Factorial program in C Language
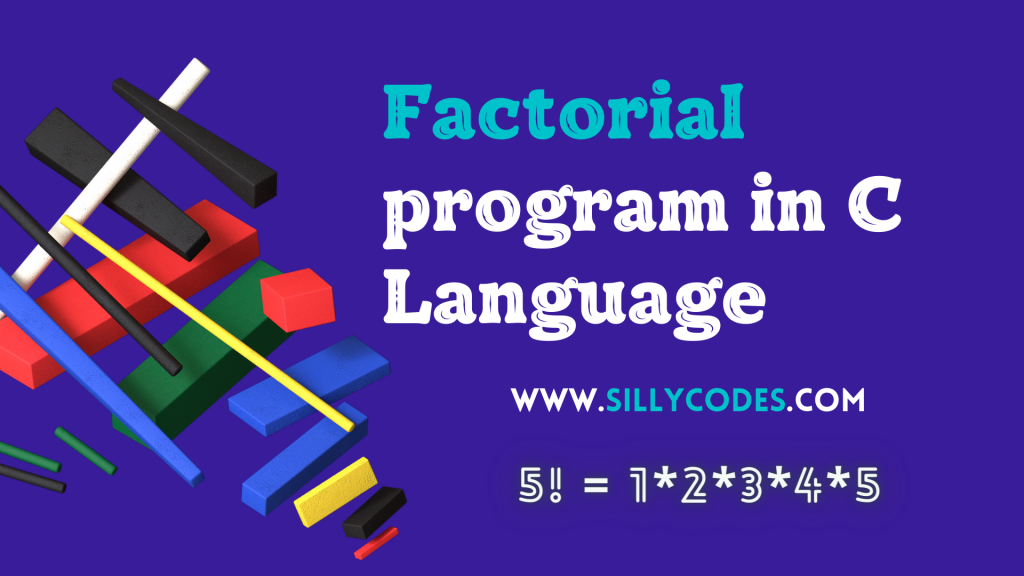
Program Description:
In this article, We are going to look at the Factorial program in C language. We are going to use the C Loops like for loop , while loop to calculate the prime number. The program should accept a positive number from the user and calculate the factorials of the number.
What is Factorial?
The factorial of positive integer n is denoted by n!
The Factorial is the product of all positive integers less than or equal to n. ( product of all numbers from 1 to n )
For example, a Factorial of a number 5 is
5! = 1 * 2 * 3 * 4 * 5 = 120
📢 The Factorial of Zero (0) is equal to 1
Factorial Program Example Input and Output:
Input:
Enter a Positive Number : 6
Output:
Factorial of 6 is : 720
The program should also handle invalid input cases.
Pre-Requisites:
It is recommended to know the basics of the C Loops and Goto statement. Please go through the following articles to understand more about them
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
- Goto Statement in C Language
Factorial Program in C Algorithm:
- Take the input number from the user. And we store it in a variable named n
- Check if the
n is positive or negative. As there is no factorial for negative numbers, Display the error message (
No Factorial for Negative Numbers, Please try again) if the user enters a negative number. Also, allow the user to input the value again.
- We use the Goto Statement to jump back to the start of the program. To achieve this, We use the goto START; ( at line 20) and START; (at line 11) Label.
- Now the
n is a positive number. If the number
n is equal to
Zero(0), Then the factorial of
zero(0) is
1. Display the result and return.
- or you can even remove this check, by default we use the fact variable value as 1, and i value as 1. So if the user enters to check factorial, The loop won’t execute as condition i<=n will be False, So we simply print the default fact number which is 1. )
- If the number is any other positive number. We use the Loop to calculate the Factorial of the number.
- The main Idea is to Multiply all numbers from 1 to n by iterating from numbers 1 to n
- The Loop starts with the number
1 (We can start from
2) and goes all the way up to the number
n
- At Each Iteration, We multiply the fact variable(which is initialized with 1) with the present loop control variable i. fact = fact * i;
- The above loop will continue until the loop condition i < = n becomes False.
- Once the loop is terminated, The fact variable contains the Factorial of the given number n
Let’s convert the above Pseudo code into the C program.
Factorial program in C language with for loop:
Let’s use the for loop to calculate the factorial of the number
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program: Factorial program in C     Author: sillyCodes.com */ #include<stdio.h> int main() {     // define variables     int n,fact=1,i;     START:    // goto statement label.     // Take the input from the user     printf("Enter a Positive Number : ");     scanf("%d",&n);         // check if the 'n' is Negative.     if(n<0)     {         printf("No Factorial for Negative Numbers, Please try again \n");         goto START;  // Takes control backs to 'START' label.     }      // Factorial of '0' is '1'     if(n == 0)         fact = 1;     else     {         // Calculate the factorial using for loop.         for(i=1;i<=n;i++)         {             // multiply all numbers from 1 to 'n'             fact = fact*i;         }     }         printf("Factorial of %d is : %d\n",n,fact);     return 0; } |
We can even change the above program by removing the if (n == 0) check like below, As the default value of the fact variable is 1, So if the user provides , the for loop won’t execute and we display the default value i.e 1.. Which also gives the same result.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/*     Program: Factorial program in C     Author: sillyCodes.com */ #include<stdio.h> int main() {     // define variables     int n,fact=1,i;     START:    // goto statement label.     // Take the input from the user     printf("Enter a Positive Number : ");     scanf("%d",&n);         // check if the 'n' is Negative.     if(n<0)     {         printf("No Factorial for Negative Numbers, Please try again \n");         goto START;  // Takes control backs to 'START' label.     }          // Calculate the factorial using for loop.     for(i=1;i<=n;i++)     {         // multiply all numbers from 1 to 'n'         fact = fact*i;     }         printf("Factorial of %d is : %d\n",n,fact);     return 0; } |
Program Output:
Let’s compile and run the program using the IDE. We are using the GCC compiler. You can learn more about the compile and run the program in Linux in the following articles
Compile the program
$ gcc factorial.c
Run the program:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Positive Number :Â Â 6 Factorial of 6 is : 720 Â $ ./a.out Enter a Positive Number :Â Â 3 Factorial of 3 is : 6 $ |
As we can see from the above output, We are getting the desired results and our program is able to calculate the Factorial of the given number.
