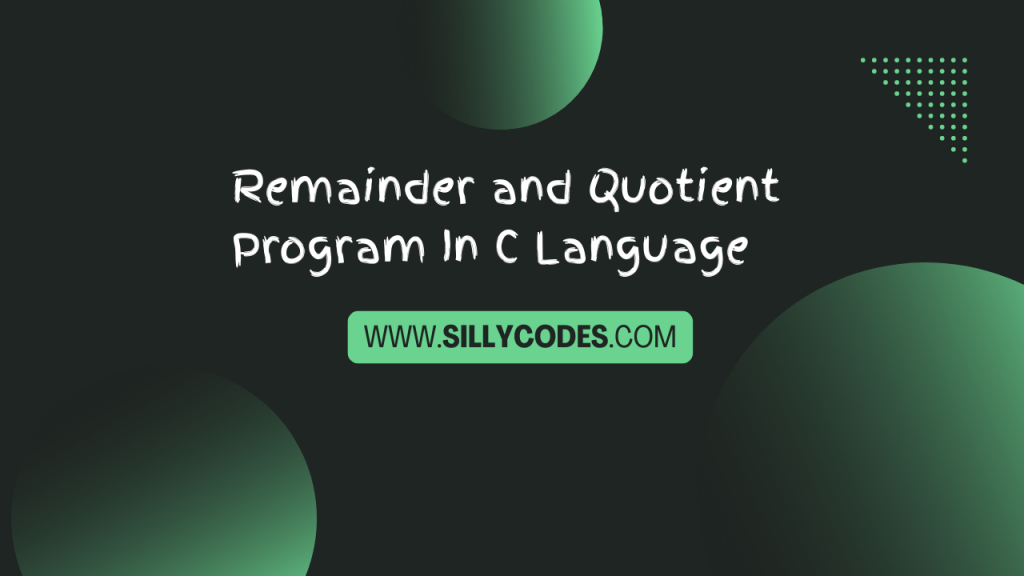
Remainder and Quotient in C Description:
Write a Program to calculate the Remainder and Quotient in C language. The program should accept two numbers from the user and calculate the Remainder and Quotient.
We should not allow the second number to be zero. Because if the second number is zero then we will get the Floating point exception.
📢 Don’t allow Floating point exceptions
Excepted Output:
Example 1:
Input:
1 |
Enter two Numbers : 23 5 |
Output:
1 |
Quotient: 4, Remainder: 3 |
Example 2:
Input:
1 |
Enter two Numbers : 100 10 |
Output:
1 |
Quotient: 10, Remainder: 0 |
Example 3:
Input:
1 |
Enter two Numbers : 21 0 |
Output:
1 |
ERROR: num2 is zero - Please provide valid numbers |
PreRequisites:
We need to know the basics of Division and modulo division operations and decision-making statements. Please look at the following articles to learn more about them
Remainder and Quotient in C Program Explanation:
- The program will accept two numbers from the user and store them in variables num1 and num2.
- Now we need to check if the num2 is zero, If num2 is zero, Then we can’t calculate the Remainder and Quotient of the num1 and num2.
- So Display the Error message and Prompt the user for new input.
- We are using the goto statement (Jump statement) to take the control back to the start of the program.
- If the num2 is not zero, Then we can calculate the Remainder and Quotient.
- We use the Division Operator to calculate the Quotient ( quo = num1 / num2)
- Then we calculate the Remainder using the Modulo division Operator. ( rem = num1 % num2)
- Finally, we print the result on the console.
Program – Remainder and Quotient in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/* Program: Program to calculate the Quotient and Remainder of two numbers Author: Sillycodes.com */ #include<stdio.h> int main() { int num1, num2, rem, quo; // take the input from the user INPUT: // goto statement label. printf("Enter two Numbers : "); scanf("%d%d", &num1, &num2); // If the `num2' is zero, // Then we will get 'Floating point exception'. // So check if 'num2' is zero if(num2 == 0) { printf("ERROR: num2 is zero - Please provide valid numbers \n"); goto INPUT; } else { // calculate the remainder and Quotient // using the division and modulo division operators rem = num1 % num2; quo = num1 / num2; printf("Quotient: %d, Remainder: %d\n", quo, rem); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
$ gcc remainder-quo.c $ ./a.out Enter two Numbers : 23 5 Quotient: 4, Remainder: 3 $ ./a.out Enter two Numbers : 100 10 Quotient: 10, Remainder: 0 $ ./a.out Enter two Numbers : 73 12 Quotient: 6, Remainder: 1 $ ./a.out Enter two Numbers : 99 8 Quotient: 12, Remainder: 3 $ ./a.out Enter two Numbers : 21 0 ERROR: num2 is zero - Please provide valid numbers Enter two Numbers : 20 3 Quotient: 6, Remainder: 2 $ |
Related Programs:
- Roots of Quadratic Equation Program in C
- Minimum of three Numbers in C langauge
- Even or Odd Number Program in C
1 Response
[…] C Program to Calculate the Remainder and Quotient […]