Number of days in Month Program in C Language
- Program Description:
- Excepted Output:
- Pre-Requisites:
- Number of Days in Month Program using if else ladder Explanation:
- Number of Days in Month Program using if else ladder:
- Program Output:
- Number of Days in Month Program using if else statement and Logical OR Operator:
- Program:
- Program Output:
- Program to calculate the Number of days in Month using the switch statement:
- Program Output:
- Related Programs:
- C Tutorials Index
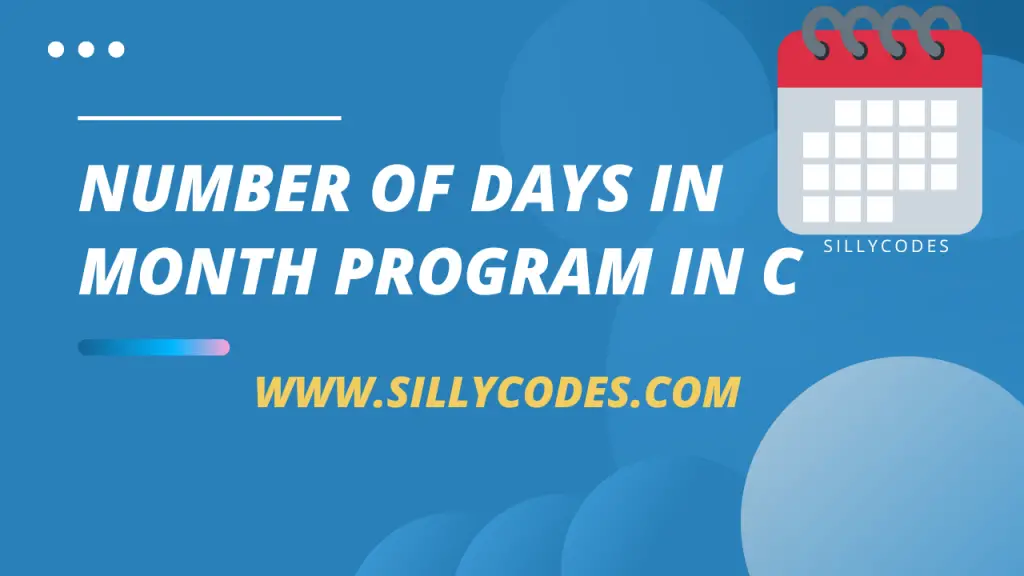
Program Description:
Write a C Program to display the Number of days in Month. The program should accept the month from the user in the form of a number. i.e 1 for January, 2 for February, 3 for March, and so on until 12 for December.
If the user enters February ( 2), Then the program should ask the year as well. Because the number of days in February depends on the leap year. so we also need the year.
Excepted Output:
Example 1: Input:
Enter a Month (1 for Jan, 2 - Feb, … 12 - Dec) : 10
Output:
October month contains 31 days
Example 2: Input:
Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 4
Output:
April month contains 30 days
Example 3: Input:
Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2
As the user enter 2 i.e Feb, We also ask for the year
Enter Year: 2022
Output:
Non Leap Year - February month contains 28 days
Pre-Requisites:
It is recommended to have the basic knowledge of the decision making statements like if else statements and if else ladder, and Logical Operator and switch case.
Please go through the following article to learn more about the above topics.
- Decision making statements if and if…else in C
- Nested if else and if..else ladder in C-Language
- Logical Operators
- Switch Statement in C Language
Number of Days in Month Program using if else ladder Explanation:
- The Program starts by asking the user for the month number ( 1 – Jan, 2 – Feb, etc) and the Program store the month number in a variable month
- We know that all months won’t contain the same number of days. Here is the list with the number of days
- January, March, May, July, August, October, and December – 31 days
- April, June, September, and November – 30 days
- February
- Leap Year – 29 days
- Non-Leap Year – 28 days
- Then we use the if else ladder to check the month. If the month is equal to 1, Then it is January, so print the number of days based above list. ( i.e 31 days)
- If the month is
February, Then we might have 28 or 29 days. So we ask the user to enter the
Year. Once the
year is entered we store it in
year variable.
- Then we calculate if the given number is a Leap Year using the Leap year formula. ((year%400 == 0) || ((year%4 == 0) && (year%100 != 0)))
- we have already covered the Leap year program in the following article – Please look at it for more details about the Leap year formula – https://sillycodes.com/c-program-to-check-given-year-is-leap/
- If the year is Leap year, Then we are printing Leap Year - February month contains 29 days
- If the year is not Leap year, Then we are displaying Non Leap Year - February month contains 28 days on the console.
- Similarly, we are displaying the number of days for each month.
- If the user enters an Invalid month number, Then we are displaying the error message – Please enter valid Number for month
Recommended Article:
Here is the program of the above logic.