What if the user enters a negative number? Let’s try to give the negative number to the program
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Positive Number :Â Â -6 No Factorial for Negative Numbers, Please try again Enter a Positive Number :Â Â -100 No Factorial for Negative Numbers, Please try again Enter a Positive Number :Â Â 10 Factorial of 10 is : 3628800 $ |
We can see that, The program displayed an error notice ( No Factorial for Negative Numbers, Please try again) when the user provided a negative number and asked for the number again. This process continues until user enters a positive number.
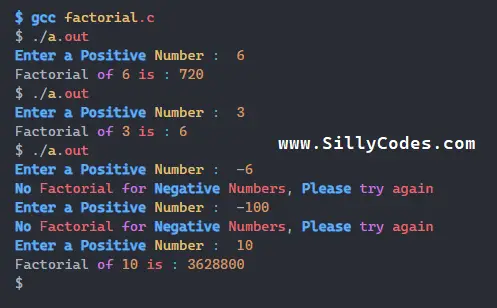
Factorial Program in C using the while loop:
We can also use the while loop to calculate the factorial of a number. The program algorithm is not going to change, Only change is we are going to use the while loop instead of the for loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/*     Program: Factorial program in C     Author: sillyCodes.com */ #include<stdio.h> int main() {     // define variables     int n,fact=1,i;     START:    // goto statement label.     // Take the input from the user     printf("Enter a Positive Number : ");     scanf("%d",&n);         // check if the 'n' is Negative.     if(n<0)     {         printf("No Factorial for Negative Numbers, Please try again \n");         goto START;  // Takes control backs to 'START' label.     }      // Calculate the factorial using while loop.     i = 1;     while(i <= n) {         // multiply all numbers from 1 to 'n'         fact = fact*i;         i++;     }         printf("Factorial of %d is : %d\n",n,fact);     return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc factorial-while.c $ ./a.out Enter a Positive Number : 8 Factorial of 8 is : 40320 $ ./a.out Enter a Positive Number : 4 Factorial of 4 is : 24 $ ./a.out Enter a Positive Number : -8 No Factorial for Negative Numbers, Please try again Enter a Positive Number : 9 Factorial of 9 is : 362880 $ |
As you can see from the above output, We are getting the desired output.
Factorial Program using do while loop:
Let’s change the program to use the do while loop instead of other loops.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/*     Program: Factorial program in C     Author: sillyCodes.com */ #include<stdio.h> int main() {     // define variables     int n,fact=1,i;     START:    // goto statement label.     // Take the input from the user     printf("Enter a Positive Number : ");     scanf("%d",&n);         // check if the 'n' is Negative.     if(n<0)     {         printf("No Factorial for Negative Numbers, Please try again \n");         goto START;  // Takes control backs to 'START' label.     }      // Calculate the factorial using do while loop.     i = 1;     do {         // multiply all numbers from 1 to 'n'         fact = fact*i;         i++;     } while(i <= n);             printf("Factorial of %d is : %d\n",n,fact);     return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ gcc factorial.c $ ./a.out Enter a Positive Number : 1 Factorial of 1 is : 1 $ ./a.out Enter a Positive Number : 0 Factorial of 0 is : 1 $ ./a.out Enter a Positive Number : 5 Factorial of 5 is : 120 $ ./a.out Enter a Positive Number : -6 No Factorial for Negative Numbers, Please try again Enter a Positive Number : 12 Factorial of 12 is : 479001600 $ |
Our program is working as expected.
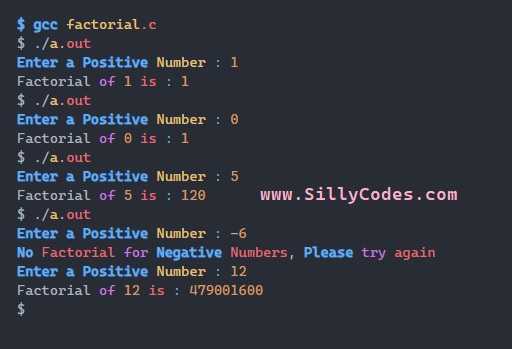
Practice Program:
- Try to write the above program without using the loops
📢 Hint: Use the Goto statement to repeat the code block
Related Programs:
- If else practice Programs
- Switch Case Practise Programs
- C program to test Leap yearÂ
- C program to Find Area and Perimeter of Rectangle.
- Calculate the Grade of Student.
- Temperature Conversion program in C. ( Centigrade to Fahrenheit ).
- Type Conversion Operators on Assignment operator.
- Prime Numbers up to n
- Calculate Sum of Digits Program in C
- Octal to Decimal Number Conversion
4 Responses
[…] C Program to Calculate the Factorial of Number […]
[…] Related Program: Calculate Factorial Program using iterative method ( Loops ) […]
[…] C Program to Calculate the Factorial of Number […]
[…] C Program to Calculate the Factorial of Number […]