Number of Days in Month Program using if else ladder:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 |
/* Program: Number of days in month in C Language */ #include<stdio.h> int main() { int month, year; // Prompt the user for Input printf("Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : "); scanf("%d", &month); if(month == 1) { // Jan printf("January month contains 31 days \n"); } else if(month == 2) { // Feb // Depending upon the leap year - Feb contains either 28 or 29 days // So we need year, so that we can check if it Leap year. // Ask user for the Year printf("Enter Year: "); scanf("%d", &year); // Leap year calculator forumula // learn more at - https://sillycodes.com/c-program-to-check-given-year-is-leap/ if ( (year%400 == 0) || ((year%4 == 0) && (year%100 != 0))) { // 'year' is leap year printf("Leap Year - February month contains 29 days \n"); } else { printf("Non Leap Year - February month contains 28 days \n"); } } else if(month == 3) { // March printf("March month contains 31 days \n"); } else if(month == 4) { // April printf("April month contains 30 days \n"); } else if(month == 5) { // May printf("May month contains 31 days \n"); } else if(month == 6) { // June printf("June month contains 30 days \n"); } else if(month == 7) { // July printf("July month contains 31 days \n"); } else if(month == 8) { // August printf("August month contains 31 days \n"); } else if(month == 9) { // Sept printf("September month contains 30 days \n"); } else if(month == 10) { // Oct printf("October month contains 31 days \n"); } else if(month == 11) { // Nov printf("November month contains 30 days \n"); } else if(month == 12) { // Dec printf("December month contains 31 days \n"); } else { // Invalid Number printf("Please enter valid Number for month \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
$ gcc days-in-month.c $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 10 October month contains 31 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 4 April month contains 30 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 29 Please enter valid Number for month $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 8 August month contains 31 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 2022 Non Leap Year - February month contains 28 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 2020 Leap Year - February month contains 29 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 100 Non Leap Year - February month contains 28 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 400 Leap Year - February month contains 29 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 1 January month contains 31 days $ |
Number of Days in Month Program using if else statement and Logical OR Operator:
If you observe the above program, There are many months that have the same number of days, So we can use the logical OR operator to group those months. So Let’s rewrite the above program by using the if-else statements and the logical operator.
Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
/* Program: Number of days in month in C Language */ #include<stdio.h> int main() { int month, year; // Prompt the user for Input printf("Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : "); scanf("%d", &month); if(month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) { // 31 days months printf("This month contains 31 days \n"); } else if(month == 4 || month == 6 || month == 9 || month == 11) { // 30 days months printf("This month contains 30 days \n"); } else if(month == 2) { // Feb // Depending upon the leap year - Feb contains either 28 or 29 days // So we need year, so that we can check if it Leap year. // Ask user for the Year printf("Enter Year: "); scanf("%d", &year); // Leap year calculator forumula // learn more at - https://sillycodes.com/c-program-to-check-given-year-is-leap/ if ( (year%400 == 0) || ((year%4 == 0) && (year%100 != 0))) { // 'year' is leap year printf("Leap Year - February month contains 29 days \n"); } else { printf("Non Leap Year - February month contains 28 days \n"); } } else { // Invalid Number printf("Please enter valid Number for month \n"); } } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
$ gcc days-in-month-logical.c $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 12 This month contains 31 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 5 This month contains 31 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 4 This month contains 30 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 2016 Leap Year - February month contains 29 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 2022 Non Leap Year - February month contains 28 days $ |
Program to calculate the Number of days in Month using the switch statement:
We can further change the above program and re-write it using the switch statement. The program logic/algorithm remains the same but it becomes more readable compared above two versions.
Here is the program with a switch statement.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
/* Program: Number of days in month in C using switch case/statement */ #include<stdio.h> int main() { int month, year; // Prompt the user for Input printf("Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : "); scanf("%d", &month); switch(month) { case 1: case 3: case 5: case 7: case 8: case 10: case 12: // 31 days months printf("This month contains 31 days \n"); break; case 4: case 6: case 9: case 11: // 30 days months printf("This month contains 30 days \n"); break; case 2: // Feb - depends on year // Depending upon the leap year - Feb contains either 28 or 29 days // So we need year, so that we can check if it Leap year. // Ask user for the Year printf("Enter Year: "); scanf("%d", &year); // Leap year calculator forumula // learn more at - https://sillycodes.com/c-program-to-check-given-year-is-leap/ if ( (year%400 == 0) || ((year%4 == 0) && (year%100 != 0))) { // 'year' is leap year printf("Leap Year - February month contains 29 days \n"); } else { printf("Non Leap Year - February month contains 28 days \n"); } break; default: printf("Invalid Month : Please provide valid month number \n"); break; } } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
$ gcc days-in-month-switch.c $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 7 This month contains 31 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 9 This month contains 30 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 2004 Leap Year - February month contains 29 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 100 Non Leap Year - February month contains 28 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 2 Enter Year: 400 Leap Year - February month contains 29 days $ ./a.out Enter a Month (1 for Jan, 2 - Feb, ... 12 - Dec) : 12 This month contains 31 days $ |
As you can see from the above output, We get the same output as the previous two version.
📢 Please make sure to use the break statement appropriately otherwise the subsequent case also executes.
Related Programs:
- Roots of a Quadratic Equation
- Calculate Remainder and Quotient in C
- Grade of the Students Program
- Pyramid and Star Pattern Programs in C
2 Responses
[…] C Program to calculate the Number of days in Month using the switch statement […]
[…] C Program to calculate the Number of days in Month using the switch statement […]